"Mastering Higher-Order Functions and Loops in JavaScript: A Comprehensive Guide"

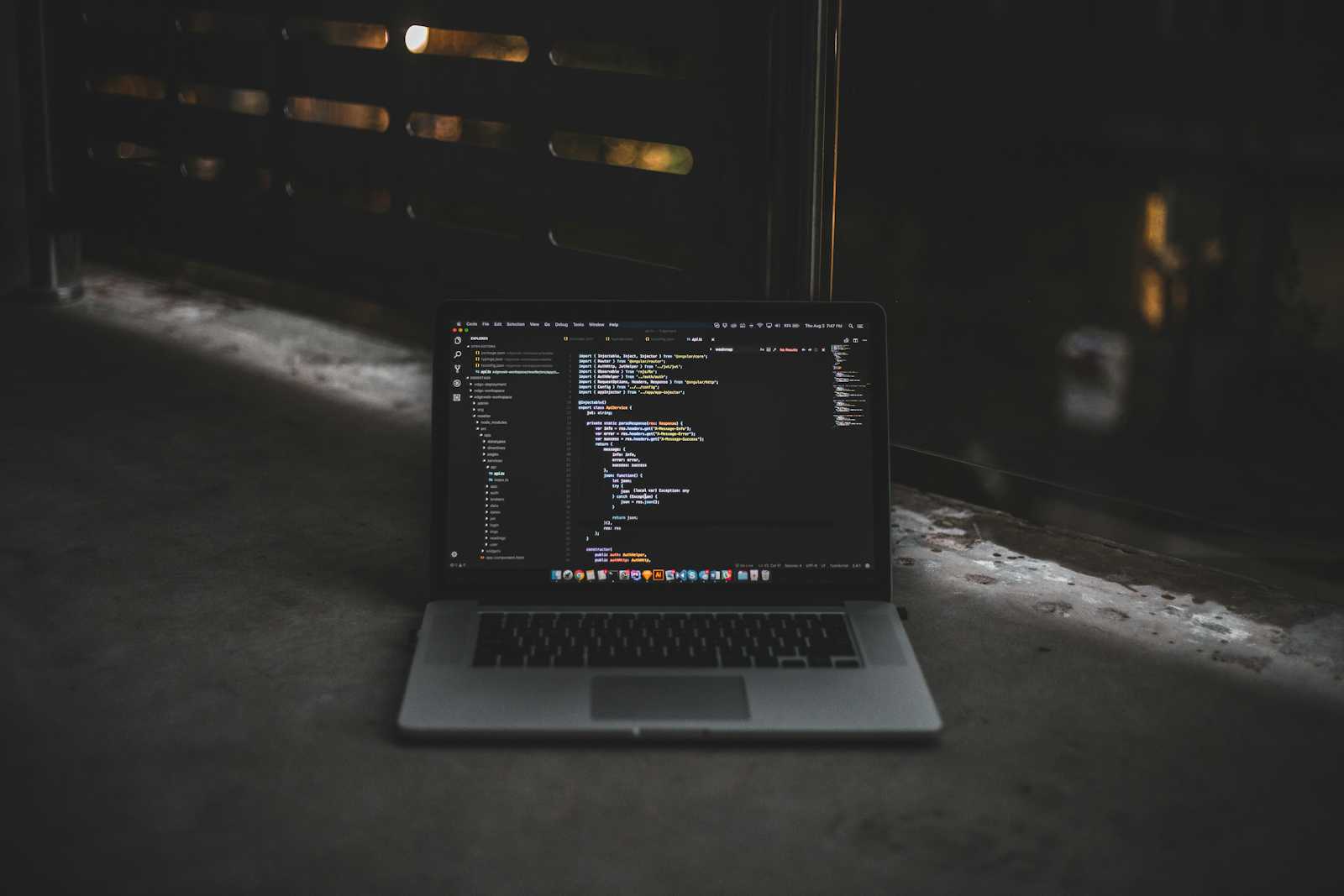
In JavaScript, higher-order functions are functions that can take other functions as arguments or return functions as their result. They are fundamental in functional programming and allow for powerful abstractions. Here are some of the most commonly used higher-order functions in JavaScript:
Array Higher-Order Functions
Array.prototype.forEach
Executes a provided function once for each array element.const array = [1, 2, 3]; array.forEach(value => console.log(value));
Array.prototype.map
Creates a new array populated with the results of calling a provided function on every element in the calling array.const array = [1, 2, 3]; const doubled = array.map(value => value * 2);
Array.prototype.filter
Creates a new array with all elements that pass the test implemented by the provided function.const array = [1, 2, 3]; const even = array.filter(value => value % 2 === 0);
Array.prototype.reduce
Executes a reducer function on each element of the array, resulting in a single output value.const array = [1, 2, 3]; const sum = array.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
Array.prototype.reduceRight
Applies a function against an accumulator and each value of the array (from right-to-left) to reduce it to a single value.const array = [1, 2, 3]; const sum = array.reduceRight((accumulator, currentValue) => accumulator + currentValue, 0);
Array.prototype.every
Tests whether all elements in the array pass the test implemented by the provided function.const array = [1, 2, 3]; const allEven = array.every(value => value % 2 === 0);
Array.prototype.some
Tests whether at least one element in the array passes the test implemented by the provided function.const array = [1, 2, 3]; const someEven = array.some(value => value % 2 === 0);
Array.prototype.find
Returns the value of the first element in the array that satisfies the provided testing function.const array = [1, 2, 3]; const found = array.find(value => value > 1);
Array.prototype.findIndex
Returns the index of the first element in the array that satisfies the provided testing function.const array = [1, 2, 3]; const index = array.findIndex(value => value > 1);
Array.prototype.flatMap
First maps each element using a mapping function, then flattens the result into a new array.const array = [1, 2, 3]; const flatMapped = array.flatMap(value => [value, value * 2]);
Array.prototype.sort
Sorts the elements of an array in place and returns the array.const array = [3, 1, 2]; array.sort((a, b) => a - b);
Array.prototype.flat
Creates a new array with all sub-array elements concatenated into it recursively up to the specified depth.const array = [1, [2, [3]]]; const flattened = array.flat(2);
Array.prototype.concat
Merges two or more arrays into a new array.const array1 = [1, 2]; const array2 = [3, 4]; const merged = array1.concat(array2);
Function Higher-Order Functions
Function.prototype.bind
Creates a new function that, when called, has itsthis
keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.const module = { x: 42, getX: function() { return this.x; } }; const unboundGetX = module.getX; const boundGetX = unboundGetX.bind(module); console.log(boundGetX()); // 42
Function.prototype.call
Calls a function with a giventhis
value and arguments provided individually.function greet() { console.log(`Hello, ${this.name}`); } const person = { name: 'John' }; greet.call(person); // Hello, John
Function.prototype.apply
Calls a function with a giventhis
value, and arguments provided as an array (or an array-like object).function greet(greeting) { console.log(`${greeting}, ${this.name}`); } const person = { name: 'John' }; greet.apply(person, ['Hello']); // Hello, John
Summary
In total, the primary higher-order functions in JavaScript can be categorized under array methods and function methods. These are:
Array Methods:
forEach
map
filter
reduce
reduceRight
every
some
find
findIndex
flatMap
sort
flat
concat
Function Methods:
bind
call
apply
These higher-order functions are fundamental tools in JavaScript, enabling powerful and expressive functional programming techniques.
Certainly! Besides higher-order functions and loops, there are several other important concepts and features in JavaScript that you should be familiar with. These include, but are not limited to, the following:
Mastering JavaScript: Essential Concepts and Features
1. Promises and Asynchronous Programming
Promises: Objects representing the eventual completion or failure of an asynchronous operation.
const promise = new Promise((resolve, reject) => { setTimeout(() => { resolve('Success!'); }, 1000); }); promise.then(result => { console.log(result); // 'Success!' }).catch(error => { console.error(error); });
Async/Await: Syntactic sugar over promises, making asynchronous code look synchronous.
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error(error); } } fetchData();
2. Modules
ES6 Modules: Using
import
andexport
to manage dependencies and organize code.// math.js export function add(a, b) { return a + b; } // main.js import { add } from './math.js'; console.log(add(2, 3)); // 5
3. Closures
Functions that have access to the outer (enclosing) function’s variables even after the outer function has returned.
function makeCounter() { let count = 0; return function() { count++; return count; }; } const counter = makeCounter(); console.log(counter()); // 1 console.log(counter()); // 2
4. Event Handling
Event Listeners: Responding to user interactions and other events in the DOM.
document.getElementById('myButton').addEventListener('click', () => { console.log('Button clicked!'); });
5. Error Handling
Try/Catch: Handling exceptions and errors gracefully.
try { throw new Error('Something went wrong'); } catch (error) { console.error(error.message); }
6. Destructuring
Extracting values from arrays or properties from objects into distinct variables.
const person = { name: 'John', age: 30 }; const { name, age } = person; console.log(name, age); // 'John', 30
7. Template Literals
Template strings with embedded expressions.
const name = 'John'; const message = `Hello, ${name}!`; console.log(message); // 'Hello, John!'
8. Spread and Rest Operators
Spread: Expanding elements of an iterable (like an array) or properties of an object.
const array = [1, 2, 3]; const newArray = [...array, 4, 5]; console.log(newArray); // [1, 2, 3, 4, 5]
Rest: Collecting arguments into an array.
function sum(...numbers) { return numbers.reduce((acc, curr) => acc + curr, 0); } console.log(sum(1, 2, 3, 4)); // 10
9. Classes and Inheritance
ES6 Classes: Creating objects and inheritance in a more familiar syntax compared to traditional prototypes.
class Person { constructor(name, age) { this.name = name; this.age = age; } greet() { console.log(`Hello, my name is ${this.name}`); } } class Student extends Person { constructor(name, age, grade) { super(name, age); this.grade = grade; } study() { console.log(`${this.name} is studying`); } } const student = new Student('John', 20, 'A'); student.greet(); // 'Hello, my name is John' student.study(); // 'John is studying'
10. Prototypes and Prototype Chain
Understanding how inheritance works in JavaScript using prototypes.
function Person(name, age) { this.name = name; this.age = age; } Person.prototype.greet = function() { console.log(`Hello, my name is ${this.name}`); }; const person = new Person('John', 30); person.greet(); // 'Hello, my name is John'
Types of For Loop in JavaScript
In JavaScript, there are several types of loops that you can use to iterate over arrays, objects, and other iterable structures. Here are the main ones:
1. for
Loop
The traditional for
loop is used for general-purpose iteration with a counter.
for (let i = 0; i < array.length; i++) {
console.log(array[i]);
}
2. for...in
Loop
The for...in
loop is used to iterate over the enumerable properties of an object.
const obj = {a: 1, b: 2, c: 3};
for (const key in obj) {
if (obj.hasOwnProperty(key)) {
console.log(key, obj[key]);
}
}
3. for...of
Loop
The for...of
loop is used to iterate over the values of an iterable (like an array, string, map, set, etc.).
const array = [1, 2, 3];
for (const value of array) {
console.log(value);
}
4. Array.prototype.forEach
Method
The forEach
method is used to execute a provided function once for each array element.
const array = [1, 2, 3];
array.forEach(value => {
console.log(value);
});
5. while
Loop
The while
loop is used to execute a block of code as long as a specified condition is true.
let i = 0;
while (i < array.length) {
console.log(array[i]);
i++;
}
6. do...while
Loop
The do...while
loop is similar to the while
loop, but it executes the block of code once before checking the condition.
let i = 0;
do {
console.log(array[i]);
i++;
} while (i < array.length);
Summary
In summary, the main types of loops in JavaScript are:
for
loopfor...in
loopfor...of
loopArray.prototype.forEach
methodwhile
loopdo...while
loop
Each loop type serves different purposes and can be chosen based on the specific requirements of your iteration task.
Mutable and Immutable in JS
In JavaScript, mutability refers to whether an object or value can be changed after it's created. Let's break down mutable and immutable elements in JS:
Mutable (can be changed):
Objects
Arrays
Functions
Sets
Maps
Immutable (cannot be changed):
Primitive values:
Numbers
Strings
Booleans
null
undefined
Symbol
frozen objects (using Object.freeze())
Key points:
When you assign a new value to a primitive, you're creating a new instance.
Modifying an object or array changes the original, not creates a new one.
const doesn't make objects immutable, it just prevents reassignment.
Mutable
A mutable object is one that can be changed after it is created. Most objects and arrays in JavaScript are mutable.
Examples of Mutable Types:
Objects:
- You can add, delete, or modify properties of an object.
javascriptCopy codeconst obj = { name: "Alice", age: 25 };
obj.age = 26; // Modify
obj.city = "New York"; // Add
delete obj.name; // Delete
console.log(obj); // Output: { age: 26, city: 'New York' }
Arrays:
- You can change elements, add new elements, or remove elements.
javascriptCopy codeconst arr = [1, 2, 3];
arr[0] = 10; // Modify
arr.push(4); // Add
arr.pop(); // Remove
console.log(arr); // Output: [10, 2, 3]
Immutable
An immutable object is one that, once created, cannot be changed. Primitive values (strings, numbers, booleans, null, undefined, and symbols) are immutable in JavaScript.
Examples of Immutable Types:
Strings:
- Strings cannot be changed after they are created. Any operation that appears to modify a string actually creates a new string.
javascriptCopy codelet str = "hello";
str[0] = 'H'; // This has no effect
str = "Hello"; // This creates a new string
console.log(str); // Output: "Hello"
Numbers, Booleans, Null, Undefined, Symbols:
- These types are immutable. Any operation on them that seems to change their value actually results in a new value.
javascriptCopy codelet num = 42;
num += 1; // This creates a new number 43
console.log(num); // Output: 43
Immutability in Practice
Although objects and arrays are mutable by default, there are techniques to create immutable versions of them:
Object.freeze
:- Freezes an object, making it immutable (no properties can be added, removed, or modified).
javascriptCopy codeconst obj = { name: "Alice", age: 25 };
Object.freeze(obj);
obj.age = 26; // This will have no effect
console.log(obj); // Output: { name: 'Alice', age: 25 }
Immutable Data Structures:
- Libraries like Immutable.js provide immutable data structures for JavaScript.
javascriptCopy codeconst { Map } = require('immutable');
let map1 = Map({ a: 1, b: 2, c: 3 });
let map2 = map1.set('b', 50); // map2 is a new Map, map1 remains unchanged
console.log(map1.get('b')); // Output: 2
console.log(map2.get('b')); // Output: 50
Copying and Updating:
- Instead of modifying an object or array directly, you can create a copy with the updated values.
javascriptCopy codeconst arr = [1, 2, 3];
const newArr = [...arr, 4]; // Create a new array by copying the old one and adding a new element
console.log(arr); // Output: [1, 2, 3]
console.log(newArr); // Output: [1, 2, 3, 4]
Understanding mutability and immutability is crucial for managing state in JavaScript applications, especially in frameworks like React where immutability helps in optimizing re-rendering and maintaining predictable state updates.
Subscribe to my newsletter
Read articles from Kumar Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
