Destructuring in Javascript

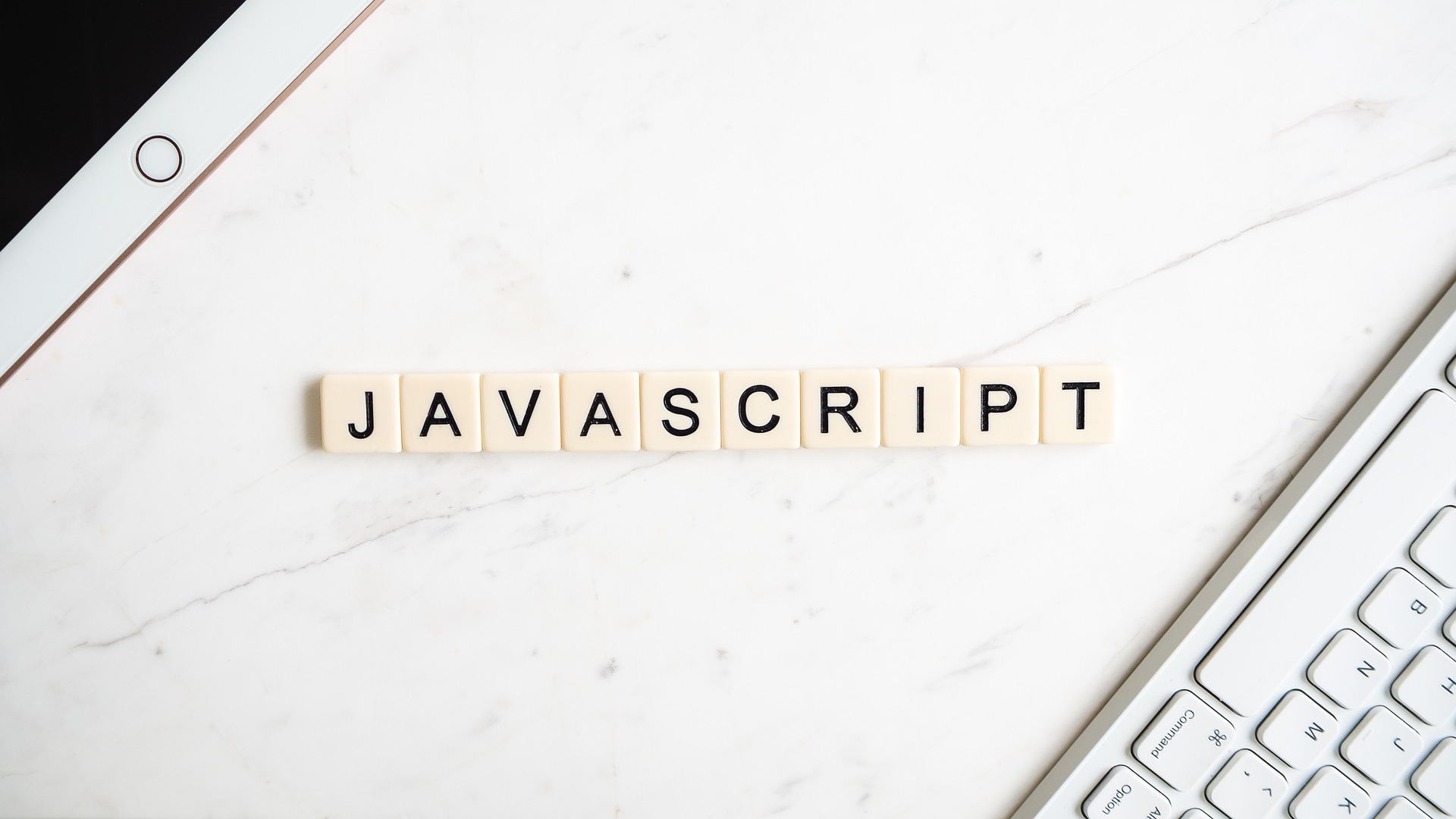
Table of Contents
Introduction
What is Destructuring
Array Destructuring
Object Destructuring
Conclusion
Destructuring in Javascript is a feature introduced in ES6 (ECMAScript 2015). It makes it easier to extract values from arrays or properties from objects and assign them to variables in a readable way.
What is Destructuring?
Destructuring is a technique that allows you to unpack values from arrays or objects into separate variables. This process involves breaking down complex data structures into simpler parts, making it easier to work with them.
Array Destructuring
Let's start with array destructuring. We'll use the following example. Without destructuring, extracting values from an array can be overly complex:
In this example, you're extracting the values from the myFavoriteMeal array and assigning them to variables firstMeal, secondMeal, thirdMeal,fourthMeal, respectively.
Skipping Elements from the Array
You can choose to ignore certain elements by omitting them from the destructuring pattern:
In this example, you're destructuring the myFavouriteMeal array but only assigning values to the firstMeal, secondMeal and fourthMeal of myFavouriteMeal variables. You're skipping the second element in the array by placing a comma without a variable name between secondMeal and fourthMeal. This allows you to extract specific elements from the array while ignoring others, providing more flexibility and control in your destructuring patterns.
Nested Array Destructuring
Array destructuring can also be nested. Here's an example:
In this code, we have a nested array called nestedArray. Using nested array destructuring, you're extracting values from both the outer and inner arrays and assigning them to variables firstValue, secondValue, thirdValue, and fourthValue.
How to Use Array Destructuring While Separating Variable Declarations from Their Assignments
Whenever you use array destructuring, JavaScript allows you to separate your variable declarations from their assignments.
Here’s an example:
What if you want "Temitope" assigned to the firstName variable, and the rest of the array items to another variable? How would you do that? Let’s find out below.
How to Use Array Destructuring to Assign the Rest of an Array Literal to a Variable
JavaScript allows you to use the rest operator within a destructuring array to assign the rest of a regular array to a variable.
Here’s an example:
Note: Always use the rest operator as the last item of your destructuring array to avoid getting a SyntaxError.
Now, what if you only want to extract "github.com", the technique you can use will be discussed below
.
How to Use Array Destructuring to Extract Values at Any Index
Here’s how you can use array destructuring to extract values at any index position of a regular array:
In the snippet above, we used commas to skip variables at the destructuring array's first and second index positions.
By so doing, we were able to link the website variable to the third index value of the regular array on the right side of the assignment operator (that is, "github.com").
At times, the value you wish to extract from an array is undefined. In that case, JavaScript provides a way to set default values in the destructuring array,set one inside a destructuring array.
watch the video below for more info on Array Destructuring
https://www.youtube.com/watch?v=UHZcJyVXtLo&pp=ygUZYXJyYXkgZGlzdHJ1Y3R1cmluZyBpbiBqcw%3D%3D
Object Destructuring
Moving on to object destructuring, consider the following object:
Regular Destructuring
Object destructuring allows you to extract properties from objects:
In this example, { name, age, city } is the destructuring syntax. It means you're extracting the name, age, and city properties from the person object and assigning them to variables of the same name. Therefore name will have the value "Adams Smith”, age will be 42, and city will have "Arizona".
Furthermore, { hobbies, occupation } is also the destructuring syntax, which means you are extracting the hobbies and occupation properties from the person object then assigning them to variables of the same name, which would output the array inner [“ Reading”, “Coding”,”Designing”] for hobbies and “Software Engineer” for occupation.
Destructuring with Different Names
You can assign extracted properties to variables with different names:
In this example, you're using a syntax like { name: personName, age: personAge, city: personCity } which allows you to assign extracted properties to variables with different names. Here, name from the person object is assigned to personName, age is assigned to personAge, and city is assigned to personCity.
Nested Object Destructuring
Assuming you have a staffList object which has a name object as the property:
The following statement destructures the properties of the nested name object into individual variables:
Its possible to do multiple assignment of a property to multiply varaibles. Check the snippet below:
Destructuring Function Arguments
Suppose you have a function that displays the person object:
It possible to destructuring the object argument passed into the function like this:
It looks less wordy especially when you use many properties of the argument objects. This technique is often used in React.
watch the video below for more info on Object Destructuring
https://www.youtube.com/watch?v=BQs0gTgFz04&pp=ygUab2JqZWN0IGRpc3RydWN0dXJpbmcgaW4ganM%3D
Conclusion
"Array destructuring is a syntax feature in javascript that allows you to extract values from an array and assign them to variables in a concise way".
“ Object destructuring assigns the properties of an object to variable with the same names by default.”
Subscribe to my newsletter
Read articles from Oluwatimilehin Samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
