How to Set Up a Production-Ready MERN Stack Backend Project
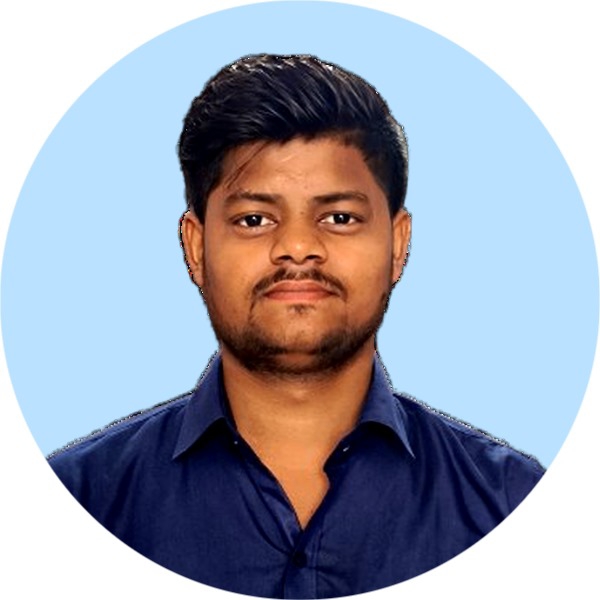
In today's web development landscape, setting up a robust backend infrastructure is pivotal for building scalable and maintainable applications. This guide provides a detailed walkthrough on creating a professional-level backend project using the MERN stack (MongoDB, Express.js, React.js, Node.js). From project initialization to directory structure organization and essential configurations, let's dive into each step with clarity and understanding.
Step 1: Initialize Your Project
The first step is to create a new directory for your backend project and initialize it with npm. This sets up your project's configuration and manages dependencies.
mkdir backend
cd backend
npm init -y
Step 2: Add a README.md File
Documentation is essential for any project. Create a README.md
file to provide an overview, installation instructions, usage guidelines, and other relevant information for developers who will work on or use your project.
touch README.md
Step 3: Setup Environment Variables with dotenv
Managing environment variables securely is crucial, especially for sensitive information like database credentials or API keys. Use the dotenv
package to load environment variables from a .env
file into process.env
.
npm install dotenv
touch .env
In your .env
file, define your environment variables:
PORT=3000
DATABASE_URL=mongodb://localhost:27017/mydatabase
SECRET_KEY=mysecretkey
Step 4: Configure Git and GitHub
Version control with Git allows you to track changes and collaborate effectively. Create a Git repository for your project and link it to a remote repository on GitHub.
git init
# Add and commit your initial files
git remote add origin <repository-url>
git add .
git commit -m "Initial commit"
git push -u origin main
Step 5: Organize Your Source Code
Organizing your source code into structured directories helps maintain clarity and scalability in your project. Create a src
directory and add essential files to kickstart your backend application.
mkdir src
cd src
touch app.js constants.js index.js
app.js
: Initializes your Express application and sets up middleware.constants.js
: Stores constants used throughout your application.index.js
: Starts your server and connects to the database.
Step 6: Setup Prettier for Code Formatting
Consistent code formatting enhances readability and maintains a professional codebase. Install Prettier and create configuration files to enforce code style across your project.
npm install --save-dev prettier
touch .prettierrc .prettierignore
Add your preferred Prettier configurations in .prettierrc
and specify files to ignore in .prettierignore
.
Step 7: Add Nodemon for Development
During development, automatically restarting your server on file changes saves time and effort. Install Nodemon as a development dependency.
npm install --save-dev nodemon
Update your package.json
scripts to use Nodemon for running your application in development:
{
"scripts": {
"start": "node src/index.js",
"dev": "nodemon src/index.js"
}
}
Step 8: Create Essential Directories
Creating directories for different aspects of your backend application helps organize code into manageable components. Here’s a breakdown of each directory's purpose:
db/
: Manages database connection and configurations (db.js
).controllers/
: Defines application logic for handling requests and responses (userController.js
).middlewares/
: Contains middleware functions for request processing (authMiddleware.js
,errorMiddleware.js
).models/
: Defines data models for interacting with the database (User.js
).routes/
: Defines application routes and their associated logic (userRoutes.js
).utils/
: Contains utility functions used across the application (logger.js
,validator.js
).
Conclusion
Congratulations! You have successfully set up a professional-level MERN stack backend project. By following this comprehensive guide, you've established a solid foundation for building scalable and maintainable web applications. Organizing your project with a clear directory structure, managing environment variables securely, and configuring essential tools like Prettier and Nodemon are essential practices in modern web development.
This structured approach not only improves code maintainability and scalability but also fosters collaboration among team members. With your backend infrastructure in place, you're now equipped to implement robust APIs, integrate with frontend applications, and deploy your project confidently.
Continue exploring advanced topics such as authentication, data validation, and error handling to enhance your backend application further. Embrace best practices, stay updated with industry trends, and enjoy building powerful web solutions with the MERN stack. Happy coding!
Subscribe to my newsletter
Read articles from Vivek Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
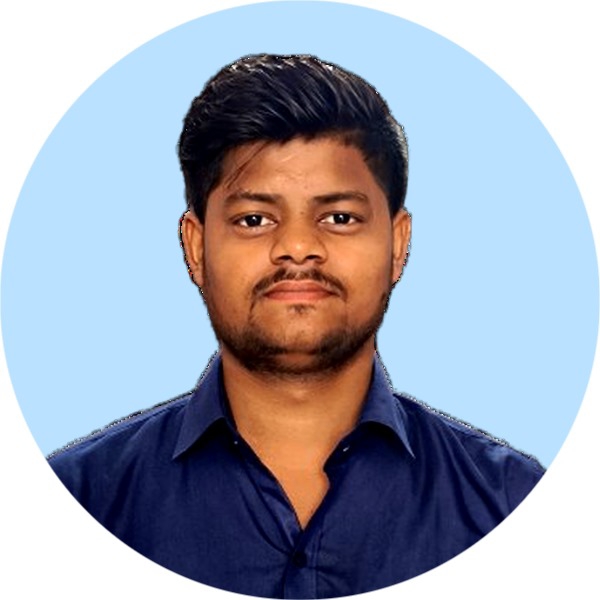
Vivek Kumar
Vivek Kumar
🚀 Full-Stack Web Developer | Problem-Solver Passionate about building solutions that simplify lives. From streamlining workflows to creating seamless digital experiences, I thrive on turning complex challenges into elegant, intuitive technology. Sharing insights, code, and real-world problem-solving to empower the dev community.