Generating a Nigeria Bank List and Their Bank Codes Using PHP and Paystack API
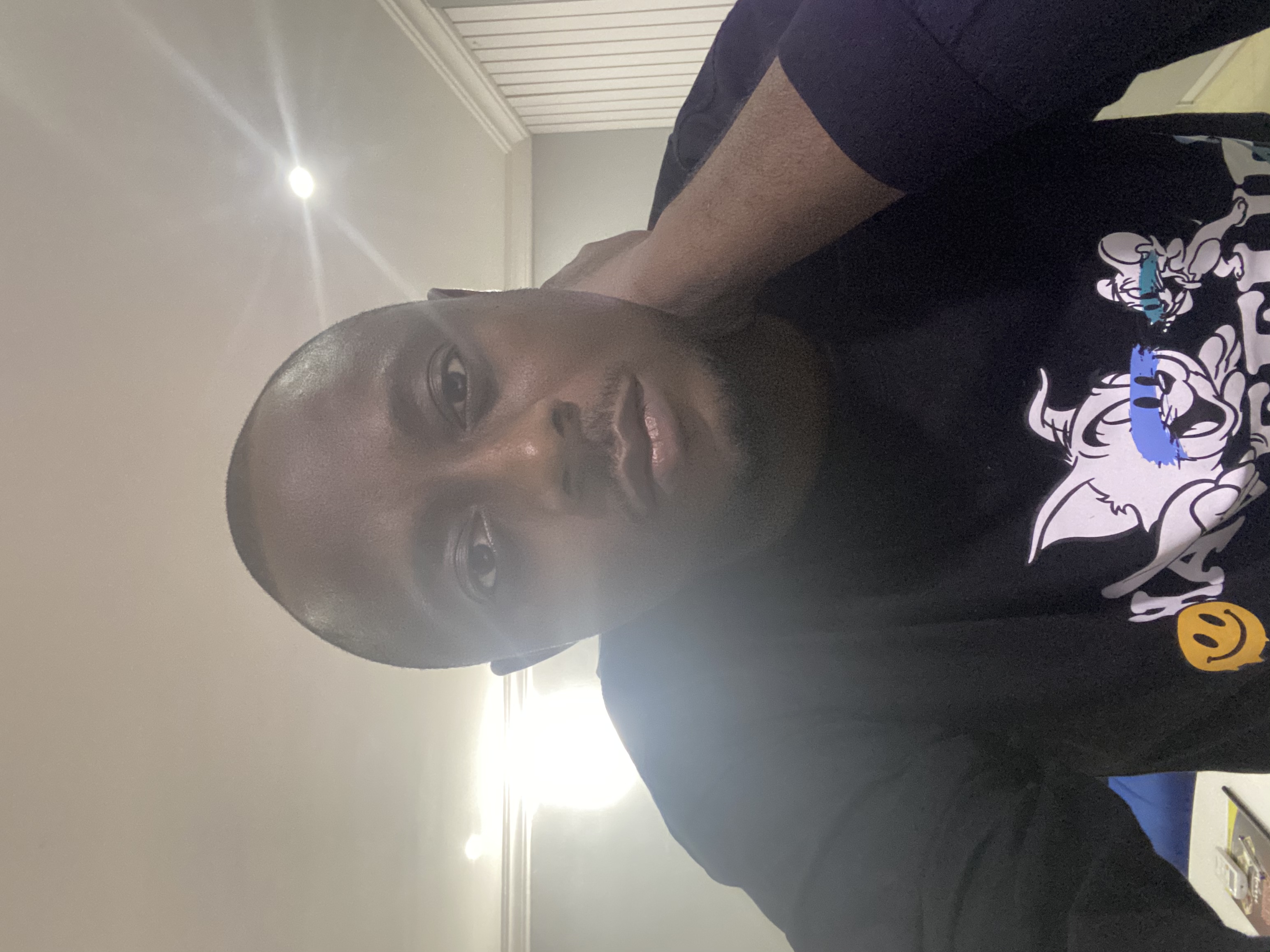
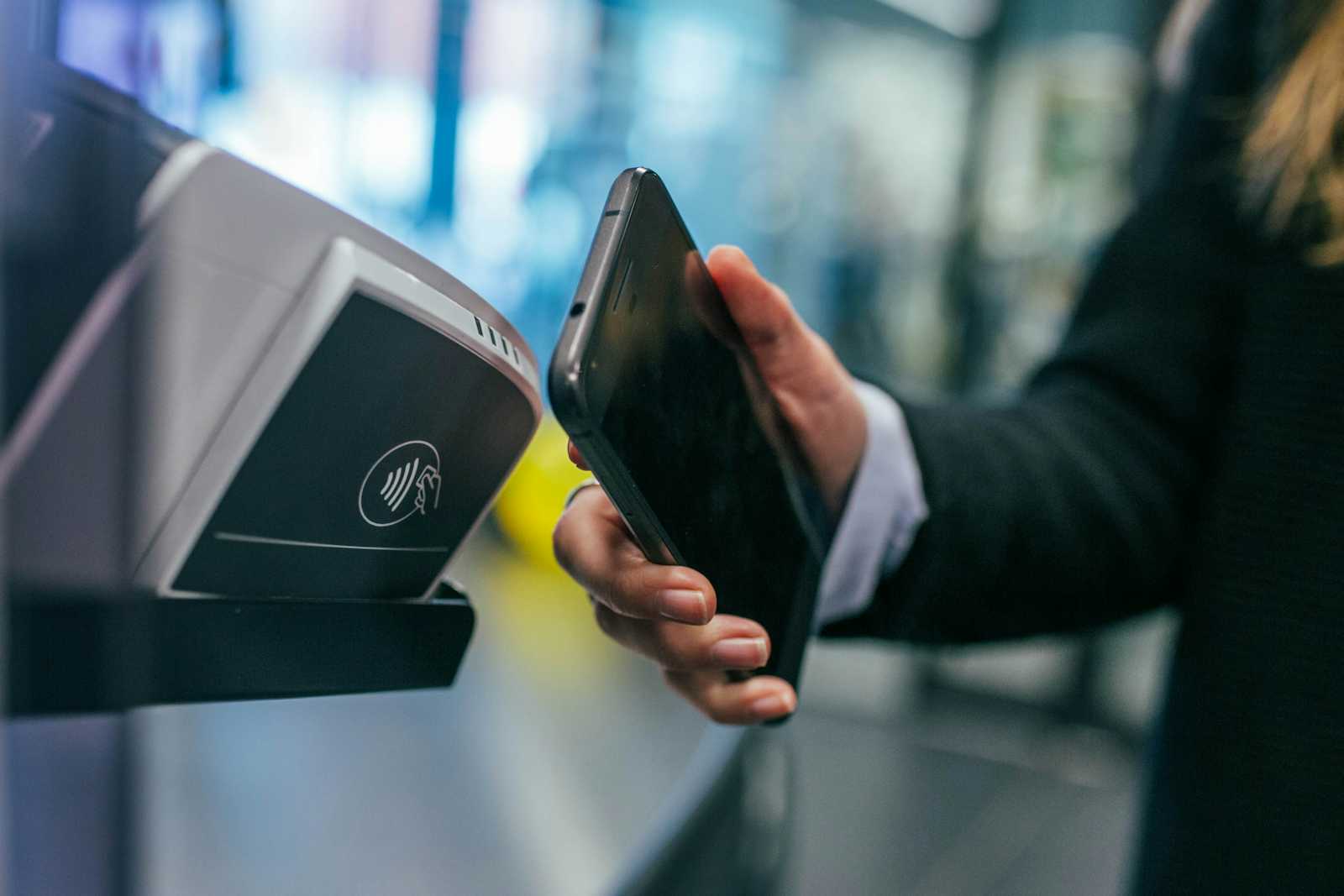
When building financial applications, it's often necessary to provide users with a list of available banks to choose from. This is especially true in Nigeria, where multiple banks operate, each with its unique code. One efficient way to dynamically generate and display a list of Nigerian banks and their corresponding codes is by using the Paystack API. In this post, we'll walk through the process of fetching this data and generating a select dropdown in PHP.
Step-by-Step Guide
Set Up Your Environment: Ensure you have PHP installed and a Paystack account with access to the secret key.
Configuration: Store your Paystack secret key and the API endpoint URL.
Initialize cURL: Set up cURL to make a request to the Paystack API.
Execute the Request: Fetch the data and handle any potential errors.
Generate the Select Dropdown: Parse the response and create HTML
<option>
elements for each bank.
Detailed Steps
1. Configuration
First, store your Paystack secret key and the URL of the Paystack bank list endpoint.
<?php
$paystackSecretKey = 'your_secret_key';
$url = "https://api.paystack.co/bank";
2. Initialize cURL
Initialize cURL to prepare for making an API request. This involves setting the URL, return transfer option, and headers.
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $paystackSecretKey"
]);
3. Execute the Request
Execute the cURL request to fetch the bank list. Check for any errors that might occur during the process.
$response = curl_exec($ch);
$curl_error = curl_error($ch);
curl_close($ch);
if ($curl_error) {
die("CURL Error: " . $curl_error);
}
4. Decode the Response
Decode the JSON response from Paystack and check the status of the response.
$banks = json_decode($response, true);
if (!$banks['status']) {
die("Failed to fetch banks: " . json_encode($banks));
}
5. Generate the Select Dropdown
Finally, generate the HTML <select>
dropdown, iterating over the bank data to create each <option>
element.
echo '<select name="bank_code">';
foreach ($banks['data'] as $bank) {
echo '<option value="' . $bank['code'] . '">' . $bank['name'] . '</option>';
}
echo '</select>';
Full Code
Here's the complete PHP script, embedded in an HTML form:
<div>
<label>Select Bank Name</label>
<?php
// Configuration
$paystackSecretKey = 'your_secret_key';
$url = "https://api.paystack.co/bank";
// Initialize cURL
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $paystackSecretKey"
]);
// Execute the request and decode the response
$response = curl_exec($ch);
$curl_error = curl_error($ch);
curl_close($ch);
if ($curl_error) {
die("CURL Error: " . $curl_error);
}
$banks = json_decode($response, true);
if (!$banks['status']) {
die("Failed to fetch banks: " . json_encode($banks));
}
// Generate the select dropdown
echo '<select name="bank_code">';
foreach ($banks['data'] as $bank) {
echo '<option value="' . $bank['code'] . '">' . $bank['name'] . '</option>';
}
echo '</select>';
?>
</div>
Conclusion
By following these steps, you can dynamically generate a list of Nigerian banks and their codes using the Paystack API. This approach ensures that your application always has the most up-to-date bank information, improving the user experience and reducing manual updates. Integrating this into your application is straightforward and leverages the robust capabilities of the Paystack API for handling financial operations.
For further customization, you can style the dropdown to match your application's theme or add additional form validation to ensure users select a valid bank.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
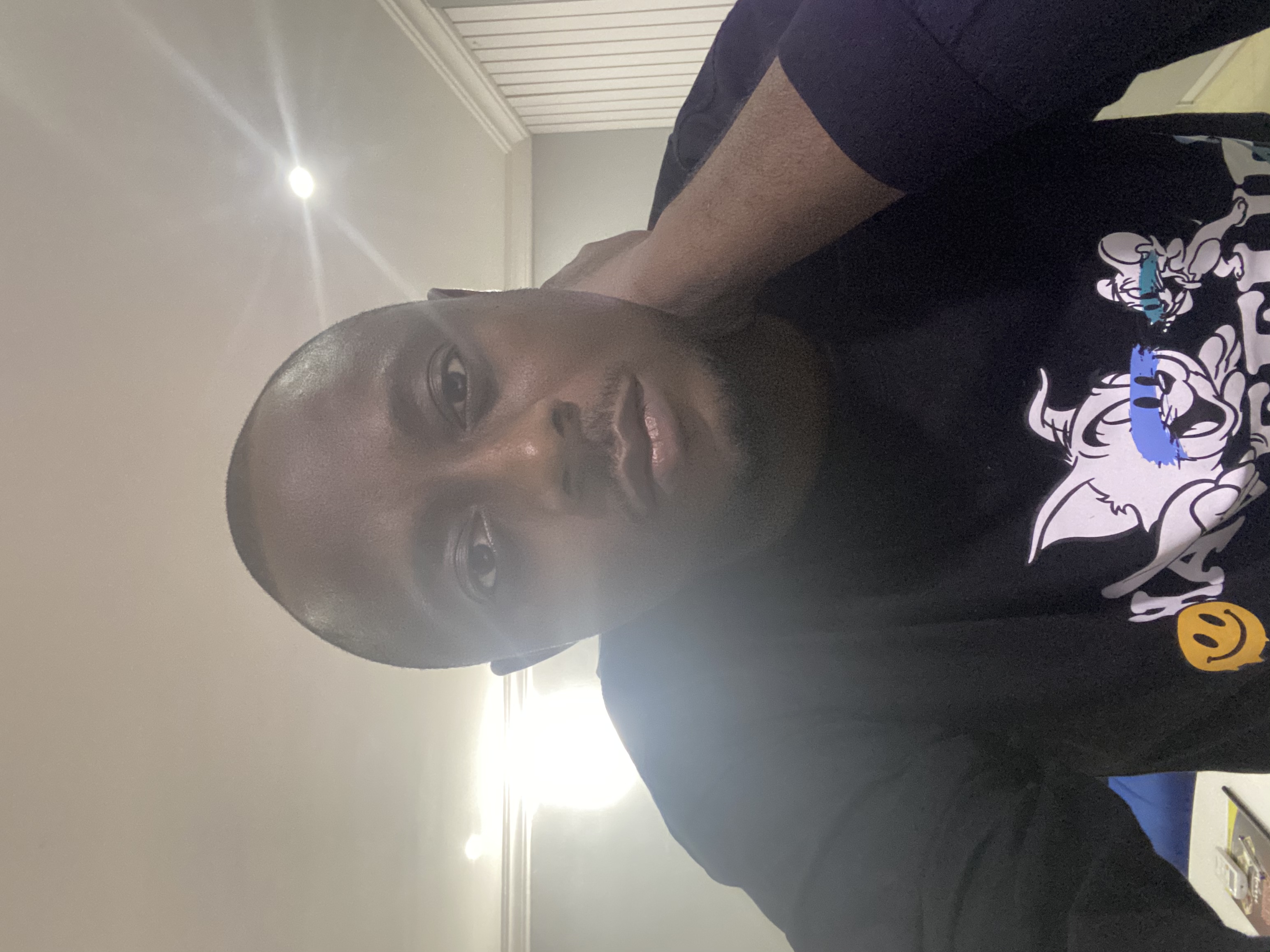
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.