Mastering DOM Manipulation in JavaScript: Essential Techniques

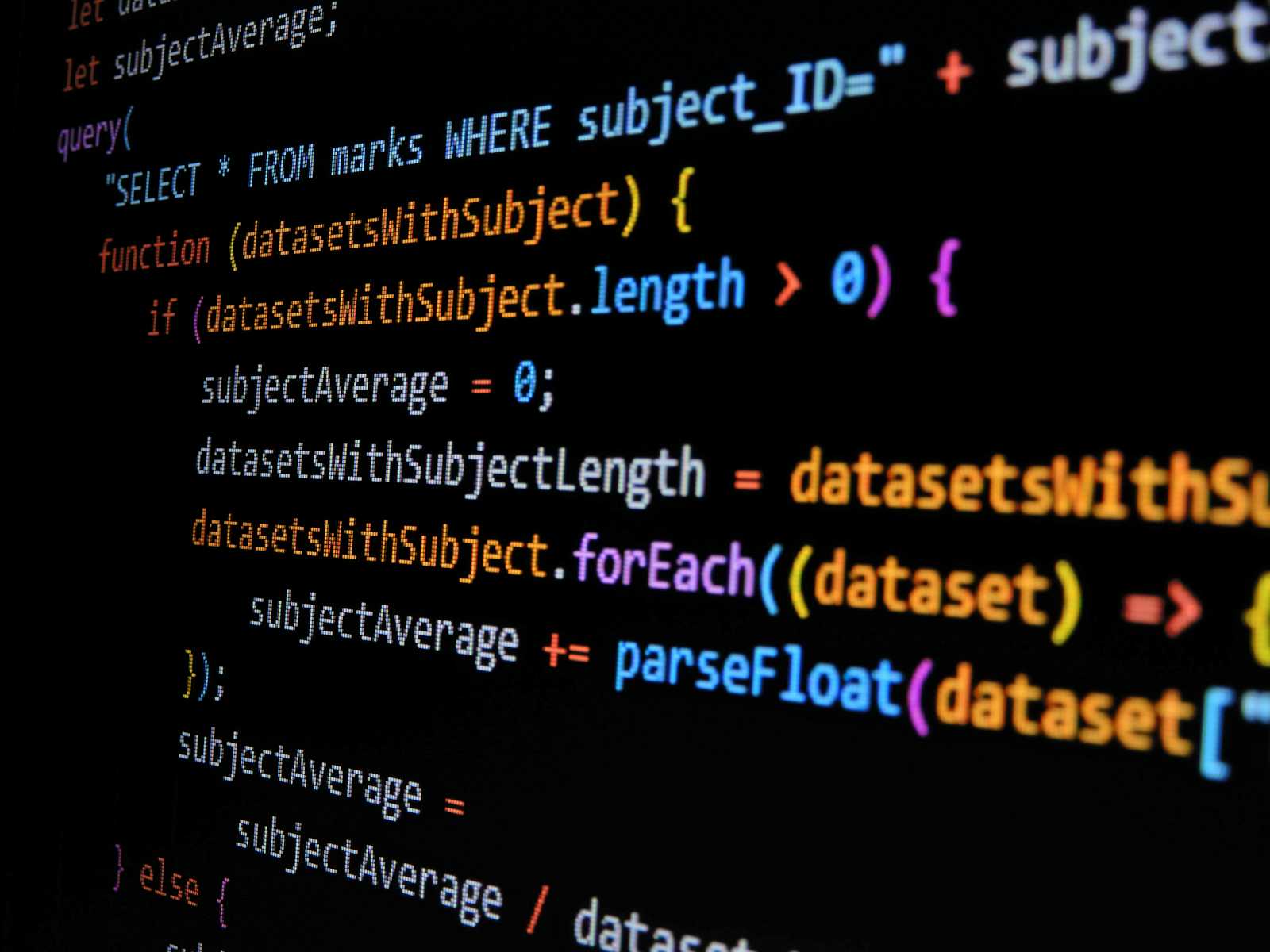
Web development often involves manipulating the Document Object Model (DOM) to create dynamic, interactive web pages. Becoming proficient in DOM manipulation is crucial for any developer. This guide will cover 14 essential techniques that will make you a DOM manipulation master.
Welcome back to Web Dev Simplified! I'm Kyle, and my goal is to simplify web development so you can build your dream projects faster.
If you're eager to elevate your JavaScript skills, consider subscribing to my channel for more tutorials. For a more comprehensive learning experience, check out my full JavaScript course linked in the description below.
Adding Elements to the Page
Using append
and appendChild
Adding elements to your webpage is foundational. You can add elements using two methods: append
and appendChild
. Here's how to do it:
Select the Element:
const body = document.body;
Appending Strings and Elements:
With
append
:body.append('Hello World');
With
appendChild
:const div = document.createElement('div'); body.appendChild(div); div.innerText = 'Hello World';
While appendChild
only accepts nodes, append
can handle strings and multiple elements at once, making it more versatile.
Creating Elements
To create a new element, use document.createElement
:
const div = document.createElement('div');
body.append(div);
Modifying Text Inside Elements
Using innerText
and textContent
You can modify the text inside elements using innerText
or textContent
:
div.innerText = 'Hello World';
div.textContent = 'Hello World 2';
innerText
considers the CSS and only includes visible text.textContent
includes all text, even if it's not visible due to CSS.
Modifying HTML Inside Elements
Use innerHTML
to insert HTML:
div.innerHTML = '<strong>Hello World</strong>';
Be cautious with innerHTML
, as it can expose your site to security risks if user-generated content is inserted.
Alternatively, you can build elements manually for better security:
const strong = document.createElement('strong');
strong.innerText = 'Hello World';
div.appendChild(strong);
Removing Elements
Removing elements is straightforward:
const elementToRemove = document.querySelector('#element-id');
elementToRemove.remove();
Or, use removeChild
:
const parent = document.querySelector('#parent-id');
const child = document.querySelector('#child-id');
parent.removeChild(child);
Accessing and Modifying Attributes
Using getAttribute
, setAttribute
, and removeAttribute
Get Attribute:
const id = element.getAttribute('id');
Set Attribute:
element.setAttribute('id', 'new-id');
Remove Attribute:
element.removeAttribute('id');
Working with Data Attributes
Data attributes start with data-
and are useful for storing information within HTML elements:
<span data-test="sample" data-longer-name="example"></span>
Access them using dataset
:
const span = document.querySelector('span');
console.log(span.dataset.test); // Output: sample
span.dataset.newName = 'hello';
Managing Classes
Using classList
classList
provides methods for manipulating classes:
Add Class:
element.classList.add('new-class');
Remove Class:
element.classList.remove('old-class');
Toggle Class:
element.classList.toggle('toggle-class');
Directly Modifying Styles
Change CSS properties directly via style
:
element.style.color = 'red';
element.style.backgroundColor = 'blue';
Remember to camel-case multi-word properties like backgroundColor
.
Conclusion
Mastering these DOM manipulation techniques will make your web development journey smoother and more efficient. If you're eager to dive deeper, check out my full JavaScript course for more insights and advanced techniques.
Thank you for reading, and happy coding!
Subscribe to my newsletter
Read articles from Hrithik Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hrithik Singh
Hrithik Singh
I am a graduate in computer science and engineering. With over a year of working as a fullstack developer in a legal company and joining lots of hackathons, I've gained a bunch of skills and improved my problem solving skills. I know my way around C, C++, and JavaScript, Python, and I'm pretty good with frameworks like Node.js, ReactJs, and NextJs, handling both front and back-end stuff.