24 Java - Multithreading 1
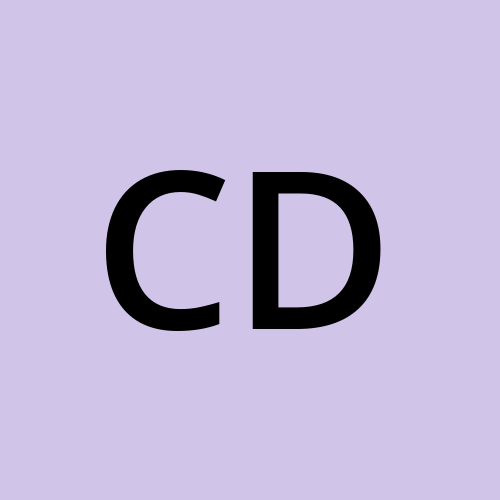
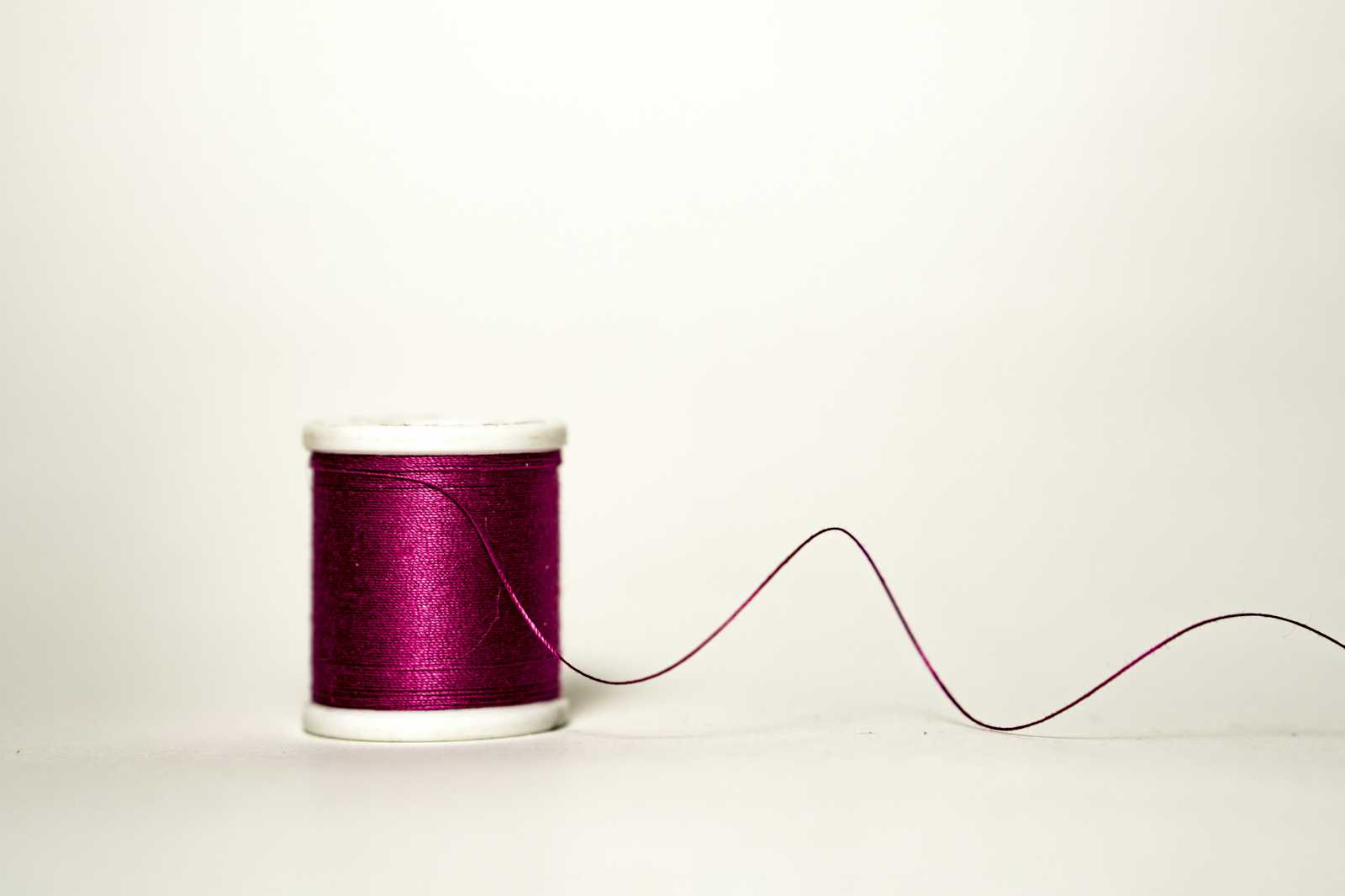
Introduction
- Before we understand what is multithreading, lets first understand Thread and Process.
Process
Process is an instance of a program that is getting executed.
It has its own resource like memory, thread etc. OS allocate these resource to process when its created.
Compilation (javac Test.java): generates bytecode that can be executed by JVM.
Execution (java Test): At this point, JVM starts the new Process, here Test is the class which has
public static void main(String args[])
method.
How much memory does process gets?
While creating the process java MainClassName
command, a new JVM instance will get created and we can tell how much heap memory need to be allocated.
java -Xms256m -Xmx2g MainClassName
-Xms<size>: This will set the initial heap size, above we allocated 256MB
-Xmx<size>: Max heap size, process can get, above we allocated 2GB, if tries to allocated more memory, OutOfMemoryError
will occur.
Thread
Thread is known as lightweight process
(OR) Smallest sequence of instructions that are executed by CPU independently
1 Process can have multiple threads.
When a Process is created, it start with 1 thread and that initial thread known as
main thread
and from we can create multiple threads to perform task concurrently.
public class Main {
public static void main(String[] args) {
System.out.println("Thread Name: "+Thread.currentThread().getName());
}
}
//Thread Name: main
Architecuture
Code Segment
Contains the compiled BYTECODE (i.e machine code) of the Java Program.
Its read only
All threads within the same process, share the same code segment.
In essence,
javac
converts the code to bytecode, and then Java converts the bytecode to machine code.
Data Segment
Contains the GLOBAL and STATIC variables.
All threads within the same process, share the same data segment.
Threads can read and modify the same data.
Sychronization is required between multiple threads.
Heap
Objects created at runtime using
new
keyword are allocated in the heap.Heap is shared among all the threads of the same process. (but NOT WITHIN PROCESS)
- lets say in Process1, X8000 heap memory pointing to some location in physical memory, same X8000 heap memory point to different location for Process2.
Threads can read and modify the heap data.
Synchronization is required between multiple threads.
Stack
Each thread has its own STACK.
It manages, method calls, local variables
Register
When JIT(Just-in time) compiles converts the Bytecode into native machine code, its uses register to optimized the generated machine code.
Also helps in context switching.
Each thread has its own Register.
Counter
Also known as Program Counter, it points to the instruction which is getting executed.
Increments its counter after successfully executiong of the instruction
All these are managed by JVM
CPU Execution
Each CPU can execute only one thread at a time. If we have four cores in the system, meaning we have four CPUs that can execute four threads in parallel.
So, if a process has three threads and we have one CPU, we can execute one thread for some time, store the state of the current thread execution in its register, then move to the next thread, execute it for some time, and store its state in its register again. We continue this process until we reach the last thread. This is known as context switching.
Even if we have a single thread and perform context switching, it is not considered parallel execution.
Definition of Multithreading
Allows a program to perform multiple operation at the same time.
Multiple threads share the same resource such as memory space but still can perform operation independently
Benefits
Improved performance by task parallelism
Responsiveness
Resource Sharing
Challenges
Concurrency issue like deadlock, data inconsistency etc.
Sychronized overhead
Testing and Debugging is difficult.
Multitasking vs Multithreading
Mulittasking | Multithreading | |
Defintion | Executing different processes in parallel is called multitasking. | Executing different threads of the process parallely is called multithreading |
Share Resources? | No, 2 processes do not share the same resources | Yes, 2 Threads share the same resource |
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
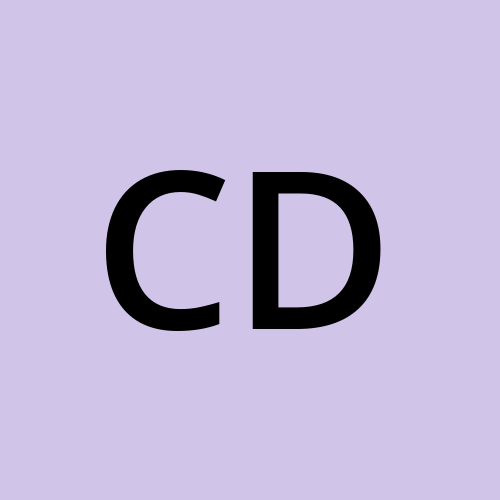
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.