Day 4: Exploring Randomness, Lists, and Conditional Logic in Python
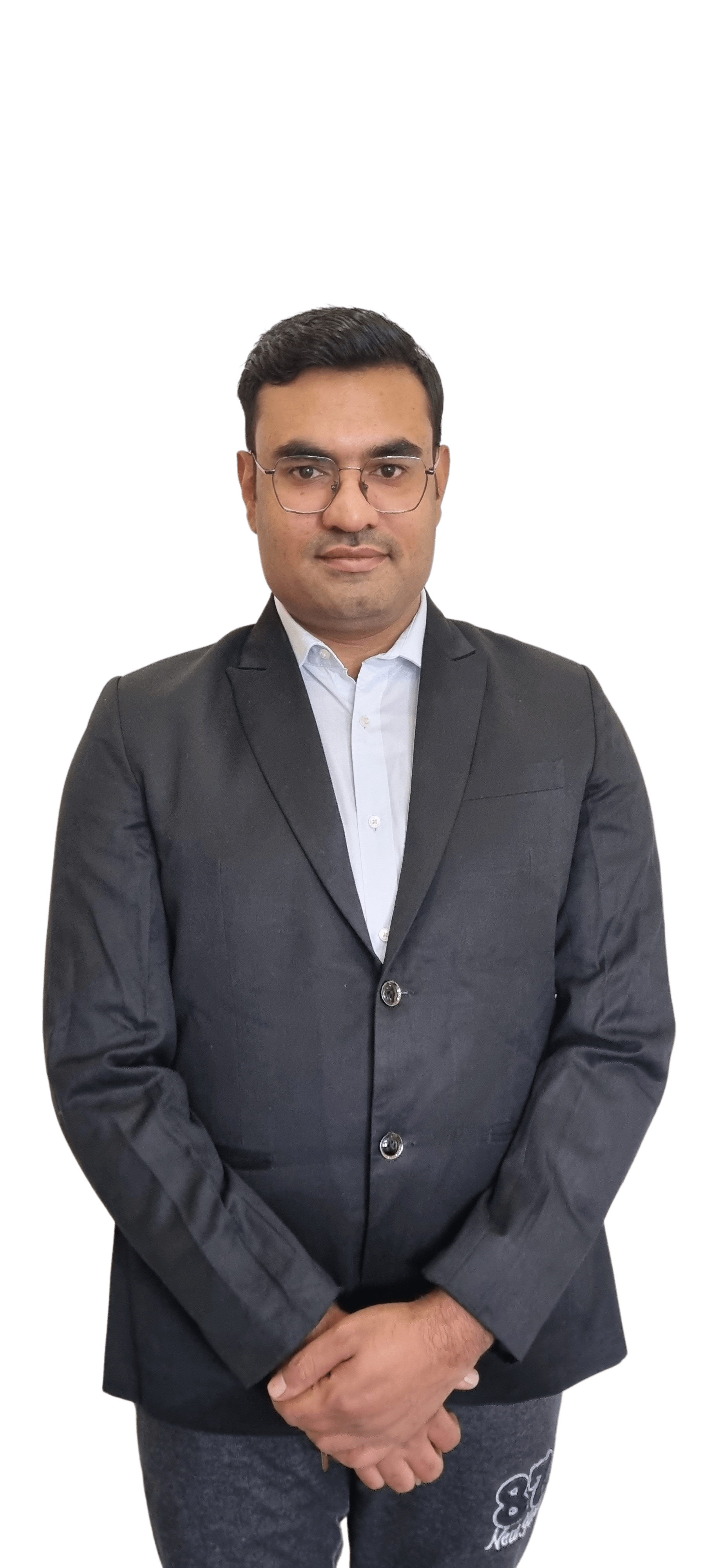
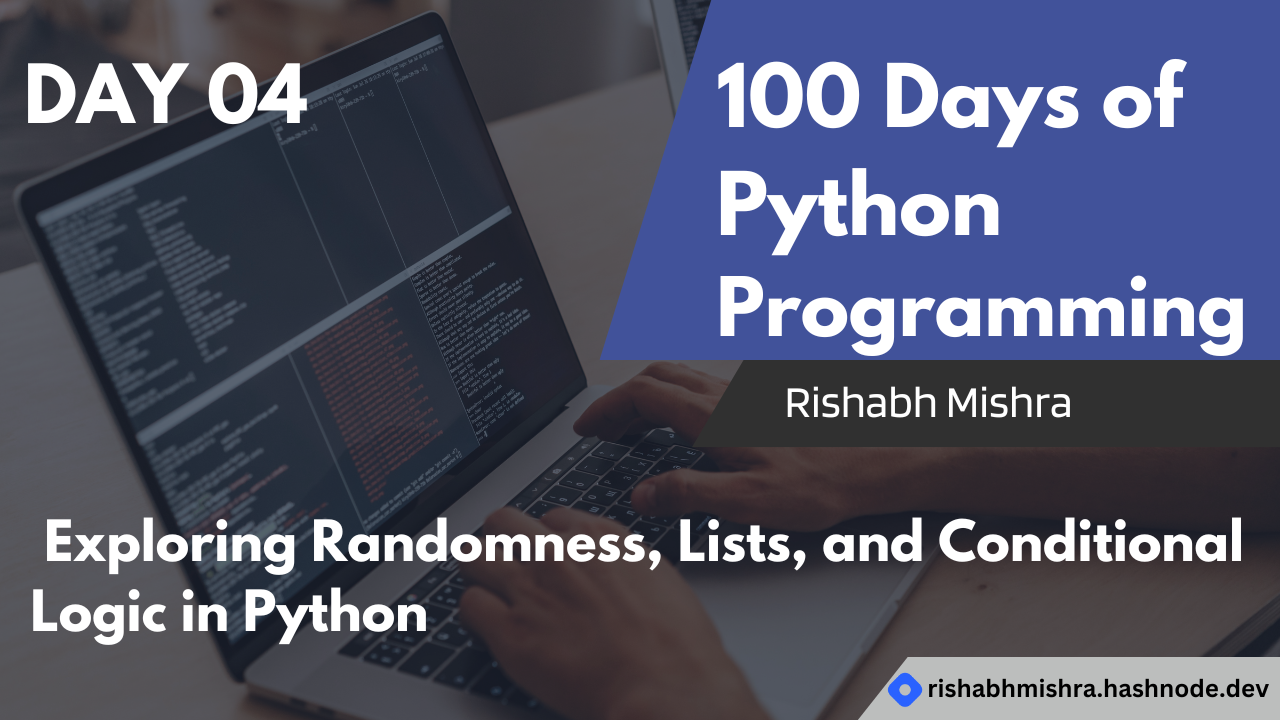
Welcome back to my Python learning journey! Today, I delved deeper into Python programming with a focus on practical applications. Let's explore the concepts I mastered and the code I wrote.
1. Harnessing Randomness with random
Module
Python's random
module is invaluable for introducing unpredictability into programs. By using random.randint()
, I could generate random integers within a specified range. This is foundational for creating simulations, games, and more dynamic applications.
import random
random_integer = random.randint(1,10)
print(random_integer)
Key Concept:
- Random Number Generation: The
random.randint()
function is used to generate random integers inclusively within a given range. This is useful for creating varied and unpredictable behavior in programs.
2. Random Selection from a List
Another practical use of the random
module is selecting a random item from a list. Here's an example where a random name is chosen to determine who pays today's bill:
import random
name = ["Angela", "Ben", "Jenny", "Michael", "Chloe"]
print(len(name))
random_choice = random.randint(0,len(name)-1)
random_name = name[random_choice]
print(f"The Person going to pay today's bill is {random_name}")
Key Concept:
- Random Selection: Using
random.randint()
to generate a random index, you can select a random element from a list. This technique is useful for scenarios where a random choice is needed, such as selecting winners or assigning tasks randomly.
3. Applying Conditional Statements
Conditional statements (if
, else
) allow programs to make decisions based on specific conditions. I applied this concept in a simple coin flip simulation where the outcome (heads or tails) was determined randomly.
import random
random_integer = random.randint(0,1)
if random_integer == 0 :
print("Tails")
else :
print("Heads")
Key Concept:
- Conditional Logic: Using
if
andelse
statements enables programs to execute different blocks of code based on whether certain conditions are true or false. This is essential for controlling the flow of a program.
4. Managing Data with Lists
Lists in Python are versatile data structures used to store collections of items. I created lists of Indian states and union territories, and also explored nested lists for more complex data organization.
#India has 28 states and 8 union territories:
#States
#Andhra Pradesh, Arunachal Pradesh, Assam, Bihar, Chhattisgarh, Goa, Gujarat, Haryana, Himachal Pradesh,
#Jharkhand, Karnataka, Kerala, Madhya Pradesh, Maharashtra, Manipur, Meghalaya, Mizoram, Nagaland, Odisha,
#Punjab, Rajasthan, Sikkim, Tamil Nadu, Telangana, Tripura, Uttar Pradesh
#Union territories
#Andaman and Nicobar Islands, Chandigarh, Dadra and Nagar Haveli and Daman and Diu, Delhi, Jammu and Kashmir,
#Ladakh, Lakshadweep, and Puducherry
UTs = ["Andaman and Nicobar Islands", "Chandigarh", "Dadra and Nagar Haveli and Daman and Diu", "Delhi", "Jammu and Kashmir",
"Ladakh", "Lakshadweep","Puducherry"]
States = ["Andhra Pradesh","Arunachal Pradesh","Assam","Bihar","Chhattisgarh","Goa","Gujarat","Haryana","Himachal Pradesh","Jharkhand",
"Karnataka","Kerala","Maharashtra","Madhya Pradesh","Manipur","Meghalaya","Mizoram","Nagaland","Odisha","Punjab","Rajasthan","Sikkim",
"Tamil Nadu","Tripura","Telangana","Uttar Pradesh","Uttarakhand","West Bengal"]
print(len(States))
print(UTs[-1])
States_and_UT = [States , UTs]
print(States_and_UT)
Key Concept:
- Lists: Lists are sequences in Python used to store collections of items. They are mutable (can be changed) and allow indexing and slicing operations, making them ideal for managing and manipulating data.
5. Interactive Programming with User Input : Rock, Paper, Scissors game
Incorporating input()
allowed me to create interactive programs where users could provide their choices. I developed a Rock, Paper, Scissors game where users play against the computer, implementing logic to determine the winner based on their selections.
import random
RPS = ["Rock", "Paper", "Scissor"]
user_input = input("Enter you choice out of Rock , Paper, Scissor : - ")
random_choice = RPS[random.randint(0,2)]
print(f"You entered {user_input} and computer entered {random_choice}")
if user_input == random_choice :
print("Game is Draw")
else :
if (user_input == "Rock" and random_choice == "Paper") or (user_input == "Scissor" and random_choice == "Rock") or (user_input == "Paper" and random_choice == "Scissor") :
print("Computer wins the game")
else :
if (user_input == "Paper" and random_choice == "Rock") or (user_input == "Rock" and random_choice == "Scissor") or (user_input == "Scissor" and random_choice == "Paper") :
print("You win the Game")
else :
print("You have entered a wrong choice")
Key Concept:
- User Input and Conditional Statements: Using
input()
allows interaction with users, while conditional statements (if
,elif
,else
) provide logical branching based on user choices. This is crucial for creating interactive applications.
6. Conclusion
Day 4 was filled with hands-on learning and practical applications. From mastering random number generation to implementing game logic with conditional statements and managing data with lists, each exercise enriched my understanding of Python's capabilities.
Stay tuned for more updates as I progress on my Python journey. If you have any questions or suggestions, feel free to reach out. Until next time!
Subscribe to my newsletter
Read articles from Rishabh Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
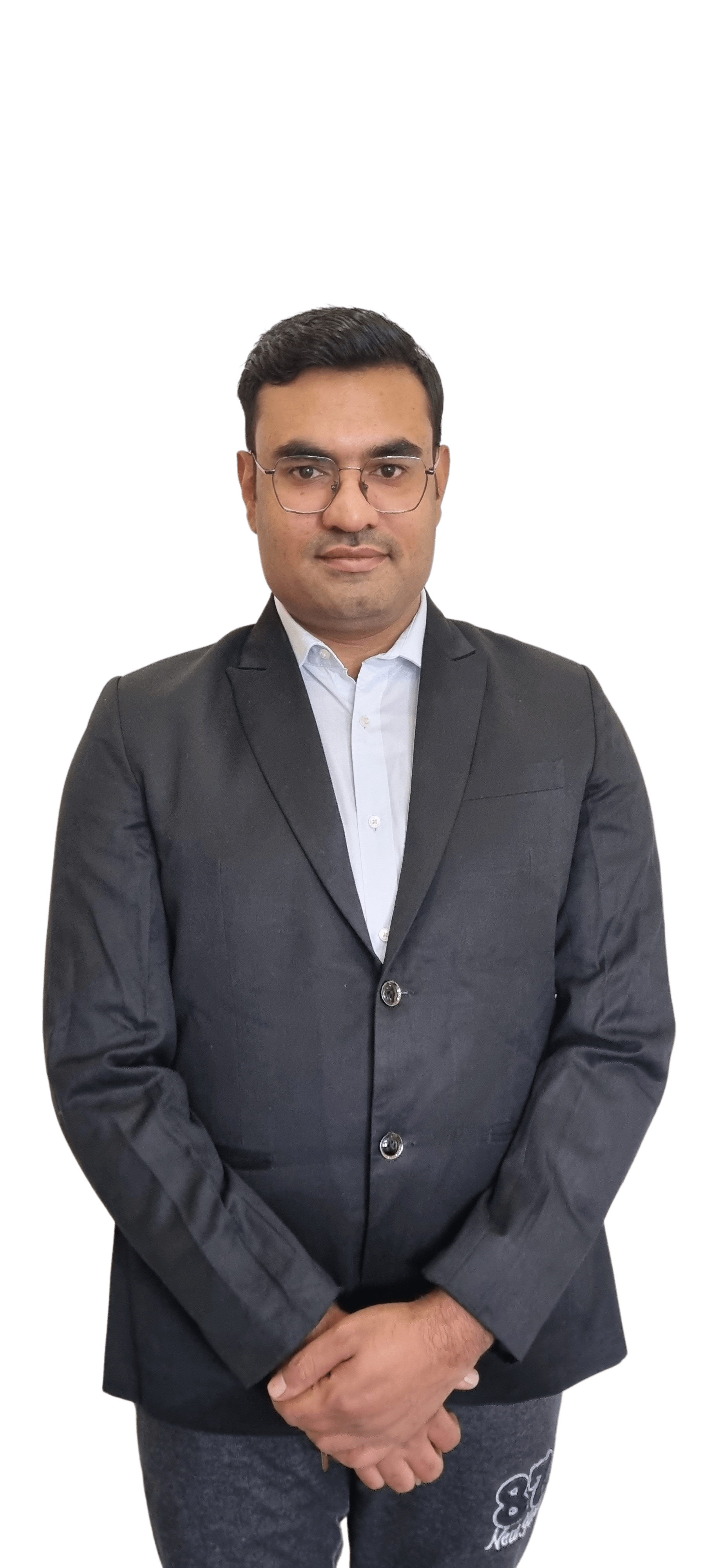
Rishabh Mishra
Rishabh Mishra
I'm Rishabh Mishra, an AWS Cloud and DevOps Engineer with a passion for automation and data analytics. I've honed my skills in AWS services, containerization, CI/CD pipelines, and infrastructure as code. Currently, I'm focused on leveraging my technical expertise to drive innovation and streamline processes. My goal is to share insights, learn from the community, and contribute to impactful projects in the DevOps and cloud domains. Let's connect and collaborate on Hashnode!