Understanding Callbacks in JavaScript: A Practical Guide
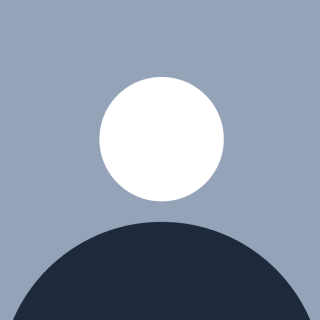
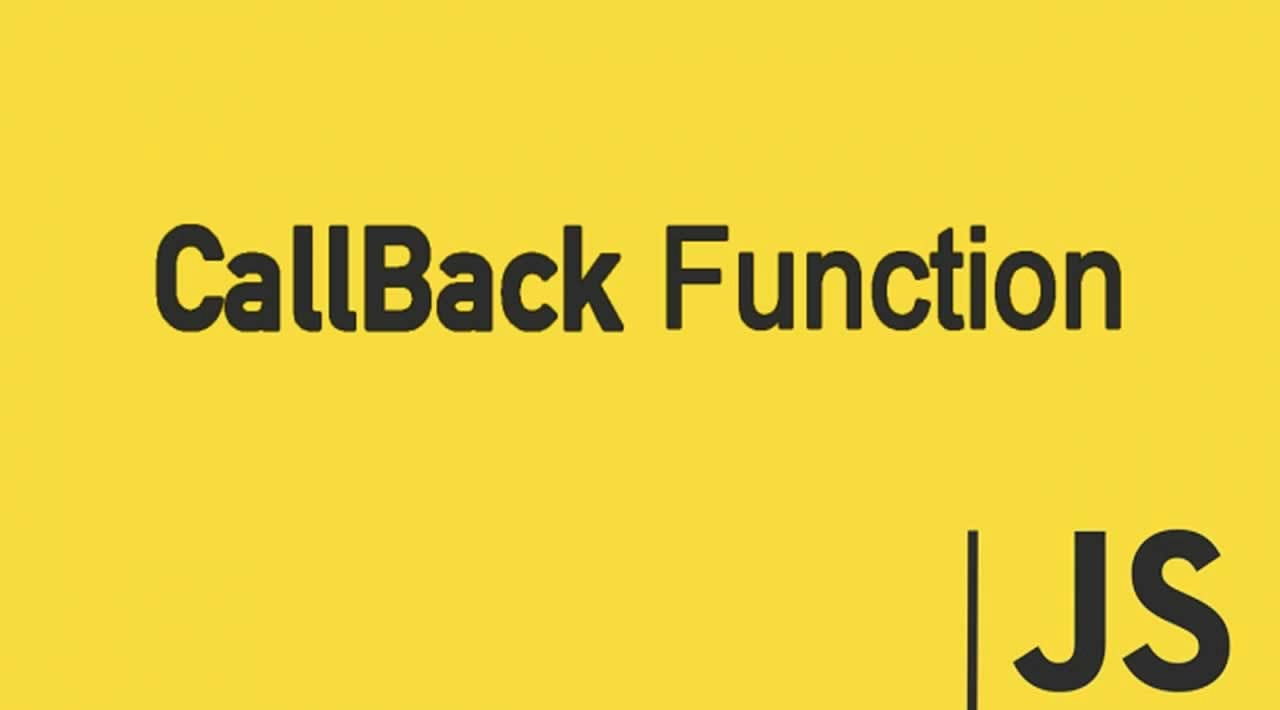
Introduction
In JavaScript, callbacks are fundamental in asynchronous programming. They allow us to define behavior that should occur after a certain task has completed or in response to an event. Understanding callbacks is crucial for mastering JavaScript development, especially when dealing with asynchronous operations such as handling HTTP requests in web applications.
The Problem
Imagine you have a web server built using Node.js and Express.js. You define several routes (/route1, /route2, /route3) that handle incoming HTTP requests. Each route may have different requirements for handling errors (err
), accessing request (req
) and response (res
) objects, and calling the next middleware (next
). To handle these scenarios effectively, you need to grasp how callbacks work and how they can be used to manage varying conditions within each route handler.
app.get("/rout1", (req, res)=>{
//access req, res
})
app.get("/rout2", (err, req, res, next)=>{
//access err, req, res, next
})
app.get("/rout3", (req, res, next)=>{
//access req, res, next
})
What is a Callback?
A callback in JavaScript is simply a function that is passed as an argument to another function and is executed after some asynchronous operation completes or when a certain condition is met. Callbacks are commonly used to handle events, asynchronous operations like file I/O, network requests, or timer functions.
Example 1: Basic Callback Function (fn
)
Let's start with a simple example of defining a function fn
that takes a callback function as an argument. The fn
function executes the callback, passing it two functions (a
and b
) as arguments:
function fn(callback) {
// Define two example functions a and b
function a() {
console.log('Function a called');
}
function b() {
console.log('Function b called');
}
// Call the callback function with functions a and b as arguments
callback(a, b);
}
// Example usage of fn
fn((ar, br) => {
ar(); // Calls function a
br(); // Calls function b
});
Example 2: Each handler may require different actions
Now, let's apply the concept of callbacks to a scenario where you define route handlers in an Express.js application. Each handler may require different actions based on the number of arguments it receives:
function fn(callback) {
// Example functions a and b
function a() {
console.log('Function a called');
}
function b() {
console.log('Function b called');
}
function c() {
console.log('Function c called');
}
function d() {
console.log('Function d called');
}
// Check if callback is a function
if (typeof callback !== 'function') {
console.log('Invalid callback provided');
return;
}
if (callback.length === 2) {
// Execute for two arguments
callback('b', 'c');
} else if (callback.length === 3) {
// Execute for three arguments
callback('b', 'c', 'd');
} else if (callback.length === 4) {
// Execute for four arguments
callback('a', 'b', 'c', 'd');
} else {
// Handle other cases if needed
console.log("Invalid number of arguments in the callback function");
}
}
// Example callback functions
// Executes for two arguments
fn((br, cr) => {
br(); // Calls function a
cr(); // Calls function b
});
// Executes for three arguments
fn((br, cr, dr) => {
br(); // Calls function b
cr(); // Calls function c
dr(); // Calls function d
});
// Executes for two arguments
fn((ar, br, cr, dr) => {
ar(); // Calls function a
br(); // Calls function b
cr(); // Calls function c
dr(); // Calls function d
});
Thank You for Reading!
Thank you for taking the time to read this post. Your support and feedback are greatly appreciated!
Subscribe to my newsletter
Read articles from SOURIN PAL directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by