Exploring Enhanced Enums in Flutter: A Simple Guide for Beginners and Experienced Developers
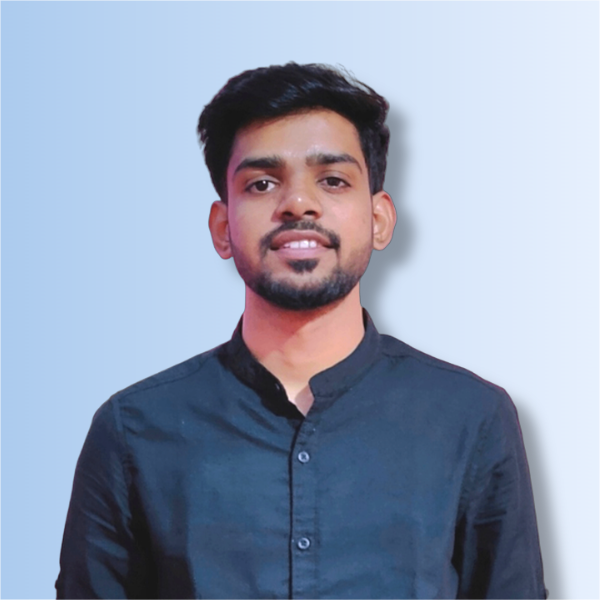
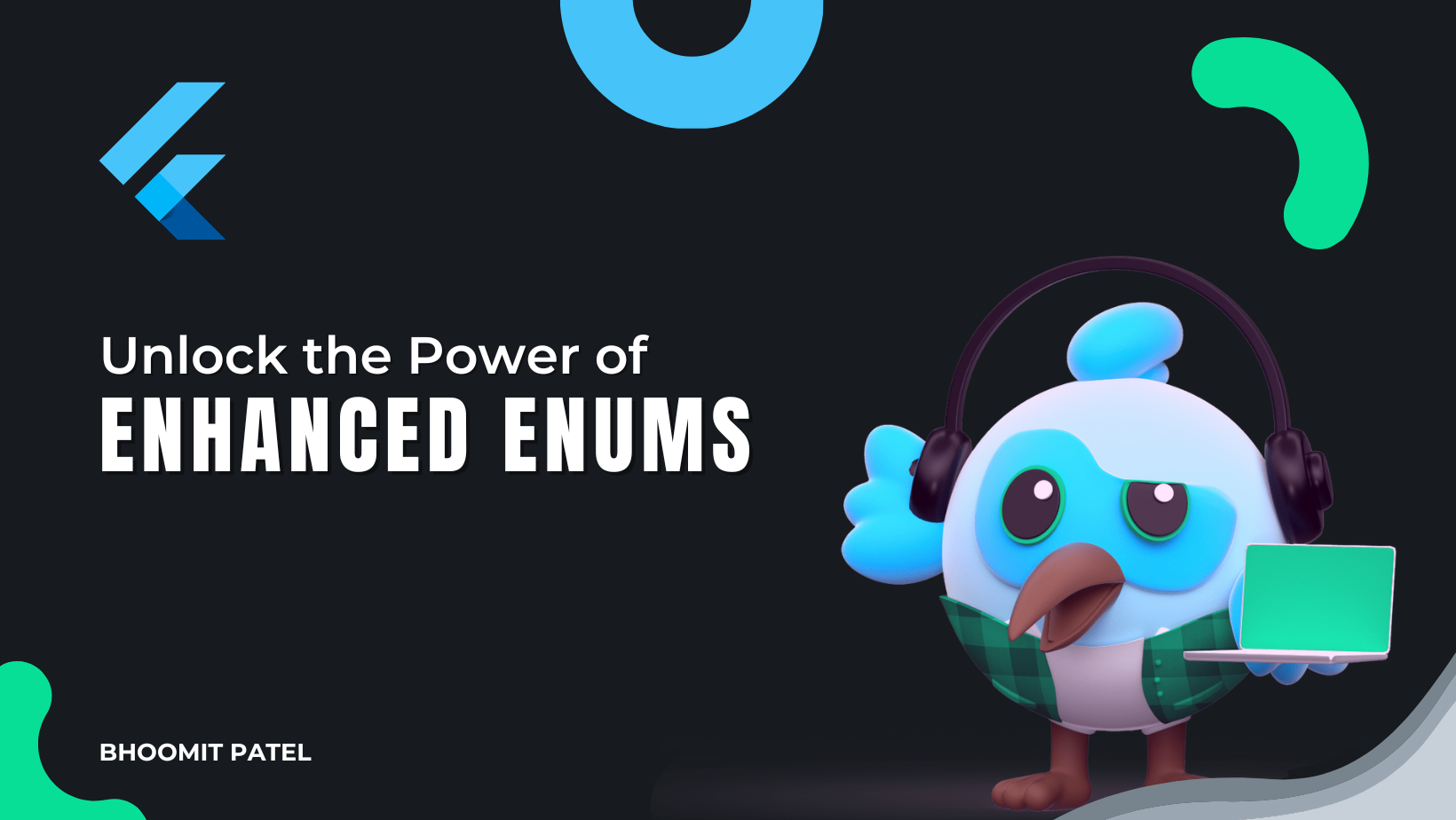
Hello Flutter enthusiasts! Whether you're just starting your journey with Flutter or have been developing with it for a while, you know how enums can be incredibly useful. In this blog, we'll dive into the concept of enhanced enums in Flutter. We'll break it down in a way that's easy to understand and apply in your day-to-day projects. So, let's get started!
What Are Enums?
Before we dive into enhanced enums, let's quickly recap what enums are. Enums, short for enumerations, are a special kind of class used to define a collection of constants. They're great for representing a fixed set of related values. For example, let's say you're building a weather app and want to represent different weather conditions like sunny, cloudy, rainy, etc. You can use an enum for this.
enum WeatherCondition {
sunny,
cloudy,
rainy,
snowy,
}
Why Use Enhanced Enums?
While basic enums are useful, sometimes you need more than just a list of constants. You might want to add additional data or methods to your enums to make them more powerful and versatile. This is where enhanced enums come into play.
Enhanced enums in Dart allow you to add more functionality to your enums. You can include fields, constructors, and methods, making your enums much more flexible and useful.
Example 1: Enhanced Enums in Action
Let's consider an example where we need to represent different user roles in an app. Each role has a name, a description, and a set of permissions. We'll use enhanced enums to achieve this.
Step 1: Define the Enhanced Enum
First, let's define our UserRole
enum with additional fields and a constructor.
enum UserRole {
admin('Administrator', 'Has full access to all features', ['read', 'write', 'delete']),
editor('Editor', 'Can edit content', ['read', 'write']),
viewer('Viewer', 'Can only view content', ['read']);
final String name;
final String description;
final List<String> permissions;
const UserRole(this.name, this.description, this.permissions);
bool hasPermission(String permission) {
return permissions.contains(permission);
}
}
Step 2: Using the Enhanced Enum
Now that we have our enhanced enum, let's see how we can use it in our app.
void main() {
UserRole role = UserRole.editor;
print('Role: ${role.name}');
print('Description: ${role.description}');
print('Permissions: ${role.permissions.join(', ')}');
String permissionToCheck = 'write';
if (role.hasPermission(permissionToCheck)) {
print('${role.name} has $permissionToCheck permission.');
} else {
print('${role.name} does not have $permissionToCheck permission.');
}
}
Example 2: Simplifying Payment Methods
enum PaymentMethod {
creditCard('Credit Card', 1.5),
paypal('PayPal', 2.0),
bankTransfer('Bank Transfer', 0.5);
final String displayName;
final double feePercentage;
const PaymentMethod(this.displayName, this.feePercentage);
double calculateFee(double amount) {
return amount * (feePercentage / 100);
}
}
In this example, we enhance the PaymentMethod
enum in Dart to include additional properties such as displayName
and feePercentage
. This allows for clearer and more structured representation of payment options in your code. Each payment method now encapsulates its name and fee percentage, streamlining how fees are calculated and improving code readability.
Enhanced enums provide several advantages:
Associated Data: They allow enum constants to store additional information like strings, lists, or custom objects.
Readability: By bundling related data with enum constants, code becomes more understandable and self-documenting.
Type Safety: Each enum constant maintains type integrity, reducing errors related to data mismatches.
Maintainability: Consolidating data within enums improves code organization and reduces dependency on external structures.
Behavioral Methods: Enums can include methods to perform actions based on their associated data, enhancing versatility.
Conclusion
Enhanced enums in Flutter are a powerful tool that can help you write more organized and maintainable code. By adding fields, constructors, and methods to your enums, you can encapsulate more logic and data within them. This makes your code cleaner and easier to manage.
We hope this guide has helped you understand how to use enhanced enums in your Flutter projects. Now, go ahead and give it a try in your own app. Happy coding!
For insightful tips and best practices follow me on linkedin.
Subscribe to my newsletter
Read articles from Bhoomit Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
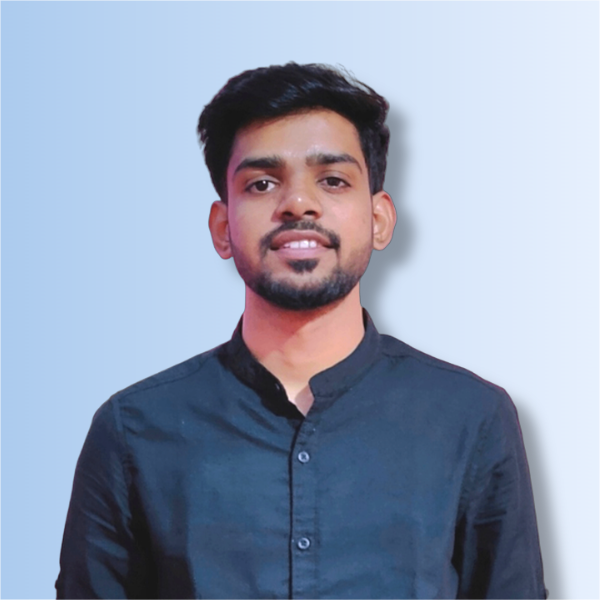
Bhoomit Patel
Bhoomit Patel
Flutter Developer with 4 years of experience creating user-friendly apps. Passionate about transforming ideas into efficient, beautiful solutions using Flutter. Skilled in UI/UX design and backend integration, with a creative approach to problem-solving and innovation.