Optional chaining in JavaScript

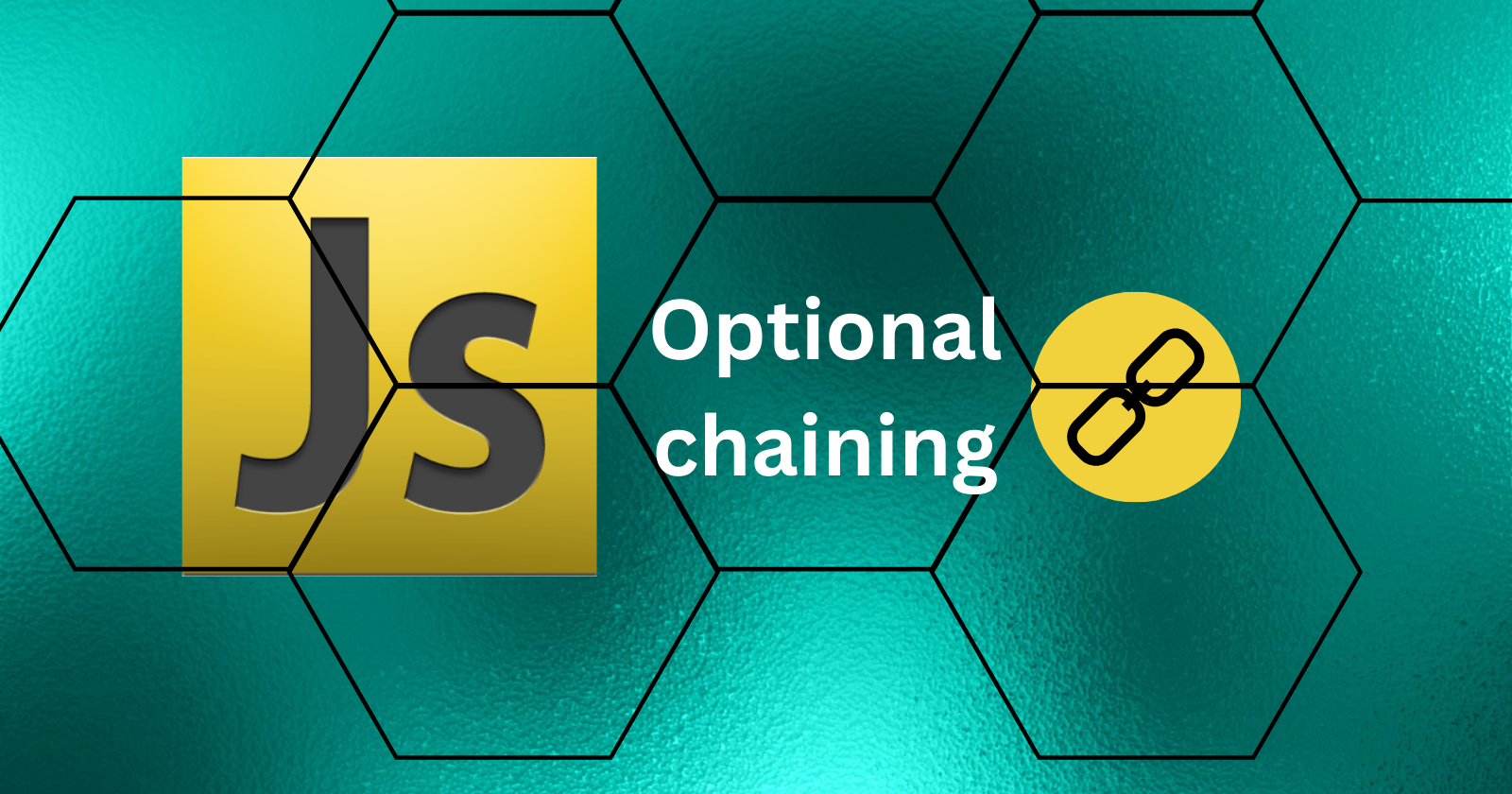
Hey Welcome Techies👋🏼
Hello everyone, my name is Aryan Sharma, and I hope you're all doing well.
I'm thrilled to begin this JavaScript blog on Transpilers and Pollyfills for absolute beginners.
You can find my other blogs on JavaScripthere↗️
Optional Chaining
We can say like it is used when we don't want an error code,
this occurs when we access an object's property that does not give an output or having null
or undefined
value, the expression returns to undefined
instead of throwing an error.
// Without Optional Chaining
const country = user.address && user.address.city && user.address.city.country;
// With Optional Chaining
const country = user?.address?.city?.country;
The syntax is straightforward – a question mark (?
) is placed before each property or method that may be null or undefined.
Some Points...
The optional chaining
?.
stops the evaluation if the value before?.
isundefined
ornull
and returnsundefined
.In other words,
value?.prop
:works as
value.prop
, ifvalue
exists,otherwise (when
value
isundefined/null
) it returnsundefined
.
Overuse of Optional Chaining
What is the user if there isn't a single variable user?Anything can cause an error to occur.
user?.name // gives an error
// user not defined
The declaration of variables that is var let const or object should be done, optional chaining only works for the declared variables.
Use Cases for Optional Chaining
We can classify optional chaining into some cuse cases according to the type of data it is dealing with.
Nested Object Properties:
const city = user?.address?.city;
Array Elements:
const thirdElement = myArray?.[2];
Function Calls:
const result = myFunction?.();
Combining with Nullish Coalescing:
const name = user?.name ?? 'Default Name';
As we said, ?.
stop the code from executing further and the left part doesn’t exist.
This concept is called short-circuiting.
Short-circuiting
In JavaScript, short-circuiting behavior is applicable to logical operators like &&
(logical AND) and ||
(logical OR). Here's how it works in JavaScript:
Logical AND (&&
):
If the left operand is falsy (e.g.,
false
,0
,null
,undefined
,NaN
), JavaScript does not evaluate the right operand, and the result is the falsy value of the left operand.If the left operand is truthy, JavaScript evaluates the right operand and returns its value.
Example:
javascriptCopy codelet result = false && someFunction();
Logical OR (||
):
If the left operand is truthy, JavaScript does not evaluate the right operand, and the result is the truthy value of the left operand.
If the left operand is falsy, JavaScript evaluates the right operand and returns its value.
Example:
javascriptCopy codelet result = true || someFunction();
Short-circuiting is often used in JavaScript to write concise and efficient code.
Other examples: ?.(), ?.[]
The optional chaining ?.
is not an operator, but a special syntax construct, that also works with functions and square brackets.
Thanks for reading!!
Don't forget to follow this blog💖
Subscribe to my newsletter
Read articles from aryan sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

aryan sharma
aryan sharma
Hey, Awesome ones! Aryan this side👋 Full-Stack Developer, Life-Long Learner, Optimistic Using this blog to help code newbies. Learn with me! :)