Building a Mini Computer with Raspberry Pi: A Comprehensive Guide (MiniMind)

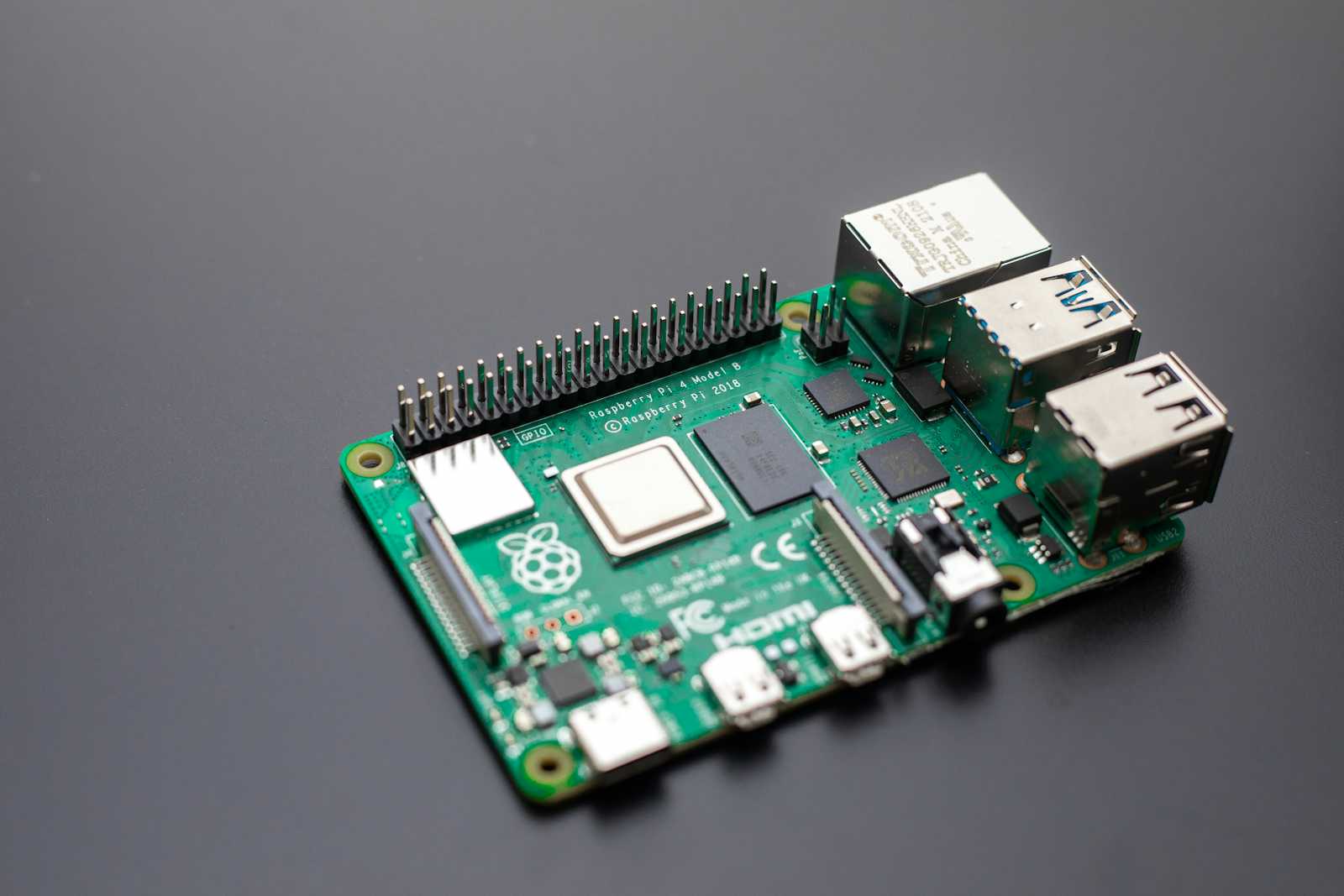
Building a mini computer using a Raspberry Pi is an exciting project that combines creativity, technical skills, and practical knowledge. This guide will walk you through the process, adhering to best practices for design, incorporating feedback, innovating for performance, and managing timelines effectively.
Step 1: Conceptual Design and Planning
Objective: Develop a conceptual design for your mini computer using a Raspberry Pi.
Define Requirements: Identify the purpose of your mini computer (e.g., media center, personal server, educational tool).
Select Raspberry Pi Model: Choose a model that meets your requirements. For most projects, the Raspberry Pi 4 Model B is ideal due to its processing power and versatility.
List Components: Compile a list of necessary components, including the Raspberry Pi, power supply, case, cooling system, storage (microSD card), peripherals (keyboard, mouse), and display.
Key Metrics:
Cost: Total budget for components.
Compatibility: Ensure all components are compatible with your chosen Raspberry Pi model.
Step 2: Detailed Design Using CAD
Objective: Create a detailed CAD design for the mini computer housing and internal layout.
CAD Software: Use software like AutoCAD or Fusion 360 to design the case and internal layout.
Component Placement: Designate space for the Raspberry Pi, cooling system (heatsinks, fans), and cable management.
Accessibility: Ensure ports (USB, HDMI, audio) are easily accessible.
Cooling System: Integrate a cooling system to maintain optimal operating temperature.
Key Metrics:
Thermal Efficiency: Adequacy of the cooling system (measured in °C).
Space Utilization: Efficient use of internal space for component placement.
Step 3: Prototype and Assemble
Objective: Assemble the components based on the detailed CAD design.
3D Print or Fabricate Case: Use a 3D printer or other fabrication methods to create the case.
Component Installation: Carefully install the Raspberry Pi, cooling system, and other components into the case.
Cable Management: Organize and secure cables to prevent obstruction and ensure airflow.
Key Metrics:
Build Time: Time taken to assemble the mini computer.
Fit and Finish: Quality and precision of assembly.
Step 4: Attaching the Screen
Objective: Attach and configure a screen to the Raspberry Pi via GPIO pins.
Select a Screen: Choose a screen that fits your design and meets your size and resolution requirements. The official Raspberry Pi touchscreen display is a common choice.
Mount the Screen: Secure the screen to the case using appropriate mounts or stands. Ensure it is positioned for optimal viewing and access.
Connect to GPIO: Connect the screen to the Raspberry Pi using the GPIO pins. Refer to the screen's documentation for the correct GPIO pin configuration and wiring.
Install Screen Driver:
Before connecting the screen, ensure you have installed the appropriate driver for the Raspberry Pi's GPIO-connected screen. Follow these steps to install the driver:
# Update package list sudo apt-get update # Install required packages sudo apt-get install -y git # Clone the repository containing the screen driver git clone https://github.com/goodtft/LCD-show.git # Navigate to the directory cd LCD-show # Run the script to install the driver sudo ./LCD35-show # Replace 'LCD35-show' with appropriate script for your screen model # Reboot the Raspberry Pi for changes to take effect sudo reboot
Configure Display Settings: After rebooting, configure the display settings to ensure the screen works correctly. Adjust resolution, orientation, and touchscreen calibration if applicable.
Key Metrics:
Display Quality: Resolution and clarity of the screen.
Connection Stability: Reliability of the screen connection.
Ease of Use: User experience with the screen interface.
Step 5: Software Installation
Objective: Install the operating system and necessary software on the Raspberry Pi.
Choose OS: Select an operating system (e.g., Raspberry Pi OS, Ubuntu, RetroPie).
Install OS: Write the OS image to the microSD card and boot up the Raspberry Pi.
Install Applications: Set up necessary applications based on your mini computer's purpose (e.g., media server software, development tools).
Key Metrics:
Installation Time: Duration of OS and software installation.
System Performance: Boot time and responsiveness of the system.
Step 6: Testing and Feedback
Objective: Test the mini computer's performance and gather feedback for improvements.
Functional Testing: Verify that all components (USB ports, HDMI, network) are working correctly.
Performance Testing: Measure system performance under various loads.
Feedback Collection: Gather feedback from users and make necessary adjustments.
Key Metrics:
System Stability: Number of crashes or errors during testing.
User Satisfaction: Feedback rating from users.
Step 7: Innovation and Optimization
Objective: Innovate and optimize the mini computer for better performance and user experience.
Performance Tweaks: Optimize the operating system and applications for better performance.
Hardware Upgrades: Consider adding hardware upgrades such as additional cooling, more storage, or a better power supply.
Customizations: Personalize the mini computer with custom scripts, themes, or unique functionalities.
Key Metrics:
Cycle Time: Time taken to implement optimizations.
Performance Gains: Improvement in key performance metrics (e.g., processing speed, thermal performance).
Step 8: Face Recognition Integration
Objective: Integrate face recognition capabilities using the Raspberry Pi and the facerecognition repository.
Clone the Repository: Clone the facerecognition repository using Git.
git clone https://github.com/niladrridas/facerecognition.git
Install Dependencies: Install the required dependencies for face recognition, including OpenCV and dlib.
pip install opencv-python dlib
Configure Face Recognition: Configure the face recognition system to recognize faces using the Raspberry Pi's camera module.
import cv2 import dlib # Initialize the camera cap = cv2.VideoCapture(0) # Load the face recognition model detector = dlib.get_frontal_face_detector() while True: ret, frame = cap.read() gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) faces = detector(gray, 0) for face in faces: x, y = face.left(), face.top() cv2.rectangle(frame, (x, y), (x + face.width(), y + face.height()), (0, 255, 0), 2) cv2.imshow('Face Recognition', frame) if cv2.waitKey(1) & 0xFF == ord('q'): break cap.release() cv2.destroyAllWindows()
Train the Model: Train the face recognition model using a dataset of images.
import os import cv2 import dlib # Load the face recognition model detector = dlib.get_frontal_face_detector() # Create a directory for the dataset dataset_dir = 'dataset' if not os.path.exists(dataset_dir): os.makedirs(dataset_dir) # Train the model using the dataset for file in os.listdir(dataset_dir): img = cv2.imread(os.path.join(dataset_dir, file)) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) faces = detector(gray, 0) for face in faces: x, y = face.left(), face.top() cv2.rectangle(img, (x, y), (x + face.width(), y + face.height()), (0, 255, 0), 2) cv2.imshow('Training', img) cv2.waitKey(1) cv2.destroyAllWindows()
Key Metrics:
Face Recognition Accuracy: Accuracy of the face recognition system in identifying faces.
Recognition Speed: Time taken to recognize a face.
Step 9: Chatbot Integration
Objective: Integrate the chatbot repository to enable voice commands and conversational interactions.
Clone the Repository: Clone the chatbot repository using Git.
git clone https://github.com/niladrridas/chatbot.git
Install Dependencies: Install the required dependencies for the chatbot, including NLTK and spaCy.
pip install nltk spacy
Configure Chatbot: Configure the chatbot to recognize voice commands and respond accordingly.
import speech_recognition as sr import nltk # Initialize the speech recognition engine r = sr.Recognizer() # Load the chatbot model chatbot = nltk.Chatbot() while True: with sr.Microphone() as source: audio = r.listen(source) try: text = r.recognize_google(audio, language='en-US') response = chatbot.respond(text) print(response) except sr.UnknownValueError: print("Sorry, I didn't understand that.")
Key Metrics:
Voice Command Accuracy: Accuracy of the chatbot in recognizing voice commands.
Response Time: Time taken to respond to voice commands.
Step 10: Voice Assistant Chatbot Integration
Objective: Integrate the voice assistant chatbot repository to enable advanced conversational interactions.
Clone the Repository: Clone the voice assistant chatbot repository using Git.
git clone https://github.com/niladrridas/voice-assistant-chatbot.git
Install Dependencies: Install the required dependencies for the voice assistant chatbot, including PyTorch and transformers.
pip install torch transformers
Configure Voice Assistant Chatbot: Configure the voice assistant chatbot to recognize voice commands and respond accordingly.
import torch import transformers # Load the voice assistant chatbot model model = transformers.VoiceAssistantChatbot() while True: with sr.Microphone() as source: audio = r.listen(source) try: text = r.recognize_google(audio, language='en-US') response = model.respond(text) print(response) except sr.UnknownValueError: print("Sorry, I didn't understand that.")
Key Metrics:
Conversational Accuracy: Accuracy of the voice assistant chatbot in understanding conversational context.
Response Time: Time taken to respond to voice commands.
Step 11: Documentation and Maintenance
Objective: Document the build process and establish a maintenance plan.
Documentation: Create a comprehensive guide detailing the build process, components used, and any custom configurations.
Maintenance Plan: Outline a maintenance plan for software updates and hardware checks.
Key Metrics:
Documentation Quality: Clarity and thoroughness of the documentation.
Maintenance Frequency: Recommended intervals for system checks and updates.
Conclusion
Building a mini computer with a Raspberry Pi involves careful planning, detailed design, and iterative improvements. By following this guide and focusing on key metrics such as cost, thermal efficiency, build time, system performance, and user satisfaction, you can create a powerful and efficient mini computer tailored to your needs. Additionally, integrating face recognition capabilities using the facerecognition repository, chatbot integration using the chatbot repository, and voice assistant chatbot integration using the voice assistant chatbot repository can enhance the mini computer's functionality and user experience. Whether for personal use or as an educational project, this process will enhance your technical skills and provide a rewarding experience.
You can visit the web page for this project at https://niladrridas.github.io/device/ to learn more about the mini computer and its capabilities.
Here are the features of the project:
This project combines the power of Raspberry Pi, face recognition, and conversational AI to create a unique and interactive device. The device can recognize and respond to individual faces, engage in conversations, and even control other devices. In this article, we'll explore the features and functionalities of this project, as well as how it can be used as a Network-Attached Storage (NAS) device.
Hardware Features:
Raspberry Pi: The project is built around a Raspberry Pi, a small, low-cost, and highly capable single-board computer.
Touchscreen Display: The device features a touchscreen display, allowing users to interact with the device using touch inputs.
Camera Module: The project includes a camera module, which enables face recognition and other computer vision capabilities.
Microphone and Speaker: The device has a microphone and speaker, allowing for voice commands and audio output.
External Storage: The device can be connected to external storage devices, such as hard drives or SSDs, to expand its storage capacity.
Software Features:
Face Recognition: The project includes face recognition capabilities, allowing the device to recognize and respond to individual faces.
Chatbot Integration: The device is integrated with a chatbot, enabling users to interact with the device using natural language processing (NLP) and conversational AI.
Voice Assistant: The project includes a voice assistant, allowing users to control the device and access information using voice commands.
Operating System: The device runs on a customized operating system, likely a variant of Raspbian, the official OS for Raspberry Pi.
NAS Software: The device can run NAS software, such as OpenMediaVault or NextCloud, to enable file sharing and storage capabilities.
Functionalities:
Face Recognition and Authentication: The device can recognize and authenticate individual faces, allowing for secure access and personalized experiences.
Conversational Interface: Users can interact with the device using natural language, asking questions, and receiving responses.
Voice Commands: The device responds to voice commands, allowing users to control the device and access information hands-free.
Personalized Experience: The device can provide a personalized experience, tailoring its responses and interactions to individual users.
File Sharing and Storage: The device can be used as a NAS, allowing users to store and share files across a network.
Using the Device as a NAS:
To use the device as a NAS, follow these steps:
Install NAS Software: Install NAS software, such as OpenMediaVault or NextCloud, on the Raspberry Pi.
Configure Storage: Configure the external storage devices, such as hard drives or SSDs, to be used with the NAS software.
Set up File Sharing: Set up file sharing protocols, such as SMB or AFP, to enable access to the stored files.
Connect to the Network: Connect the device to a network, either wirelessly or through a wired connection.
Access Files: Users can then access the stored files from other devices on the network, using the device's IP address or hostname.
Benefits of Using the Device as a NAS:
Centralized Storage: The device provides a centralized storage solution, allowing users to access and share files from a single location.
Secure Access: The device's face recognition and authentication capabilities ensure secure access to the stored files.
Convenient Access: Users can access the stored files using voice commands or conversational interfaces, making it easy to retrieve and share files.
Cost-Effective: The device is a cost-effective solution for file sharing and storage, using low-cost hardware and open-source software.
Other Features:
Customizable: The project is highly customizable, allowing users to modify and extend the device's capabilities using various programming languages and tools.
Cost-Effective: The project is built using low-cost, widely available components, making it an affordable option for developers and users.
Compact and Portable: The device is compact and portable, making it easy to integrate into various environments and applications.
These features and functionalities make the project a perfect blend of innovation and practicality, offering a unique and powerful tool for developers, researchers, and users interested in AI, computer vision, and conversational interfaces. By seamlessly integrating the power of Raspberry Pi, face recognition, and conversational AI, this project creates a flawless and interactive device that can be used as a NAS, providing a centralized, secure, and highly efficient storage solution for users.
Subscribe to my newsletter
Read articles from Niladri Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
