Learn to Develop an Age Calculator Using HTML, CSS, and JavaScript
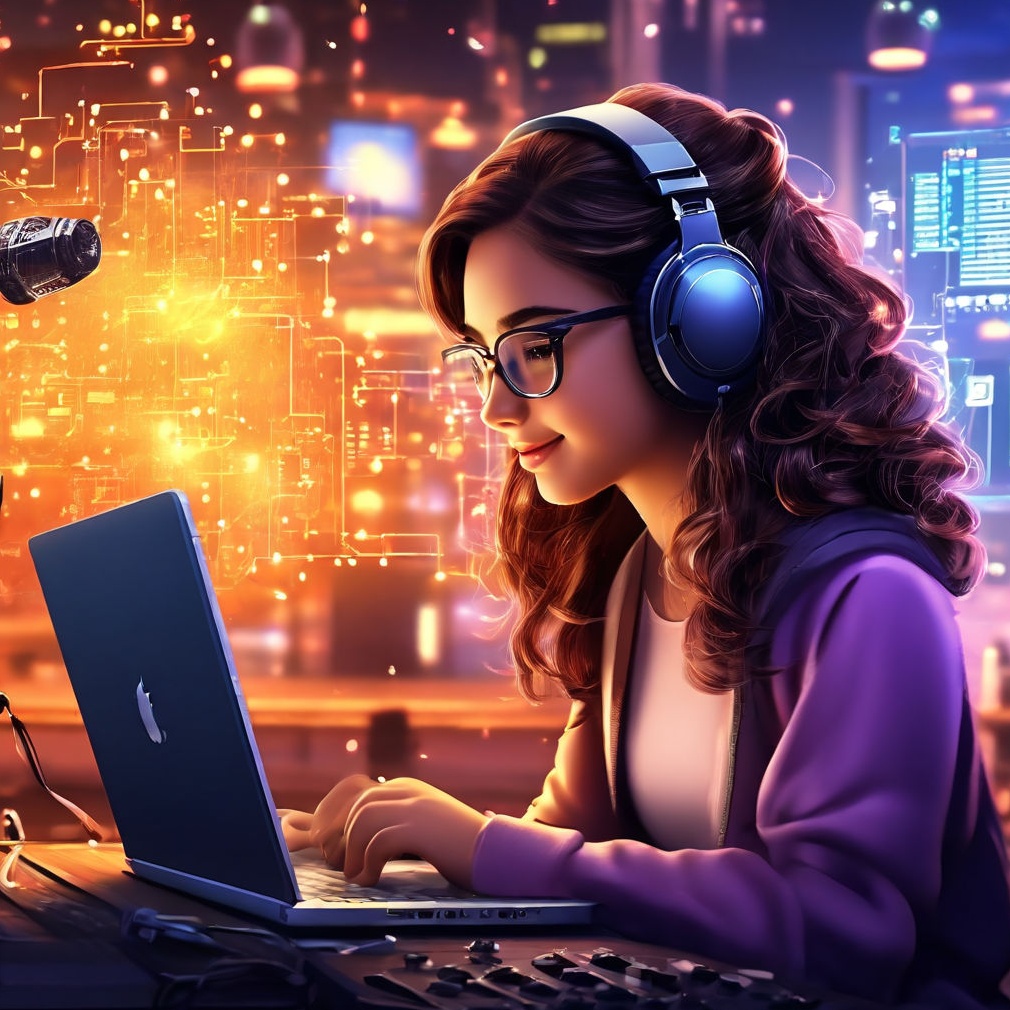
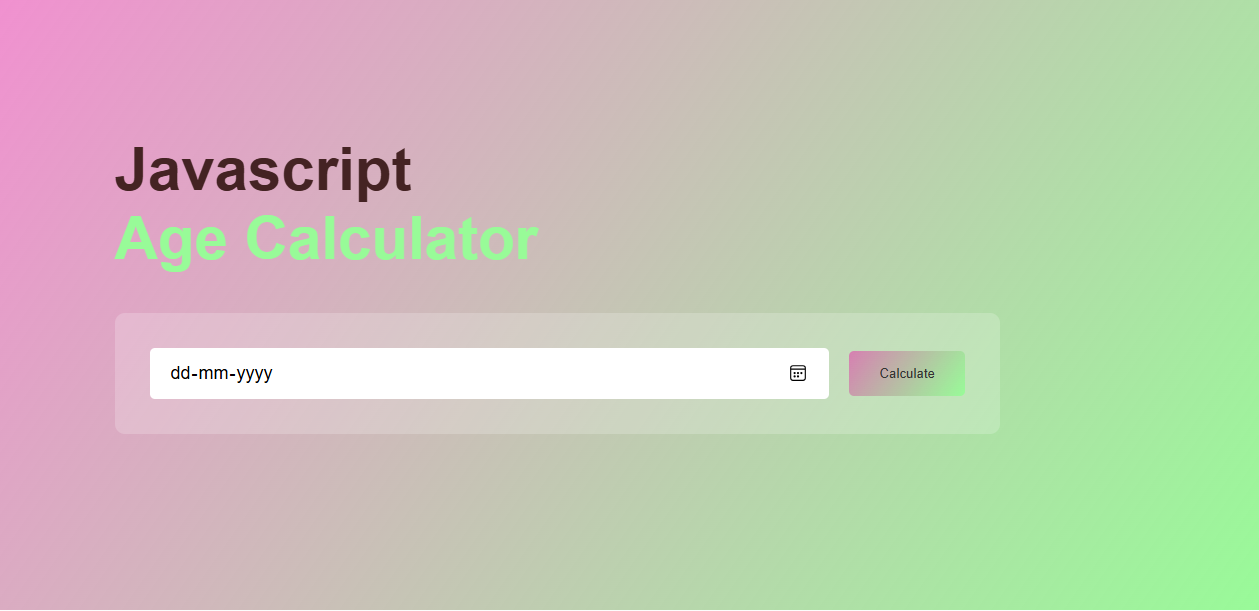
<HTML/>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Age Calculator</title>
</head>
<body>
<div class="container">
<div class="calculator">
<h1>Javascript<br><span>Age Calculator</span></h1>
<div class="input-box">
<input type="date" id="date">
<button onclick="calculateAge()">Calculate</button>
</div>
<p id="ageDetails"></p>
</div>
</div>
<script src="script.js">
</script>
</body>
</html>
After typing all the necessary parts, we can move into the body tag.
The first div with the class "container" will contain the elements we are going to add.
Inside this div, there is another div with the class name "calculator." It will contain the heading, input-box, and a calculate button.
Then, add an onclick function to the calculate button. The function is called calculateAge();
(we will explain this in the JavaScript part).
The input box has an input type of date, which will automatically show a calendar panel when clicked. Also, add an ID for future use in JavaScript.
Lastly, add a paragraph tag that will display the calculated age of a person below the calculator.
function calculateAge() {
var birthDate = new Date(document.getElementById('date').value);
var today = new Date();
// Calculate age
var yearsDiff = today.getFullYear() - birthDate.getFullYear();
var monthsDiff = today.getMonth() - birthDate.getMonth();
var daysDiff = today.getDate() - birthDate.getDate();
// Adjust negative months
if (monthsDiff < 0 || (monthsDiff === 0 && daysDiff < 0)) {
yearsDiff--;
monthsDiff += 12;
}
// Adjust negative days
if (daysDiff < 0) {
monthsDiff--;
var tempDate = new Date(today.getFullYear(), today.getMonth(), 0);
daysDiff = tempDate.getDate() - birthDate.getDate() + today.getDate();
}
// Display results
document.getElementById('ageDetails').innerText = 'You are ' + yearsDiff + ' years, ' + monthsDiff + ' months, and ' + daysDiff + ' days old.';
}
The calculateAge()
function starts by assigning values to variables. var
means variable.
var birthDate = new Date(document.getElementById('date').value);
We get the value of the element identified by its ID name "date". This value is a string, so to convert it into a date, we use the new Date()
function. The output of this function is assigned to a variable named birthDate
.
newdate(); function will give the current date so save that in a variable as today.
// Calculate age
var yearsDiff = today.getFullYear() - birthDate.getFullYear();
var monthsDiff = today.getMonth() - birthDate.getMonth();
var daysDiff = today.getDate() - birthDate.getDate();
getFullYear()
, getMonth()
, and getDate()
are functions used to get specific parts of a date. The differences are then assigned to three variables accordingly.
For example:
yearsDiff = today's year - your birth year, which means
22 = 2024 - 2002
monthsDiff = 06 - 04 and daysDiff = 23 - 21.
What if the month or the date values got as negative?
// Adjust negative months
if (monthsDiff < 0 || (monthsDiff === 0 && daysDiff < 0)) {
yearsDiff--;
monthsDiff += 12;
}
So, if the monthDiff is less than 0 or (monthDiff === 0 and daysDiff is less than 0), which means either one of the conditions is true, the function will be executed.
For example:
monthDiff = 06 - 10 = -4
Then the year will be reduced by 1, and 12 will be added to the monthDiff.
So, 2023/06 - 2024/10 means:
1 year - 4 months. As per the condition, if the month is less than 0, the year is reduced by 1, and 12 is added to the month. 1 becomes 0, and -4 + 12 = 8. So the output will be 0 years and 8 months old.
// Adjust negative days
if (daysDiff < 0) {
monthsDiff--;
var tempDate = new Date(today.getFullYear(), today.getMonth(), 0);
daysDiff = tempDate.getDate() - birthDate.getDate() + today.getDate();
}
If the daysDiff is less than 0, then monthDiff is reduced by 1. We need another variable to complete this calculation. The variable is named var tempDate = new Date(today.getFullYear(), today.getMonth(), 0);
, which represents the last day of the previous month of the current year.
So, the daysDiff will be calculated as tempDate.getDate() - birthDate.getDate() + today.getDate();
.
For example:
2024/06/23 - today's date and 2002/04/29 - birth date. When we subtract them, we get:
22 years, 2 months, -6 days.
According to the condition, the month will be reduced by 1, so:
22 years, 1 month, -6 days.
Then, daysDiff is calculated as the previous month's days - birthDate + today's date.
daysDiff = 31 - 29 + 23 = 25 days.
The output will be 22 years, 1 month, and 25 days old.
Lastly, we need to display the results:
// Display results
document.getElementById('ageDetails').innerText = 'You are ' + yearsDiff + ' years, ' + monthsDiff + ' months, and ' + daysDiff + ' days old.';
}
Already assign the paragraph element by the id name ageDetails. With the innerHTML function, we can display the result after clicking the calculate button.
By using the onclick function, when we click on calculate, it will perform the function calculateAge();
and display the result in the paragraph element's .innerHTML
.
To check out the CSS properties I used for this project, visit the link below for the source code.
Source Code: https://github.com/VaithegiV/Age-Calculator.git
Live link of the project: https://vaithegiv.github.io/Age-Calculator/
Happy coding!
Subscribe to my newsletter
Read articles from VaithegiV directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
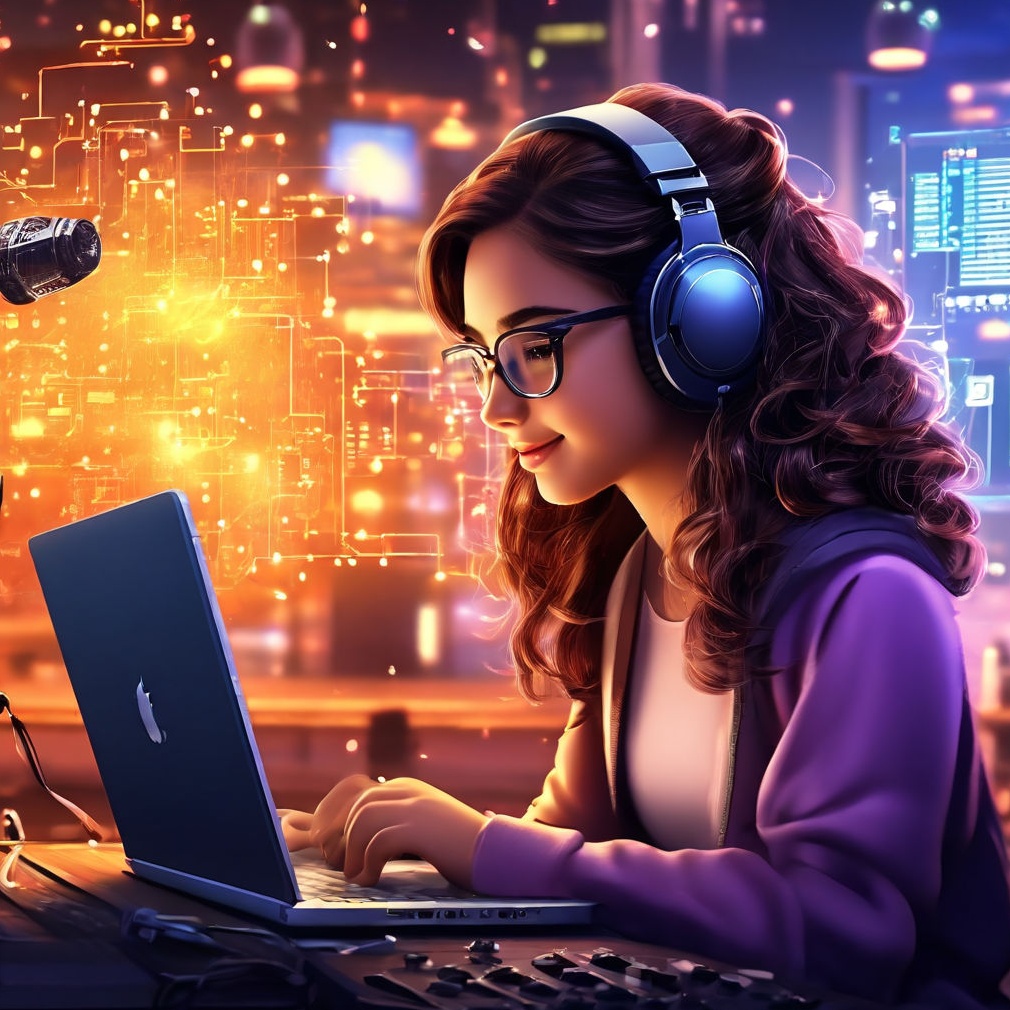
VaithegiV
VaithegiV
Hi, I'm VaithegiV Frontend developer. B.Tech graduate with a passion for web development. Proficient in HTML, CSS, and JavaScript, I strive to create intuitive and visually appealing digital experiences. With expertise in Git and GitHub, I ensure efficient collaboration and version control in projects. My dedication to continuous learning drives me to stay updated with the latest trends in technology and design. I am eager to contribute my skills and creativity to innovative projects that push boundaries and deliver exceptional user experiences.