Open-Close Principle: Keep Your Code Fresh and Flexible!
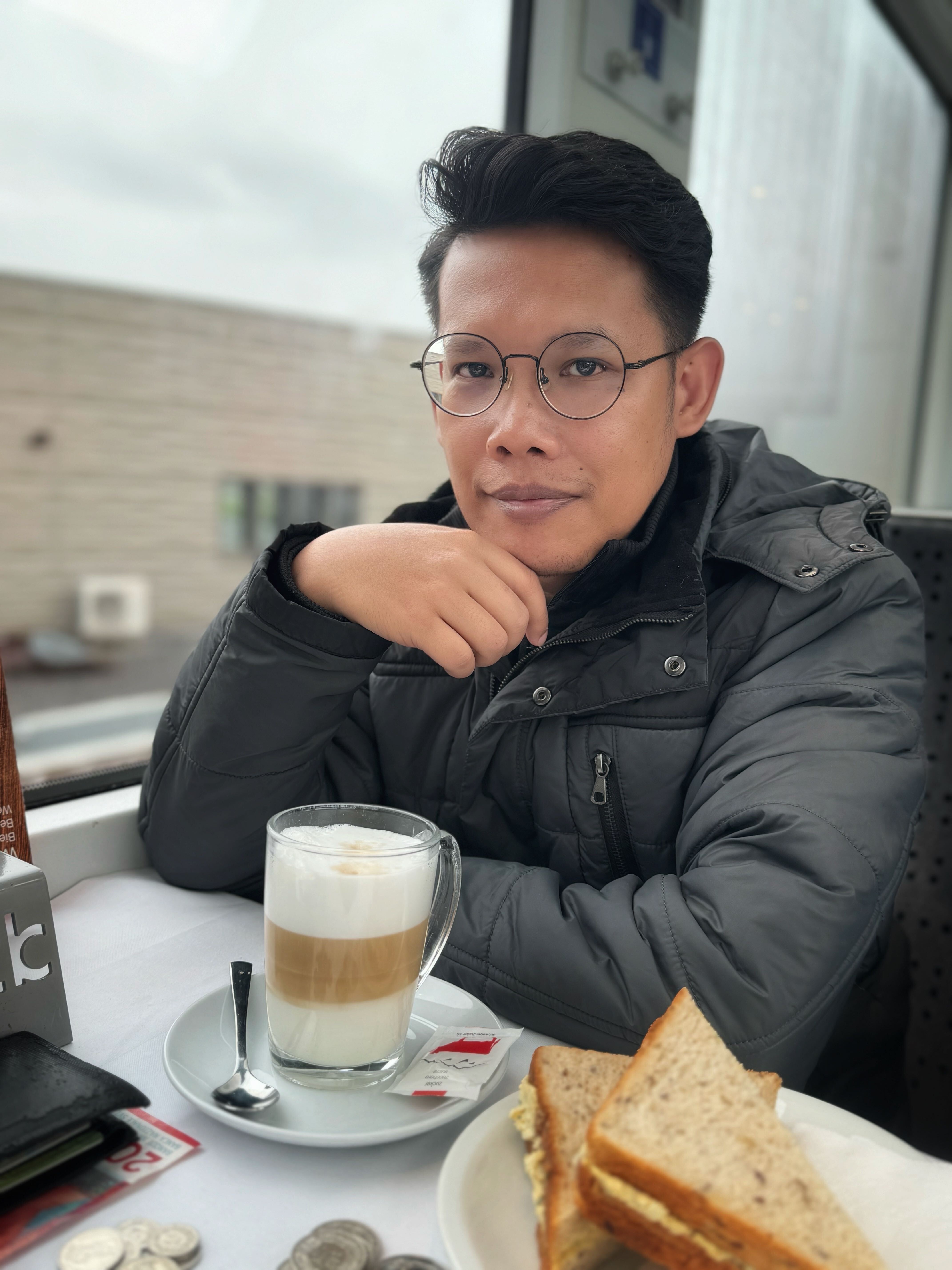
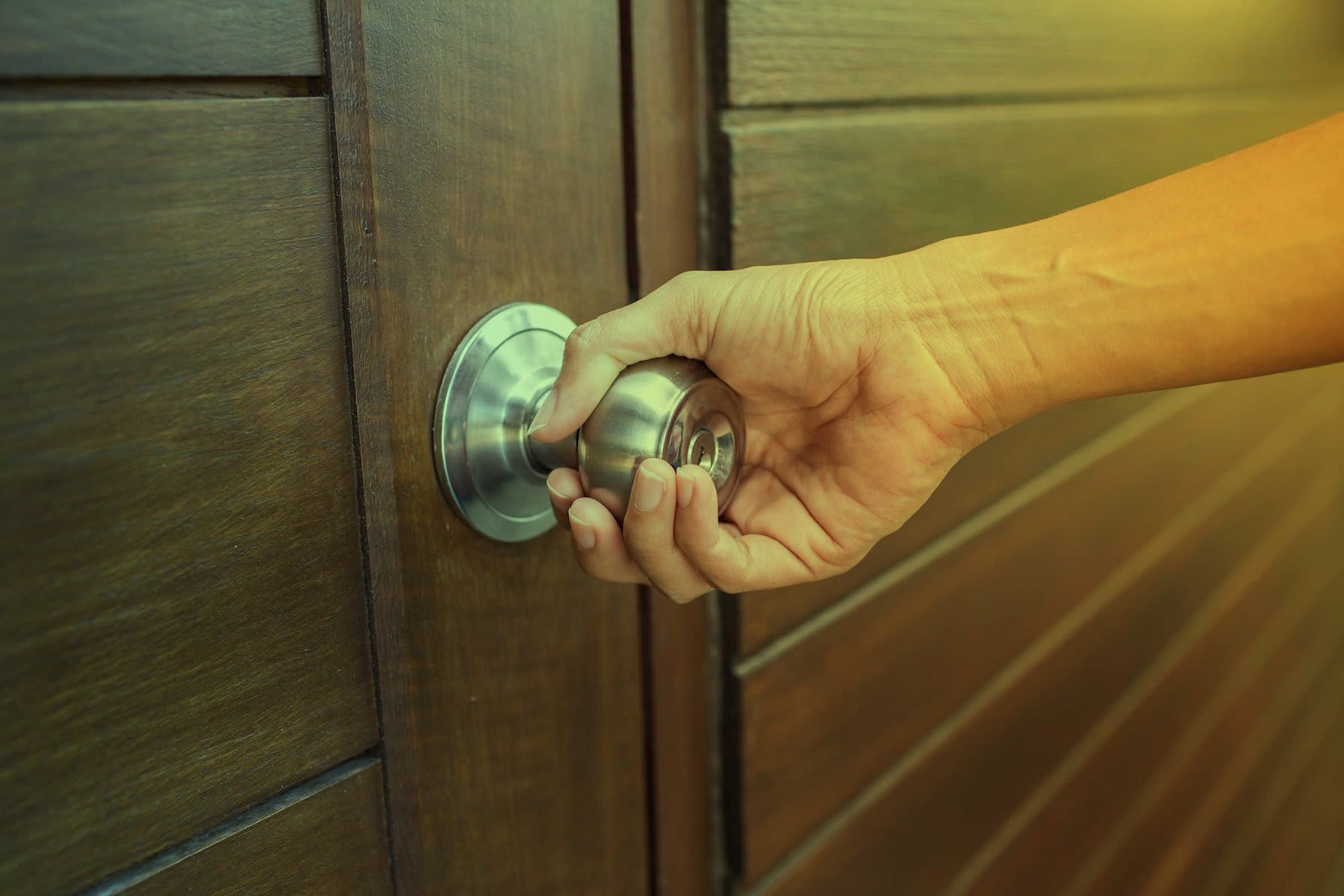
Welcome back, fellow coders! Today, we're diving into the Open-Closed Principle (OCP), one of the five SOLID principles that help us write better, cleaner, and more maintainable code. If you're just tuning in, the SOLID principles are a set of guidelines for object-oriented design that stand for Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion. But let's zero in on today's topic: the Open-Closed Principle.
What Is the Open-Closed Principle?
The Open-Closed Principle was introduced by Bertrand Meyer, and it's all about writing code that is easy to extend without needing to change what's already working. The principle states that software entities (like classes, modules, and functions) should be open for extension but closed for modification. This means you should be able to add new features or behaviors without altering the existing codebase.
Breaking It Down: Open for Extension, Closed for Modification
Open for Extension
This means you can add new functionalities to your software. Think of it like adding new apps to your smartphone without needing to rewire the whole device. In programming, this is often achieved through interfaces, abstract classes, or design patterns like Strategy, Decorator, and Observer.
Closed for Modification
Your existing code should remain untouched when you add new features. This ensures stability and minimizes the risk of introducing bugs into code that’s already been tested and proven to work.
A Common OCP Violation
Let's consider a simple example: a report generation system that generates reports in PDF or CSV format. Here’s how this might look in code:
public class ReportGenerator {
public void generateReport(String data, String reportType) {
if ("PDF".equals(reportType)) {
// Generate PDF report
System.out.println("Generating PDF report");
} else if ("CSV".equals(reportType)) {
// Generate CSV report
System.out.println("Generating CSV report");
}
// To add a new report format, you must modify this class and add another condition here.
}
}
public class Main {
public static void main(String[] args) {
ReportGenerator generator = new ReportGenerator();
generator.generateReport("Some data", "PDF");
}
}
Why This Violates OCP
Modification Required for Extension: To add a new report format (like XML), you’d need to modify the
ReportGenerator
class, which goes against the principle of keeping existing code closed for modification.Scalability Issue: Adding more formats increases the complexity of the
generateReport
method, making it harder to maintain.
How to Adhere to OCP
To stick to the Open-Closed Principle, we can refactor the code to use polymorphism. Here's how you can do it:
interface ReportGenerator {
void generateReport(String data);
}
class PDFReportGenerator implements ReportGenerator {
public void generateReport(String data) {
System.out.println("Generating PDF report, data: " + data);
}
}
class CSVReportGenerator implements ReportGenerator {
public void generateReport(String data) {
System.out.println("Generating CSV report, data: " + data);
}
}
// Adding a new format does not require modifying existing classes, just add a new class:
class XMLReportGenerator implements ReportGenerator {
public void generateReport(String data) {
System.out.println("Generating XML report, data: " + data);
}
}
class ReportService {
private ReportGenerator reportGenerator;
public ReportService(ReportGenerator reportGenerator) {
this.reportGenerator = reportGenerator;
}
public void generateReport(String data) {
reportGenerator.generateReport(data);
}
}
public class Main {
public static void main(String[] args) {
ReportService pdfReportService = new ReportService(new PDFReportGenerator());
pdfReportService.generateReport("PDF Data");
ReportService csvReportService = new ReportService(new CSVReportGenerator());
csvReportService.generateReport("CSV Data");
ReportService xmlReportService = new ReportService(new XMLReportGenerator());
xmlReportService.generateReport("XML Data");
}
}
Benefits of This Approach
No Modification Needed: Adding a new report format means creating a new class that implements the
ReportGenerator
interface. The existing code remains unchanged.Enhanced Scalability: The system can grow without becoming more complex or harder to maintain.
Why You Should Care About OCP
Adhering to the Open-Closed Principle has several benefits:
Minimize Regression Bugs: Less risk of new bugs when you don’t have to change existing, working code.
Improve Maintainability: Code becomes more modular and easier to understand, maintain, and extend.
Boost Scalability: New features can be added without disrupting the existing system.
Enhance Reusability: Components designed with OCP in mind are more likely to be reusable across different parts of the application or even different projects.
Promote Good Design Practices: Encourages the use of interfaces and abstract classes, leading to a more flexible and robust architecture.
Facilitate Testing and Integration: Well-defined interfaces make it easier to test new functionalities and integrate new components seamlessly.
Support Agile Development: Adapt easily to new requirements without compromising the stability of your application.
Using Design Patterns to Achieve OCP
Strategy Pattern
The Strategy Pattern enables an algorithm's behavior to be selected at runtime. This allows you to define a family of algorithms, encapsulate each one, and make them interchangeable, thus extending capabilities without modifying core logic.
Step-by-Step Guide:
Define Strategy Interface: Create an interface that declares the method(s) used by the algorithms.
Implement Concrete Strategies: Create classes that implement the strategy interface, each providing a different implementation of the algorithm.
Context Class: Create a context class that uses a strategy object. This class will delegate execution to the strategy's method.
Client Configuration: The client configures the context with the desired strategy implementation, allowing dynamic behavior changes.
Example: Payment Processing System
// Step 1: Define Strategy Interface
public interface PaymentStrategy {
void pay(int amount);
}
// Step 2: Implement Concrete Strategies
public class CreditCardPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paying " + amount + " using Credit Card");
}
}
public class PayPalPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paying " + amount + " using PayPal");
}
}
// Step 3: Context Class
public class PaymentContext {
private PaymentStrategy paymentStrategy;
public PaymentContext(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void executePayment(int amount) {
paymentStrategy.pay(amount);
}
}
// Step 4: Client Configuration
public class PaymentSystem {
public static void main(String[] args) {
PaymentContext context;
// Paying with Credit Card
context = new PaymentContext(new CreditCardPayment());
context.executePayment(100);
// Switching to PayPal
context = new PaymentContext(new PayPalPayment());
context.executePayment(150);
}
}
How It Adheres to OCP:
Open for Extension: New payment methods can be added by implementing the
PaymentStrategy
interface.Closed for Modification: Existing strategies and the context class remain unchanged.
Decorator Pattern
The Decorator Pattern allows behavior to be added to individual objects, either statically or dynamically, without affecting other objects. This pattern is useful for extending an object's behavior without modifying its existing code.
Step-by-Step Guide:
Component Interface: Define an interface or abstract class for objects that can have responsibilities added dynamically.
Concrete Component: Implement the interface with a concrete class.
Decorator Class: Create an abstract class implementing the component interface, with a reference to a component object.
Concrete Decorators: Implement the decorator class for each additional responsibility or behavior.
Example: Coffee Making Application
// Step 1: Component Interface
public interface Coffee {
String getDescription();
double cost();
}
// Step 2: Concrete Component
public class SimpleCoffee implements Coffee {
@Override
public String getDescription() {
return "Simple Coffee";
}
@Override
public double cost() {
return 1.0;
}
}
// Step 3: Decorator Class
public abstract class CoffeeDecorator implements Coffee {
protected Coffee decoratedCoffee;
public CoffeeDecorator(Coffee coffee) {
this.decoratedCoffee = coffee;
}
public String getDescription() {
return decoratedCoffee.getDescription();
}
public double cost() {
return decoratedCoffee.cost();
}
}
// Step 4: Concrete Decorators
public class WithMilk extends CoffeeDecorator {
public WithMilk(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return decoratedCoffee.getDescription() + ", Milk";
}
@Override
public double cost() {
return decoratedCoffee.cost() + 0.5;
}
}
public class WithSugar extends CoffeeDecorator {
public WithSugar(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return decoratedCoffee.getDescription() + ", Sugar";
}
@Override
public double cost() {
return decoratedCoffee.cost() + 0.2;
}
}
// Usage
public class CoffeeShop {
public static void main(String[] args) {
Coffee coffee = new SimpleCoffee();
System.out.println(coffee.getDescription() + " $" + coffee.cost());
Coffee milkCoffee = new WithMilk(coffee);
System.out.println(milkCoffee.getDescription() + " $" + milkCoffee.cost());
Coffee sugarMilkCoffee = new WithSugar(milkCoffee);
System.out.println(sugarMilkCoffee.getDescription() + " $" + sugarMilkCoffee.cost());
}
}
How It Adheres to OCP:
Open for Extension: New ingredients (decorators) can be added by implementing a new
CoffeeDecorator
.Closed for Modification: Existing classes remain unchanged.
Observer Pattern
The Observer Pattern involves a subject maintaining a list of dependents, called observers, and notifying them automatically of any state changes. This pattern is useful for adding new dependent objects without modifying the subject.
Step-by-Step Guide:
Subject Interface: Define an interface for attaching, detaching, and notifying observers.
Concrete Subject: Implement the subject interface with a concrete class.
Observer Interface: Define an observer interface with a notification method.
Concrete Observers: Implement the observer interface in concrete classes.
Example: Weather Station
// Step 1: Subject Interface
public interface WeatherSubject {
void attach(WeatherObserver observer);
void detach(WeatherObserver observer);
void notifyObservers();
}
// Step 2: Concrete Subject
public class WeatherStation implements WeatherSubject {
private List<WeatherObserver> observers = new ArrayList<>();
private float temperature;
@Override
public void attach(WeatherObserver observer) {
observers.add(observer);
}
@Override
public void detach(WeatherObserver observer) {
observers.remove(observer);
}
@Override
public void notifyObservers() {
for (WeatherObserver observer : observers) {
observer.update(temperature);
}
}
public void setTemperature(float temperature) {
this.temperature = temperature;
notifyObservers();
}
}
// Step 3: Observer Interface
public interface WeatherObserver {
void update(float temperature);
}
// Step 4: Concrete Observers
public class TemperatureDisplay implements WeatherObserver {
@Override
public void update(float temperature) {
System.out.println("Temperature Display: " + temperature);
}
}
public class ForecastDisplay implements WeatherObserver {
@Override
public void update(float temperature) {
if (temperature > 25) {
System.out.println("Forecast Display: It's going to be a hot day!");
} else {
System.out.println("Forecast Display: It's going to be cool.");
}
}
}
// Usage
public class WeatherApp {
public static void main(String[] args) {
WeatherStation station = new WeatherStation();
TemperatureDisplay tempDisplay = new TemperatureDisplay();
ForecastDisplay forecastDisplay = new ForecastDisplay();
station.attach(tempDisplay);
station.attach(forecastDisplay);
station.setTemperature(30); // Both displays will be updated
station.detach(tempDisplay);
station.setTemperature(20); // Only the forecast display will be updated
}
}
How It Adheres to OCP:
Open for Extension: New types of displays (observers) can be added by implementing the
WeatherObserver
interface.Closed for Modification: The
WeatherStation
class remains unchanged.
Conclusion
In conclusion, the Open-Closed Principle is about building software that is robust, maintainable, and adaptable to change. Using design patterns like Strategy, Decorator, and
Observer, you can keep your code fresh, flexible, and ready for whatever comes next. Happy coding!
Subscribe to my newsletter
Read articles from Suhaimi Sulaiman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
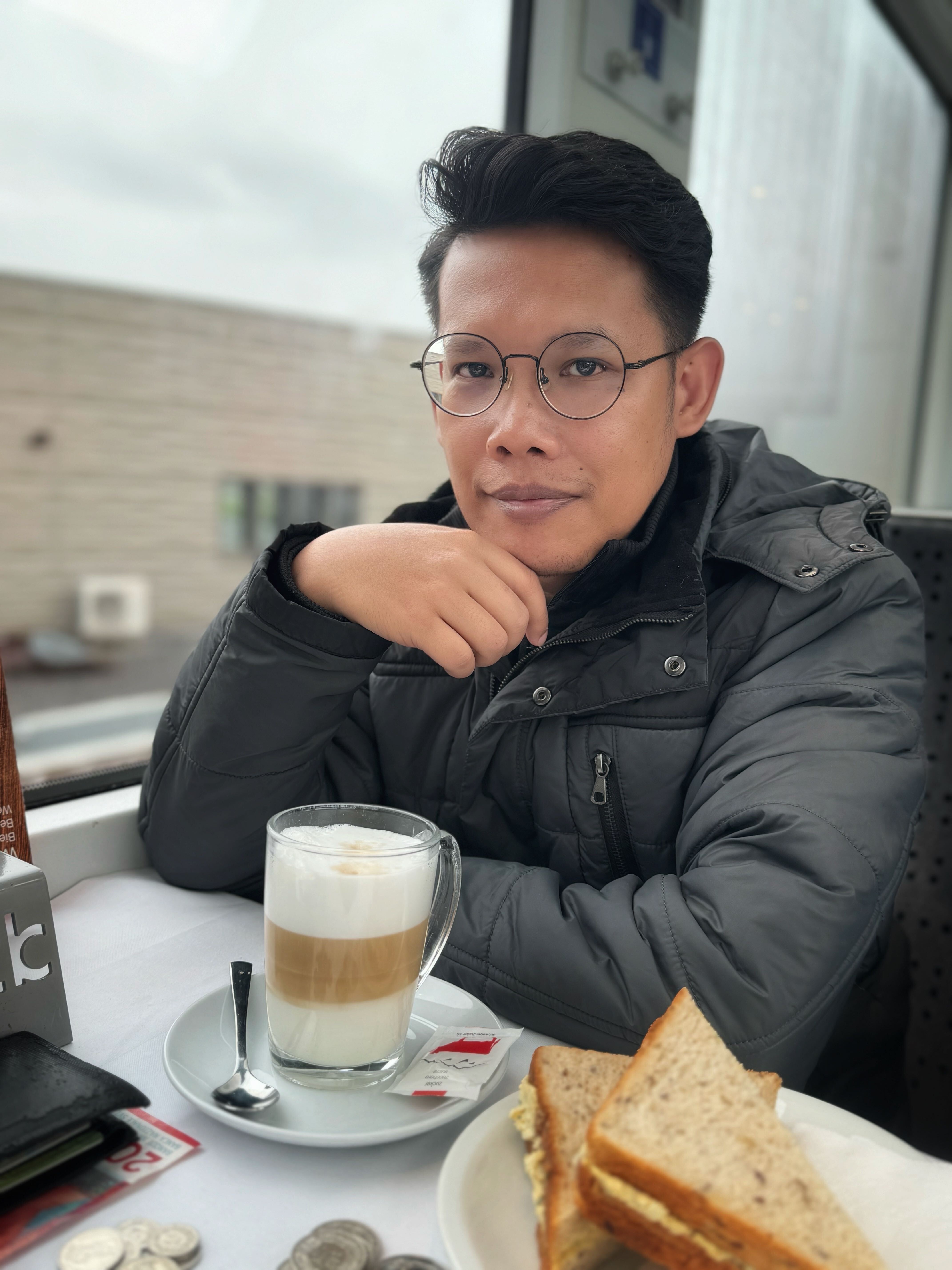
Suhaimi Sulaiman
Suhaimi Sulaiman
I am a Team Lead cum Backend Engineer at Setel Ventures Sdn Bhd.