Mastering .NET CLR: A Precise Overview

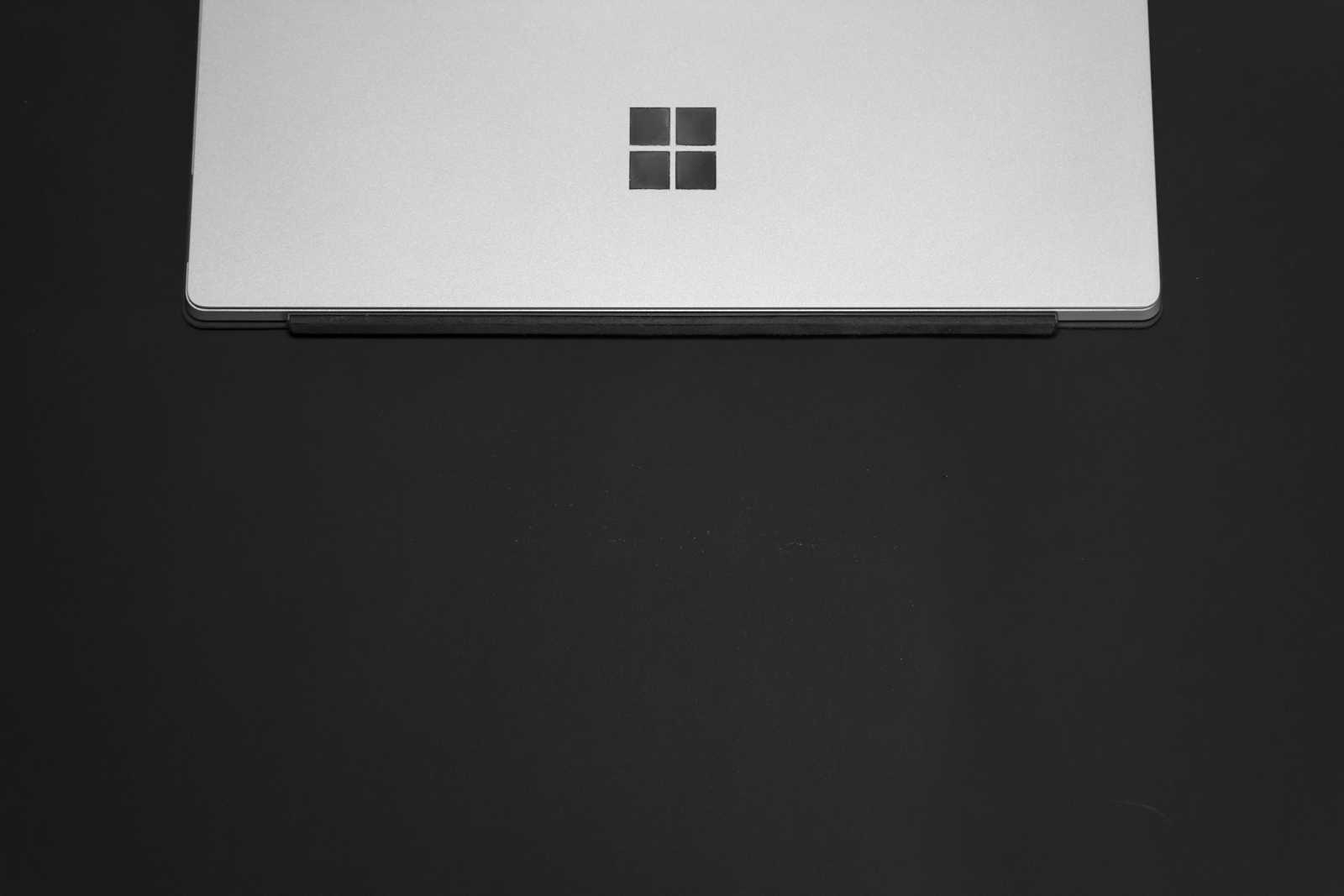
This blog post will delve into the core components of the CLR, its garbage collection process, and how it optimizes program execution.
Before getting started with CLR, It's crucial to grasp how source code is transformed into executable machine code. This process involves multiple stages, which can be broadly categorized into compile-time and runtime operations.
The following diagram illustrates this flow:
Compile-Time
Source Code: This is the original code written by developers in any .NET-supported language, such as C#, VB.NET, or F#. This code is human-readable and contains the logic and instructions for the application.
Code-Specific Compiler: The source code is passed through a language-specific compiler. This compiler translates the high-level language code into an intermediate language (IL). For .NET languages, this intermediate language is called Microsoft Intermediate Language (MSIL).
Intermediate Language Code: The output of the code-specific compiler is the IL code, which is platform-independent. This intermediate code is designed to be executed within the CLR environment, ensuring cross-platform compatibility.
Runtime
JIT Compiler: At runtime, the CLR invokes the Just-In-Time (JIT) compiler to convert the IL code into native machine code. The JIT compiler translates the IL code into platform-specific instructions that the CPU can execute. This conversion happens on-the-fly, meaning the JIT compiler only translates the portions of the code that are required during execution, which helps optimize performance.
Machine Code: The final output of the JIT compilation process is native machine code. This code is specific to the architecture and operating system of the host machine. It can be executed directly by the CPU, allowing the application to run.
Summary of the Compilation and Execution Process
Compile-Time: The source code is translated into intermediate language code by a language-specific compiler. This IL code is platform-independent and ready to be executed within the CLR.
Runtime: The CLR uses the JIT compiler to convert the IL code into native machine code, which the CPU executes.
By understanding this flow, developers can better appreciate how .NET manages the execution of applications, ensuring they are optimized for performance, compatible across platforms, and secure.
Understanding .NET CLR
The .NET Common Language Runtime (CLR) is a fundamental part of the .NET framework, providing an environment for executing .NET programs. It handles various crucial tasks such as memory management, security, and exception handling, making it essential for developers working within the .NET ecosystem.
Core Components of .NET CLR
The CLR is composed of several key components that work together to manage the execution of .NET applications. The following diagram illustrates the relationships between these components:
Intermediate Language Code (MSIL): .NET languages such as C# and VB.NET are compiled into an intermediate language known as MSIL. This intermediate code is platform-independent, allowing .NET applications to run on any system that has the CLR installed.
Common Type System (CTS): CTS standardizes data types across all .NET languages, ensuring that objects written in different languages can interact seamlessly. This uniform type system simplifies interoperability and reduces the risk of type-related errors.
Common Language Specification (CLS): CLS defines a subset of common standards and conventions that all .NET languages must follow. This ensures that code written in one .NET language can be used by other .NET languages, promoting language interoperability.
Just-In-Time Compiler (JIT): The JIT compiler converts MSIL into native machine code that can be executed by the host CPU. This process occurs at runtime, ensuring that the most optimized code is generated based on the specific environment in which the application is running.
Garbage Collector: The garbage collector manages the allocation and release of memory for applications. It automatically frees up memory occupied by objects that are no longer in use, reducing the likelihood of memory leaks and improving application performance.
The Garbage Collection Process
The garbage collection (GC) process in .NET CLR is crucial for managing memory efficiently. It follows a generational approach, dividing objects into three generations based on their lifespan:
Marking Phase: During this phase, the GC identifies which objects are still in use and marks them accordingly. This is done by traversing the object graph, starting from the root objects, and marking all reachable objects.
Sweeping Phase: In this phase, the GC removes the unmarked objects, freeing up the memory they occupied. This phase helps in reclaiming memory that is no longer needed by the application.
Compacting Phase: The GC then compacts the remaining objects to reduce fragmentation. This phase involves moving the objects to a contiguous memory space, which optimizes memory usage and improves access times.
Generational Management:
Generation 0: This is the youngest generation, containing objects with the shortest lifespan. The GC frequently collects these objects as they are usually temporary and quickly become unreachable.
Generation 1: Objects that survive the Generation 0 collection are moved to Generation 1. These objects have a medium lifespan and are collected less frequently.
Generation 2: This generation contains long-lived objects that have survived multiple collections. The GC collects these objects the least frequently, as they are assumed to be in use for a more extended period.
Conclusion
The .NET CLR plays a pivotal role in the execution and management of .NET applications. By converting code into a platform-independent intermediate language, providing a uniform type system, and enforcing common language specifications, the CLR ensures seamless interoperability and efficient execution of .NET programs. Additionally, the garbage collector's generational approach optimizes memory management, further enhancing application performance. Understanding these core components and processes is essential for developers looking to leverage the full potential of the .NET framework.
Subscribe to my newsletter
Read articles from Chahak Jadon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
