Understanding Java ArrayList for DSA
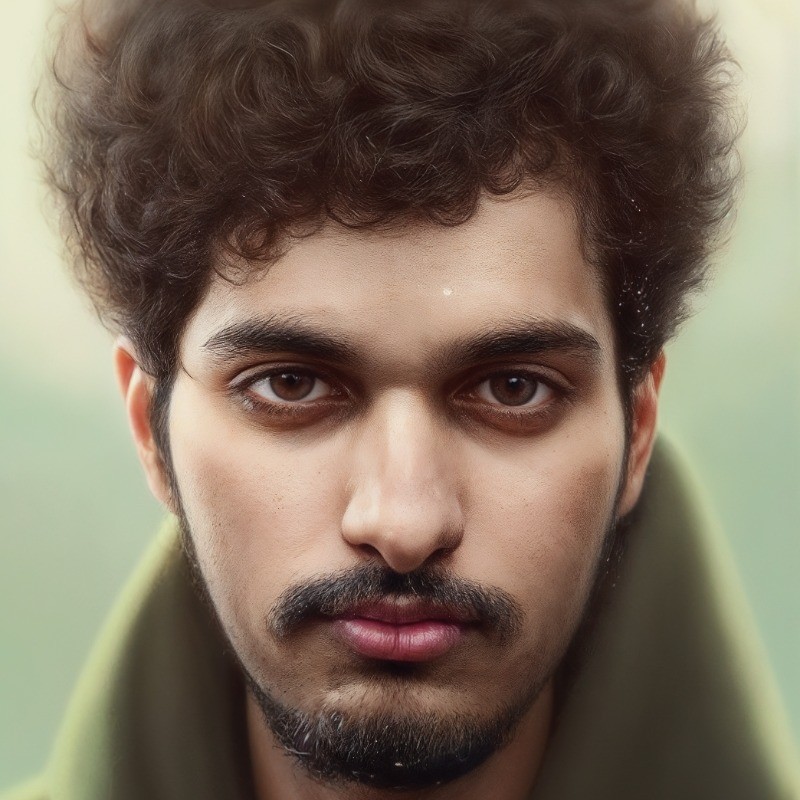
ArrayList
is a fundamental part of the Java Collections Framework, providing a dynamic array implementation of the List
interface. It allows for storing and manipulating a collection of elements, with the ability to grow and shrink dynamically as needed.
Key Characteristics
Dynamic Array: Unlike arrays,
ArrayList
can dynamically increase or decrease in size as elements are added or removed.Ordered Collection: Maintains the insertion order of elements.
Null Elements: Allows the storage of null values.
Duplicates: Allows duplicate elements.
Not Synchronized: By default,
ArrayList
is not thread-safe. For concurrent access, useCollections.synchronizedList
orCopyOnWriteArrayList
.Random Access: Supports fast random access to elements, making it efficient for retrieval operations.
Constructors
ArrayList(): Constructs an empty list with an initial capacity of ten.
ArrayList<String> list = new ArrayList<>();
ArrayList(Collection<? extends E> c): Constructs a list containing the elements of the specified collection in the order they are returned by the collection's iterator.
List<String> anotherList = Arrays.asList("A", "B", "C"); ArrayList<String> list = new ArrayList<>(anotherList);
ArrayList(int initialCapacity): Constructs an empty list with the specified initial capacity.
ArrayList<String> list = new ArrayList<>(20);
Common Methods
add(E e): Appends the specified element to the end of this list.
list.add("Apple");
add(int index, E element): Inserts the specified element at the specified position in this list.
list.add(1, "Banana");
get(int index): Returns the element at the specified position in this list.
String fruit = list.get(0);
remove(int index): Removes the element at the specified position in this list.
list.remove(1);
remove(Object o): Removes the first occurrence of the specified element from this list if it is present.
list.remove("Apple");
contains(Object o): Returns true if this list contains the specified element.
boolean hasApple = list.contains("Apple");
size(): Returns the number of elements in this list.
int size = list.size();
isEmpty(): Returns true if this list contains no elements.
boolean isEmpty = list.isEmpty();
clear(): Removes all of the elements from this list.
list.clear();
set(int index, E element): Replaces the element with the specified element at the specified position in this list.
list.set(0, "Cherry");
Iteration
ArrayList
can be iterated using various methods such as for-each loop, iterators, and streams.
for (String item : list) {
System.out.println(item);
}
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
list.forEach(System.out::println);
Performance Considerations
Random Access: O(1)
Insertion/Deletion at the end: O(1) amortized
Insertion/Deletion at a specific index: O(n)
Search: O(n)
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
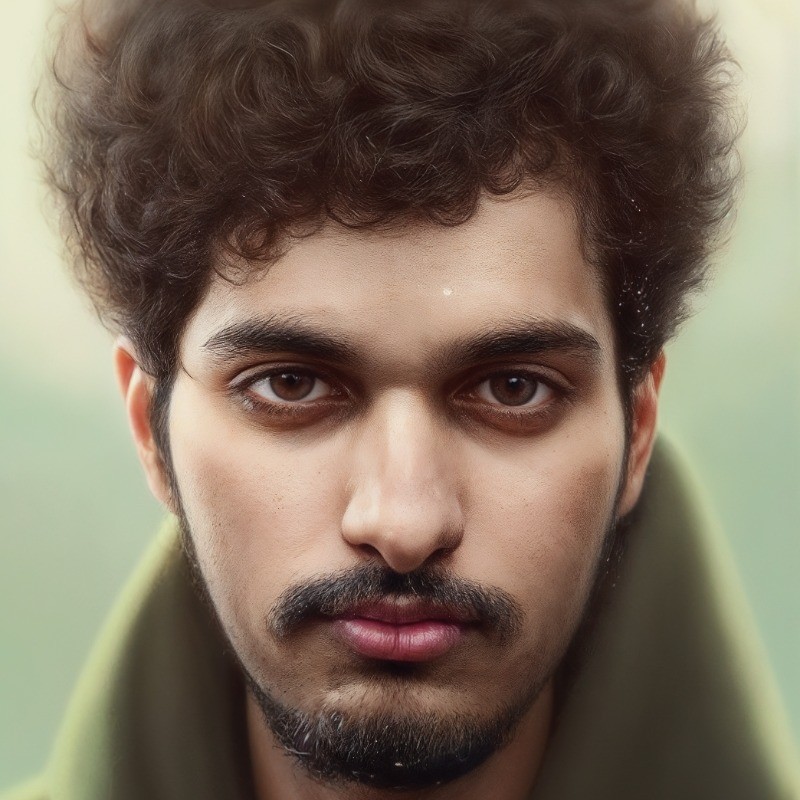
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?