Understanding YAML: A Beginner's Guide

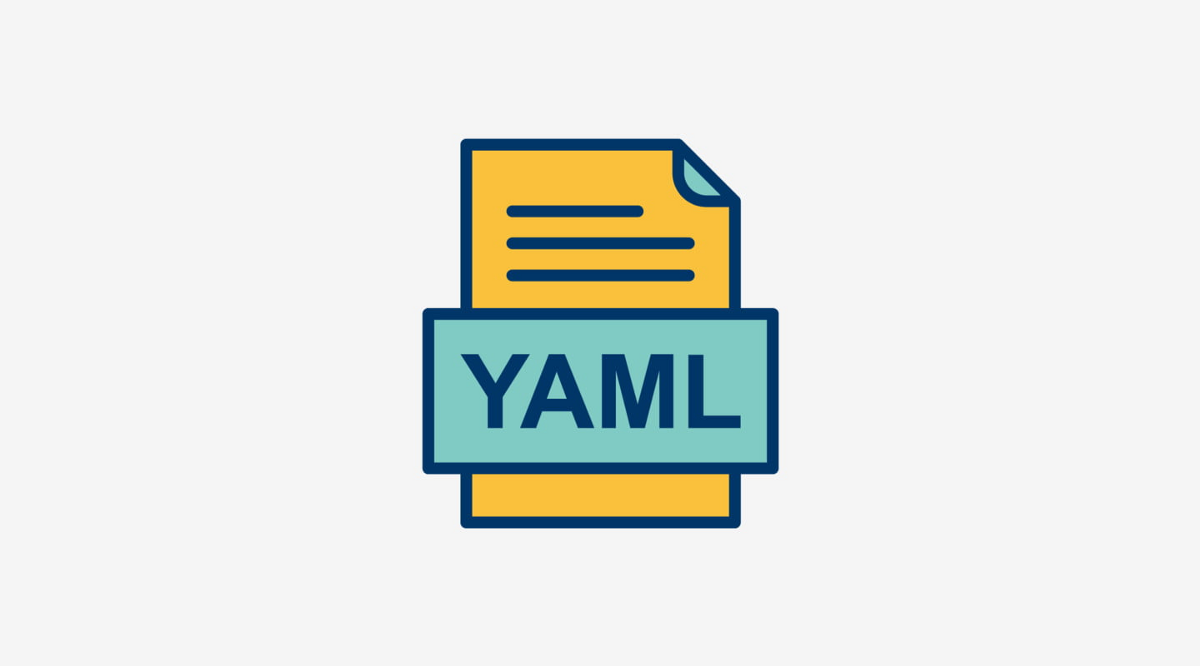
YAML, which stands for YAML Ain't Markup Language, is a human readable data serialization language which is often use for writing configurations file. It can also be used in conjunction with any programming language. Due to YAML’s syntax, it’s considered easier to read. It is a superset of JSON, another data serialization language, which means that a YAML file can contain JSON objects.
YAML Syntax
YAML file has a .yml or .yaml extensions and some specific syntax.
Unlike JSON and XML, it doesn't have any format symbols like quotation marks, square brackets, curly brackets and braces, instead they use indentation to define the structure and indicate nesting. It uses simple key-value pairs to represent data structures. Comments are identifies using hash symbol(#). It is advised to use comments as they define the intention of the code and improve the code understandability. As of now, multiline comments are not supported by YAML. You need to add hash symbol at each line of comment.
Due to non dependency on braces for its structures, availability of comments and improved human readability, YAML is a viable replacement for JSON.
How YAML store data ?
YAML can store different type of data, including scalar(Boolean, string , integer), sequence(list) and mapping.
Scalars
Scalars are simple values like strings and numbers separated by a colon(:). You can provide the value in plain text, single quotation or double quotation.
# three dash use to signal start of a file
---
string_value: your_name
integer_value: 42
double_quotation: "your_name"
Sequence(list)
Lists include values listed in specific order. A list item starts with dash(-) and space and indentation separates it from its parent.
---
Hobbies:
- Singing
- Dancing
- Reading
Mapping
Mapping is the collection of key and values separated by colon (:) and space. Values can be scalar, mapping and sequence.
Student:
Name: Joey Green
Age: 23
City: New York
Is_student: true
Subjects:
- Maths
- Chemistry
- Hindi
Parsing YAML file
You need to install yaml-cpp. You can either install it using package manager or build it from source. Here is a simple YAML file, let's see how can we parse it using yaml old api.
#A yaml file student.yaml
---
name: Robert Cummin
age: 20
is_student: true
skills:
- C++
- Python
- YAML
- Java
The file need to have .yml or .yaml extensions. This file has a map structure and the values have integers, string , Boolean and and a list. To parse the file using C++, you need to include yaml header file so to access yaml pre defined function for parsing.
#include <iostream>
#include <fstream>
#include <yaml-cpp/yaml.h>
int main() {
// Open the YAML file
std::ifstream fin("student.yaml");
// Create a parser object
YAML::Parser parser(fin);
// Create a root node
YAML::Node rootNode;
parser.GetNextDocument(rootNode);
// Accessing scalar values
std::string name;
int age;
bool is_student;
//The >> operator is used to extract values from the YAML::Node into C++ variables.
rootNode["name"] >> name;
rootNode["age"] >> age;
rootNode["is_student"] >> is_student;
// Accessing sequence values
const YAML::Node& skills = rootNode["skills"];
for (unsigned i = 0; i < skills.size(); i++) {
std::string skill;
skills[i] >> skill;
std::cout << "Skill " << i + 1 << ": " << skill << std::endl;
}
// Printing the values
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
std::cout << "Is Student: " << (is_student ? "Yes" : "No") << std::endl;
return 0;
}
Conclusion
YAML is a perfect replacement for JSON file for configurations data. Here, we discussed its basics, usage and parsed a yaml file using C++. In another article we will parse a complex file and also write a generic code that can parse any type of yaml file.
Subscribe to my newsletter
Read articles from Varsha Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Varsha Gupta
Varsha Gupta
I passionate Web Developer and a Competetive Programmer who is also a pre-final year in her graduation from Lovely Professional University.