Understanding Java Stack for DSA
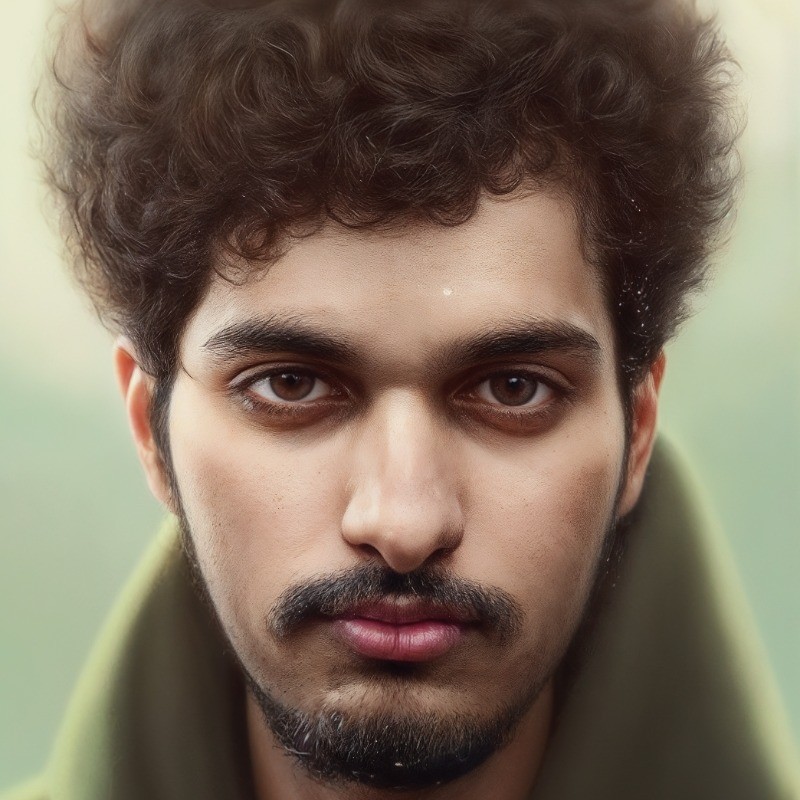
A Stack
in Java is a data structure that follows the Last-In-First-Out (LIFO) principle. This means that the last element added to the stack will be the first one to be removed. The Stack
class in Java extends the Vector
class, which means it inherits all the methods from Vector
but is specifically designed to operate as a stack.
Key Characteristics
LIFO Principle: The last element added to the stack is the first to be removed.
Thread-Safe: Since
Stack
extendsVector
, it is synchronized and thus thread-safe.Null Elements: Allows the storage of null values.
Duplicates: Allows duplicate elements.
Constructor
Stack(): Creates an empty stack.
Stack<String> stack = new Stack<>();
Common Methods
push(E item): Pushes an item onto the top of this stack.
stack.push("Apple");
pop(): Removes the object at the top of this stack and returns that object as the value of this function.
String fruit = stack.pop();
peek(): Looks at the object at the top of this stack without removing it from the stack.
String fruit = stack.peek();
empty(): Tests if this stack is empty.
boolean isEmpty = stack.empty();
search(Object o): Returns the 1-based position where an object is on this stack. If the object
o
occurs as an item in this stack, this method returns the distance from the top of the stack of the occurrence nearest the top of the stack; the topmost item on the stack is considered to be at a distance1
. If the object is not on the stack, it returns-1
.int position = stack.search("Apple");
Iteration
Stack
can be iterated using various methods such as for-each loop, iterators, and streams.
for (String item : stack) {
System.out.println(item);
}
Iterator<String> iterator = stack.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
stack.forEach(System.out::println);
Performance Considerations
Push/Pop/Peek: O(1)
Search: O(n)
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
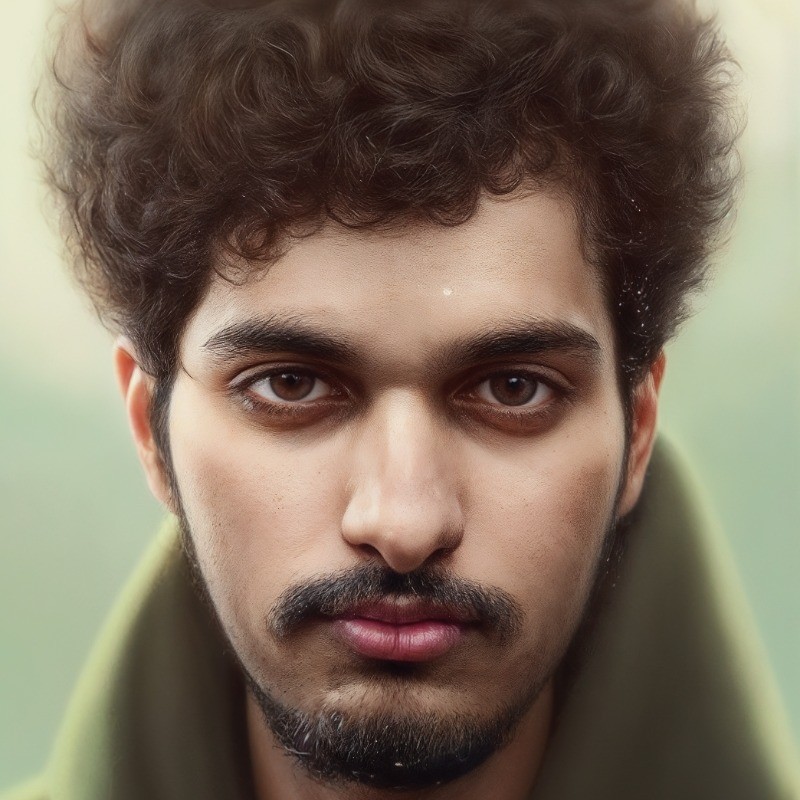
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?