Understanding Node.js Architecture

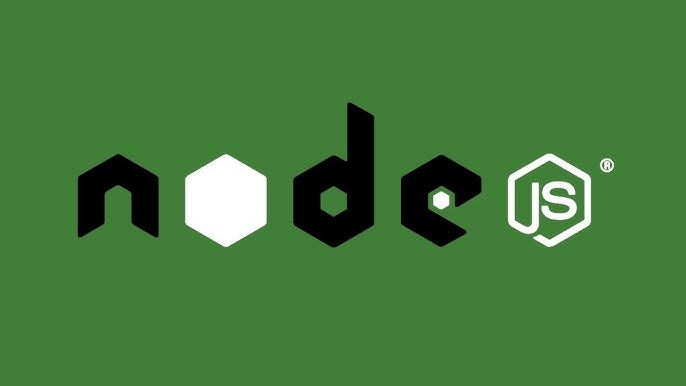
Node.js has revolutionized the way we build server-side applications by allowing developers to use JavaScript on the server. Its architecture is unique and highly efficient, making it a popular choice for building scalable network applications. In this blog, we will dive deep into the architecture of Node.js, breaking down its core components, and understanding how they work together with practical examples.
1. Introduction to Node.js
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside of a web browser. It was created by Ryan Dahl in 2009, and its primary purpose is to build scalable network applications.
2. Key Features of Node.js
Asynchronous and Event-Driven: Node.js uses an event-driven, non-blocking I/O model, making it efficient and suitable for data-intensive applications.
Single-Threaded: Despite being single-threaded, Node.js can handle a large number of connections concurrently thanks to its event loop and non-blocking I/O.
Fast Execution: Built on Google Chrome's V8 JavaScript engine, Node.js executes code quickly.
Rich Ecosystem: The Node Package Manager (NPM) provides access to thousands of reusable libraries and modules.
3. Core Components of Node.js Architecture
Event-Driven Architecture
Node.js follows an event-driven architecture where an event-driven design is utilized to handle asynchronous operations. Events are emitted, and listeners are triggered to process these events. This pattern is highly efficient for I/O-bound applications.
Single-Threaded Model
Node.js operates on a single-threaded event loop. This means it can handle multiple concurrent operations without creating multiple threads. Instead, it relies on asynchronous operations and callbacks.
Non-Blocking I/O
Non-blocking I/O means that operations like reading from the file system, network, or database do not block the execution of the program. Instead, Node.js will continue to execute other tasks while waiting for these operations to complete.
The Event Loop
The event loop is the heart of Node.js. It continuously checks the event queue and executes callback functions as events are processed. Here's a simplified view of how it works:
Timers: Executes callbacks scheduled by
setTimeout
andsetInterval
.Pending Callbacks: Executes I/O callbacks deferred to the next loop iteration.
Idle, Prepare: Only used internally.
Poll: Retrieves new I/O events; executes I/O-related callbacks.
Check: Executes callbacks scheduled by
setImmediate
.Close Callbacks: Executes close event callbacks like
socket.on('close')
.
4. Detailed Examples
Building a Simple Server
Let's build a simple HTTP server to understand how Node.js works in practice.
const http = require('http');
// Create a server object
const server = http.createServer((req, res) => {
// Write response
res.write('Hello, World!');
res.end();
});
// The server listens on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this example:
We import the
http
module.We create a server that responds with "Hello, World!" to any request.
We make the server listen on port 3000.
Handling Asynchronous Operations
To demonstrate non-blocking I/O and the event loop, let's read a file asynchronously.
const fs = require('fs');
console.log('Before reading file');
// Read file asynchronously
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
console.log('After reading file');
OUTPUT
Before reading file
After reading file
Content of example.txt
In this example:
We import the
fs
module.We use
fs.readFile
to read the fileexample.txt
asynchronously.The
console.log('After reading file')
statement executes immediately after initiating the read operation, demonstrating non-blocking behavior.
Subscribe to my newsletter
Read articles from Vishwajit Vm directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vishwajit Vm
Vishwajit Vm
Hey, my name is Vishwajit, and I’m from New Delhi. I specialize in backend development and AI, working with technologies like Python, Node.js, Express.js, GraphQL, and vector databases to build intelligent, scalable solutions. Alongside my backend and AI expertise, I also work with PHP, Laravel, MySQL, MongoDB, React, Next.js, HTML, CSS, and more. I enjoy combining robust technical architecture with creative design, offering clients sophisticated solutions that are also cost-effective. I believe in continuously learning new tools and strategies to stay ahead of trends and deliver exceptional results. Through dedication and hard work, I’m focused on growing as a skilled software engineer and designer. With my passion for technology and design, I’m confident I can help you bring your creative vision to life. Let’s collaborate and create something truly extraordinary!