React Context API
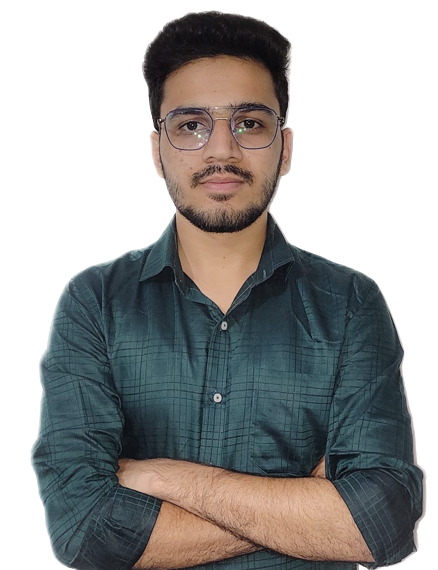
The Challenge of Sharing Data in React
In React, passing data from one component to another is a common task. Traditionally, this has been done using props, where a parent component passes data down to its children. However, as your application grows, managing and passing data becomes more complex. This blog will walk you through the problems of traditional prop passing, introduce you to the Context API, and show you how it elegantly solves these problems.
As you can see, if child2
need any prop, must be passed down through multiple components, even if some intermediate components don’t need it. This makes your code hard to manage and maintain. This problem is known as prop drilling.
Prop drilling can make your code more difficult to read and maintain, and can also make it harder to refactor your components later on.
The Context API to the Rescue
The Context API in React provides a way to share values between components without having to pass props through every level of the tree. This can be particularly useful for global data like the logged-in user, theme settings, or language preferences.
The Context API lets you store data at a higher level in the component tree, so any component that needs it can access it directly without passing props through many layers. This approach simplifies state management and makes your code easier to maintain.
Context API Helps Maintain Logged-in User Data
In a React application, managing the state of a logged-in user can be complex, especially when multiple components need access to the user's data. The Context API provides a powerful solution to this problem by offering a way to share data across the entire component tree without the need for prop drilling. Here's how the Context API helps maintain the logged-in user data:
Centralized State Management
When a user logs in, their data (such as username, email, and authentication token) needs to be stored and made accessible to various components throughout the application. By using the Context API, you can create a centralized state that holds the user's data. This centralization simplifies the management of user data, ensuring that all components access the same, consistent state.
How to Get Started with the Context
Create Context Object
First, you create a context object using the
createContext
function from the 'react' library. This context object will hold the user's data. This context acts as a global store that any component can access.Create a new file named
userContext
in thesrc
folder and add the following code to create a context object:import React, { createContext } from 'react'; export const UserContext = createContext(null);
In the above code, we're importing
createContext
from React and using it to create a new context object named "userContext". Then, we are exporting the context object so that we can use it in other parts of our application.Creating the Provider Component
Next, you create a provider component that will wrap your entire application (or parts of it where the user data is needed). This provider will use React's state to manage the user's data and make it available to any component that consumes the context
export const UserProvider = ({ children }) => { const [user, setUser] = useState(null); const loginUser = (userData) => { setUser(userData); }; return ( <UserContext.Provider value={{ user, loginUser }}> {children} </UserContext.Provider> ); };
Wrapping the Application with the Provider:
Wrap your main application component with the
UserProvider
. This ensures that the user context is available throughout your app.import React from 'react'; import ReactDOM from 'react-dom'; import App from './App'; import { UserProvider } from './UserContext'; ReactDOM.render( <UserProvider> <App /> </UserProvider>, document.getElementById('root') );
Consume the Context
Now that we've created the provider component, we need to consume the context in other components. To do this, we use the "useContext" hook from React.
Any component that needs access to the user's data can now consume the context using the
useContext
hook. This allows components to access and display user information without prop drilling.import React, { useContext } from 'react'; import { UserContext } from './UserContext'; const NavBar = () => { const { user } = useContext(UserContext); return ( <nav> {user ? <p>Welcome, {user.name}</p> : <p>Please log in</p>} </nav> ); };
Benefits of Using the Context API for User Data
Avoids Prop Drilling:
- With the Context API, there's no need to pass user data through multiple levels of components. Any component can directly access the user data from the context.
Simplifies State Management:
- By centralizing user data in a context provider, you can easily manage and update the state in one place. This makes the codebase cleaner and more maintainable.
Ensures Consistent Data:
- All components accessing the context will get the same, up-to-date user information. This consistency reduces the risk of data discrepancies.
Eases Refactoring:
- When you need to refactor your components, you don’t have to adjust the prop chain. Components can be moved or restructured without worrying about how they access the user data.
Conclusion
The Context API in React is a powerful tool for managing global state, such as logged-in user data. It simplifies sharing state across multiple components, avoids the issues of prop drilling, and improves the maintainability and scalability of your application. By centralizing user data in a context, you ensure that all components have consistent access to the information they need, making your React application more robust and easier to manage.
Subscribe to my newsletter
Read articles from Sumit Sonawane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
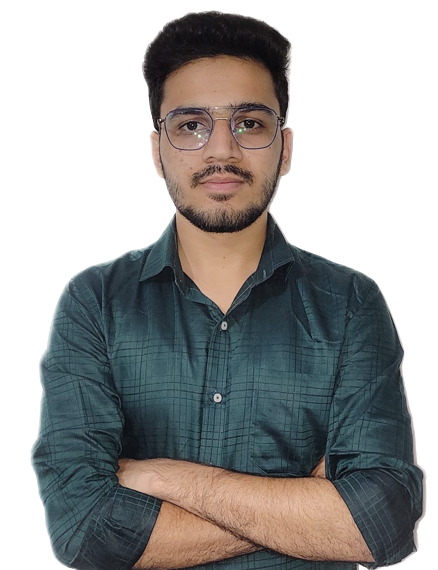