Android : Managing WebView Callbacks with BuildConfig Securely.
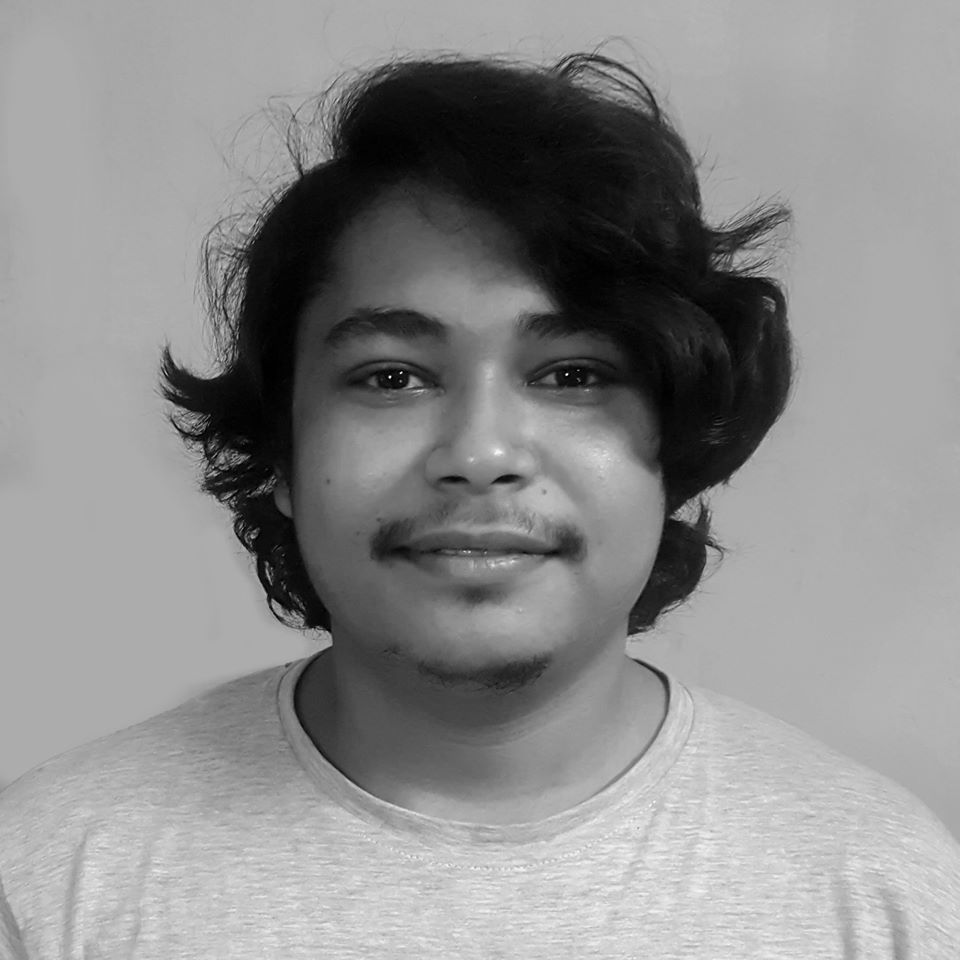
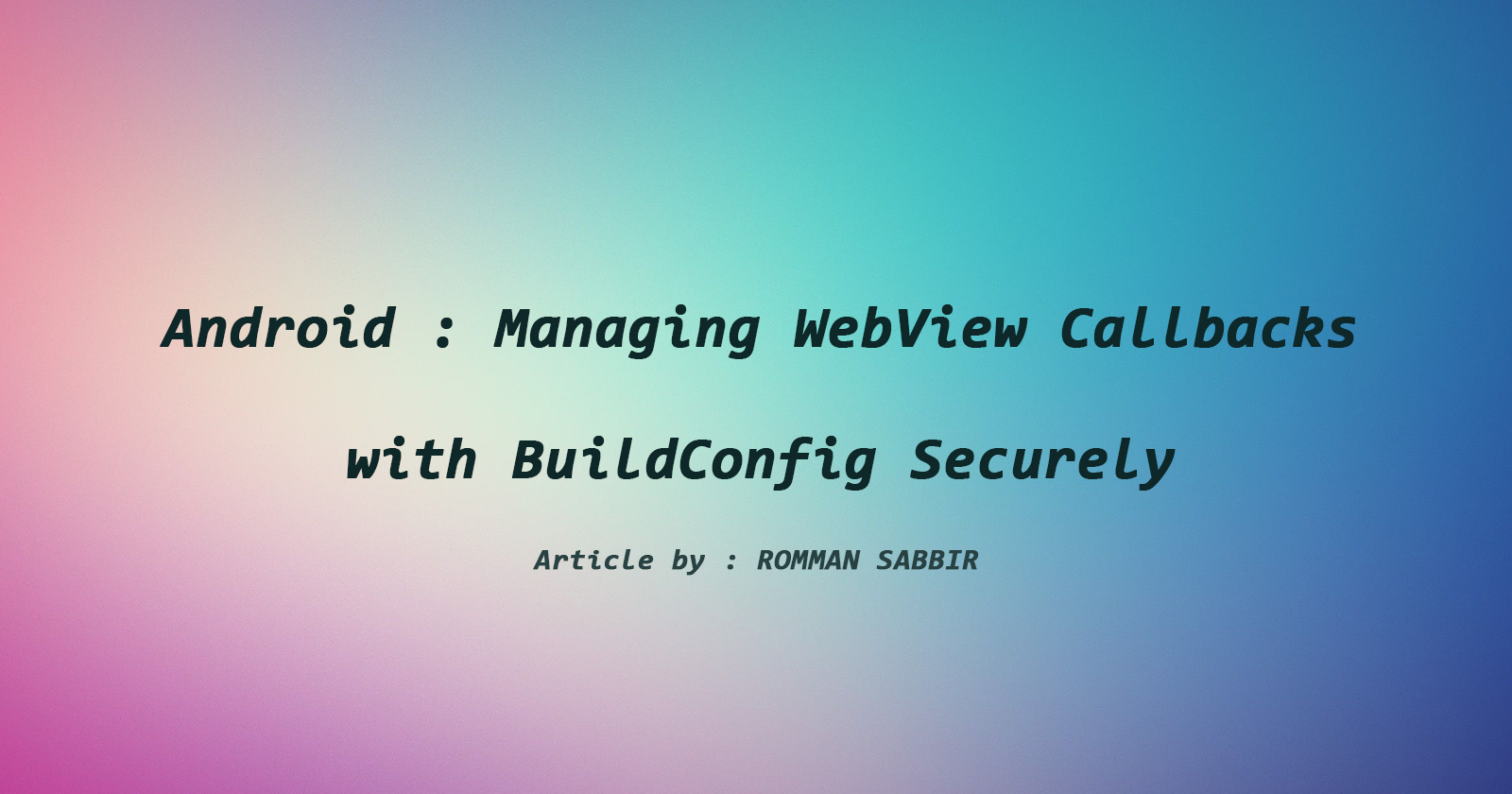
In Android development, buildConfigField
is a powerful tool for defining constants at build time. It allows developers to embed configuration values directly into the generated BuildConfig
class, making them accessible throughout the application code. One common scenario where buildConfigField
is invaluable is in managing WebView
callbacks, especially for tasks like payment verification, order processing, or any other critical operations involving web interactions.
Scenario: Managing WebView Callbacks
WebView
is often used in Android apps to show web content, like payment forms, order confirmations, or any interaction needing secure verification. Handling callbacks from WebView
means making sure the callback URL
s are trusted and processed securely within the app.
Understanding buildConfigField
Before we look at practical examples, let's understand how buildConfigField
works:
Definition:
buildConfigField
is set up in ourbuild.gradle
file inside theandroid
block. It lets us define constants of different types (String
,boolean
,int
, etc.) that are added to your app'sBuildConfig
class during the build process.Usage: Constants defined with
buildConfigField
can be accessed through theBuildConfig
class in our Kotlin or Java code. They allow us to customize our app based on build flavors, build types, or specific settings.
Step-by-Step Guide
Let’s go through how to use buildConfigField
to manage callback URLs securely in our Android app:
1. Define Callback URLs inbuild.gradle
:
First, define the callback URLs as a String
field in our build.gradle
file. For example:
android {
...
buildTypes {
release {
buildConfigField("String", "PAYMENT_CALLBACK_URL", "\"https://yourdomain.com/payment/callback\"")
buildConfigField("String", "ORDER_CALLBACK_URL", "\"https://yourdomain.com/order/callback\"")
// Define other callback URLs as needed
}
debug {
buildConfigField("String", "PAYMENT_CALLBACK_URL", "\"https://dev.yourdomain.com/payment/callback\"")
buildConfigField("String", "ORDER_CALLBACK_URL", "\"https://dev.yourdomain.com/order/callback\"")
// Define other debug callback URLs as needed
}
}
}
In this example:
PAYMENT_CALLBACK_URL
andORDER_CALLBACK_URL
are set with different values forrelease
anddebug
build types. Change these URLs based on our environment (production, staging, development, etc.).
2. Accessing Callback URLs in Kotlin Code:
Next, access these callback URLs from our Kotlin code, such as in an Activity
or a ViewModel
:
val paymentCallbackUrl = BuildConfig.PAYMENT_CALLBACK_URL
val orderCallbackUrl = BuildConfig.ORDER_CALLBACK_URL
// Access other callback URLs as needed
3.WebView
Integration:
Integrate WebView
in our app to handle loading and navigation events. Make sure that when WebView
loads a URL, we check if it matches any of the allowed callback URLs defined in BuildConfig
.
webView.webViewClient = object : WebViewClient() {
override fun shouldOverrideUrlLoading(view: WebView?, request: WebResourceRequest?): Boolean {
val url = request?.url.toString()
// Check if the URL matches any of the allowed callback URLs
if (url == paymentCallbackUrl) {
// Handle payment confirmation logic
// Example: Update payment status in our app
return true // Consumes the URL, prevents WebView from loading it
} else if (url == orderCallbackUrl) {
// Handle order confirmation logic
// Example: Update order status in our app
return true // Consumes the URL, prevents WebView from loading it
}
// For other URLs, allow WebView to load normally
return false
}
}
4. Enhancing Security and Maintenance:
Security: By using
buildConfigField
to define callback URLs, we manage them in one place and ensure they are included in our app during the build process. This lowers the risk of hardcoding sensitive URLs directly in our code.Maintenance: We can easily update callback URLs for different environments (e.g., production, staging) by changing
build.gradle
without altering your app’s code.
5. Error Handling and Edge Cases:
Implement strong error handling for situations where unexpected URLs are loaded in
WebView
.We have to think about edge cases like network failures, server timeouts, and user interruptions during
WebView
interactions.
Conclusion
Using buildConfigField
in Android apps, especially for managing WebView
callbacks, boosts security, simplifies maintenance, and adds flexibility for different build setups. By setting callback URLs as build configuration fields, developers can securely manage payment verification, order confirmations, and other important tasks involving WebView
.This method makes development easier and improves the reliability and security of WebView
integrations in our Android app.
That's it for today. Happy Coding...
Subscribe to my newsletter
Read articles from Romman Sabbir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
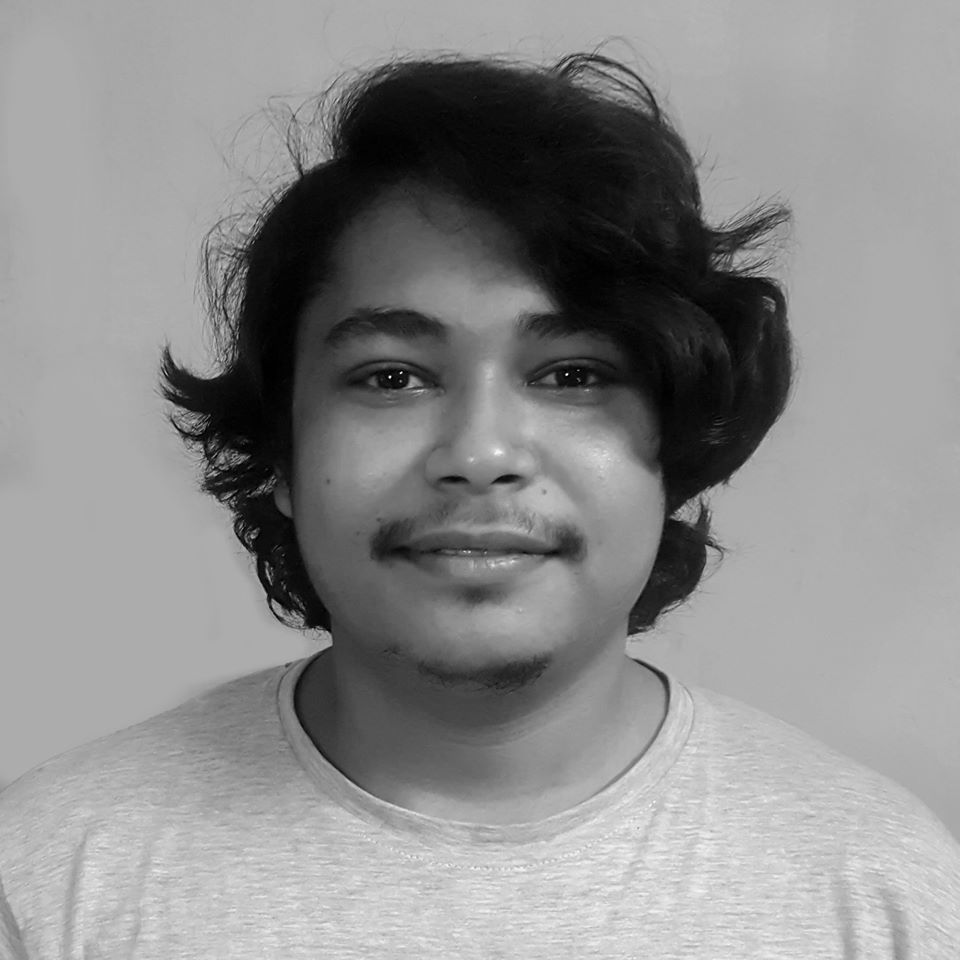
Romman Sabbir
Romman Sabbir
Senior Android Engineer from Bangladesh. Love to contribute in Open-Source. Indie Music Producer.