Understanding Java Queue for DSA
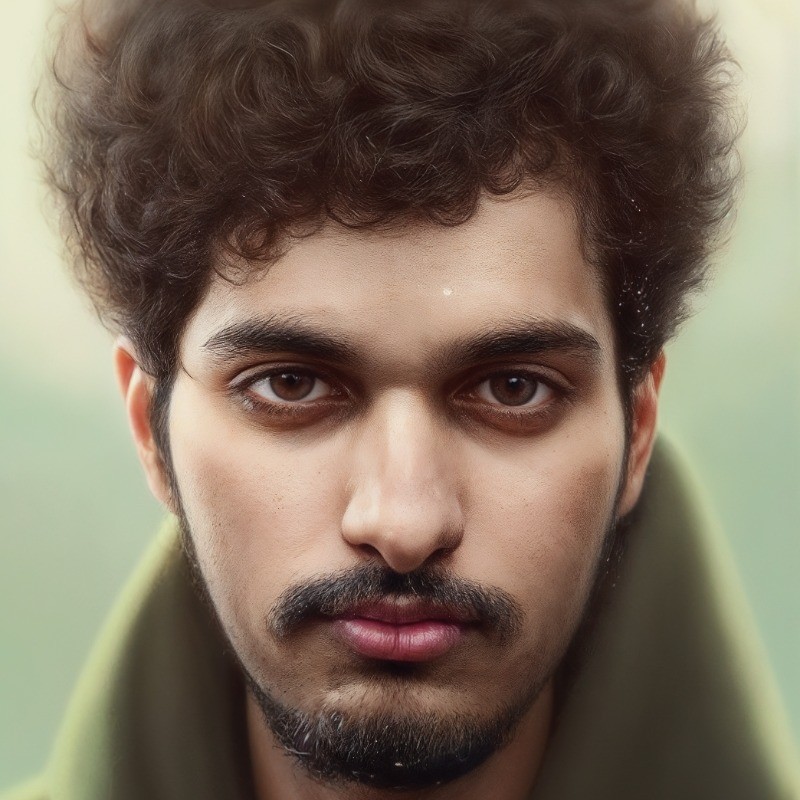
A Queue
in Java is an interface that represents a collection designed for holding elements before processing, following the First-In-First-Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed. The Queue
interface extends the Collection
interface and provides additional methods for adding, removing, and inspecting elements.
Key Characteristics
FIFO Principle: The first element added to the queue is the first one to be removed.
Null Elements: Some implementations allow null elements, but it's generally discouraged as
null
is used as a special return value for some methods.Duplicates: Allows duplicate elements.
Not Synchronized: The
Queue
interface itself does not provide synchronization. Specific implementations likeLinkedList
are not synchronized, while others likeArrayBlockingQueue
are.
Common Methods
add(E e): Inserts the specified element into this queue if it is possible to do so immediately without violating capacity restrictions, returning
true
upon success and throwing anIllegalStateException
if no space is currently available.queue.add("Apple");
offer(E e): Inserts the specified element into this queue if it is possible to do so immediately without violating capacity restrictions. Returns
true
upon success andfalse
if no space is currently available.boolean success = queue.offer("Banana");
remove(): Retrieves and removes the head of this queue. Throws a
NoSuchElementException
if this queue is empty.String fruit = queue.remove();
poll(): Retrieves and removes the head of this queue or returns
null
if this queue is empty.String fruit = queue.poll();
element(): Retrieves, but does not remove, the head of this queue. Throws a
NoSuchElementException
if this queue is empty.String fruit = queue.element();
peek(): Retrieves, but does not remove, the head of this queue, or returns
null
if this queue is empty.String fruit = queue.peek();
Implementations
LinkedList: A doubly-linked list implementation of the
Queue
interface.Queue<String> queue = new LinkedList<>();
PriorityQueue: A
PriorityQueue
is a special type of data structure where each element has a priority assigned to it. When you want to retrieve an element from the queue, you get the one with the highest (or sometimes lowest) priority, not necessarily the one added first. In a priority queue, the retrieval order is based on the priority of the elements, not their order of arrival.Queue<String> queue = new PriorityQueue<>();
ArrayBlockingQueue: An
ArrayBlockingQueue
is a type of queue backed by an array, meaning it has a fixed capacity set when the queue is created. This queue is useful when controlling the number of elements that can be stored, such as in producer-consumer problems.Queue<String> queue = new ArrayBlockingQueue<>(10);
LinkedBlockingQueue: A
LinkedBlockingQueue
is a type of queue that can grow and shrink as needed. Unlike theArrayBlockingQueue
, which has a fixed size, aLinkedBlockingQueue
does not have a pre-defined capacity limit unless specified. It also handles multiple tasks, trying to add or remove items simultaneously, making it useful in multi-threaded applications.Queue<String> queue = new LinkedBlockingQueue<>();
Iteration
Queue
can be iterated using various methods such as for-each loop, iterators, and streams.
for (String item : queue) {
System.out.println(item);
}
Iterator<String> iterator = queue.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
queue.forEach(System.out::println);
Performance Considerations
LinkedList: Adding/removing elements at the head or tail is O(1).
PriorityQueue: Insertion and removal operations are O(log n).
ArrayBlockingQueue/LinkedBlockingQueue: Insertion and removal operations are O(1) under normal conditions.
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
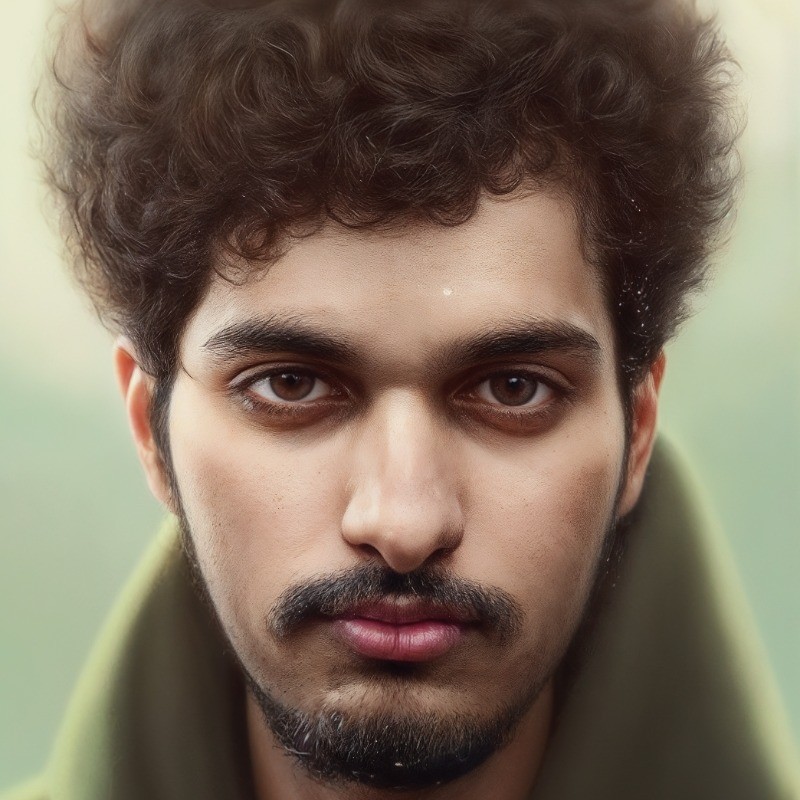
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?