Python Learning Day 5: Mastering Loops, Conditions, and Password Generation
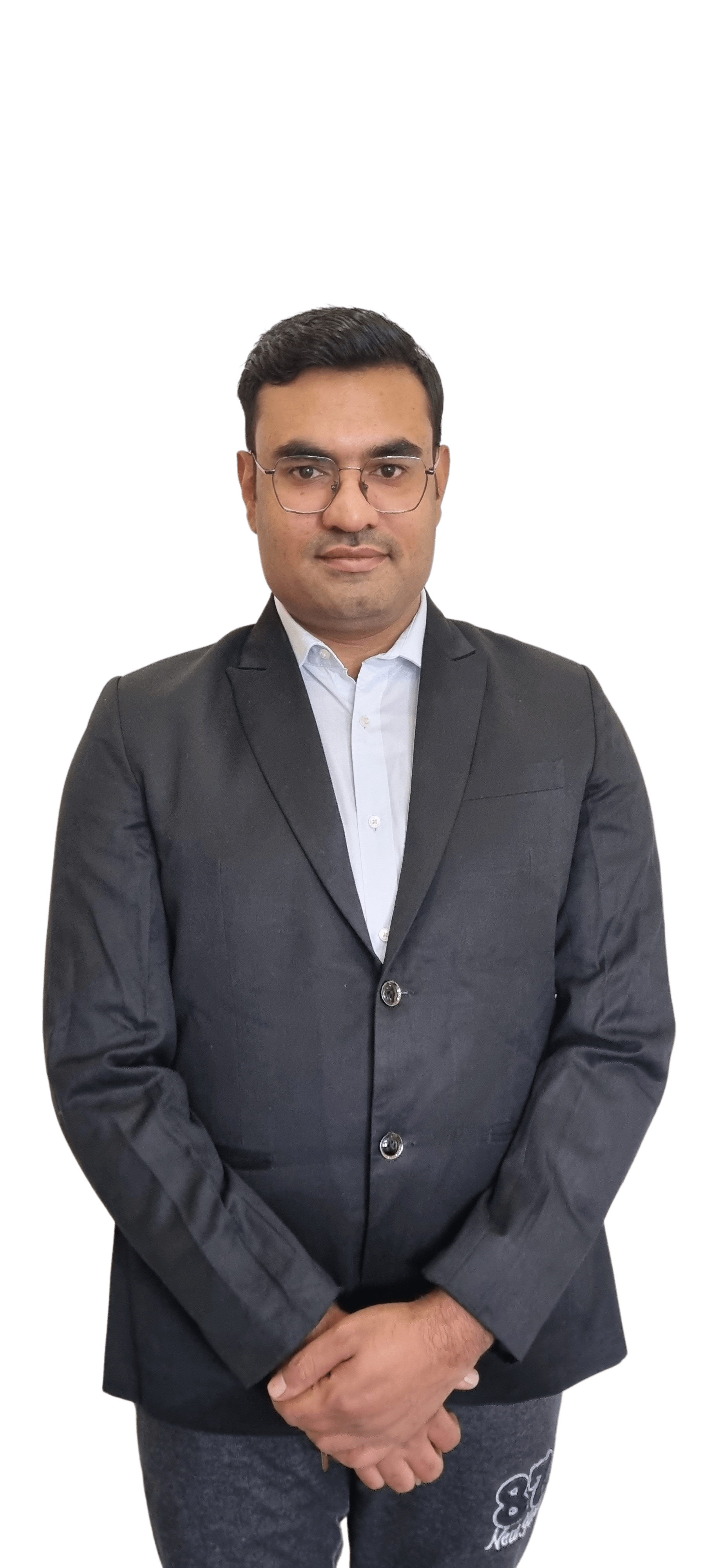
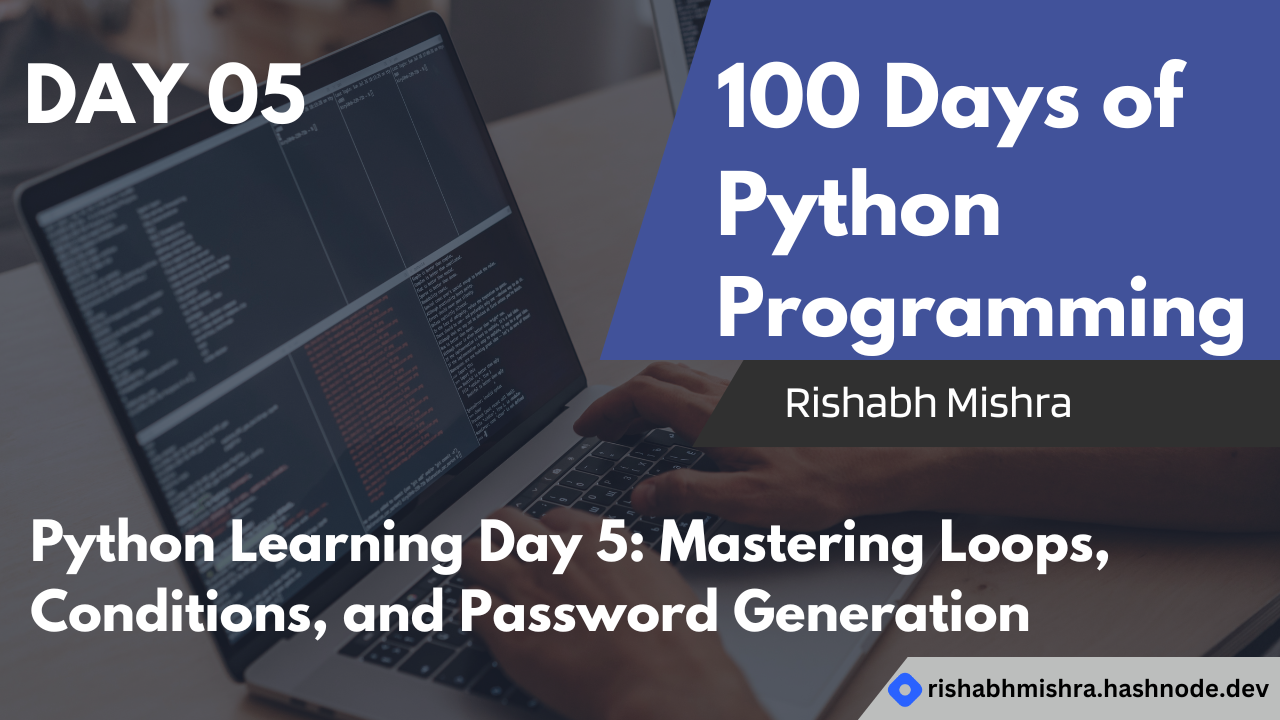
1. Calculating the Sum of Even Numbers
Concept:
This program calculates the sum of all even numbers from 1 to a given number, X. It uses a loop and the range()
function to iterate through even numbers up to X.
Code Explanation:
sum = 0
n = int(input("Enter the number till which the number needs to be added: "))
for num in range(0, n + 2, 2):
sum += num
print(f"The sum of all even numbers between 0 and {n} is {sum}")
Initialization:
sum
is initialized to 0 to store the cumulative sum of even numbers.Input:
n
is taken as user input to specify the upper limit till which even numbers are considered.Loop:
for num in range(0, n + 2, 2)
iterates over even numbers from 0 ton
(inclusive) with a step of 2.Sum Calculation: Inside the loop, each even number
num
is added tosum
.Output: Finally, it prints the total sum of even numbers up to
n
.
2. Calculating Average Student Height
Concept:
This program calculates the average height of students from a list of heights without using built-in functions like sum()
or len()
.
Code Explanation:
sum = 0
num = 0
student_height = [151, 145, 179]
for student in student_height:
sum += student
num += 1
average_height = round(sum / num)
print(f"The total height of students is {sum}")
print(f"The total number of students is {num}")
print(f"The average height of students is {average_height}")
Initialization:
sum
starts at 0 to accumulate the total height, andnum
starts at 0 to count the number of students.Loop:
for student in student_height
iterates through each student's height in thestudent_height
list.Sum and Count: Inside the loop, it adds each student's height to
sum
and incrementsnum
.Average Calculation:
average_height
is calculated as the rounded result ofsum / num
.Output: It prints the total height, total number of students, and average height.
3. FizzBuzz Game
Concept:
The FizzBuzz game prints numbers from 1 to 100, replacing multiples of 3 with "Fizz", multiples of 5 with "Buzz", and multiples of both with "FizzBuzz".
Code Explanation:
for num in range(1, 101):
if num % 3 == 0 and num % 5 == 0:
print("FizzBuzz")
elif num % 3 == 0:
print("Fizz")
elif num % 5 == 0:
print("Buzz")
else:
print(num)
image
Loop:
for num in range(1, 101)
iterates from 1 to 100 (inclusive).Conditions:
if num % 3 == 0 and num % 5 == 0:
checks ifnum
is divisible by both 3 and 5.elif num % 3 == 0:
checks ifnum
is divisible by 3.elif num % 5 == 0:
checks ifnum
is divisible by 5.else:
prints the number itself if none of the above conditions are met.
Output: It prints "Fizz", "Buzz", "FizzBuzz", or the number depending on the conditions.
4. Finding the Highest Score
Concept:
This program finds the highest score from a list of student scores without using max()
.
Code Explanation:
High_Score = 0
student_scores = [78, 65, 89, 86, 55, 91, 64, 89]
for score in student_scores:
if score > High_Score:
High_Score = score
print(f"The highest score in the class is: {High_Score}")
Initialization:
High_Score
starts at 0 to track the highest score found.Loop:
for score in student_scores
iterates through each score instudent_scores
.Comparison: Inside the loop, it compares each score with
High_Score
and updatesHigh_Score
if the current score is higher.Output: It prints the highest score found in the list.
5. Password Generator
Concept:
The Password Generator creates a random password with specified numbers of letters, symbols, and numbers.
Code Explanation:
import random
letters = ['a', 'b', ..., 'Z']
numbers = ['0', '1', ..., '9']
symbols = ['!', '#', ..., '+']
nr_letters = int(input("How many letters would you like in your password?\n"))
nr_symbols = int(input("How many symbols would you like?\n"))
nr_numbers = int(input("How many numbers would you like?\n"))
password_list = []
# Adding letters
for char in range(1, nr_letters + 1):
password_list.append(random.choice(letters))
# Adding symbols
for char in range(1, nr_symbols + 1):
password_list.append(random.choice(symbols))
# Adding numbers
for char in range(1, nr_numbers + 1):
password_list.append(random.choice(numbers))
random.shuffle(password_list) # Shuffle the password list
password = ""
for char in password_list:
password += char
print(f"Your password is: {password}")
Imports:
import random
imports therandom
module for generating random elements.Lists:
letters
,numbers
, andsymbols
store possible characters for the password.User Input:
nr_letters
,nr_symbols
, andnr_numbers
are taken as input for the number of letters, symbols, and numbers in the password.Password Generation:
Loops add random characters to
password_list
based on user input.random.shuffle(password_list)
shuffles the elements inpassword_list
.A loop concatenates elements in
password_list
to formpassword
.
Output: It prints the generated password.
These projects have helped me deepen my understanding of Python's foundational concepts. Stay tuned for more updates on my Python learning journey!
Subscribe to my newsletter
Read articles from Rishabh Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
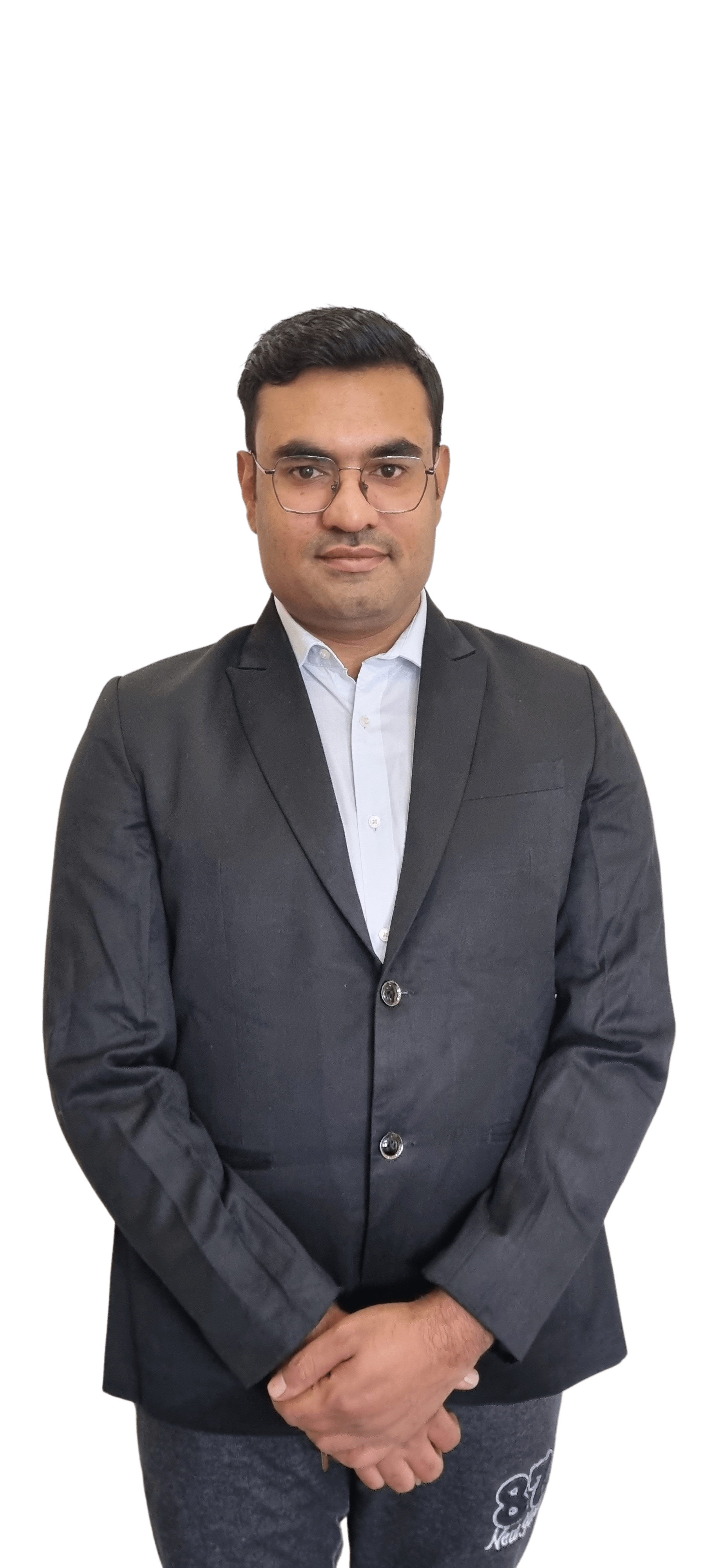
Rishabh Mishra
Rishabh Mishra
I'm Rishabh Mishra, an AWS Cloud and DevOps Engineer with a passion for automation and data analytics. I've honed my skills in AWS services, containerization, CI/CD pipelines, and infrastructure as code. Currently, I'm focused on leveraging my technical expertise to drive innovation and streamline processes. My goal is to share insights, learn from the community, and contribute to impactful projects in the DevOps and cloud domains. Let's connect and collaborate on Hashnode!