[Rust] Data Types part 3

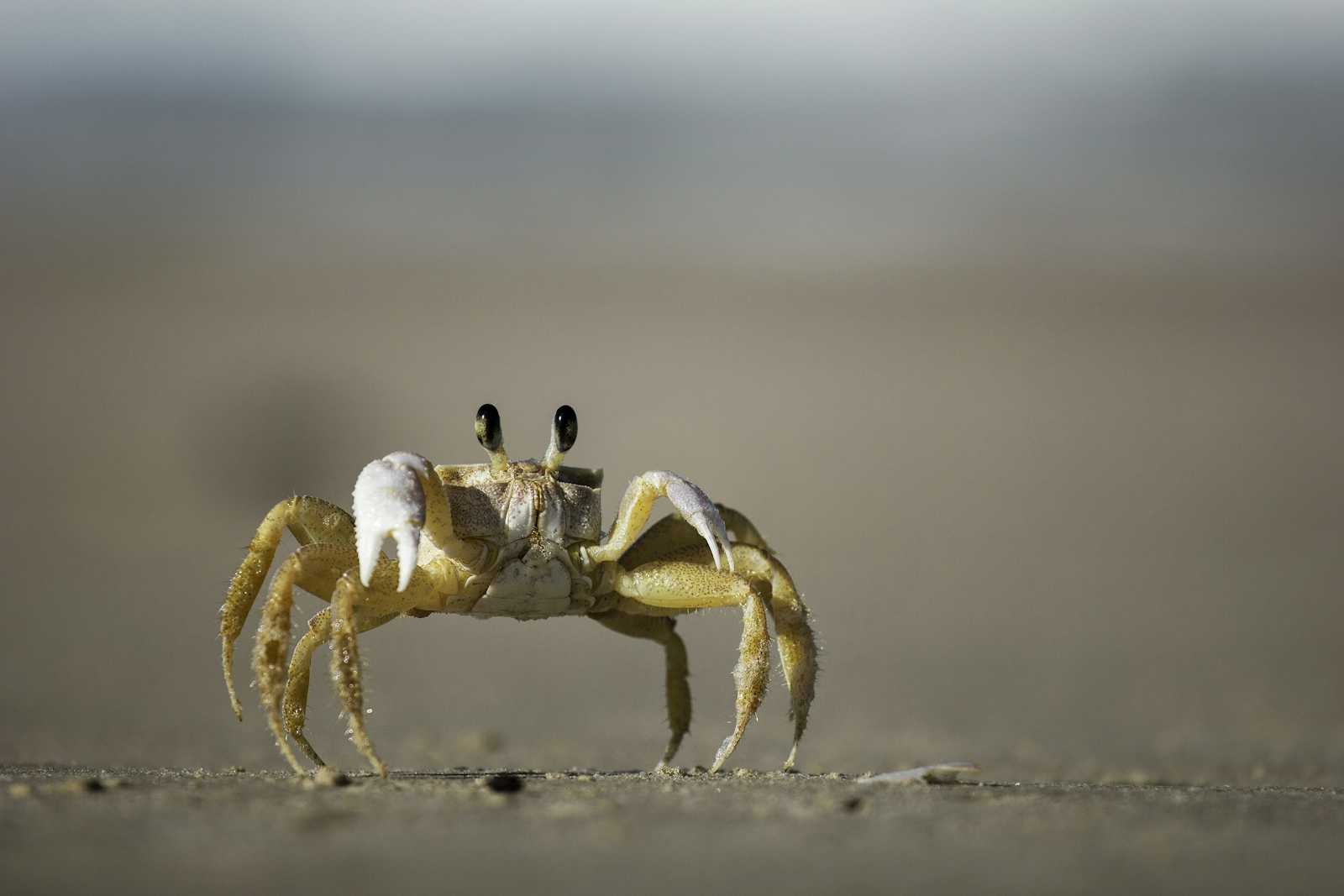
A data type system represents the different types of values supported by a language.
It allows for code hints and automated documentation while validating the values provided before they are stored or manipulated by the program and verifying that the code behaves as planned.
Rust is a statically typed language.
Every value in Rust is a specific data type, and the compiler automatically infers the data type of a variable based on the assigned value.
Declaring variables
Declaring variables with the let keyword
fn main() {
let company_string = "HR&T";
let rating_float = 1.5;
let is_growing_boolean = true;
let icon_char = '♥';
println!("company name is:{}",company_string);
println!("company rating on 5 is:{}",rating_float);
println!("company is growing :{}",is_growing_boolean);
println!("company icon is:{}",icon_char);
}
In the example above, the data type of a variable is inferred from its assigned value.Assign a string data type to the variable company_string and a float data type to rating_float.
println The macro takes two arguments.
{} Placeholder
Variable name or constant name
Scalar Types
The scalar format represents a single value.
Integer
Floating-point
Booleans
Characters
Integer
Integer는 정수를 나타냅니다.
Signed와 Unsigned로 분류합니다.
크기가 시스템 아키텍처에서 파생됩니다.
x86에서는 32bit x64에서는 64bit
for example
fn main() {
let result = 10;
let age:u32 = 20;
let sum:i32 = 5-15;
let mark:isize = 10;
let count:usize = 30;
println!("result value is {}",result);
println!("sum is {} and age is {}",sum,age);
println!("mark is {} and count is {}",mark,count);
}
If you replace the age variable with a real number in the above code, you will get a compilation error.
Integer Range
각 부호 있는 변수는 -(2^(n — 1)에서 2^(n — 1) — 1까지의 숫자를 저장
부호 없는 변수는 0 부터 2^n — 1 까지의 숫자를 저장
여기서 n은 변수가 사용하는 비트 수
i8은 -(2⁷) 부터 (2⁷) — 1 까지 표현
u8은 0 부터 2⁸ — 1 까지 표현
Integer Overflow
An integer overflow occurs when the value assigned to an integer variable exceeds the range defined by Rust for the data type.
fn main() {
let age:u8 = 255;
// 0 to 255 only allowed for u8
let weight:u8 = 256; //overflow value is 0
let height:u8 = 257; //overflow value is 1
let score:u8 = 258; //overflow value is 2
println!("age is {} ",age);
println!("weight is {}",weight);
println!("height is {}",height);
println!("score is {}",score);
}
위 예제의 결과는
age is 255
weight is 0
height is 1
score is 2
로 표현되어 있었으나, 실제 rustc로 컴파일 하면 위 그림처럼 에러 발생합니다.
Float
Rust의 Float 데이터 유형은 f32와 f64로 분류합니다.
f32 유형은 단정밀도 부동 소수점이고 f64 유형은 배정밀도 부동 소수점
기본은 f64
fn main() {
let result = 10.00; //f64 by default
let interest:f32 = 8.35;
let cost:f64 = 15000.600; //double precision
println!("result value is {}",result);
println!("interest is {}",interest);
println!("cost is {}",cost);
}
Automatic Type Casting
Rust doesn't allow automatic type casting.
fn main() {
let interest:f32 = 8; // integer assigned to float variable
println!("interest is {}",interest);
}
Number Separator
To make large numbers easier to read, you can use the visual separator _ underscore to separate numbers.
fn main() {
let float_with_separator = 11_000.555_001;
println!("float value {}",float_with_separator);
let int_with_separator = 50_000;
println!("int value {}",int_with_separator);
}
Boolean
bool 형식은 true 또는 false의 두 가지 가능한 값 사용합니다.
bool 키워드를 사용하여 bool 변수를 선언합니다.
fn main() {
let isfun:bool = true;
println!("Is Rust Programming Fun ? {}",isfun);
}
Character
Rust's character data type supports numbers, alphabets, Unicode, and special characters. Use the char keyword to declare variables of the character data type.
Rust의 char 유형은 유니코드 스칼라 값을 표현합니다.
ASCII 그 이상을 나타낼 수 있습니다.
유니코드 스칼라 값의 범위는 U+0000 ~ U+D7FF 및 U+E000 ~ U+10FFFF 포함합니다.
fn main() {
let special_character = '@'; //default
let alphabet:char = 'A';
let emoji:char = '😁';
println!("special character is {}",special_character);
println!("alphabet is {}",alphabet);
println!("emoji is {}",emoji);
}
Subscribe to my newsletter
Read articles from Harold Lippin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harold Lippin
Harold Lippin
Hansei Cyber Security High Schoool (2022/3/2 ~ 2025/2/10)