State and Props in React

Table of contents
- Introduction
- Understanding State and Props in React
- Fundamentals of State
- Managing State in Class Components
- Managing State in Functional Components
- Advanced State Management
- Lifecycle Methods and State
- Props: The Basics
- Using Props Effectively
- Props in Functional Components
- Props in Class Components
- State and Props Interaction
- Common Patterns and Practices
- Handling Complex State and Props
- Debugging State and Props Issues
- Best Practices and Recommendations
- Conclusion
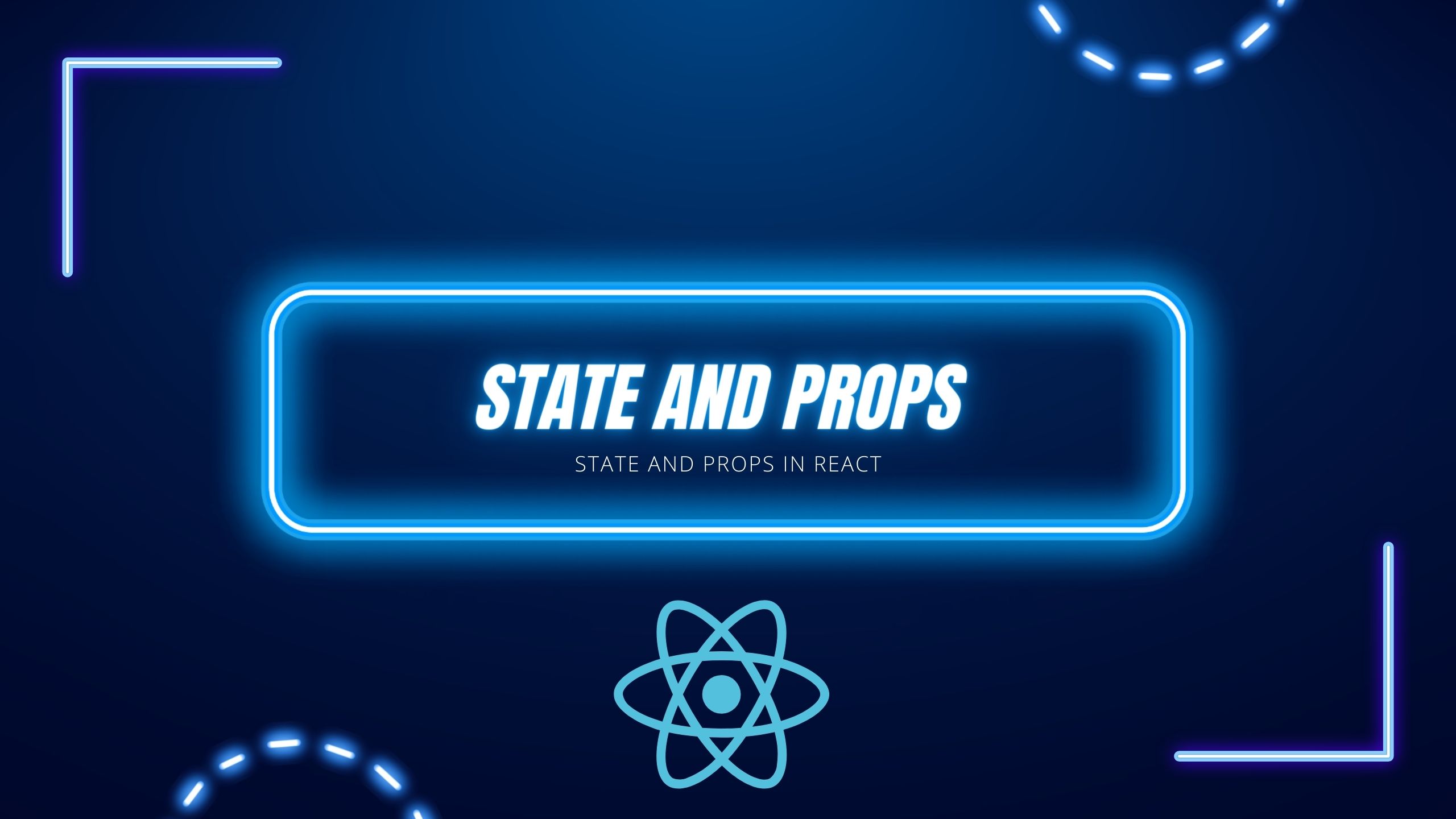
Introduction
React, a robust JavaScript library for building user interfaces, is predicated on two fundamental concepts: state and props. These constructs form the backbone of React's declarative paradigm, enabling developers to create dynamic, responsive, and interactive applications. To harness the full power of React, it's imperative to grasp the nuances of state and props, and understand how they interplay to drive component behavior and render the user interface.
Understanding State and Props in React
State and props are essential elements that govern how React components function and communicate. While state is mutable and encapsulated within a component, allowing it to manage and respond to changes over time, props are immutable inputs passed from parent to child components, facilitating data flow and component interaction.
The Role of State and Props in React Development
State and props serve distinct yet complementary roles in React development. State manages the internal data and influences the component's rendering behavior based on user interactions or other events. Props, on the other hand, enable components to receive data and functions from their parent components, ensuring a unidirectional data flow that promotes predictable and maintainable code.
Fundamentals of State
Defining State in React
State in React refers to a component's local data storage, which determines how the component renders and behaves. It is a dynamic data structure that can change over time, typically in response to user actions or network requests.
How State Differs from Props
While both state and props hold information that influences component rendering, state is managed within the component and can change over its lifecycle. Props are read-only attributes passed to the component, which cannot be modified by the component itself, ensuring a clear separation of concerns and promoting component reusability.
Setting Initial State in Class Components
In class components, initial state is typically set within the constructor using this.state
:
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
}
Setting Initial State in Functional Components with useState
Functional components leverage the useState
hook to manage state, providing a more concise and readable syntax:
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
return <div>{count}</div>;
}
Managing State in Class Components
Using this.state to Initialize State
Class components use the this.state
object within the constructor to initialize state. This setup allows the component to manage its own state independently of its parent components.
Updating State with this.setState
State updates in class components are handled through this.setState
, which ensures that state changes are merged and the component re-renders:
this.setState({ count: this.state.count + 1 });
Best Practices for State Management in Class Components
When managing state in class components, it is crucial to minimize direct state mutations, ensure state updates are performed in a controlled manner, and leverage lifecycle methods to handle state changes appropriately.
Managing State in Functional Components
Introduction to useState Hook
The useState
hook, introduced in React 16.8, allows functional components to have state. It returns an array with the current state value and a function to update it.
Updating State with useState
State updates in functional components are performed by calling the state update function returned by useState
:
const [count, setCount] = useState(0);
setCount(count + 1);
Handling Multiple State Variables with useState
Functional components can manage multiple state variables by calling useState
multiple times, each with its own state slice:
const [name, setName] = useState('');
const [age, setAge] = useState(0);
Advanced State Management
Using useReducer for Complex State Logic
For complex state logic involving multiple sub-values or state transitions, useReducer
provides a more scalable and maintainable approach:
const [state, dispatch] = useReducer(reducer, initialState);
Combining useState and useReducer
In some scenarios, combining useState
for simple state and useReducer
for complex state can yield a more efficient state management strategy.
Performance Considerations with Complex State
Complex state management can impact performance. It is essential to optimize state updates and avoid unnecessary re-renders by using memoization and other React performance optimization techniques.
Lifecycle Methods and State
State Management in componentDidMount
The componentDidMount
lifecycle method is used to perform state updates based on initial props or external data fetching:
componentDidMount() {
fetchData().then(data => this.setState({ data }));
}
State Updates in componentDidUpdate
State updates in response to prop changes or other conditions are managed within componentDidUpdate
:
componentDidUpdate(prevProps) {
if (this.props.data !== prevProps.data) {
this.setState({ data: this.props.data });
}
}
Cleaning Up with componentWillUnmount
Cleanup operations, such as cancelling network requests or clearing timers, are handled in componentWillUnmount
:
componentWillUnmount() {
clearTimeout(this.timerID);
}
Props: The Basics
Defining Props in React
Props are read-only attributes passed from parent to child components, enabling data flow and event handling between components.
How Props Differ from State
Unlike state, which is managed within the component, props are external inputs provided by parent components and cannot be modified by the child component.
Passing Props to Child Components
Props are passed to child components in JSX syntax, allowing parent components to configure and control child component behavior:
<MyComponent name="John" />
Using Props Effectively
Destructuring Props for Cleaner Code
Destructuring props in the function signature or within the component body leads to cleaner and more readable code:
function MyComponent({ name, age }) {
return <div>{name} is {age} years old.</div>;
}
Setting Default Props with defaultProps
Default props ensure components have default values for props that are not explicitly provided:
MyComponent.defaultProps = {
name: 'Guest',
};
Validating Props with PropTypes
PropTypes help validate props and ensure they meet the expected type and structure, enhancing code robustness:
MyComponent.propTypes = {
name: PropTypes.string,
age: PropTypes.number,
};
Props in Functional Components
Passing and Using Props in Functional Components
Functional components receive props as function parameters, allowing them to be directly accessed and used within the component body.
Props and useEffect Hook
The useEffect
hook can leverage props to trigger side effects based on prop changes:
useEffect(() => {
console.log(`Name changed to ${name}`);
}, [name]);
Sharing State Between Components with Props
Props facilitate state sharing between components by passing state and state update functions from parent to child components, ensuring a unidirectional data flow.
Props in Class Components
Passing and Using Props in Class Components
Class components receive props via this.props
, enabling them to access and use props within the component methods and lifecycle events.
Updating Child Components with New Props
Child components receive updated props from parent components, triggering re-renders and state updates as needed.
Using Props in Lifecycle Methods
Props can be accessed and utilized in various lifecycle methods to manage component behavior based on prop changes:
componentDidUpdate(prevProps) {
if (this.props.data !== prevProps.data) {
// Handle prop change
}
}
State and Props Interaction
Syncing State and Props
Synchronizing state and props ensures that components accurately reflect the current data and user interactions. This involves setting state based on initial props and updating state in response to prop changes.
When to Lift State Up
Lifting state up to a common ancestor component is necessary when multiple components need to share and synchronize state. This promotes a single source of truth and ensures consistent state management.
Best Practices for State and Props Interaction
Maintain a clear separation between state and props, use controlled components for form inputs, and ensure state updates are handled in a predictable manner to prevent bugs and maintain code clarity.
Common Patterns and Practices
Controlled Components Using State and Props
Controlled components use state and props to manage form inputs, ensuring the component's rendered output reflects the current state:
<input type="text" value={this.state.value} onChange={this.handleChange} />
Uncontrolled Components and Refs
Uncontrolled components manage their own state internally, with refs used to access and manipulate DOM elements directly:
<input type="text" ref={inputRef} />
Using Context API with State and Props
The Context API provides a way to pass state and props deeply through the component tree without prop drilling, enhancing scalability and maintainability.
Handling Complex State and Props
Strategies for Large Applications
For large applications, use state management libraries like Redux or MobX, modularize state logic using custom hooks or HOCs, and maintain a clear state structure.
Using Custom Hooks for State Management
Custom hooks encapsulate reusable state logic, promoting code reuse and reducing duplication across components.
Optimizing Performance with Memoization
Memoization techniques, such as useMemo
and useCallback
, optimize performance by preventing unnecessary re-renders and recalculations.
Debugging State and Props Issues
Common Pitfalls and How to Avoid Them
Avoid common issues such as direct state mutations, improper state initialization, and forgetting to handle prop changes. Use proper state management practices and thorough testing to mitigate these pitfalls.
Using React DevTools for State and Props
React DevTools provides insights into component hierarchies, state, and props, aiding in debugging and optimizing component behavior.
Testing Components with State and Props
Use testing frameworks like Jest and React Testing Library to write unit and integration tests that cover state and prop changes, ensuring components behave as expected under various scenarios.
Real-world Examples
Case Study: State and Props in a To-Do App
A to-do app showcases the practical application of state and props, managing tasks, and user interactions through state updates and prop passing.
Building a Dynamic Form with State and Props
A dynamic form leverages state to manage form data and props to handle form submission and validation, providing a seamless user experience.
State and Props in a Redux-Integrated Application
Integrating Redux with React demonstrates advanced state management techniques, using global state and props to manage application-wide data and behavior.
Best Practices and Recommendations
State and Props Best Practices
Adopt best practices such as minimizing stateful components, using controlled components for forms, and leveraging hooks for state management to build maintainable and efficient applications.
Ensuring Component Reusability
Design components to be reusable by keeping them stateless where possible, using props to configure behavior, and encapsulating complex logic in custom hooks.
Future-Proofing Your React Components
Stay updated with the latest React features and best practices, refactor code regularly, and adopt modern patterns to future-proof your React components.
Conclusion
Recap of Key Points
State and props are integral to React, each serving unique purposes in managing component data and behavior. Mastery of these concepts is crucial for effective React development.
Final Thoughts on State and Props in React
Balancing state and props, leveraging hooks, and adhering to best practices ensures that your React components are robust, maintainable, and performant.
Future Directions in React Development
As React evolves, new patterns and tools will emerge to simplify state and props management. Staying informed and adaptable will be key to leveraging these advancements in your projects.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast