Important Javascript Interview Questions And Answer
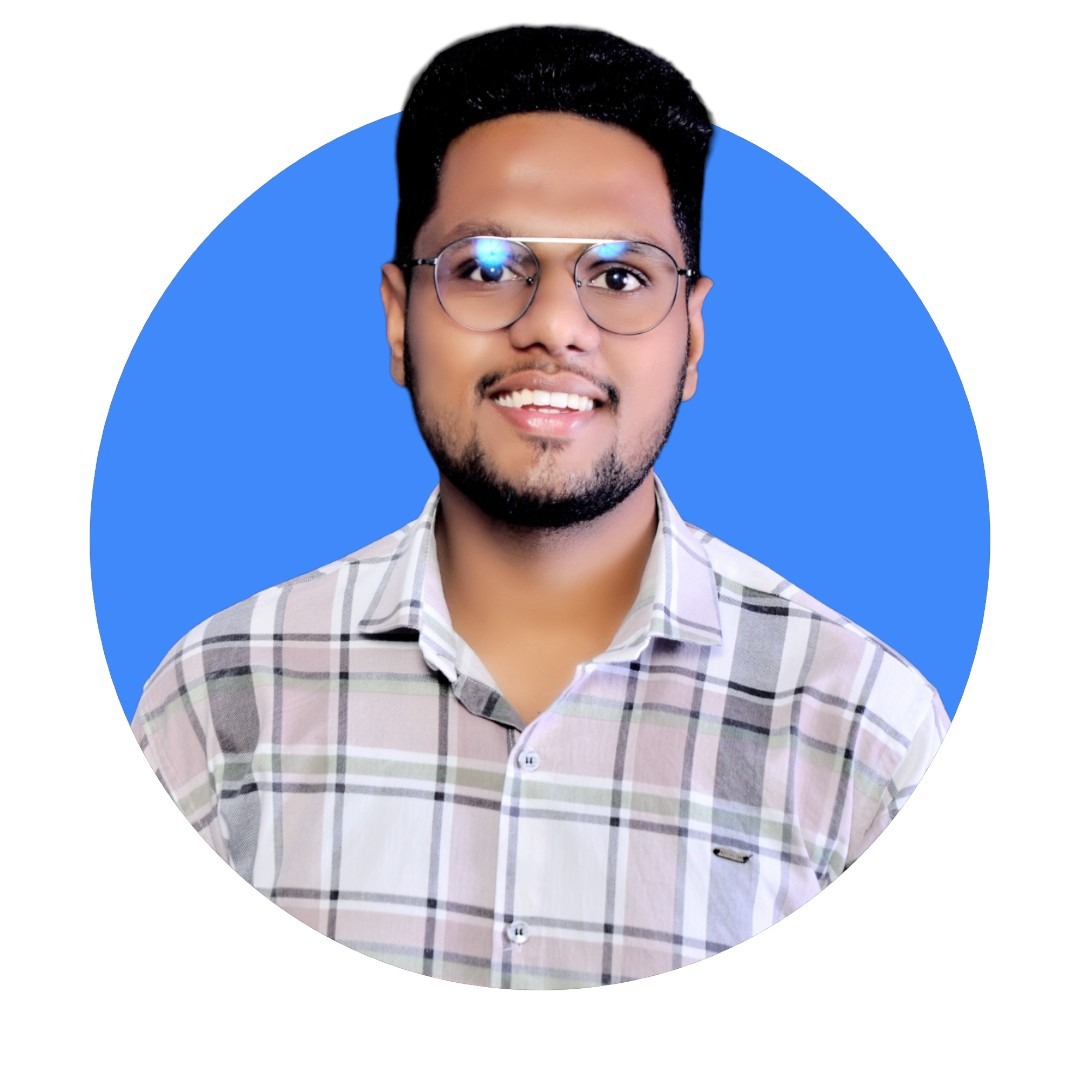
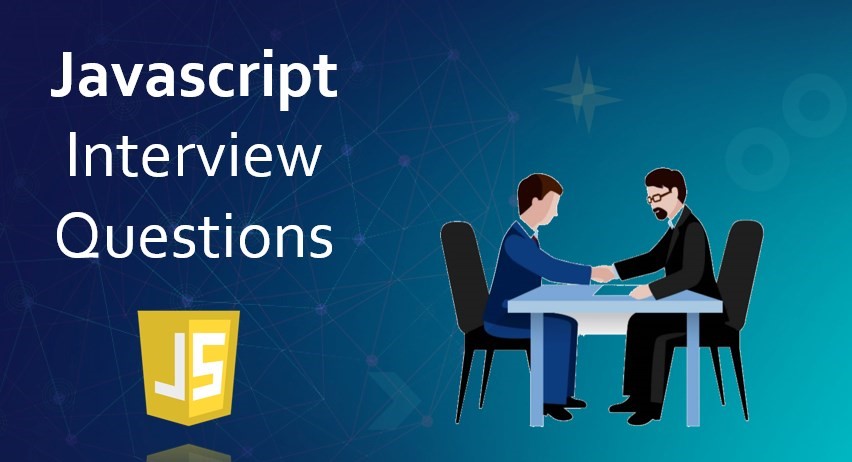
JavaScript Interview Questions :
JavaScript interview questions and answers for provides a list of top 20 interview questions. The frequently asked JavaScript interview questions with answers for beginners and professionals are given below.
1) What is JavaScript?
JavaScript is a scripting language.
It is different from Java language. It is object-based, lightweight, cross-platform translated language.
It is widely used for client-side validation.
The JavaScript Translator (embedded in the browser) is responsible for translating the JavaScript code for the web browser
2) List some features of JavaScript?
Some of the features of JavaScript are:
Lightweight
Interpreted programming language
Good for the applications which are network-centric
Complementary to Java
Complementary to HTML
Open source
Cross-platform
3) Who developed JavaScript, and what was the first name of JavaScript?
JavaScript was developed by Brendan Eich, who was a Netscape programmer.
Brendan Eich developed this new scripting language in just ten days in the year September 1995.
At the time of its launch, JavaScript was initially called Mocha.
After that, it was called Live Script and later known as JavaScript.
4) List some of the advantages of JavaScript?
Some of the advantages of JavaScript are:
Server interaction is less
Feedback to the visitors is immediate
Interactivity is high
Interfaces are richer
5) List some of the disadvantages of JavaScript?
Some of the disadvantages of JavaScript are:
No support for multithreading
No support for multiprocessing
Reading and writing of files is not allowed
No support for networking applications.
6) Define a named function in JavaScript.
The function which has named at the time of definition is called a named function. For example
function msg()
{
document.writeln("Named Function");
}
msg();
7) Name the types of functions?
The types of function are:
Named - These type of functions contains name at the time of definition. For Example:
function display() { document.writeln("Named Function"); } display();
Anonymous - These type of functions doesn't contain any name. They are declared dynamically at runtime.
var display=function()
{
document.writeln("Anonymous Function");
}
display();
8) Define anonymous function?
It is a function that has no name.
These functions are declared dynamically at runtime using the function operator instead of the function declaration.
The function operator is more flexible than a function declaration.
It can be easily used in the place of an expression.
For example:
var display=function()
{
alert("Anonymous Function is invoked");
}
display();
9) Can an anonymous function be assigned to a variable?
Yes, you can assign an anonymous function to a variable.
10) In JavaScript what is an argument object?
The variables of JavaScript represent the arguments that are passed to a function.
11) What is the difference between JavaScript and JScript?
Netscape provided the JavaScript language.
Microsoft changed the name and called it JScript to avoid the trademark issue.
In other words, you can say JScript is the same as JavaScript, but Microsoft provides it.
12)What are the key differences between Java and JavaScript? / How is JavaScript different from Java?
JavaScript is a lightweight programming language (most commonly known as scripting language) developed by Netscape, Inc. It is used to make web pages interactive. It is not a part of the Java platform. Following is a list of some key differences between Java and JavaScript
A list of key differences between Java and JavaScript
Java | JavaScript |
Java is a complete and strongly typed programming language used for backend coding. In Java, variables must be declared first to use in the program, and the type of a variable is checked at compile-time. | JavaScript is a weakly typed, lightweight programming language (most commonly known as scripting language) and has more relaxed syntax and rules. |
Java is an object-oriented programming (OOPS) language or structured programming languages such as C, C++, or .Net. | JavaScript is a client-side scripting language, and it doesn't fully support the OOPS concept. It resides inside the HTML documents and is used to make web pages interactive (not achievable with simple HTML). |
Java creates applications that can run in any virtual machine (JVM) or browser. | JavaScript code can run only in the browser, but it can now run on the server via Node.js. |
The Java code needs to be compiled. | The JavaScript code doesn't require to be complied. |
Java Objects are class-based. You can't make any program in Java without creating a class. | JavaScript Objects are prototype-based. |
Java is a Complete and Standalone language that can be used in backend coding. | JavaScript is assigned within a web page and integrates with its HTML content. |
Java programs consume more memory. | JavaScript code is used in HTML web pages and requires less memory. |
The file extension of the Java program is written as ".Java" and it translates source code into bytecodes which are then executed by JVM (Java Virtual Machine). | The JavaScript file extension is written as ".js" and it is interpreted but not compiled. Every browser has a JavaScript interpreter to execute the JS code. |
Java supports multithreading. | JavaScript doesn't support multithreading. |
Java uses a thread-based approach to concurrency. | JavaScript uses an event-based approach to concurrency. |
13) What is BOM?
BOM stands for Browser Object Model.
It provides interaction with the browser.
The default object of a browser is a window. So, you can call all the functions of the window by specifying the window or directly.
The window object provides various properties like document, history, screen, navigator, location, innerHeight, innerWidth.
14) What is DOM? What is the use of document object?
DOM stands for Document Object Model.
A document object represents the HTML document.
It can be used to access and change the content of HTML.
15) What is the use of window object?
The window object is created automatically by the browser that represents a window of a browser.
It is not an object of JavaScript. It is a browser object.
The window object is used to display the popup dialog box.
Let's see with description.
Method | Description |
alert() | displays the alert box containing the message with ok button. |
confirm() | displays the confirm dialog box containing the message with ok and cancel button. |
prompt() | displays a dialog box to get input from the user. |
open() | opens the new window. |
close() | closes the current window. |
setTimeout() | performs the action after specified time like calling function, evaluating expressions. |
16) How to write a comment in JavaScript?
There are two types of comments in JavaScript.
Single Line Comment: It is represented by // (double forward slash)
Multi-Line Comment: Slash represents it with asterisk symbol as /* write comment here */
17) What is the difference between == and ===?
The == operator checks equality only whereas === checks equality, and data type, i.e., a value must be of the same type.
18) How to write HTML code dynamically using JavaScript?
The innerHTML property is used to write the HTML code using JavaScript dynamically. Let's see a simple example:
document.getElementById('mylocation').innerHTML="<h2>This is heading using JavaScript</h2>";
19) How to create objects in JavaScript?
There are 3 ways to create an object in JavaScript.
By object literal
By creating an instance of Object
By Object Constructor
20) How to create an array in JavaScript?
There are 3 ways to create an array in JavaScript.
By array literal
By creating an instance of Array
By using an Array constructor
21) Difference between Client side JavaScript and Server side JavaScript?
Client-side JavaScript
comprises the basic language and predefined objects which are relevant to running JavaScript in a browser.
The client-side JavaScript is embedded directly by in the HTML pages.
The browser interprets this script at runtime.
Server-side JavaScript
resembles client-side JavaScript.
It has a relevant JavaScript which is to run in a server.
The server-side JavaScript are deployed only after compilation.
22) In which location cookies are stored on the hard disk?
The storage of cookies on the hard disk depends on the OS and the browser.
The Netscape Navigator on Windows uses a cookies.txt file that contains all the cookies.
The path is c:\Program Files\Netscape\Users\username\cookies.txt
The Internet Explorer stores the cookies on a file [email protected]
The path is: c:\Windows\Cookies\[email protected]
23) What is the real name of JavaScript?
The original name was Mocha, a name chosen by Marc Andreessen, founder of Netscape.
In September of 1995, the name was changed to LiveScript.
In December 1995, after receiving a trademark license from Sun, the name JavaScript was adopted.
24) What is the difference between undefined value and null value?
Undefined value: A value that is not defined and has no keyword is known as undefined value.
For example:
- int number;//Here, a number has an undefined value.
Null value: A value that is explicitly specified by the keyword "null" is known as a null value.
For example:
- String str=null;//Here, str has a null value.
25) What is this [[[]]]?
This is a three-dimensional array.
- var myArray = [[[]]];
26) Are Java and JavaScript same?
No, Java and JavaScript are the two different languages. Java is a robust, secured and object-oriented programming language whereas JavaScript is a client-side scripting language with some limitations.
27) What are the pop-up boxes available in JavaScript?
Alert Box
Confirm Box
Prompt Box
28) How can we detect OS of the client machine using JavaScript?
The navigator.appVersion string can be used to detect the operating system on the client machine.
29) How to handle exceptions in JavaScript?
By the help of try/catch block, we can handle exceptions in JavaScript. JavaScript supports try, catch, finally and throw keywords for exception handling.
30) What is the requirement of debugging in JavaScript?
JavaScript didn't show any error message in a browser. However, these mistakes can affect the output. The best practice to find out the error is to debug the code. The code can be debugged easily by using web browsers like Google Chrome, Mozilla Firebox.
To perform debugging, we can use any of the following approaches:
- Using console.log() method
Using debugger keyword
31) What is the role of a strict mode in JavaScript?
The JavaScript strict mode is used to generates silent errors. It provides "use strict"; expression to enable the strict mode. This expression can only be placed as the first statement in a script or a function. For example:
"use strict";
x=10;
console.log(x)
32) What is the use of Math object in JavaScript?
The JavaScript math object provides several constants and methods to perform a mathematical operation. Unlike date object, it doesn't have constructors.
For example:
function display()
{
var map=new Map();
map.set(1,"jQuery");
map.set(2,"AngularJS");
map.set(3,"Bootstrap");
document.writeln(map.get(1)+"<br>");
document.writeln(map.get(2)+"<br>");
document.writeln(map.get(3));
}
display();
33)Important Differences:
jQuery | JavaScript |
It is a javascript library. | It is a dynamic and interpreted web-development programming language. |
The user only need to write the required jQuery code | The user needs to write the complete js code |
It is less time-consuming. | It is more time consuming as the whole script is written. |
There is no requirement for handling multi-browser compatibility issues. | Developers develop their own code for handling multi-browser compatibility. |
It is required to include the URL of the jQuery library in the header of the page. | JavaScript is supportable on every browser. Any additional plugin need not to be included. |
It depends on the JavaScript as it is a library of js. | jQuery is a part of javascript. Thus, the js code may or may not depend on jQuery. |
It contains only a few lines of code. | The code can be complicated, as well as long. |
It is quite an easy, simple, and fast approach. | It is a weakly typed programming approach. |
jQuery is an optimized technique for web designing. | JavaScript is one of the popular web designing programming languages for developers that introduced jQuery. |
jQuery creates DOM faster. | JavaScript is slow in creating DOM. |
Difference between JavaScript and PHP
Following lists the various differences between JavaScript and PHP.
JavaScript | PHP |
Currently, it is a full-stack programming language. It means it can serve both the client as well as server sites. | It is a server-side scripting language. It serves only the backend of the website. |
It is faster but difficult to learn. | It is slower but easy to learn. |
It is an asynchronous programming language that does not wait for the execution of the input-output operations. | It is a synchronous programming language. Thus, it waits for the execution of the input-output operations. |
It is supportable by every web browser, such as Mozilla, Google Chrome, and many more. | It is supportable on Windows, Linux, Mac, etc. platforms. Supported by IIS, Apache, and Lighttpd web servers. |
It carries less securable code. | PHP code is highly securable. |
It requires an environment for accessing the database. | It allows easy and direct access to the database. |
The code is written within <script>...<script> tags. | The code is written within <?php....?> tag. |
Earlier, javascript was able to create interactive pages for the clients. But, at present, it is able to build real-time games and applications, mobile applications, too. | A PHP program is able to generate dynamic pages, send cookies, receive cookies, collect form data, etc. |
It is a single-threaded language. Thus, each input-output operation is concurrently carried out. | It is a multi-threaded language. Thus, it blocks if multiple input-output operations execute. |
The extension used to save an external javascript file is '.js'. | Files are saved using '.php' extension. |
We can embed the js code in HTML, XML, and AJAX. | We can embed the PHP code only with HTML. |
It supports fewer features. | It supports more advanced features as compared to JavaScript. |
Popular frameworks of JavaScript are Angular, React, Vue.js, Meteor, etc. | Popular frameworks of PHP are Laravel, Symfony, FuelPHP, CakePHP, and many more. |
Websites built in JavaScript are Twitter, LinkedIn, Amazon, etc. | Websites built in PHP are Wordpress, Tumblr, MailChimp, iStockPhoto, etc. |
Different between Dart and JavaScript
The following comparison chart explains the head-to-head comparison between Dart and JavaScript:
Features | Dart | JavaScript |
Ease of use | Dart has a Java-like syntax and coding style, so developers with an OOPS experience can easily use it. | JavaScript is simple to use, and it has various frameworks and libraries available online and allows developers to reuse existing code to create apps faster. |
Editor and IDE support | Dart code may be written in lightweight editors like Sublime or VIM. The most commonly used IDEs for Dart application development is IntelliJ IDEA and Android Studio, which come with the Dart plugin. | It provides some excellent editors and IDEs for app development. It is not always necessary to use a full-fledged IDE; instead, developers may use lightweight editors such as VIM, Sublime Text, Emacs, or Atom. Some IDEs, such as WebStorm and Visual Studio Code, can be useful for JavaScript application creation. |
Productivity | Dart and Flutter have a smaller community as well as several online communities. Despite excellent documentation, many experienced developers are still confused about understanding Dart. | It is easier for new developers to learn because it's a simpler, lightweight, dynamic programming language. It increases developer productivity by providing various JavaScript frameworks and thousands of easily available web packages. |
Type safety | It is more type-safe than JavaScript because it allows for both loose and strong prototyping. | It is not a type-safe language because it supports both duck-typing and dynamic. |
Learning Curve | Dart programming language can be challenging for beginners because it is not a widely-used programming language. There are very few Dart programming courses or books available online. | For beginners, JavaScript is not an easy language to learn, but knowing the fundamentals of programming makes JavaScript simple. |
Commercial Use | Dart is supported by Google and is also used by big brands such as Blossom, WorkTrails, Whale, Mobile, and others. | JavaScript is widely used in large corporations such as Instagram, Slack, Reddit, eBay, and Airbnb to build web and cross-platform mobile applications. |
Speed | Dart may be compiled both JIT and AOT, which allows for creating apps in different ways. Dart is much faster than JavaScript. | JavaScript is an interpreted language, so it could be felt lighter and faster than other programming languages. It is faster than Java and other compiled languages. |
Web and Mobile | Dart could be used to develop both web and mobile applications. | JavaScript may be used in both web and mobile app development with several frameworks. |
Comparison between JavaScript and Angular JS
Here, we are going to discuss a head-to-head comparison between JavaScript and Angular JS:
Features | JavaScript | Angular JS |
Definition | It is an object-oriented scripted language that is used to develop mobile and dynamic web applications. | It an open-source framework used to develop dynamic web and large single-page web applications. |
Developed | Netscape Communications developed it in 1995. | Google mainly developed it in 2010. |
Syntax | Its syntax is much complex than Angular JS. | Its syntax is simple and easy. |
Programmed | Its interpreters are written in the C and C++ languages. | It is written in the JavaScript language. |
Filters | It doesn't support the filters. | It does support filters. |
Learnability | It isn't very easy to learn. | It is easy to learn if anyone knows the basic knowledge of JavaScript. |
Concept | It is based on the dynamic typing concept. | Angular JS is based on the concept of the model view controller to build the applications. |
Dependency injection | It doesn't support the dependency injection. | It supports both data binding and dependency injection. |
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
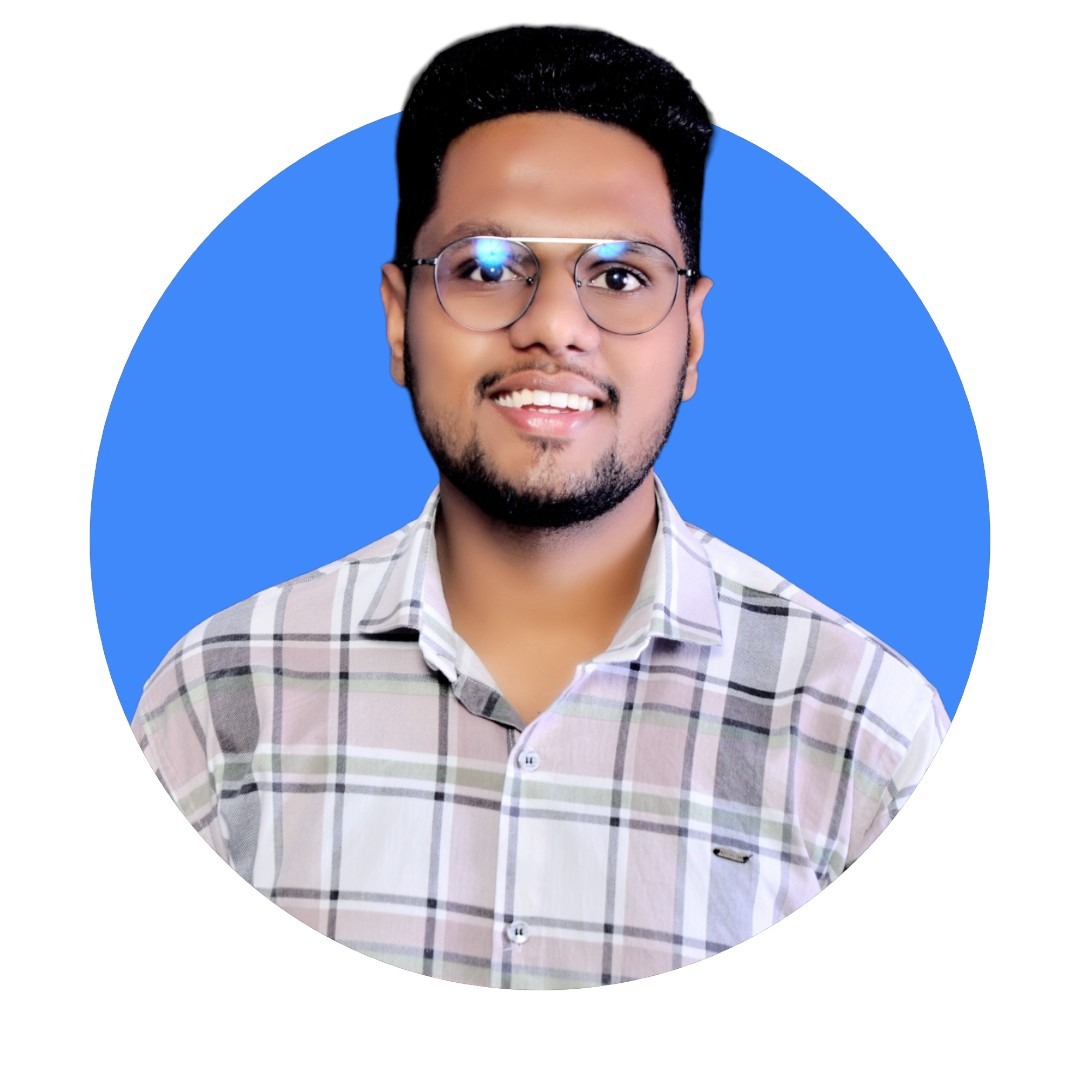
Aditya Gadhave
Aditya Gadhave
๐ Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.