Building Secure Applications: Best Practices for Developers
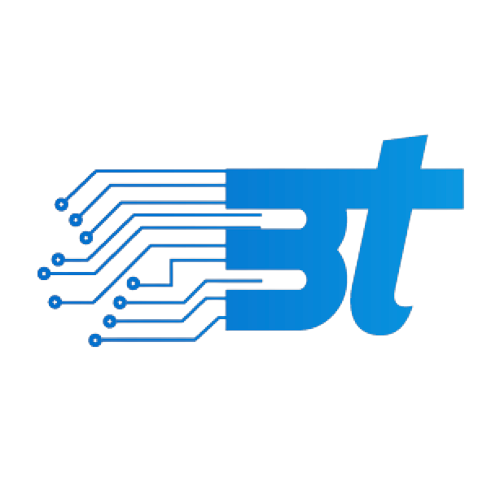
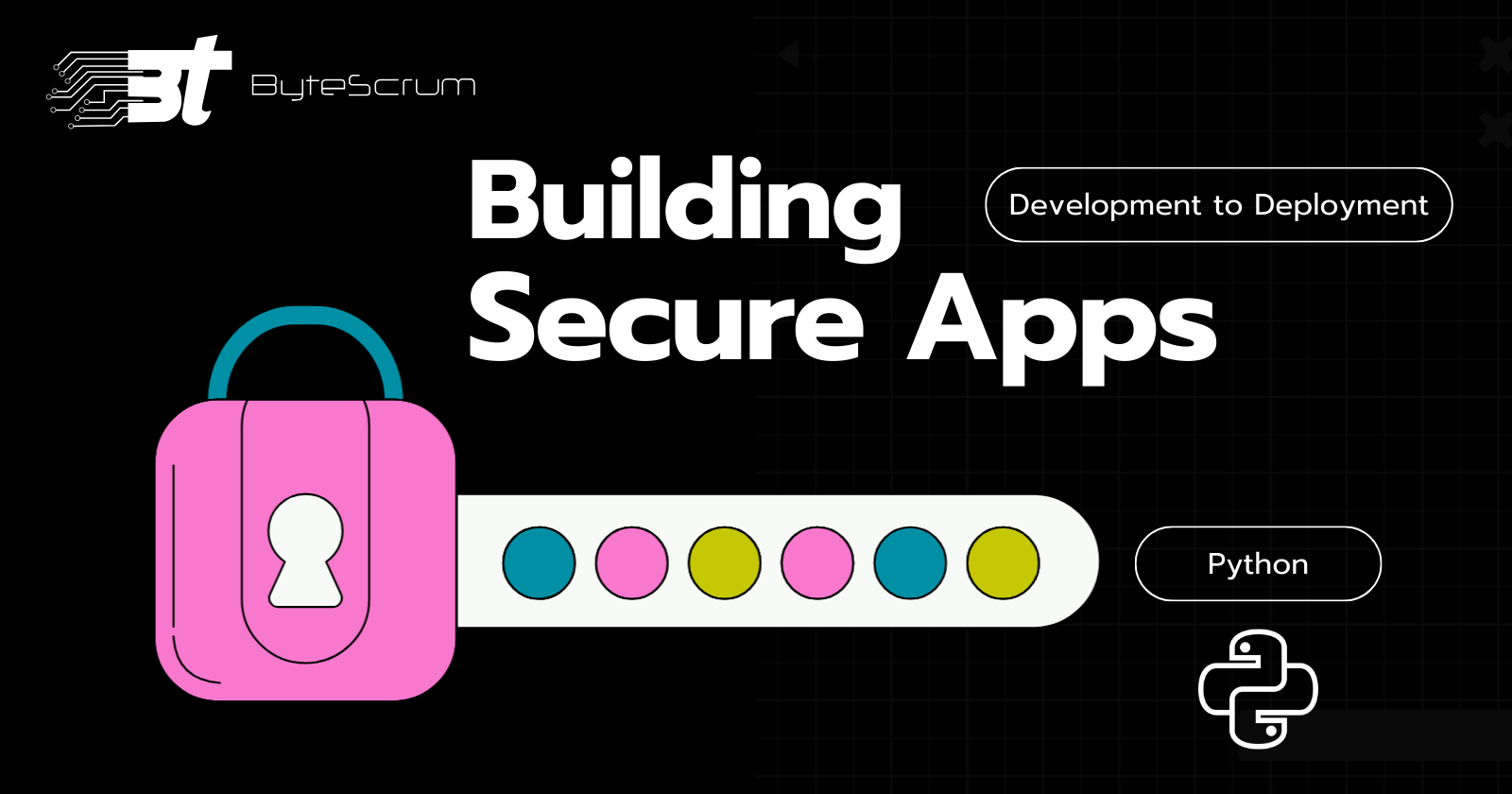
In an increasingly digital world, security is paramount. As developers, it’s our responsibility to ensure that the applications we build are robust and secure, protecting user data and maintaining trust. Here are some essential best practices to follow when building secure applications, along with practical examples.
1. Understand the Basics of Security
Before diving into specific techniques, it's crucial to have a solid understanding of the fundamental principles of security:
Confidentiality: Ensuring that data is accessible only to those authorized to access it.
Integrity: Maintaining the accuracy and completeness of data.
Availability: Ensuring that data and services are available to users when needed.
2. Implement Strong Authentication and Authorization
Authentication
Use Strong Password Policies: Enforce complex passwords and require regular updates. Use libraries like bcrypt to hash passwords securely.
import bcrypt password = b"super_secret_password" hashed = bcrypt.hashpw(password, bcrypt.gensalt()) if bcrypt.checkpw(password, hashed): print("Password matches")
Multi-Factor Authentication (MFA): Implement MFA to add an extra layer of security. Services like Authy or Google Authenticator can be integrated to provide MFA.
Authorization
Role-Based Access Control (RBAC): Define roles and permissions clearly, ensuring that users only have access to the resources they need.
roles = { "admin": ["add_user", "delete_user", "view_data"], "user": ["view_data"] } def check_permission(role, action): if action in roles.get(role, []): return True return False
- Least Privilege Principle: Always grant the minimal level of access required for users to perform their tasks.
3. Protect Sensitive Data
Data Encryption
Encrypt Data in Transit: Use TLS (Transport Layer Security) to encrypt data being transmitted between clients and servers.
# Example for Flask app from flask import Flask, request from OpenSSL import SSL app = Flask(__name__) context = SSL.Context(SSL.TLSv1_2_METHOD) context.use_privatekey_file('path/to/private.key') context.use_certificate_file('path/to/certificate.crt') @app.route('/') def index(): return 'Hello, Secure World!' if __name__ == "__main__": app.run(ssl_context=context)
Encrypt Data at Rest: Encrypt sensitive data stored in databases and file systems using strong encryption algorithms like AES (Advanced Encryption Standard).
from cryptography.fernet import Fernet key = Fernet.generate_key() cipher_suite = Fernet(key) cipher_text = cipher_suite.encrypt(b"Sensitive Data") plain_text = cipher_suite.decrypt(cipher_text)
Data Masking
Mask Sensitive Data: Mask or obfuscate sensitive data (like credit card numbers) when displaying it to users, ensuring that only necessary parts are visible.
def mask_credit_card(card_number): return "**** **** **** " + card_number[-4:] print(mask_credit_card("1234567812345678")) # Output: **** **** **** 5678
4. Secure Your Code
Input Validation
Validate Inputs: Always validate and sanitize user inputs to prevent common attacks like SQL injection and cross-site scripting (XSS).
from flask import request from markupsafe import escape @app.route('/user/<username>') def show_user_profile(username): username = escape(username) return f'User {username}'
Use Whitelisting: Prefer whitelisting over blacklisting for input validation to ensure only known good data is accepted.
ALLOWED_EXTENSIONS = {'png', 'jpg', 'jpeg', 'gif'} def allowed_file(filename): return '.' in filename and filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
Secure Coding Practices
Avoid Hardcoding Secrets: Never hardcode sensitive information such as API keys, passwords, or cryptographic keys in your source code. Use environment variables or secret management tools like AWS Secrets Manager or HashiCorp Vault.
import os api_key = os.getenv("API_KEY")
- Regular Code Reviews: Conduct regular code reviews to identify and fix security vulnerabilities early in the development process.
5. Implement Logging and Monitoring
Logging
Log Security Events: Log critical security events, such as failed login attempts, access to sensitive resources, and configuration changes.
import logging logging.basicConfig(filename='app.log', level=logging.INFO) logging.info('User logged in')
- Secure Logs: Ensure that logs are stored securely and that sensitive information within logs is encrypted or masked.
Monitoring
Continuous Monitoring: Implement continuous monitoring to detect and respond to security incidents in real-time. Use tools like Splunk or ELK Stack for log analysis and monitoring.
Alerting: Set up alerts for suspicious activities, such as multiple failed login attempts or unauthorized access to sensitive data.
6. Keep Your Dependencies and Environment Secure
Dependency Management
Use Trusted Sources: Only use libraries and frameworks from trusted sources. Regularly audit your dependencies for known vulnerabilities.
pip install safety safety check
- Regular Updates: Keep your dependencies up to date to ensure that security patches are applied promptly.
Secure Configuration
Environment Hardening: Secure your application environment by disabling unnecessary services, applying security patches, and configuring firewalls.
Least Privilege for Services: Run application services with the least privileges necessary, reducing the potential impact of a security breach.
7. Educate and Train Your Team
Security Awareness
Regular Training: Conduct regular security training sessions for your development team to keep them updated on the latest security threats and best practices.
Security Champions: Designate security champions within your team to advocate for security best practices and conduct peer reviews.
Security Policies
Develop Policies: Establish clear security policies and guidelines for your organization, covering aspects like password management, data handling, and incident response.
Enforce Policies: Ensure that security policies are enforced consistently across your organization.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
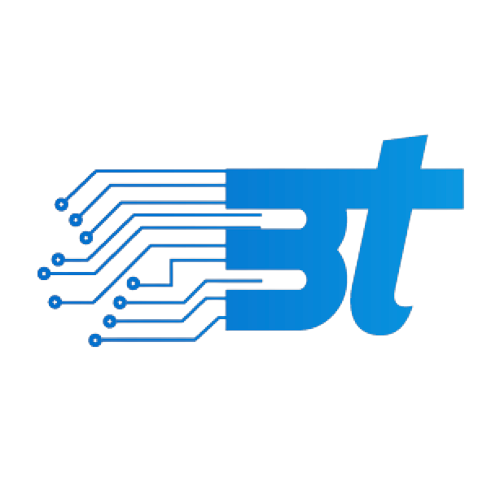
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.