Handling Events in React

Table of contents
- Introduction
- Basics of Handling Events
- Handling Events in JSX
- Commonly Used Events
- Handling Form Events
- Event Bubbling and Capturing
- Event Delegation in React
- SyntheticEvent in React
- Handling Asynchronous Events
- Event Handling Best Practices
- Testing Event Handlers
- Event Handling in Class Components
- Event Handling in Functional Components
- Real-World Examples
- Conclusion
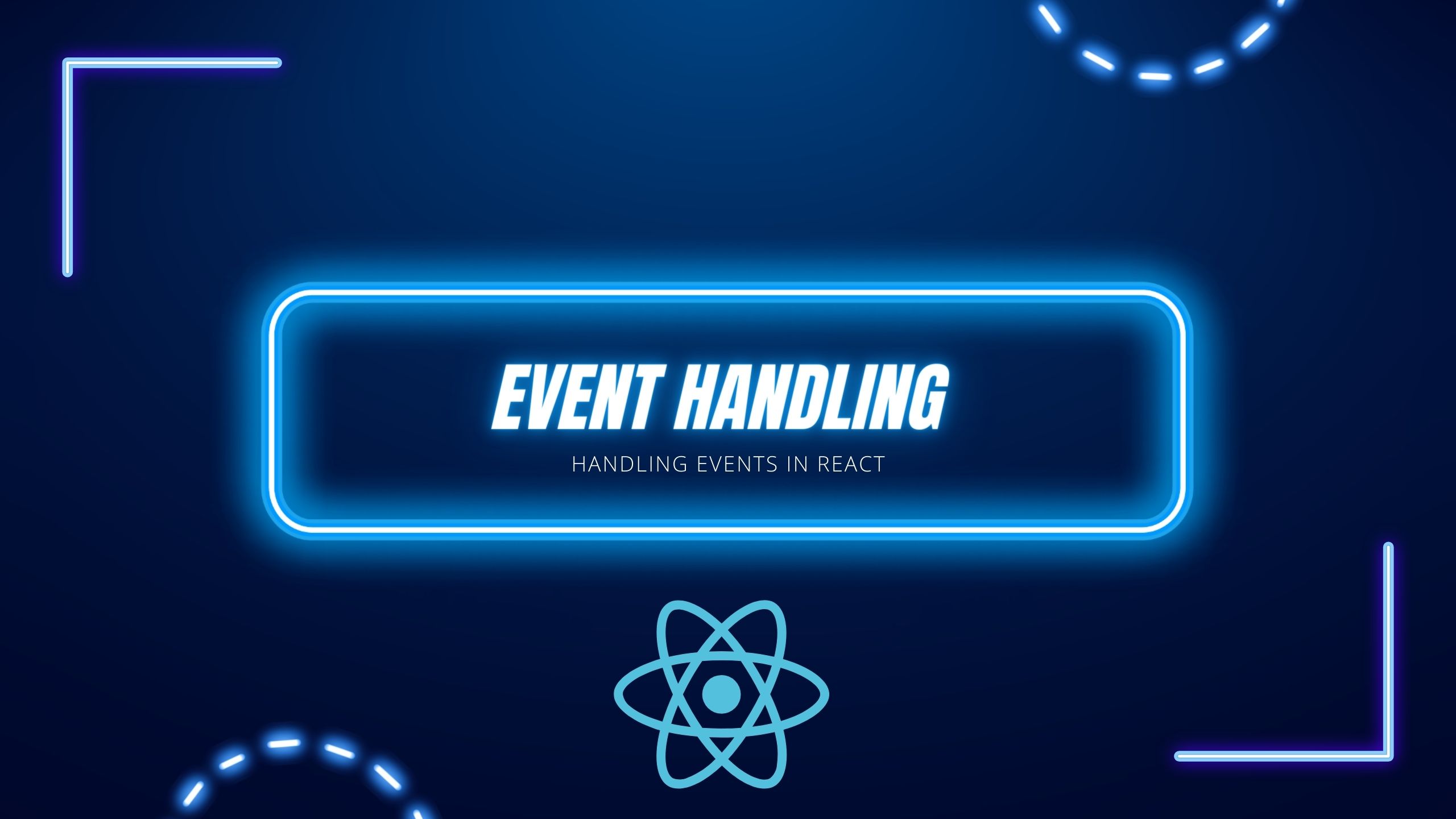
Introduction
Event handling is crucial in React development, influencing how users interact with applications. Understanding its nuances ensures responsive and intuitive user interfaces.
Understanding Event Handling in React
Event handling in React allows developers to capture and respond to user actions, making applications interactive and dynamic.
The Importance of Event Handling in Frontend Development
Efficient event handling enhances user experience by enabling seamless interaction with web applications, ensuring responsiveness and usability.
Basics of Handling Events
Overview of Events in JavaScript and React
Events in JavaScript are actions triggered by users or the system, such as clicks or keystrokes. In React, events are handled similarly but with JSX syntax.
Event Listeners and Event Handlers in React
Event listeners attach functions to specific events, while event handlers are functions that respond to these events, updating the UI or performing actions.
Handling Events in JSX
Inline Event Handlers in JSX
Inline event handlers in JSX allow developers to directly specify event handling logic within JSX tags, simplifying code and enhancing readability.
Passing Arguments to Event Handlers
Event handlers can receive arguments to provide context or additional data, enabling dynamic event handling based on user input or application state.
Preventing Default Behavior
By calling event.preventDefault()
within event handlers, developers can override default browser behavior like form submissions or anchor link navigation.
Commonly Used Events
onClick, onSubmit, onChange: Explained
These events are fundamental in React:
onClick
: Triggered by mouse clicks.onSubmit
: Fired upon form submission.onChange
: Reacts to input field changes, like text input or checkbox toggling.
Keyboard Events: onKeyDown, onKeyUp, onKeyPress
Keyboard events capture keystrokes:
onKeyDown
: Fires when a key is pressed down.onKeyUp
: Triggers when a key is released.onKeyPress
: Registers character input, excluding non-printable keys.
Mouse Events: onMouseOver, onMouseOut, etc.
Mouse events respond to mouse interactions:
onMouseOver
: Activates when the mouse moves over an element.onMouseOut
: Fires when the mouse leaves an element.And more, catering to diverse user interactions.
Handling Form Events
Form Submission and Validation
Handling form submission involves capturing form data and validating inputs to ensure data integrity and user-friendly error messages.
Controlled vs. Uncontrolled Components
Controlled components manage form data through state, while uncontrolled components use refs for direct DOM manipulation, each suited for different scenarios.
Event Bubbling and Capturing
Understanding Event Bubbling in React
Event bubbling propagates events from the target element up through its ancestors, allowing multiple elements to respond to the same event.
Event Capturing and its Usage
Event capturing occurs before bubbling, capturing events at the top of the DOM hierarchy down to the target element, useful for specific event handling strategies.
Event Delegation in React
Advantages of Event Delegation
Event delegation optimizes performance by attaching a single event listener to a parent element, handling events for all its descendants efficiently.
Implementing Event Delegation with React
In React, event delegation simplifies managing dynamic UIs by centralizing event handling logic, reducing complexity and improving code maintainability.
SyntheticEvent in React
Introduction to SyntheticEvent
SyntheticEvent is React's cross-browser wrapper for native DOM events, ensuring consistent event handling across different browsers.
Accessing Event Properties in SyntheticEvent
SyntheticEvent provides properties like event.target
and event.preventDefault()
, empowering developers with rich event data and control.
Handling Asynchronous Events
Using Promises with Event Handlers
Promises manage asynchronous operations triggered by events, handling tasks like fetching data or executing time-consuming operations without blocking the UI.
Async/Await with Event Handling
Async/Await simplifies asynchronous event handling, making code cleaner and easier to reason about, especially when chaining multiple async operations.
Event Handling Best Practices
Separation of Concerns: Single Responsibility Principle
Adhering to the Single Responsibility Principle ensures event handlers focus on specific tasks, promoting code clarity and maintainability.
Avoiding Inline Functions for Event Handlers
Avoiding inline functions in JSX prevents unnecessary function re-creations on each render, optimizing performance and minimizing memory usage.
Testing Event Handlers
Writing Tests for Event Handling Functions
Unit tests validate event handling logic, ensuring events trigger expected behavior and interactions perform as intended across different scenarios.
Using Testing Libraries for Event Handling Tests
Testing libraries like Jest and React Testing Library provide utilities to simulate events and verify event handling functionality, enhancing test coverage and reliability.
Event Handling in Class Components
Defining Event Handlers in Class Components
In class components, event handlers are defined as methods, allowing access to component state and lifecycle methods for complex event handling scenarios.
Binding Event Handlers in Class Components
Binding event handlers in class components ensures the correct this
context within event handler methods, avoiding errors and maintaining expected behavior.
Event Handling in Functional Components
Using Hooks for Event Handling: useEffect
Functional components utilize useEffect hook for event handling, managing side effects and subscribing to events during component lifecycle.
Best Practices for Event Handling in Functional Components
Encapsulating event handling logic in custom hooks promotes reusability and separation of concerns, facilitating clean and modular code structures.
Real-World Examples
Implementing Event Handling in a To-Do List App
A to-do list app showcases practical event handling: adding tasks via form submission, toggling task completion with clicks, and filtering tasks by category.
Interactive Forms: Event Handling Techniques
Forms demonstrate event handling versatility: validating inputs on change events, dynamically updating form data, and submitting forms with controlled inputs.
Conclusion
Summary of Event Handling Techniques in React
Mastering React's event handling equips developers with tools to create interactive and responsive user interfaces, enhancing overall application usability.
Key Takeaways on Efficient Event Handling Practices
Adopting efficient event handling practices ensures smooth user interactions, optimized performance, and scalable frontend development in React.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
π Hi there! I'm Manvendra, a tech enthusiast with a knack for turning ideas into reality. I love making cool stuff that makes life easier and a lot more fun. π§ My toolbox is packed with skills in WordPress, and Iβm always on the lookout for the next exciting project to dive into. I thrive in environments where innovation meets practicality, and I enjoy collaborating with like-minded techies who share my passion for creativity. π Apart from that you can find me exploring new places, soaking in different cultures, and satisfying my inner foodie. From street food stalls in Delhi to fine dining in Bengaluru, Iβm on a never-ending quest for the next great culinary adventure. Letβs connect and chat about tech, travel, or the best pizza spots in town! ππβοΈ