Understanding Java HashMap for DSA
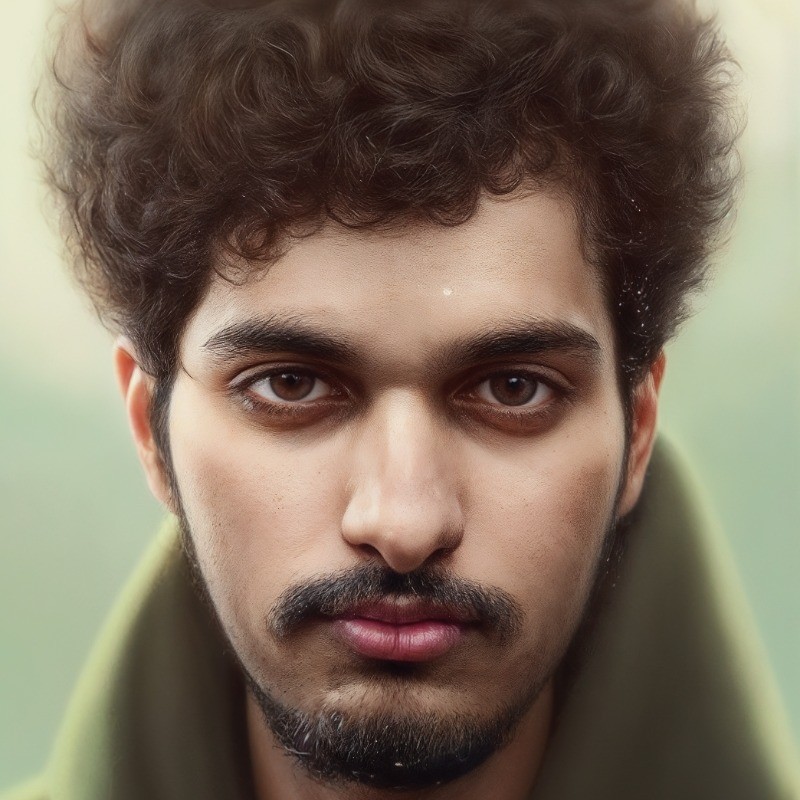
A HashMap
in Java is a part of the Java Collections Framework and provides a way to store key-value pairs, where keys are unique. It is part of the java.util
package and implements the Map
interface. The HashMap
class allows null
values and the null
key, and it does not guarantee the order of its elements over time.
Key Characteristics
Key-Value Pairs: Stores data in key-value pairs. Each key is associated with exactly one value.
Unique Keys: Keys are unique; duplicate keys are not allowed.
Null Values: Allows one
null
key and multiplenull
values.Unordered: This does not guarantee any specific order of the elements.
Not Synchronized: The
HashMap
itself is not synchronized. For concurrent access, useConcurrentHashMap
.
Constructor
Default Constructor: This creates an empty
HashMap
with the default initial capacity (16) and the default load factor (0.75).HashMap<String, Integer> map = new HashMap<>();
Constructor with Initial Capacity: This creates an empty
HashMap
with the specified initial capacity and the default load factor (0.75).HashMap<String, Integer> map = new HashMap<>(20);
Constructor with Initial Capacity and Load Factor: This creates an empty
HashMap
with the specified initial capacity and load factor.HashMap<String, Integer> map = new HashMap<>(20, 0.8f);
Constructor with Map: This creates a new
HashMap
with the same mappings as the specifiedMap
Map<String, Integer> anotherMap = new HashMap<>(); anotherMap.put("key1", 1); anotherMap.put("key2", 2); HashMap<String, Integer> map = new HashMap<>(anotherMap);
Common Methods
put(K key, V value): Associates the specified value with the specified key in this map.
hashMap.put("key1", "value1");
get(Object key): Returns the value to which the specified key is mapped or
null
if this map contains no mapping for the key.String value = hashMap.get("key1");
remove(Object key): Removes the mapping for the specified key from this map if present.
hashMap.remove("key1");
containsKey(Object key): Returns
true
if this map contains a mapping for the specified key.boolean contains = hashMap.containsKey("key1");
containsValue(Object value): Returns
true
if this map maps one or more keys to the specified value.boolean contains = hashMap.containsValue("value1");
size(): Returns the number of key-value mappings in this map.
int size = hashMap.size();
isEmpty(): Returns
true
if this map contains no key-value mappings.boolean empty = hashMap.isEmpty();
Iteration
HashMap
can be iterated in various ways such as for-each loop on keys, for-each loop on entries, and forEach
method.
for (String key : hashMap.keySet()) {
System.out.println("Key: " + key + ", Value: " + map.get(key));
}
for (Map.Entry<String, Integer> entry : hashMap.entrySet()) {
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
hashMap.forEach((key, value) -> System.out.println("Key: " + key + ", Value: " + value));
Performance Considerations
Time Complexity: The average time complexity for
get
,put
, andremove
operations is O(1). However, in the worst case (due to hash collisions), it can be O(n).Load Factor: The load factor determines when the capacity of the
HashMap
is increased. A higher load factor means less space overhead but potentially slower access times.Initial Capacity: The initial capacity of the
HashMap
determines the number of buckets when theHashMap
is created. Choosing an appropriate initial capacity can reduce the need for resizing.
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
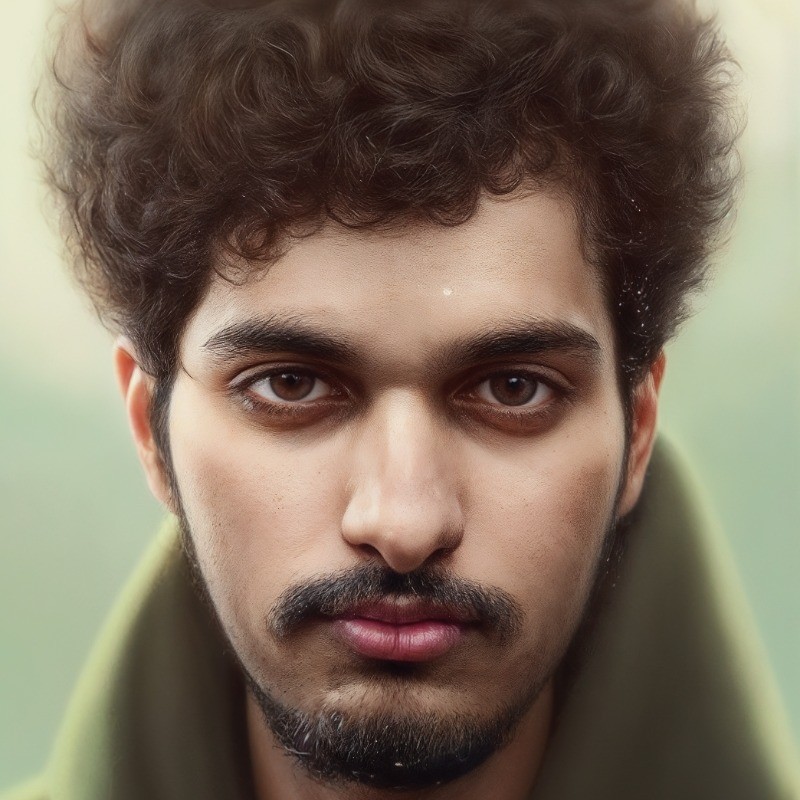
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?