Understanding Hoisting and the Temporal Dead Zone in JavaScript
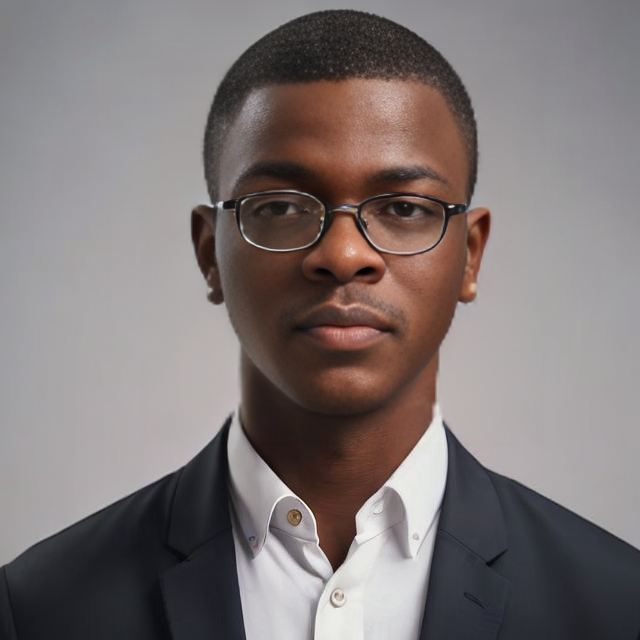
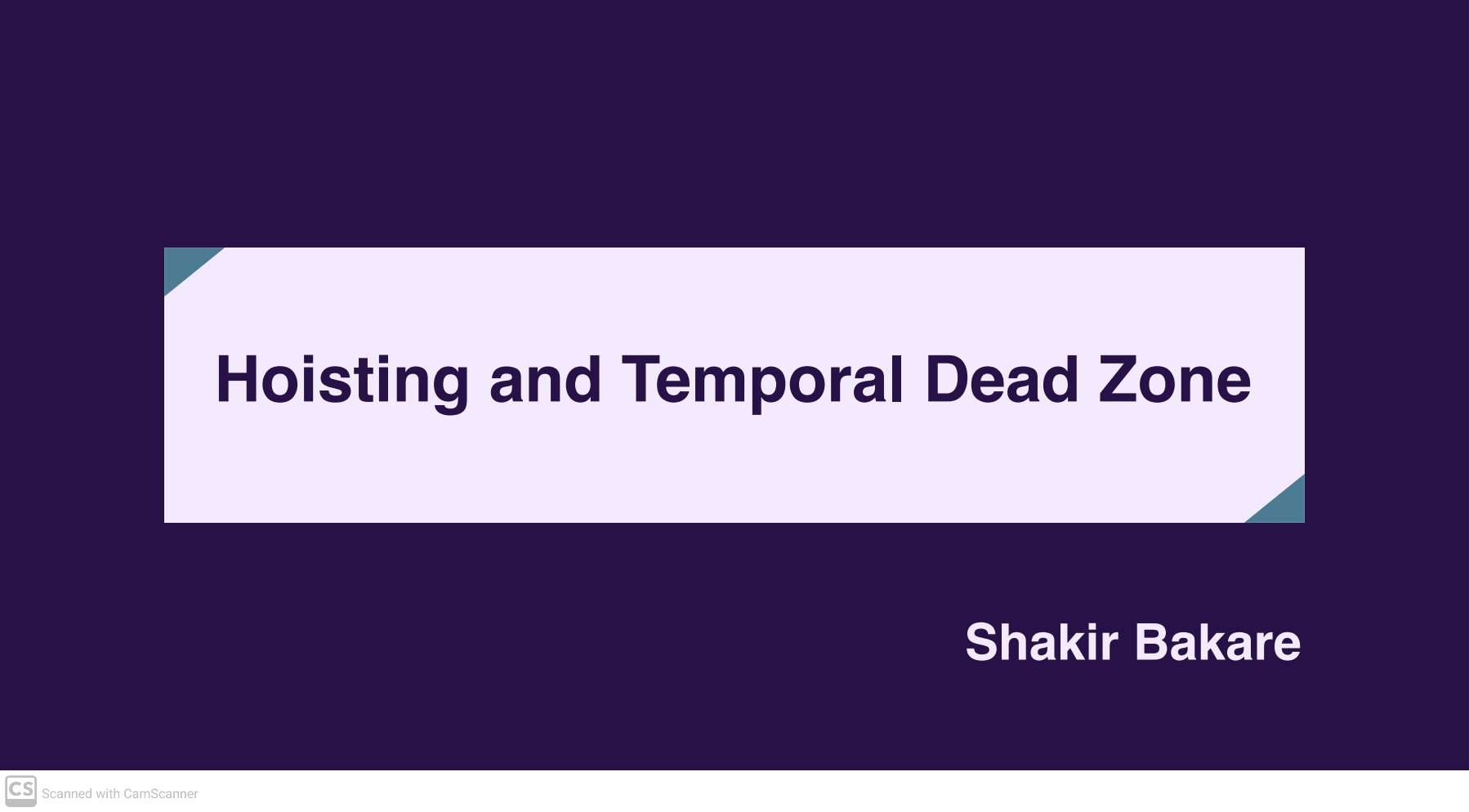
I'm sure you've encountered the error message Cannot access 'x' before initialization
or seen undefined
when running JavaScript code. These issues arise due to hoisting and the Temporal Dead Zone (TDZ). Understanding these concepts is crucial for writing predictable and error-free code. In this article, I'll explain hoisting and the Temporal Dead Zone, how they work, and how you can avoid related errors.
What is hoisting?
Hoisting is JavaScript's inbuilt behavior that moves declarations to the top of their scope during compilation.
How hoisting works
Look at this example:
console.log(name);
// outputs undefined.
var name = “Shakir”;
console.log(name);
// outputs Shakir.
In the example, you can see that the output is undefined
the first time console.log
accesses the name
variable. And the output is Shakir
(the expected value of the name variable) the second time console.log
accesses the variable. That is the work of hoisting.
To make it clearer, this is how the above example looks behind the scene during hoisting:
var name;
console.log(name);
// outputs undefined.
var name = “Shakir”;
console.log(name);
// outputs Shakir.
The full declaration and assignment var name = "Shakir"
is at the fourth line. But hoisting moves the declaration var name
to the top. That is why console.log
can access it.
But the output is undefined
because hoisting does not move the assignment = "Shakir"
to the top. And later when the full declaration and assignment is met, console.log
outputs Shakir
.
That is how hoisting works. But that's not all about it. Hoisting works differently for different declarations, depending on the keyword you use for the declarations. The way hoisting works on let
and const
, is different from the way it does on var
.
Hoisting on var
Hoisting moves var
declarations to the top, but it does not move their assignments. Hoisting keeps the assignments undefined
until the full declarations and assignments are met. You've already seen this above in the example of how hoisting works.
Hosting on let and const
Hoisting also moves let
and const
declarations to the top. But you will get ReferenceError: Cannot access 'theVariableName' before initialization
if you try to use them before their full declarations and assignments are met. This is because of Temporal Dead Zone (TDZ).
Here is what I mean:
console.log(name);
// ReferenceError:
// Cannot access 'name'
// before initialization
let name = "Shakir";
console.log(name);
// Outputs Shakir.
console.log(age);
// // ReferenceError:
// Cannot access 'name'
// before initialization
const age = 9;
console.log(age);
// Outputs 9.
Temporal Dead Zone
The Temporal Dead Zone is a behavior in JavaScript that puts variables declare with let
or const
in a state where they are inaccessible until their full declarations and assignments are met. As I state before, you will get ReferenceError
when you try to access variables that are in Temporal Dead Zone. TDZ exists to prevent access to variables before they are created and assigned values, making your code predictable and error-free.
That's about Temporal Dead Zone. But I'm not done explaining hoisting.
Hoisting on functions
Hoisting also works on functions. This means you can call functions before you define them.
Example:
intro();
// outputs I am Shakir
function intro(){
console.log("I am Shakir");
}
The function intro();
outputs I am Shakir
when it's called because hoisting moves the function declaration to the top.
This is how that looks behind the scene:
function intro(){
console.log("I am Shakir");
}
intro();
// outputs I am Shakir
function intro(){
console.log("I am Shakir");
}
As hoisting can be helpful in some cases, it can also be the cause of errors. That is why it's important to know how to prevent hoisting-related errors.
How to prevent hoisting-related errors
Use
let
andconst
instead ofvar
.Assign values to variables when you declare them.
Declare variables at the top of their scope.
Understand the scope of your variables and how hoisting affects their availability within that scope.
Conclusion
Understanding hoisting and the Temporal Dead Zone (TDZ) is crucial for writing reliable JavaScript code. Hoisting moves declarations to the top of their scope, while the TDZ prevents the use of variables until they are fully declared and assigned. By using let
and const
instead of var
, assigning values to variables upon declaration, declaring variables at the top of their scope, and understanding variable scope, you can avoid common errors associated with hoisting and TDZ.
Thank you for reading. If you found this article helpful, please like and share it with others who might benefit. I would also appreciate your feedback, so feel free to leave a comment with your thoughts and questions. All the best, and enjoy using your new knowledge of hoisting and the Temporal Dead Zone to prevent errors in your code.
Let's connect
Subscribe to my newsletter
Read articles from Shakir Bakare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
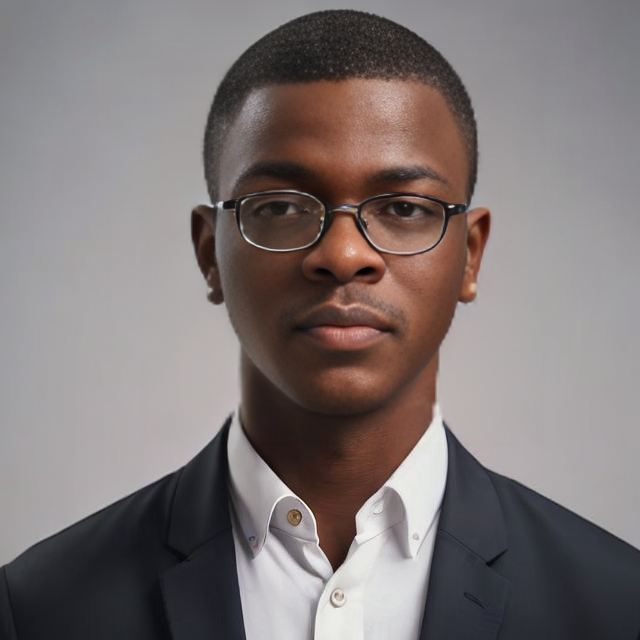
Shakir Bakare
Shakir Bakare
I'm a passionate frontend web developer with a love for creating beautiful and responsive web applications. With a strong foundation in HTML, CSS, JavaScript, and frameworks like React and Vue.js, I strive to bring innovative ideas to life. When I'm not coding, I enjoy sharing my knowledge through technical writing. I believe in the power of clear and concise documentation, and I write articles and tutorials to help others on their coding journey. Let's connect and create something amazing together!