Exploring the Latest Features in Java Version 21
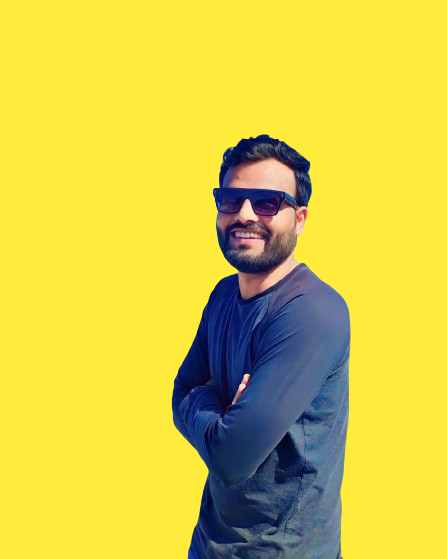
Table of contents
- 1. Sequenced Collections: Keeping Things in Order
- 2. Record Patterns: Making Patterns Easy to Understand
- 3. Virtual Threads: Handling Many Things at Once
- 4. String Templates: Making Strings More Flexible
- 5. Foreign Function & Memory API: Talking to Other Languages
- 6. Unnamed Patterns and Variables: Focusing on What Matters
Hey Java enthusiasts! Java 21 has just landed with fantastic new features that will improve your coding experience. Whether you're new to Java or a seasoned developer, let's explore what Java 21 offers with clear examples to help you understand.
1. Sequenced Collections: Keeping Things in Order
Java 21 introduces sequenced collections, which are like lists that maintain the order of elements and offer convenient methods to access them. Imagine you have a list of tasks in order:
List<String> tasks = List.of("Task A", "Task B", "Task C");
// Get the first task
String firstTask = tasks.get(0);
System.out.println("First task: " + firstTask);//Task A
// Get the last task
String lastTask = tasks.get(tasks.size() - 1); //Task C
System.out.println("Last task: " + lastTask);
With sequenced collections, you can easily retrieve the first ("Task A") and last ("Task C") elements of the list.
2. Record Patterns: Making Patterns Easy to Understand
Records in Java 21 now support record patterns, making it easier to match and process data. Here's how you can use record patterns to handle different types of shapes:
record Circle(double radius) {}
record Rectangle(double width, double height) {}
// Example usage
Shape shape = new Circle(5.0);
if (shape instanceof Circle circle) {
double area = Math.PI * circle.radius() * circle.radius();
System.out.println("Area of the circle: " + area);
}
In this example, the instanceof
check and record the pattern Circle circle
allow you to extract and use the radius of a circle shape.
3. Virtual Threads: Handling Many Things at Once
Java 21 introduces virtual threads from Project Loom, making concurrency management more efficient. Here’s how you can create and run tasks using virtual threads:
try (var executor = Executors.newVirtualThreadExecutor()) {
executor.submit(() -> {
// Perform some asynchronous task
System.out.println("Task executed by virtual thread.");
});
}
Virtual threads allow you to handle many tasks concurrently without the overhead of traditional threads, making your applications more scalable and responsive.
4. String Templates: Making Strings More Flexible
String templates in Java 21 allow you to embed expressions directly within string literals, simplifying dynamic string construction:
String name = "Java";
int version = 21;
String message = STR."Welcome to Java \{name} version \{version}!";
System.out.println(message); // Outputs: Welcome to Java 21 version 21!
With string templates, you can easily insert variables (name
and version
) into strings without concatenation, improving readability and maintainability.
5. Foreign Function & Memory API: Talking to Other Languages
Java 21 enhances the Foreign Function & Memory API, enabling Java programs to interact with native libraries and manage off-heap memory efficiently. Here’s a simplified example of using memory segments:
try (MemorySegment segment = MemorySegment.allocateNative(1024)) {
MemoryAccess.setByteAtOffset(segment, 0, (byte) 42);
byte value = MemoryAccess.getByteAtOffset(segment, 0);
System.out.println("Value at offset 0: " + value); // Outputs: Value at offset 0: 42
}
This API provides safer and more efficient ways to work with non-Java code and manage memory resources.
6. Unnamed Patterns and Variables: Focusing on What Matters
Java 21 introduces unnamed patterns and variables, allowing you to ignore parts of data or variable declarations that aren't relevant:
record Rectangle(double width, double height) {}
Rectangle rect = new Rectangle(10.0, 5.0);
if (rect instanceof Rectangle(var _, double height)) {
System.out.println("Height of the rectangle: " + height); // Outputs: Height of the rectangle: 5.0
}
In this example, var _
ignores the width of the rectangle, focusing only on retrieving and using its height.
Subscribe to my newsletter
Read articles from Pankaj Chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
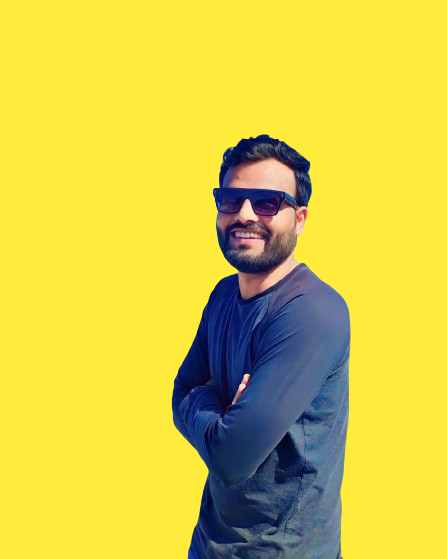
Pankaj Chaudhari
Pankaj Chaudhari
Experienced Software Engineer with over 1 year of expertise in backend development, microservices architecture, and cloud-native applications. Skilled in Java, Spring Boot, AWS, Docker, and Kubernetes. Proficient in deploying, scaling, and managing applications. Ready to contribute technical excellence to collaborative software development teams.