Understanding the Sliding Window Technique
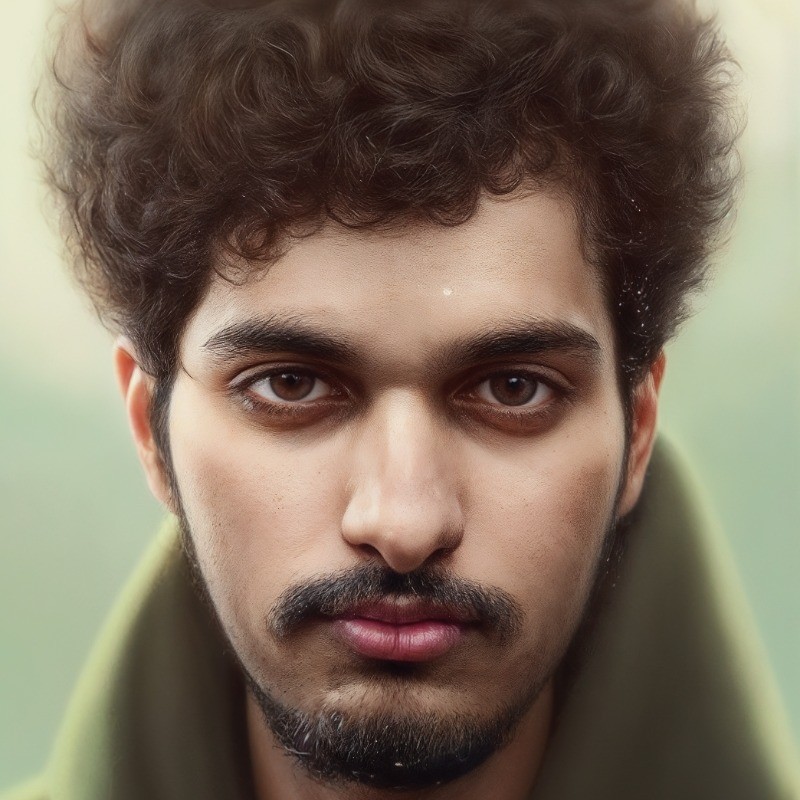
The sliding window technique is a method used to solve problems involving arrays or lists by maintaining a subset of data within a certain "window" that "slides" through the array or list. This technique is particularly useful for problems involving subarrays or substrings.
Process:
Initialization: Start by defining the initial window size and position. This could be a fixed size or dynamically adjusted based on the problem's requirements.
Window Traversal: Slide the window through the array or list, typically from the beginning to the end. This involves moving the window one element at a time or adjusting the size as needed.
Condition Check: At each step, check if the current window meets the problem's conditions. This could involve checking the sum of elements within the window, the number of unique elements, or any other criteria relevant to the problem.
Update Result: If the current window meets the conditions, update the result accordingly (e.g., update the maximum sum found and record the window's start and end positions).
Termination: Continue until the window has traversed the entire array or list.
When to Use:
Subarray Problems: When finding a subarray's maximum/minimum sum or the longest subarray with distinct elements.
Substring Problems: When you need to find the longest substring with no repeating characters or the shortest substring that contains all characters from a given set.
Consecutive Elements: When dealing with problems involving consecutive elements or sequences.
How Does It Reduce Time Complexity?
Linear Time Complexity: The sliding window approach often reduces the time complexity from O(n^2) or higher (which would be typical with nested loops) to O(n). This is because the window slides through the array or list in a single pass, and the operations within the window are typically constant time.
Efficient Subarray/Substring Checking: Instead of checking all possible subarrays or substrings (which would require multiple passes or nested loops), the sliding window efficiently checks each subarray or substring by dynamically adjusting the window size and position.
Example problem for better understanding
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
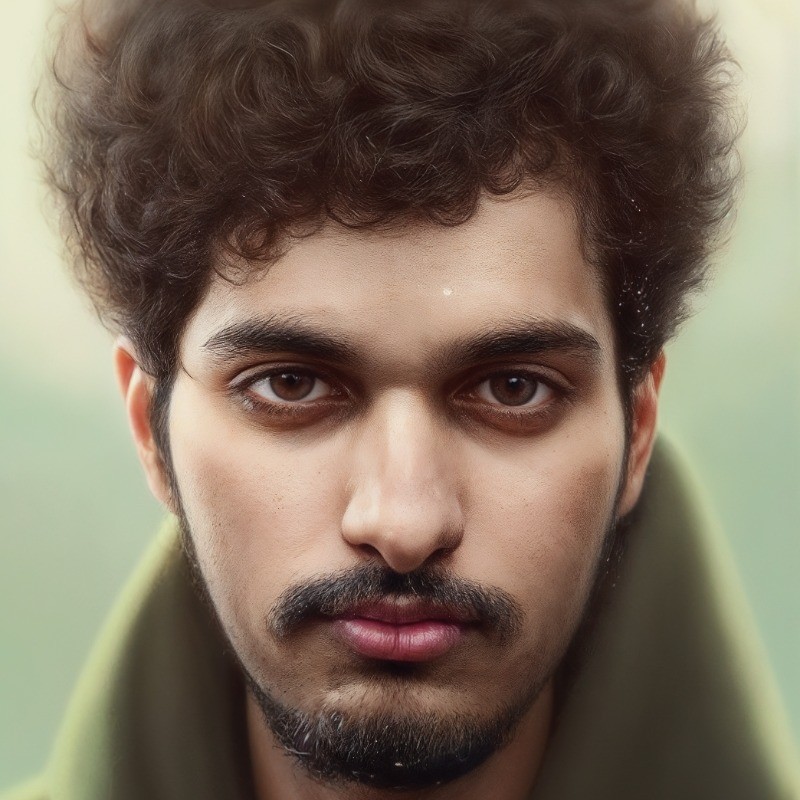
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?