How to Create Python Projects Using Poetry: A Beginner's Guide (2024 Edition)
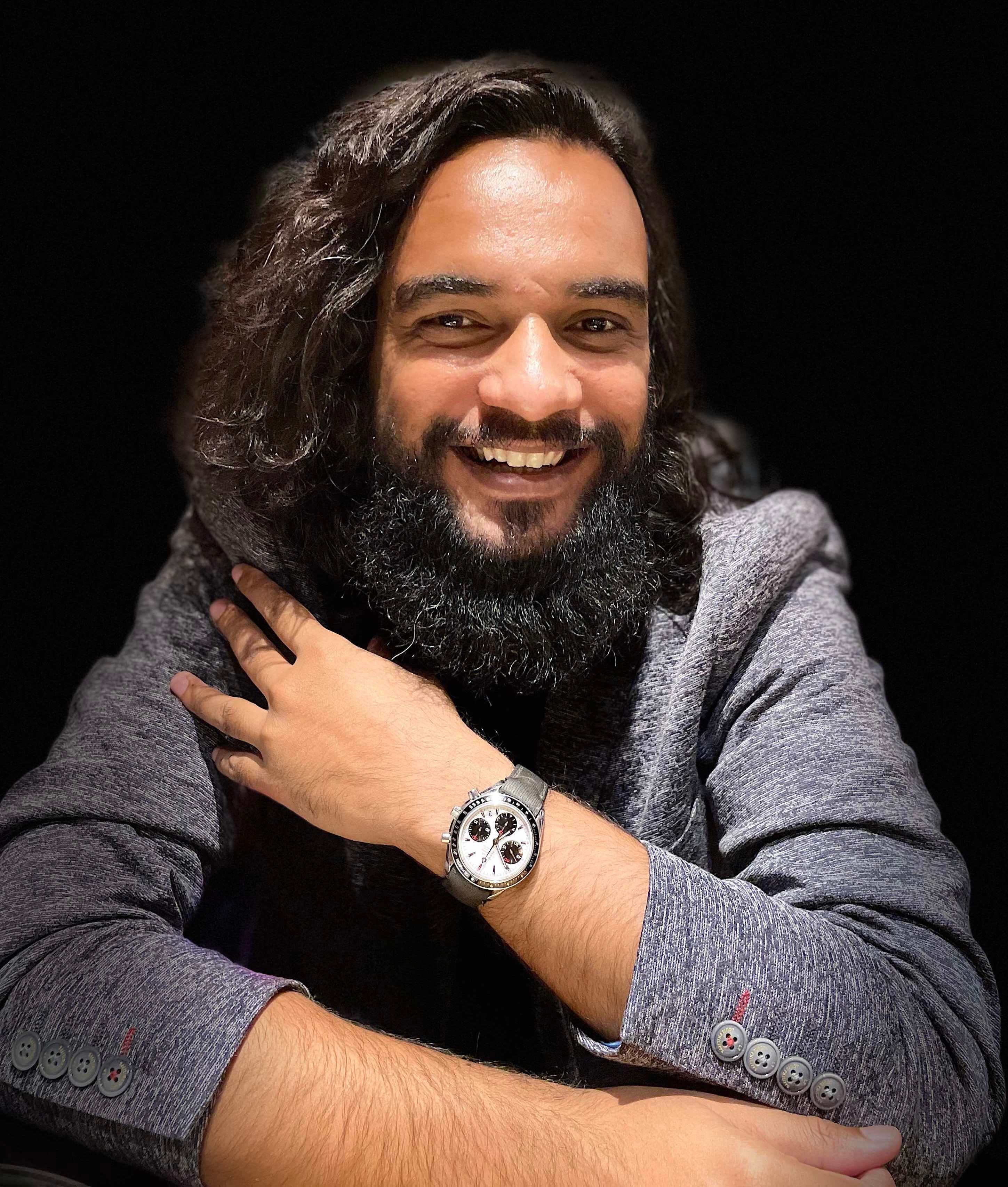
Table of contents
- Introduction
- Step 1: Installing Poetry (via Official Installer)
- Step 2a: Creating Your First Python Project with Poetry
- Step 2b: Breathing Poetry into Existing Projects
- Step 2c: Demystifying pyproject.toml
- Step 3: Poetry's Operating Modes
- Step 4: Virtual Environments Made Easy with Poetry
- Step 5: Mastering Dependency Management with Poetry
- Step 6: Dependency Groups
- Step 7: Packaging Your Project with Poetry
- Step 8: Publishing to PyPI or Private Repositories
- Conclusion
- Reference
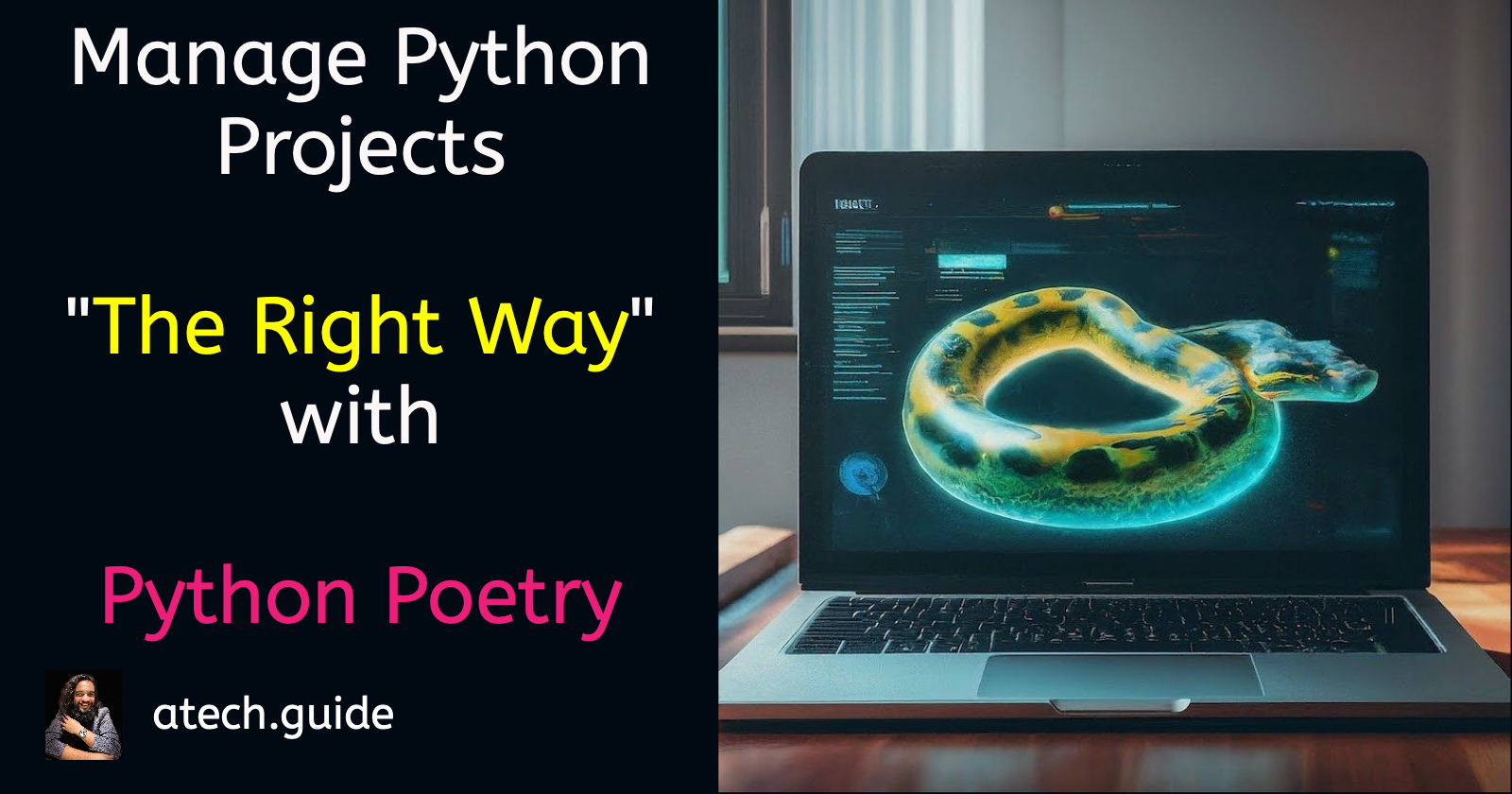
Introduction
Are you tired of wrestling with Python project dependencies, virtual environments, and the complexities of packaging?
Let's revisit the challenges that often plague Python projects:
๐ Inconsistent Environments: Have you ever run into a "works on my machine" scenario? Mismatched dependencies can cause headaches when code behaves differently across environments.
๐ Version Conflicts: The dreaded dependency hell โ when incompatible versions clash and break your code.
๐ Manual Tracking: Keeping track of every package and its version can quickly become overwhelming.
๐ Complex Packaging: Preparing your project for distribution can involve a series of manual steps.
Imagine a world where setting up your Python projects is as easy as pie.
Welcome to the world of Poetry!
Poetry addresses these issues head-on, by providing a streamlined and efficient way to handle dependencies, package projects etc making your Python projects more reliable and easier to manage.
In this beginner-friendly tutorial, we'll guide you through how to create Python projects using Poetry, step by step.
Everything you need to know.
Step 1: Installing Poetry (via Official Installer)
To embark on our Poetry journey, we need to install it.
The recommended way is via the official installer.
You can find detailed instructions on the Poetry installation page.
# For Linux, macOS, Windows (WSL)
curl -sSL https://install.python-poetry.org | python3 -
Step 2a: Creating Your First Python Project with Poetry
Once Poetry is installed, let's create a new Python project using Poetry's intuitive new
command:
poetry new my-awesome-project
Project Structure
After running the command, Poetry generates a project structure that looks like this:
my-awesome-project/
โโโ pyproject.toml
โโโ README.md
โโโ my_awesome_project/
โ โโโ __init__.py
โโโ tests/
โโโ __init__.py
pyproject.toml
: The heart of your project! This file contains project metadata, dependencies, and scripts.README.md
: A place to describe your project in markdown format.my_awesome_project/
: Your project's source code directory.tests/
: A dedicated folder for your project's tests.
Step 2b: Breathing Poetry into Existing Projects
If you already have a Python project you want to manage with Poetry, it's easy to migrate.
Navigate to your project's root directory and run:
cd pre-existing-project
poetry init
Poetry will guide you through creating a pyproject.toml
file, asking questions about your project's name, description, and dependencies.
Step 2c: Demystifying pyproject.toml
The pyproject.toml
file generated via poetry new my-awesome-project
command includes the following components:
[tool.poetry]
name = "my-awesome-project"
version = "0.1.0"
description = ""
authors = ["Kamran Ali <kamranalinitb@gmail.com>"]
readme = "README.md"
[tool.poetry.dependencies]
python = "^3.12"
[build-system]
requires = ["poetry-core"]
build-backend = "poetry.core.masonry.api"
[tool.poetry]
: Contains the main metadata for your project such asname
,version
,description
,authors
.[tool.poetry.dependencies]
: Lists all the dependencies required to run your project.[build-system]
: Specifies the build system requirements, includingrequires
andbuild-backend
which are necessary for building your project.
Step 3: Poetry's Operating Modes
Poetry offers two operating modes:
Package Mode: (default) Use this mode, if you want to package your project into an sdist or a wheel and publish it to a package index
Metadata such as name and version which are required for packaging, are mandatory.
The project itself will be installed in editable mode when running
poetry install
.
Non-package Mode: Use this mode, If you want to use Poetry only for dependency management
We can disable package mode via
[tool.poetry]
package-mode = false
Step 4: Virtual Environments Made Easy with Poetry
Poetry manages virtual environments automatically.
By default, they are created in a central location
But you can configure Poetry to create virtual environments within your project directory by setting virtualenvs.in-project
Config.
Having virtual environments within the project directory simplifies project management and ensures all project-related files are in one place.
Secondly, tools like vscode can detect the virtual environment of our project
# Enable virtualenvs.in-project via following command
poetry config virtualenvs.in-project true
# To list poetry configs
poetry config --list
Virtual Environment Commands
Command | Short Description |
poetry shell | Activates the virtual environment |
deactivate | Deactivates the virtual environment |
poetry env list | Lists all virtual environments |
External Virtual Environment Management
Poetry can also work with external virtual environments. Just activate the external virtual environment before running Poetry commands.
Step 5: Mastering Dependency Management with Poetry
Dependencies are the building blocks of any Python project. Poetry makes adding, removing, and updating them a breeze.
Adding and Removing Dependencies
To add a dependency:
poetry add <package>
To remove a dependency:
poetry remove <package>
Showing and Updating Packages
To show installed packages:
poetry show
To update packages:
poetry update
# Update specific packages
poetry update package_1 package_2
Poetry Dependency Management Commands
Command | Example | Description |
poetry add | poetry add requests | Adds a new dependency |
poetry remove | poetry remove requests | Removes a dependency |
poetry show | poetry show -t | Shows installed packages |
poetry update | poetry update | Updates all dependencies |
poetry install | poetry install | Installs dependencies defined in pyproject.toml file |
The Importance of poetry.lock
The poetry.lock
file is your project's snapshot of dependencies and their exact versions.
It ensures that everyone working on your project has the same environment preventing surprises down the road.
Step 6: Dependency Groups
Poetry introduces dependency groups, a powerful feature for organizing your project's dependencies logically based on their purpose.
You can think of dependency groups as labels associated with your dependencies
Common groups include main
and test
Creating a Test Group
poetry add --group <group> <package>
poetry add --group test pytest
The above command will create following section in pyproject.toml
[tool.poetry.group.test.dependencies]
pytest = "^8.2.2"
tool.poetry.dependencies
are part of an implicit main
group.Creating Optional Groups
Optional groups let you define dependencies that are not always required
To create an optional group:
[tool.poetry.group.docs] # Marking "docs" group as Optional
optional = true
[tool.poetry.group.docs.dependencies]
mkdocs = "*"
Flags | Example | Description |
--with | poetry install --with docs | Optional groups can be installed in addition to the default dependencies by using the --with option of the install command. |
--without | poetry install --without test,docs | To exclude one or more groups we can use --without option |
--only | poetry install --only docs | If we want to install only specific groups of dependencies without installing the default set of dependencies use the --only option. |
Step 7: Packaging Your Project with Poetry
Ready to share your masterpiece with the world?
Poetry makes packaging a breeze
To build your project:
poetry build
This command creates both a source distribution (.tar.gz
) and a wheel (.whl
) in the dist/
directory, ready for installation.
To create only wheel, use following command
poetry build -f wheel
Step 8: Publishing to PyPI or Private Repositories
Poetry streamlines publishing your package to the Python Package Index (PyPI):
To publish your package:
poetry publish
To publish to a private repository, you need to configure the repository URL:
poetry config repositories.<repo-name> <repo-url>
Then publish using:
poetry publish -r <repo-name>
Conclusion
Python Poetry empowers you to create, manage, and share your Python projects with confidence.
Say goodbye to dependency nightmares and hello to a streamlined development workflow.
From installation to publication, Poetry's intuitive interface and powerful features make it an indispensable tool for beginners and experienced developers alike.
So, why not give Poetry a try for your next Python adventure? You might just find yourself wondering how you ever managed without it.
Are you ready to start your next Python project with Poetry?
Reference
https://python-poetry.org/docs/basic-usage/
https://python-poetry.org/docs/managing-dependencies/
https://python-poetry.org/docs/libraries/
Subscribe to my newsletter
Read articles from Kamran Ali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
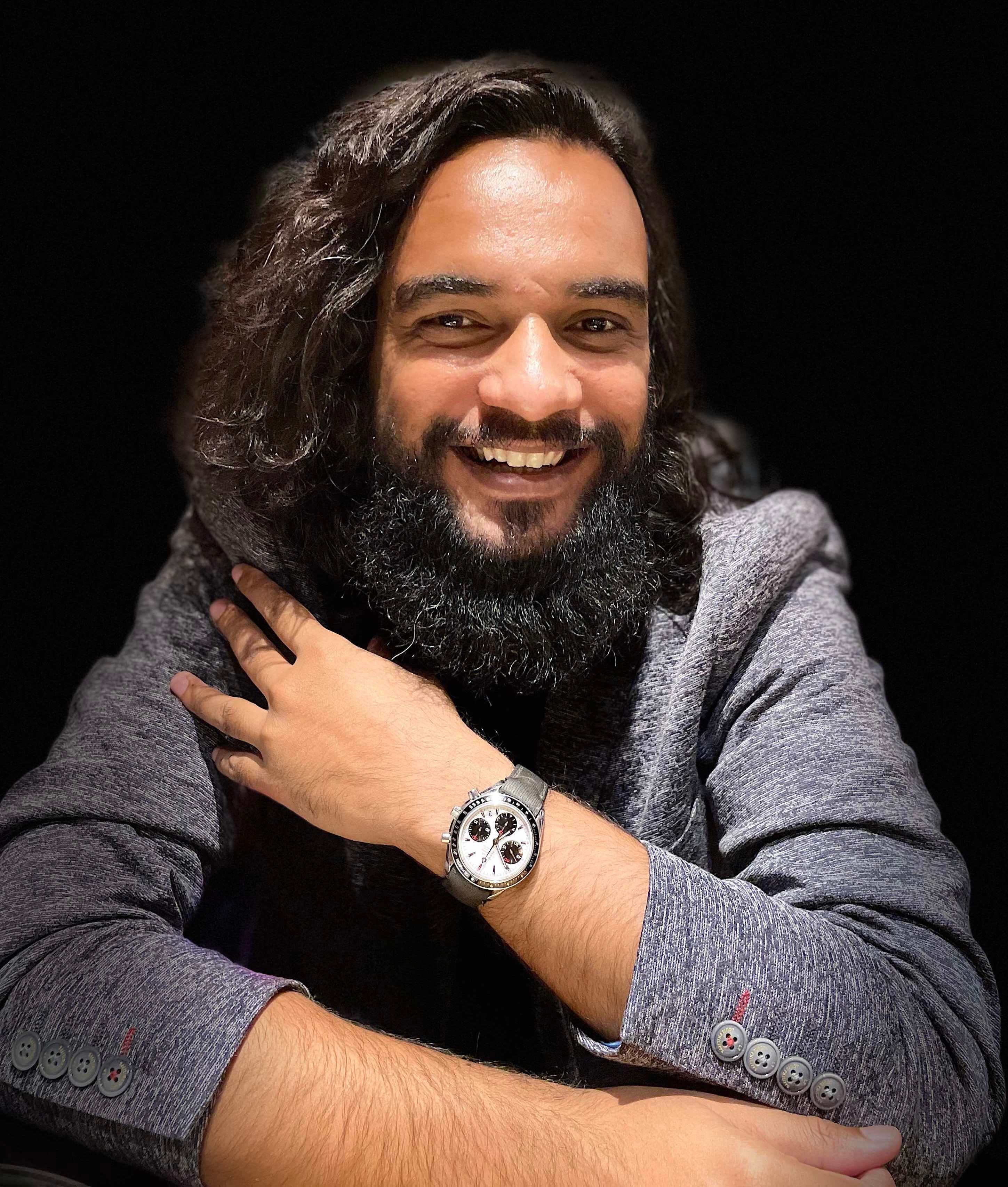
Kamran Ali
Kamran Ali
Hi, I'm Kamran Ali. I have ~11.5 years of experience in Designing and Building Transactional / Analytical Systems. I'm actually getting paid while pursuing my Hobby and its already been a decade. In my next decade, I'm looking forward to guide and mentor engineers with experience and resources