How to Approach a Coding Problem(DSA)

Table of contents
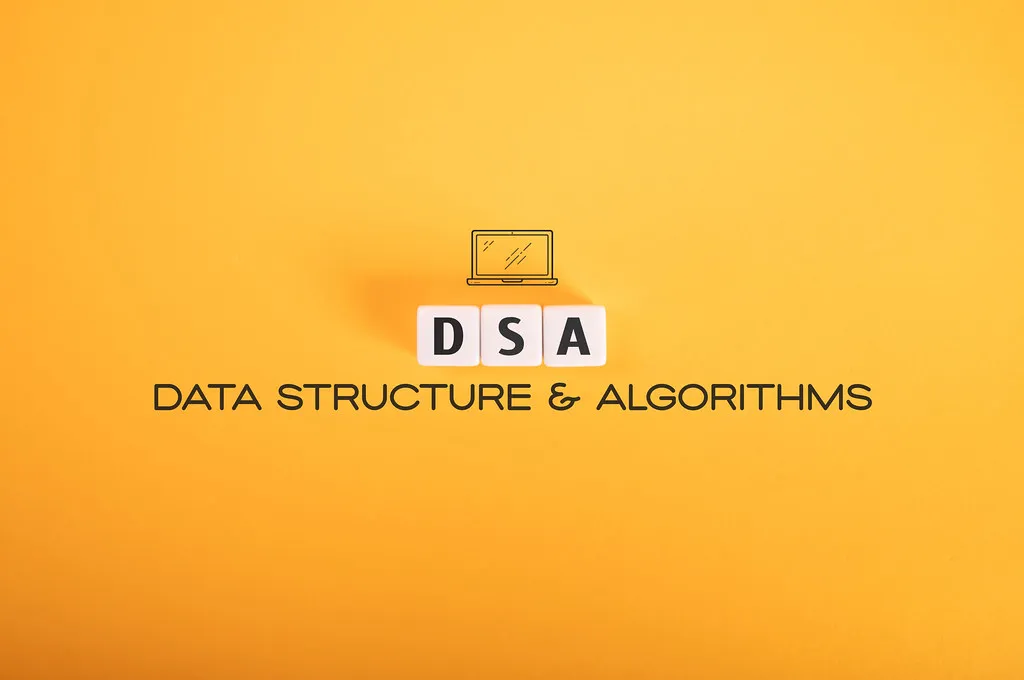
Solving Data Structures and Algorithms (DSA) problems can be challenging. This guide aims to help you solve these problems and understand them deeply. Solving problems is not just about finding a solution but developing a problem-solving mindset that strengthens your cognitive abilities, preparing you to tackle complex challenges in the future, both in coding and life.
Steps to Follow When Solving a Problem:
Understand the Question:
Read the question 2-3 times.
Ensure you understand it completely.
Write down what is given (input) and what is needed (output).
Ask yourself:
Did you understand the problem fully?
Can you explain this question to someone else?
What inputs are required?
What would be the output for those inputs?
Do you need to break down the problem into smaller parts?
Do you have enough information to solve the problem?
Estimate the Required Complexity:
Look at the constraints and time limits.
This gives you an idea of the expected time and space complexity.
Use this step to eliminate solutions that won't meet the constraints.
Find Edge Cases:
Test your solution with sample input and output provided.
Consider boundary cases that might need special handling.
Write down the edge cases that your solution should work on.
Take some sample inputs and analyze the outputs to understand the problem better.
Find a Brute-Force Solution:
Consider a straightforward approach that exhaustively checks all possible solutions.
Ensure the brute-force solution works, even if it's not efficient.
This method is useful for small-scale problems or verifying more optimized solutions.
Break Down the Problem:
Create a flowchart or UML diagram.
Divide the problem into smaller sub-problems.
Solve each sub-problem independently.
Connect the solutions of sub-problems as needed.
Use classes and objects for real-world problems where necessary.
Optimize Your Code:
Look back and analyze your solution.
Find more efficient or alternative solutions.
Use techniques like dynamic programming, greedy algorithms, divide and conquer, backtracking, or efficient data structures.
Ask yourself:
Does this code handle all possible inputs, including edge cases?
Is there a more efficient solution?
How can the code be made more readable?
Can you remove any redundant steps or functions?
Is there repetition in your code?
Dry-Run Your Solution:
Manually go through the algorithm with sample inputs.
Verify that the output is correct.
Ensure the algorithm handles all inputs, including edge cases.
Write down the sample inputs and expected outputs.
Compare the algorithm's output with the expected output.
Code & Test It On Edge Cases:
Write the code for the algorithm.
Include necessary data structures and methods.
Test the code with sample inputs and edge cases used during the dry-run.
Check for unexpected behavior or errors.
Test with additional test cases to ensure correctness and robustness.
Submit Your Solution:
After coding and testing, submit your solution for review.
The submission process varies by platform but usually involves submitting the code and any documentation.
If the solution is incorrect, debug and fix it.
Use debugging tools, print statements, or logging to find the problem.
Make necessary changes and test again.
Once the solution is correct, submit it again or move on to other problems.
Conclusion
Solving DSA problems is about developing a problem-solving mindset. By following these steps, you can approach any coding problem methodically and effectively. This process not only prepares you for job interviews and competitive programming but also enhances your cognitive skills, making you better equipped to handle complex challenges in the future.
Subscribe to my newsletter
Read articles from Riyaz Nabiyulla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Riyaz Nabiyulla
Riyaz Nabiyulla
Tech enthusiast and full-time worker ๐ Learning DSA in Java to unlock new coding adventures! โ Passionate about solving puzzles and embracing continuous learning. Let's connect and build a brighter tech future together!