Integration: TensorFlow Neural Network and Free Style Libre 2

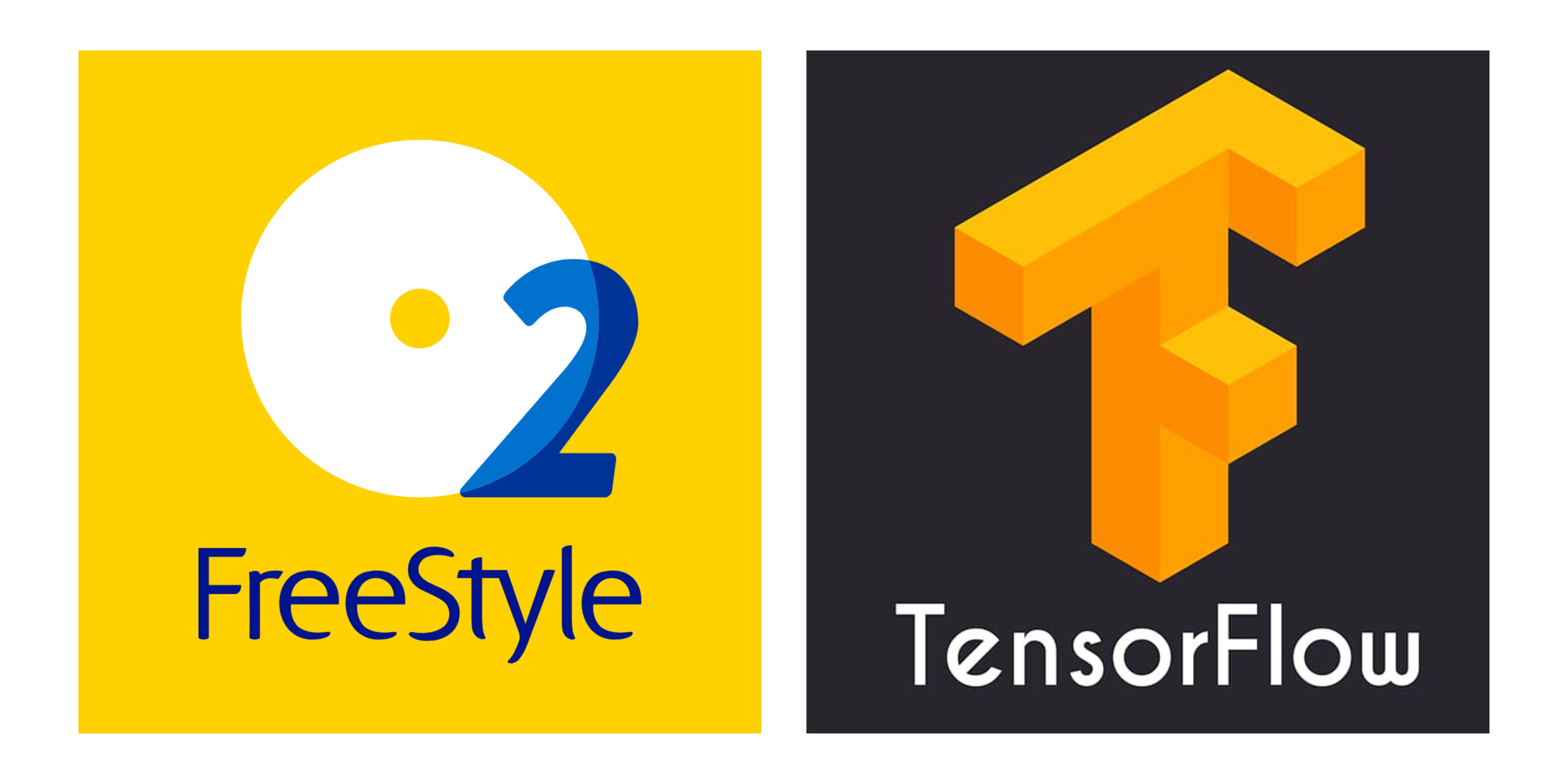
Managing a workflow that integrates data from TensorFlow's Neural Network Playground and the Freestyle Libre 2 - US app to observe the cognition of a patient with Type 2 diabetes involves several steps. Here's a structured approach to achieve this integration and observation:
1. Collect Data
A. Freestyle Libre 2 - US App Data
Download the Data: Use the Freestyle Libre 2 app to download glucose monitoring data. The app typically allows export of data in CSV format.
Data Fields: This data usually includes timestamp, glucose level, and other relevant metadata.
B. Cognitive Function Data
Design Cognitive Tests: Use standardized cognitive function tests to assess the patient's cognition. This can include memory tests, attention tests, and executive function tests.
Collect Results: Record the results of these tests along with timestamps.
C. TensorFlow's Neural Network Playground Data
Define Inputs and Outputs: Set up the playground with inputs relevant to glucose levels and cognitive function results.
Generate Synthetic Data (if needed): Use the playground to simulate scenarios and understand how changes in glucose levels could affect cognition.
2. Preprocess Data
A. Normalize and Clean Data
Glucose Data: Ensure all glucose data is cleaned and normalized. Handle missing values and outliers.
Cognition Data: Ensure consistency in the format and scale of cognitive test results.
B. Align Data
- Timestamp Alignment: Align glucose data with cognitive function test results based on timestamps. Ensure both datasets have a common timeframe for analysis.
3. Integrate Data
- Merge Datasets: Merge glucose level data and cognitive function test results into a single dataset. This may involve using pandas or similar data manipulation libraries in Python.
4. Feature Engineering
Create Features: Develop features that represent the relationship between glucose levels and cognitive function. This can include:
Time-based features (e.g., average glucose level in the past hour before a cognitive test).
Variability features (e.g., standard deviation of glucose levels over a period).
Interaction features (e.g., interaction between time of day and glucose level).
5. Model Building with TensorFlow
Define the Model: Set up a neural network model using TensorFlow. Input features will include processed glucose data and any other relevant patient data.
Train the Model: Train the model using the integrated dataset. Use a portion of the data for validation.
Evaluate the Model: Assess the model's performance in predicting cognitive function based on glucose levels.
6. Analysis and Visualization
Model Interpretation: Use techniques like SHAP or LIME to interpret the model's predictions and understand the impact of glucose levels on cognitive function.
Visualization: Create visualizations to show the relationship between glucose levels and cognitive function over time. Tools like Matplotlib or Seaborn in Python can be useful.
7. Deployment and Monitoring
Deploy the Model: If real-time monitoring is desired, deploy the model in a suitable environment (e.g., a cloud service or local server).
Monitor and Update: Continuously monitor the model's performance and update it with new data to ensure accuracy.
Sample Workflow Code Snippet
Here is a sample workflow snippet in Python using Pandas for data manipulation and TensorFlow for modeling:
import pandas as pd
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
from sklearn.model_selection import train_test_split
# Load data
glucose_data = pd.read_csv('glucose_data.csv')
cognition_data = pd.read_csv('cognition_data.csv')
# Preprocess data
glucose_data['timestamp'] = pd.to_datetime(glucose_data['timestamp'])
cognition_data['timestamp'] = pd.to_datetime(cognition_data['timestamp'])
# Merge datasets
merged_data = pd.merge_asof(glucose_data.sort_values('timestamp'), cognition_data.sort_values('timestamp'), on='timestamp')
# Feature engineering
merged_data['glucose_mean'] = merged_data['glucose_level'].rolling(window=60).mean()
merged_data['glucose_std'] = merged_data['glucose_level'].rolling(window=60).std()
# Drop NaNs created by rolling window
merged_data.dropna(inplace=True)
# Define features and target
X = merged_data[['glucose_mean', 'glucose_std']]
y = merged_data['cognitive_test_result']
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Build model
model = Sequential([
Dense(64, activation='relu', input_shape=(X_train.shape[1],)),
Dense(32, activation='relu'),
Dense(1)
])
model.compile(optimizer='adam', loss='mean_squared_error')
# Train model
model.fit(X_train, y_train, epochs=50, validation_split=0.2)
# Evaluate model
loss = model.evaluate(X_test, y_test)
print(f'Test Loss: {loss}')
Summary
Integrating data from the Freestyle Libre 2 app and cognitive tests with TensorFlow involves:
Collecting and aligning the data.
Preprocessing and feature engineering.
Building and training a neural network model.
Analyzing and visualizing the results.
This approach allows you to observe and potentially predict the impact of glucose levels on cognitive function in patients with Type 2 diabetes.
Subscribe to my newsletter
Read articles from Omar M. Wazed | Callibist LLC. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Omar M. Wazed | Callibist LLC.
Omar M. Wazed | Callibist LLC.
Hello everyone, my name is Omar M. Wazed. I am a Neuroscience Student at the University of Alberta, and I aim to develop apps and software to improve the functionality of healthcare and other industries by using AI. I hope to collaborate with anyone and everyone willing to make a difference.