Single Responsibility Principle (SRP)
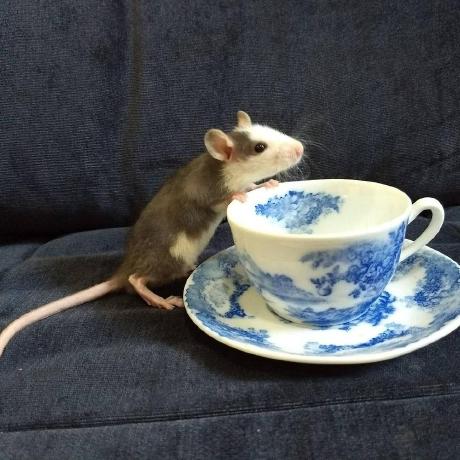
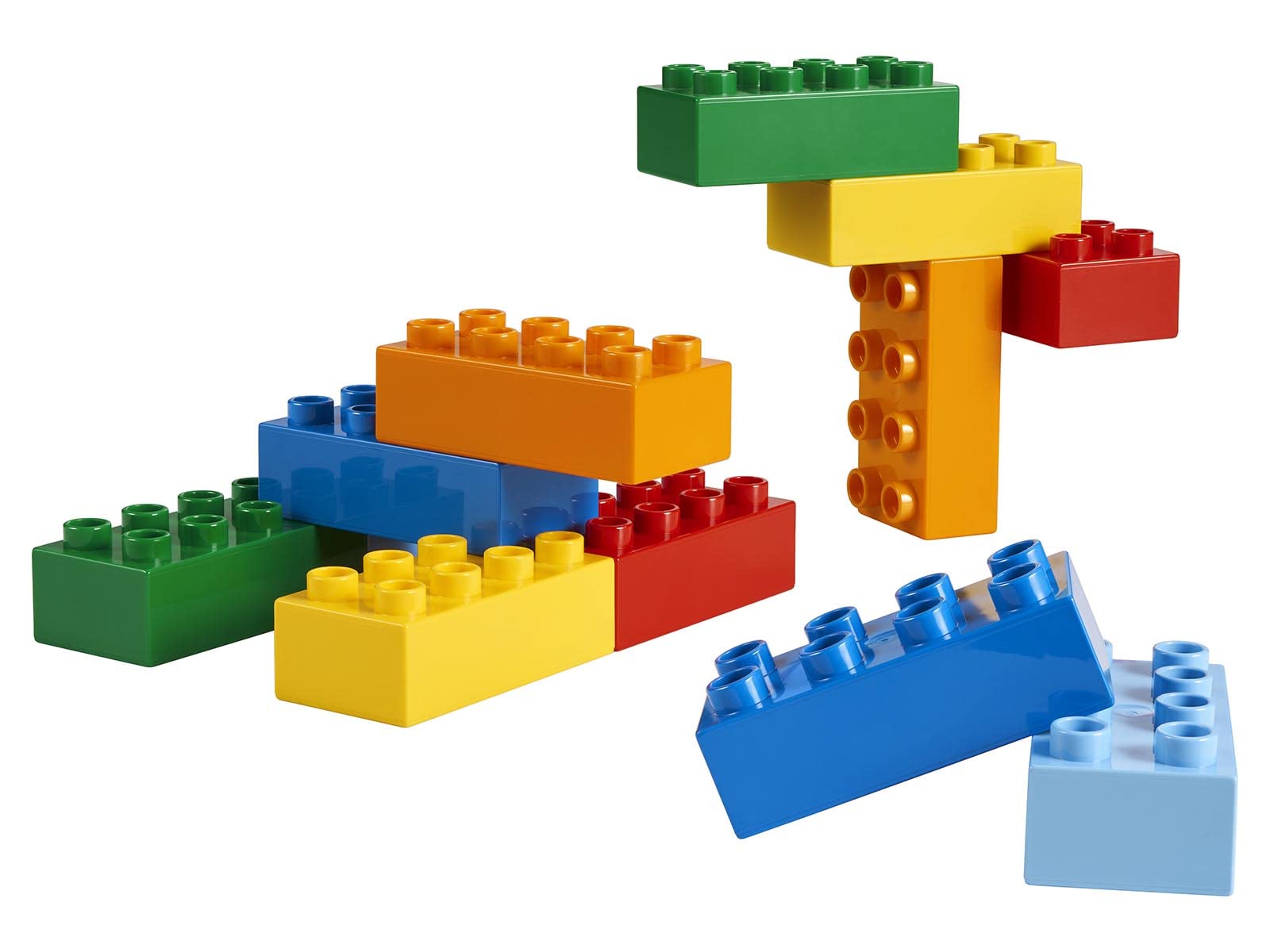
In the ever-evolving landscape of software development, maintaining clean, manageable, and scalable code is paramount. One of the foundational principles that aid in achieving this is the Single Responsibility Principle (SRP). As the first letter in the SOLID acronym, SRP is a cornerstone of object-oriented design, emphasizing the importance of having a single reason for a class to change.
But what exactly does it mean for a class to have only one responsibility? And why is it so crucial in the realm of software engineering? The concept of a "single responsibility" can be somewhat abstract, but it can be broken down into more tangible terms. A responsibility is considered a reason for change. When a class has more than one responsibility, those responsibilities become coupled. A change to one responsibility may affect the other, leading to an increase in the likelihood of bugs and a decrease in the ease of understanding and maintaining the code. For instance, consider a class that handles both the user interface logic and the data processing logic. Changes to the data processing requirements might inadvertently impact the user interface code, leading to unexpected issues. By adhering to SRP, these responsibilities would be separated into different classes, isolating the potential for unintended side effects and making the system more modular.
The benefits of SRP extend beyond just reducing bugs and improving code clarity. It also enhances the ability to reuse classes and components across different parts of an application or even in different projects. When a class has a single responsibility, it is easier to see its purpose and potential applications in other contexts. Moreover, such classes tend to be smaller and more focused, making them easier to unit test. By ensuring that each class has a distinct and clear responsibility, developers can build more robust and flexible systems that are easier to extend and modify over time. This leads to more efficient development processes and a higher overall quality of the software product.
Example
Ok, let’s dive in a practical example to see how SRP should look like.
The User Management Service
- Without SRP
public class UserManager
{
public void CreateUser(string username, string password)
{
// Validate user input
if (username == null || password == null)
{
throw new ArgumentException("Username and password cannot be null");
}
// Hash the password
var hashedPassword = HashPassword(password);
// Save user to the database
SaveUserToDatabase(username, hashedPassword);
// Send a welcome email
SendWelcomeEmail(username);
}
private string HashPassword(string password)
{
// Hashing logic here
return "hashedPassword";
}
private void SaveUserToDatabase(string username, string hashedPassword)
{
// Database save logic here
}
private void SendWelcomeEmail(string username)
{
// Email sending logic here
}
}
As we can see, the UserManager class is responsible for multiple tasks: validating user input, hashing passwords, saving user data to the database, and sending emails. This makes the class large and complex, which reduces its readability and makes it harder to understand what the class is supposed to do at a glance. Also, since UserManager class handles multiple responsibilities, any change in one responsibility might affect the others. Over time, as more changes are made, the class can become increasingly fragile and harder to maintain. Testing this class might also be a challenge, as one would need to take into accountb all the responsibilities of this class, which increases the complexity of the test.
- With SRP
public class UserValidator
{
public void Validate(string username, string password)
{
if (username == null || password == null)
{
throw new ArgumentException("Username and password cannot be null");
}
}
}
public class PasswordHasher
{
public string HashPassword(string password)
{
// Hashing logic here
return "hashedPassword";
}
}
public class UserRepository
{
public void SaveUser(string username, string hashedPassword)
{
// Database save logic here
}
}
public class EmailService
{
public void SendWelcomeEmail(string username)
{
// Email sending logic here
}
}
public class UserManager
{
private readonly UserValidator _validator;
private readonly PasswordHasher _hasher;
private readonly UserRepository _repository;
private readonly EmailService _emailService;
public UserManager()
{
_validator = new UserValidator();
_hasher = new PasswordHasher();
_repository = new UserRepository();
_emailService = new EmailService();
}
public void CreateUser(string username, string password)
{
_validator.Validate(username, password);
var hashedPassword = _hasher.HashPassword(password);
_repository.SaveUser(username, hashedPassword);
_emailService.SendWelcomeEmail(username);
}
}
Now, this version of the code looks better. Each of the UserManager responsibilities were extracted into separated classes. This makes it easier to understand and maintain each of the classes, as well as test them. Some of the classes, like the EmailServer, now can be reused someqhere else in the application if needed. The UserManager class itself becomes just, well, a manager. It is the one that knows the other classes and knows when to call them.
Identifying SRP violations
Ok, we are all now aware that SRP is super great. But how can we identify violations of this priciple in our code? I’ll list some tips that might help.
Analyze Class Responsibilities This one is kinda obvious, right? But we have to begin somewhere. Examine each class and list all the functionalities it implements. Sometimes classes can have more than one functionality, if they are closely related to each toher. We also need to evaluate cohesion here. Classes will have multiple methods, each doing something different, and that’s what’s expected of a clas. What we have to look for is lack of cohesion between these methods. A highly cohesive class has methods and attributes that are directly related to each other. Low cohesion, where methods and attributes serve disparate purposes, indicates a violation of SRP.
Check for Multiple Reasons to Change According to the SRP, a class should have only one reason to change. If you find that modifications to different aspects of your application (e.g., changes in business rules, data format, or user interface) all require changes to the same class, SRP is likely being violated. This indicates that the class has multiple responsibilities.
Monitor Class Size and Complexity Large classes with many methods and attributes often signal SRP violations. While size alone is not a definitive indicator, complexity usually accompanies size. If a class is doing too much, it becomes harder to understand and maintain. Consider refactoring large classes into smaller, more focused ones.
Consider Testing Challenges If writing unit tests for a class is difficult, it may be due to SRP violations. Classes with multiple responsibilities require more complex tests that cover all possible interactions and side effects. Simplifying classes by ensuring they adhere to SRP will result in simpler, more focused tests.
By using these strategies, developers can systematically identify and address SRP violations, leading to cleaner, more maintainable codebases.
Conclusion
I hope this text has helped you to better understand the SIngle Responsibility Pinciple (SRP), one of the fundameltal guidelines in software design. By recognizing and rectifying SRP violations—through analyzing class responsibilities, checking for multiple reasons to change, reviewing method groupings, monitoring class size and complexity, evaluating cohesion, and considering testing challenges—you will be able to significantly improve the quality of your code. Embracing SRP not only simplifies the development process but also enhances the scalability and robustness of software applications, ultimately leading to more efficient and effective coding practices.
Subscribe to my newsletter
Read articles from Lucas Guzzo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
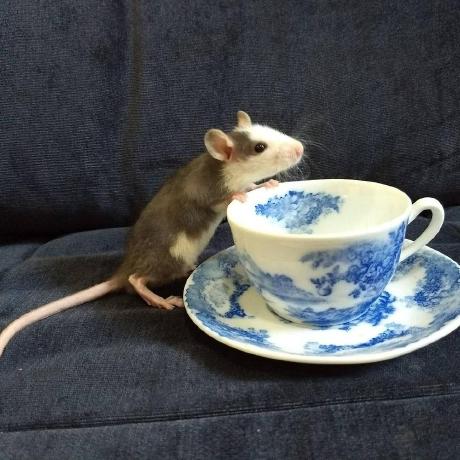