Understanding In-place Reversal of a Linked List
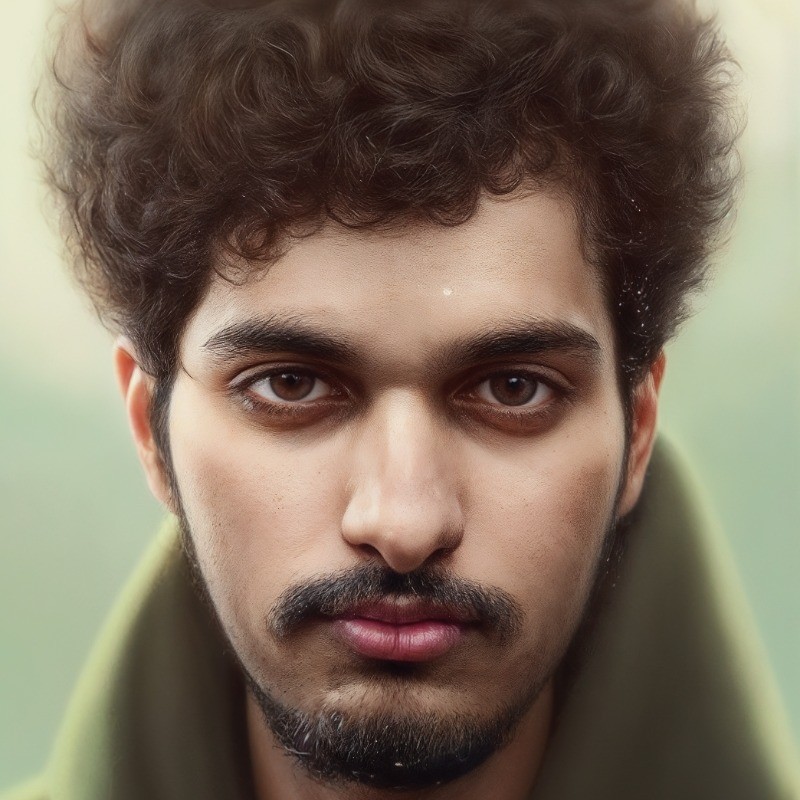
The In-place Reversal of a Linked List is a common technique for solving linked list problems. It involves reversing the linked list without using extra space, typically by manipulating the pointers of the nodes directly.
Process
Initialization: Start by initializing three pointers:
prev
,current
, andnext
. Initially,prev
is set tonull
,current
is set to the head of the linked list, andnext
is set tonull
.Traversal: Traverse the linked list using the
current
pointer. For each node, perform the following steps:Store the next node in
next
(i.e.,next = current.next
).Reverse the direction of the
current
node's pointer to point toprev
(i.e.,current.next = prev
).Move the
prev
pointer to thecurrent
node (i.e.,prev = current
).Move the
current
pointer to thenext
node (i.e.,current = next
).
Termination: The process terminates when the
current
pointer reaches the end of the linked list (i.e.,current
becomesnull
). At this point,prev
will be pointing to the new head of the reversed linked list.
When to Use
Reversing a Linked List: To reverse a linked list in-place is a common operation in many linked list problems.
Partial Reversal: To reverse a portion of a linked list, such as reversing a sublist or reversing every k nodes in a linked list.
How Does It Reduce Time Complexity?
Linear Time Complexity: The in-place reversal of a linked list has a time complexity of O(n), where n is the number of nodes in the linked list. This is because each node is visited exactly once.
Constant Space Complexity: The space complexity is O(1) since no additional space is used other than a few pointers.
Example problem for better understanding
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
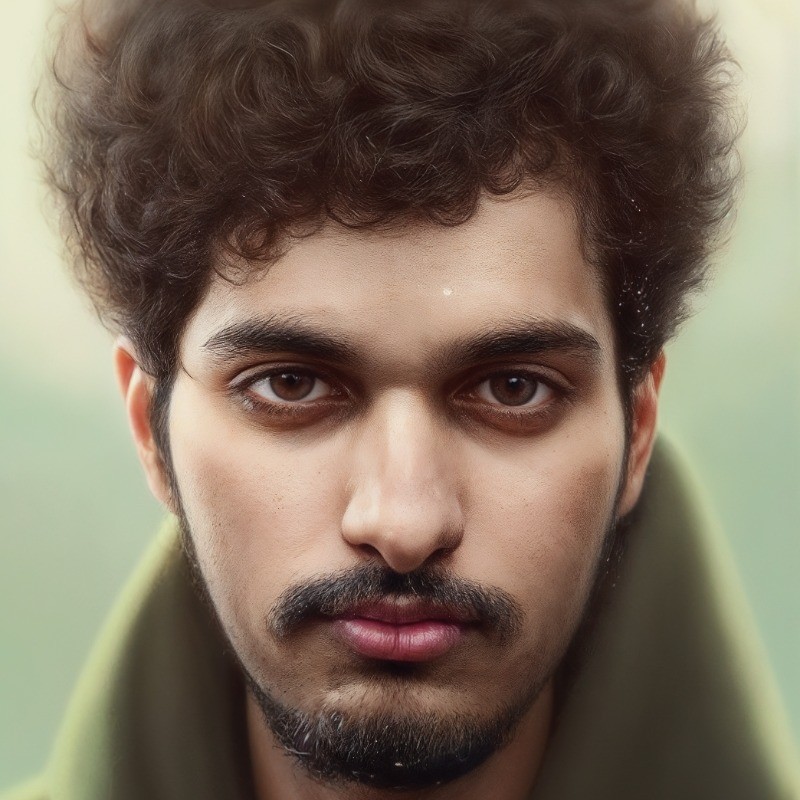
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?