React Lifecycle Methods Explained

Table of contents
- Introduction to React Lifecycle Methods
- Understanding the Component Lifecycle in React
- Overview of React Lifecycle Phases
- Detailed Look at Mounting Phase
- Exploring the Updating Phase
- Understanding the Unmounting Phase
- Special Lifecycle Methods in Error Handling
- Best Practices for Using Lifecycle Methods
- Deprecated Lifecycle Methods
- Migrating to Modern React with Hooks
- Practical Examples of Lifecycle Methods
- Common Pitfalls and How to Avoid Them
- Lifecycle Methods in Functional Components
- Debugging Lifecycle Methods
- Advanced Topics in Lifecycle Methods
- Conclusion
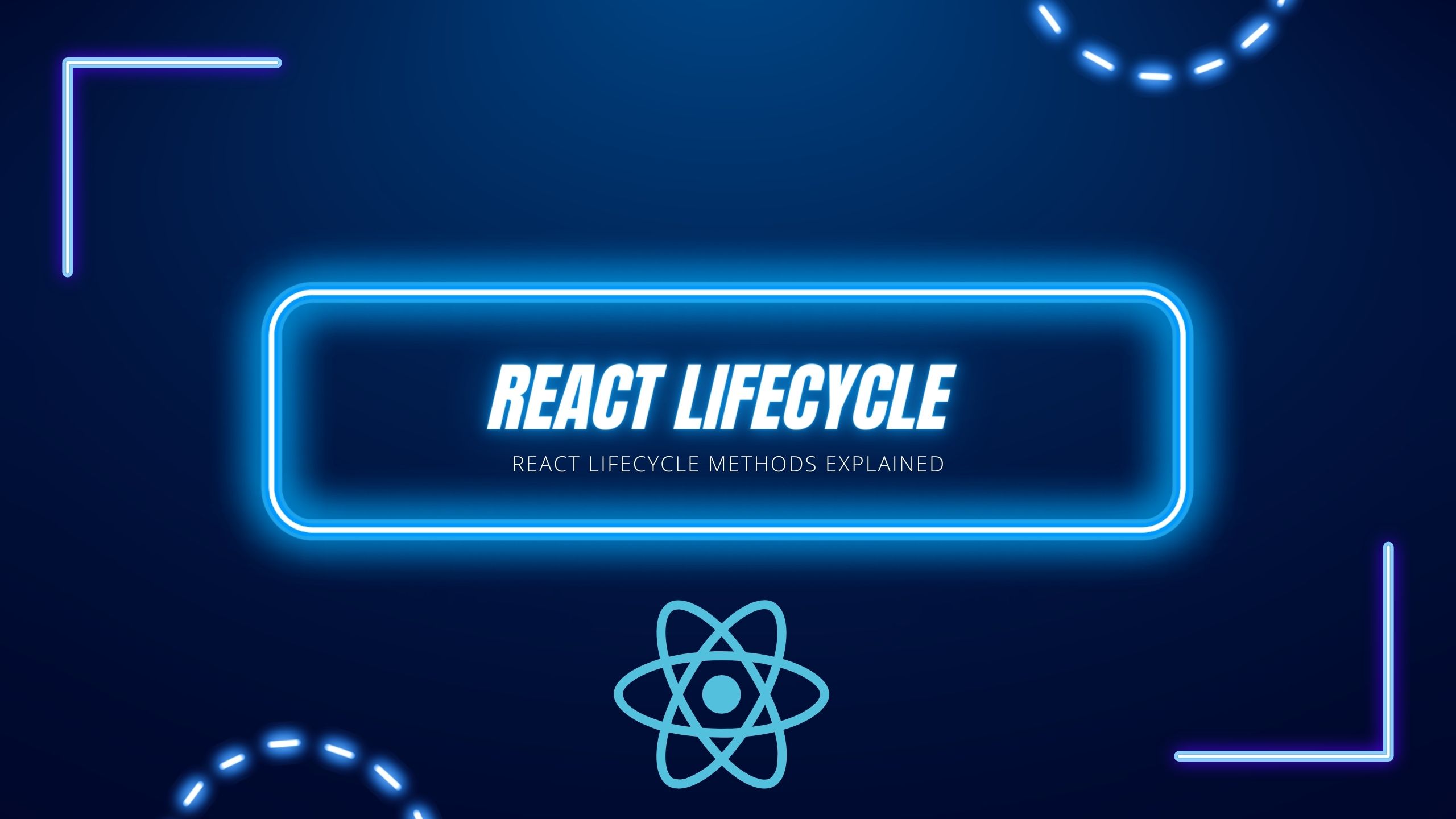
Introduction to React Lifecycle Methods
In the world of React, understanding component lifecycle methods is crucial for developing robust and efficient applications. These methods offer hooks into different stages of a component's existence, from its initial creation to its eventual removal from the DOM. By mastering these lifecycle methods, developers can optimize performance, manage state effectively, and handle side effects seamlessly.
Understanding the Component Lifecycle in React
What is a Component Lifecycle?
The component lifecycle in React refers to the sequence of events that occur from the moment a component is instantiated until it is destroyed. This lifecycle includes phases such as initialization, mounting, updating, and unmounting. Each phase provides specific methods that developers can override to execute code at particular points during the component’s life.
Importance of Lifecycle Methods in React Development
Lifecycle methods are essential for controlling and optimizing component behavior. They allow developers to perform tasks such as fetching data, managing state, interacting with external APIs, and cleaning up resources. Proper use of these methods ensures that components are efficient, responsive, and maintainable, leading to a better overall user experience.
Overview of React Lifecycle Phases
Initialization Phase
The initialization phase is where a component's state and props are first defined. During this phase, the constructor is called to set up initial state and bind event handlers.
Mounting Phase
The mounting phase occurs when a component is inserted into the DOM. This phase includes several lifecycle methods: constructor()
, static getDerivedStateFromProps()
, render()
, and componentDidMount()
.
Updating Phase
The updating phase happens when a component’s state or props change, causing it to re-render. Key methods in this phase are static getDerivedStateFromProps()
, shouldComponentUpdate()
, render()
, getSnapshotBeforeUpdate()
, and componentDidUpdate()
.
Unmounting Phase
The unmounting phase is when a component is removed from the DOM. The primary lifecycle method here is componentWillUnmount()
, used for cleanup activities.
Detailed Look at Mounting Phase
constructor(): Setting Up Initial State
The constructor
method is the first to be invoked in the mounting phase. It is used to set up initial state and bind methods. In modern React, it is also the place to set initial props via super(props)
.
static getDerivedStateFromProps(): Syncing State and Props
static getDerivedStateFromProps
is called before rendering, both on the initial mount and on subsequent updates. It allows the component to update its internal state in response to changes in props, ensuring that the state is always in sync with the prop values.
render(): Rendering UI Elements
The render
method is crucial as it returns the JSX that defines the component's UI. It is a pure method, meaning it does not modify component state and returns the same output given the same input.
componentDidMount(): Executing Post-Mount Operations
componentDidMount
is called immediately after the component is inserted into the DOM. This method is ideal for performing tasks that require a DOM node, such as initializing third-party libraries, making network requests, and setting up subscriptions.
Exploring the Updating Phase
static getDerivedStateFromProps(): Handling Props Changes
Similar to its role in the mounting phase, static getDerivedStateFromProps
is invoked when the component’s props change. It allows the component to update its state based on prop changes, providing a way to react to prop updates.
shouldComponentUpdate(): Optimizing Re-renders
shouldComponentUpdate
is a performance optimization method. It is called before rendering when new props or state are received. By returning false
, a component can prevent unnecessary re-renders, improving performance.
render(): Re-rendering UI Elements
During the updating phase, the render
method is called again to re-render the component. This ensures that the UI reflects the latest state and props.
getSnapshotBeforeUpdate(): Capturing Pre-update Information
getSnapshotBeforeUpdate
is called right before the most recently rendered output is committed to the DOM. It captures information from the DOM (e.g., scroll position) that can be used in componentDidUpdate
.
componentDidUpdate(): Reacting to Updates
componentDidUpdate
is called after the component's updates are flushed to the DOM. It is a good place to perform DOM manipulations, network requests, or other side effects that depend on the component's updated state or props.
Understanding the Unmounting Phase
componentWillUnmount(): Cleaning Up Before Unmounting
componentWillUnmount
is invoked immediately before a component is removed from the DOM. This method is essential for cleanup activities such as invalidating timers, cancelling network requests, or cleaning up subscriptions to avoid memory leaks.
Special Lifecycle Methods in Error Handling
static getDerivedStateFromError(): Catching Errors in Rendering
static getDerivedStateFromError
is used to handle errors during rendering, in lifecycle methods, and in constructors of the whole tree. It updates the state so the next render shows an error UI.
componentDidCatch(): Logging Error Information
componentDidCatch
is called after an error has been thrown by a descendant component. It can be used to log error information and display a fallback UI.
Best Practices for Using Lifecycle Methods
Avoiding Anti-patterns
Avoid using lifecycle methods for tasks better suited to other parts of React, such as manipulating the DOM directly in methods other than componentDidMount
or componentDidUpdate
. Always prefer state and props over directly modifying the DOM.
Ensuring Performance Optimization
Use shouldComponentUpdate
to prevent unnecessary re-renders. Additionally, ensure that any side effects are minimal and managed properly to avoid performance bottlenecks.
Deprecated Lifecycle Methods
componentWillMount(): Understanding Why It’s Deprecated
componentWillMount
is deprecated because its functionality can be achieved in constructor
or componentDidMount
. It was often a source of confusion and bugs.
componentWillReceiveProps(): Alternatives in Modern React
componentWillReceiveProps
is deprecated in favor of static getDerivedStateFromProps
. The latter provides a safer and more consistent way to update state in response to prop changes.
componentWillUpdate(): Using getDerivedStateFromProps and getSnapshotBeforeUpdate
componentWillUpdate
is deprecated. Its functionalities are covered by getDerivedStateFromProps
and getSnapshotBeforeUpdate
, which provide a clearer lifecycle model.
Migrating to Modern React with Hooks
Introduction to React Hooks
React Hooks, introduced in React 16.8, provide a way to use state and lifecycle methods in functional components, making code more concise and easier to understand.
Replacing Lifecycle Methods with useEffect and useState
useEffect
hook can replace many lifecycle methods. For example, use it to handle side effects like data fetching and subscriptions, effectively combining componentDidMount
, componentDidUpdate
, and componentWillUnmount
.
Practical Examples of Lifecycle Methods
Example 1: Fetching Data on Component Mount
Using componentDidMount
or useEffect
, you can fetch data when a component mounts. This ensures data is available as soon as the component is displayed.
Example 2: Implementing a Component Update Optimization
Implement shouldComponentUpdate
or use React.memo
to avoid unnecessary re-renders, optimizing performance by rendering only when relevant props or state change.
Example 3: Cleaning Up Subscriptions on Unmount
In componentWillUnmount
or the cleanup function of useEffect
, ensure subscriptions or listeners are removed to prevent memory leaks and unexpected behavior.
Common Pitfalls and How to Avoid Them
Misusing Lifecycle Methods
Avoid side effects in render
or making asynchronous calls in methods not designed for it. Stick to using methods as intended to maintain component predictability.
Ignoring Asynchronous Behavior
Always handle asynchronous operations like data fetching and DOM updates with care. Ensure that any asynchronous logic accounts for the component lifecycle to avoid issues like memory leaks or stale state.
Lifecycle Methods in Functional Components
useEffect: The Workhorse of Functional Components
useEffect
manages side effects in functional components, effectively replacing lifecycle methods. It supports both immediate execution and cleanup operations, making it versatile and powerful.
Comparing Class Component Lifecycle Methods with useEffect
While class components use multiple lifecycle methods, useEffect
consolidates these into a single, flexible hook. This reduces boilerplate and simplifies component logic.
Debugging Lifecycle Methods
Using React Developer Tools
React Developer Tools is a powerful browser extension for inspecting React components, including their state and props. It helps trace lifecycle method calls and debug issues effectively.
Logging Lifecycle Method Calls
Insert console logs in lifecycle methods to trace their execution order and diagnose issues. This simple technique can quickly highlight where things might be going wrong.
Advanced Topics in Lifecycle Methods
Context API and Lifecycle Methods
The Context API allows passing data through the component tree without prop drilling. It can be combined with lifecycle methods to manage state and side effects across deeply nested components.
Using Lifecycle Methods in Higher-Order Components
Higher-Order Components (HOCs) can leverage lifecycle methods to enhance or modify the behavior of wrapped components, providing a powerful pattern for reusability and abstraction.
Conclusion
Recap of Key Points
React lifecycle methods are foundational for managing component behavior, ensuring efficient updates, and handling side effects. With the advent of hooks, managing lifecycle events has become more streamlined and functional.
Future Trends in React Component Lifecycle Management
As React continues to evolve, hooks and other functional paradigms will likely gain prominence. Staying abreast of these changes will help developers build more efficient and maintainable applications.
Additional Resources for Further Learning
Explore the official React documentation, advanced tutorials, and community blogs to deepen your understanding of lifecycle methods and their modern counterparts. Continuous learning is key to mastering React Development.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast