Understanding Tree Breadth-First Search
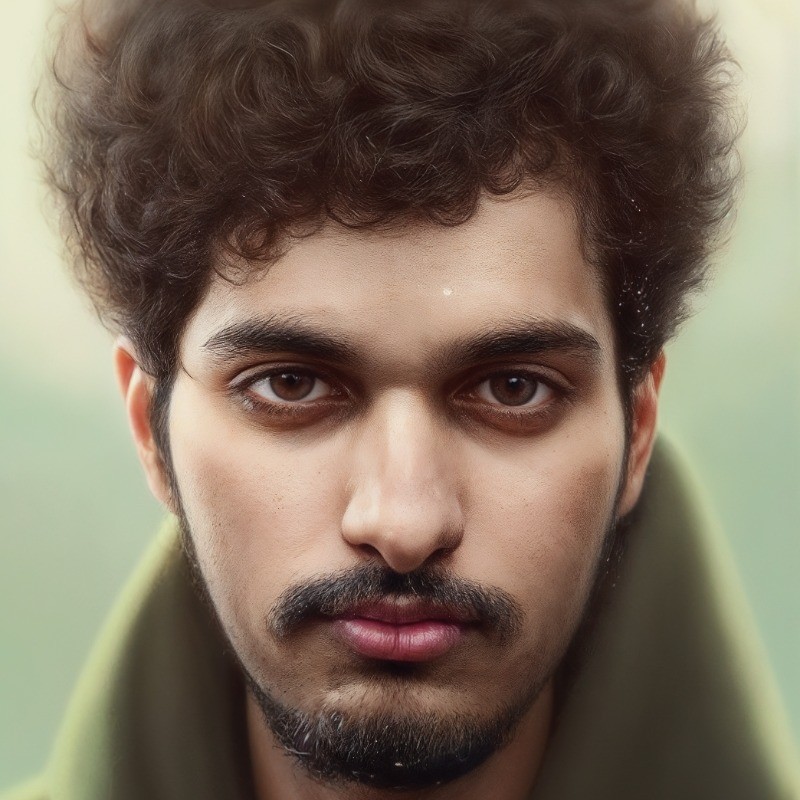
Tree BFS (Breadth-First Search) is a traversal technique used in tree data structures that explores all the nodes at the present depth level before moving on to nodes at the next depth level. This technique is often implemented using a queue data structure to keep track of the nodes to be processed.
Process
Initialization: Initialize a queue and enqueue the tree's root node.
Traversal: While the queue is not empty, perform the following steps:
Dequeue the front node from the queue.
Process the node (e.g., print its value, check a condition, etc.).
Enqueue, all the children of the dequeued node (if any).
Termination: The process terminates when the queue becomes empty, indicating that all nodes have been processed.
When to Use
Level Order Traversal: To print nodes level by level from left to right.
Finding the Shortest Path: In trees, BFS can be used to find the shortest path from the root to a specific node.
Finding the Width of the Tree: To determine the maximum width of the tree at any level.
Time Complexity
The BFS traversal has a time complexity of O(n), where n is the number of nodes in the tree. This is because each node is processed exactly once.
Example problem for better understanding
Binary Tree Level Order Traversal
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
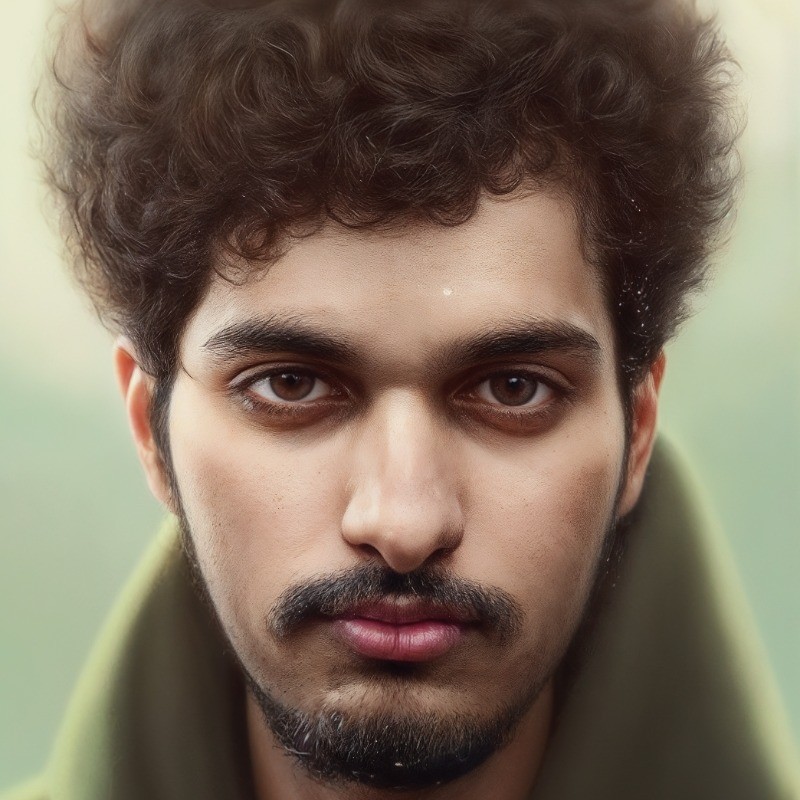
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?