Understanding Tree Depth-First Search
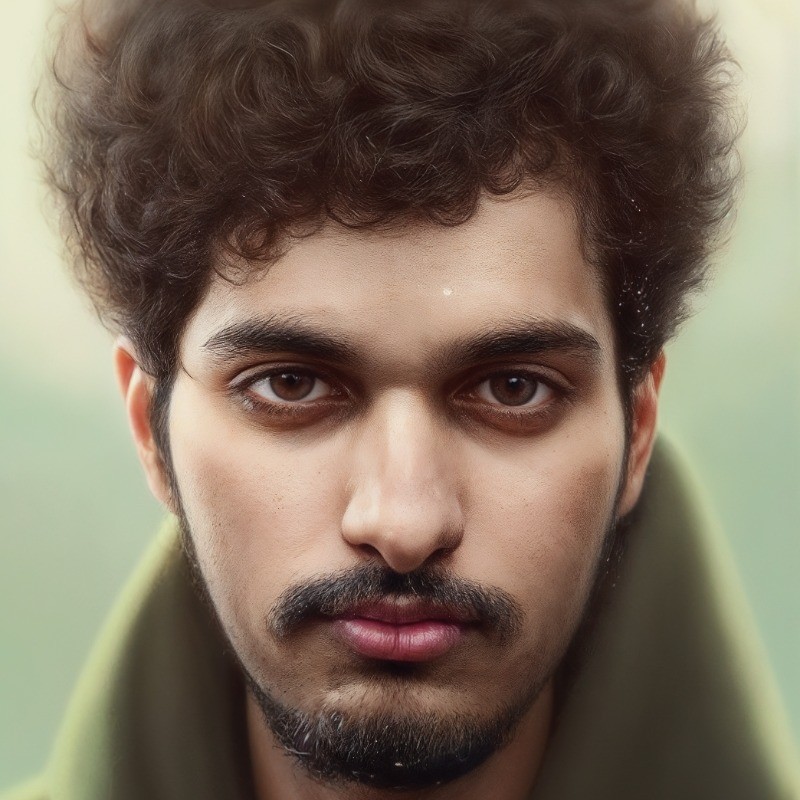
Tree DFS (Depth-First Search) is a traversal technique used in tree data structures that explores each branch as far as possible before backtracking. This technique can be implemented using recursion or an explicit stack data structure. There are three common variations of DFS for trees: Preorder, Inorder, and Postorder traversal.
Process
Initialization: Start by initializing a stack (for an iterative approach) or using recursion.
Traversal: Depending on the type of DFS traversal (Preorder, Inorder, or Postorder), perform the following steps:
Preorder (Root, Left, Right): Process the current node, then recursively traverse the left subtree, followed by the right subtree.
Inorder (Left, Root, Right): Recursively traverse the left subtree, process the current node, then traverse the right subtree.
Postorder (Left, Right, Root): Recursively traverse the left subtree, then the right subtree, and finally process the current node.
Termination: The process terminates when all nodes have been processed.
When to Use
Preorder Traversal: To create a copy of the tree or to get the prefix expression of an expression tree.
Inorder Traversal: To get nodes in non-decreasing order for binary search trees.
Postorder Traversal: To delete the tree or to get the postfix expression of an expression tree.
Time Complexity
The DFS traversal has a time complexity of O(n), where n is the number of nodes in the tree. This is because each node is processed exactly once.
Example problem for better understanding
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
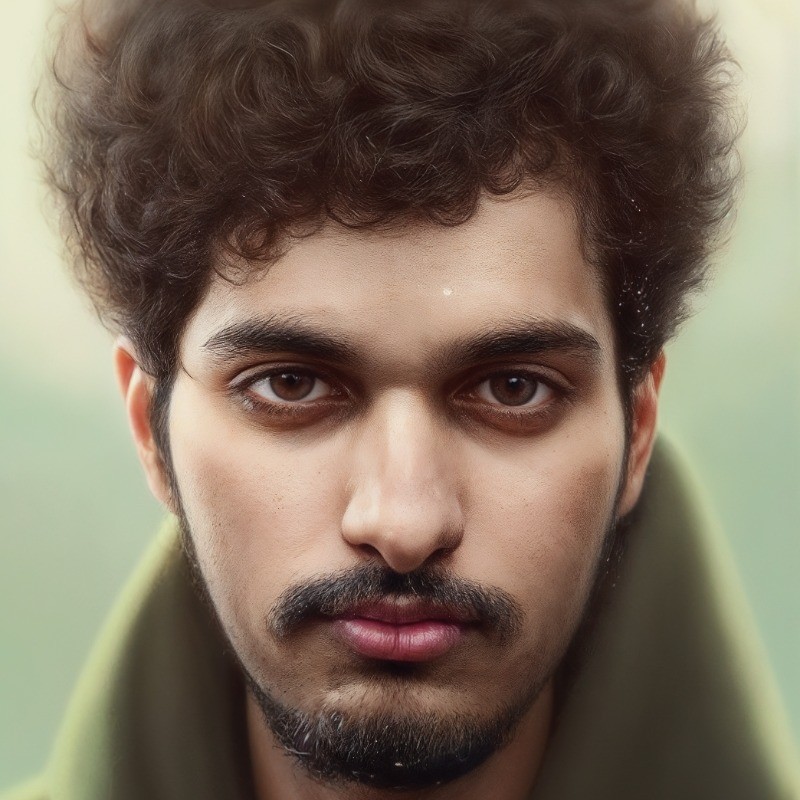
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?