Hooks: Simplifying React Components with Function Power
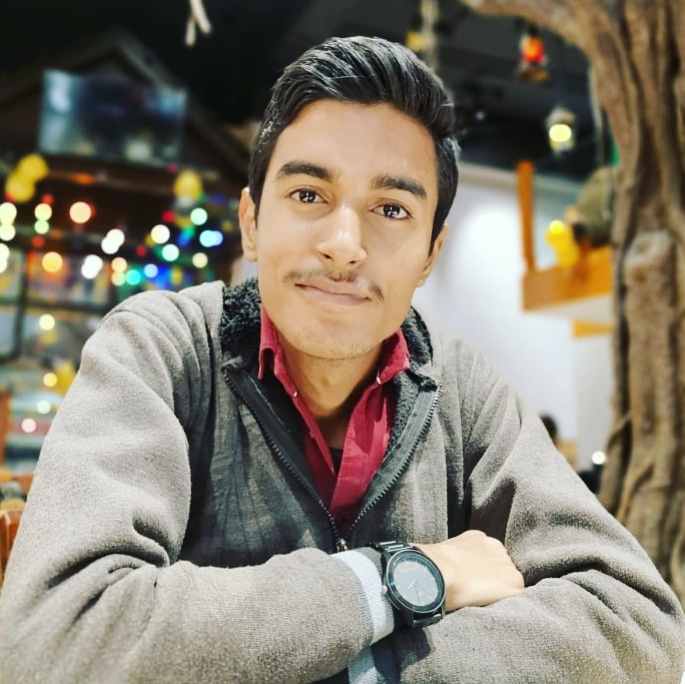
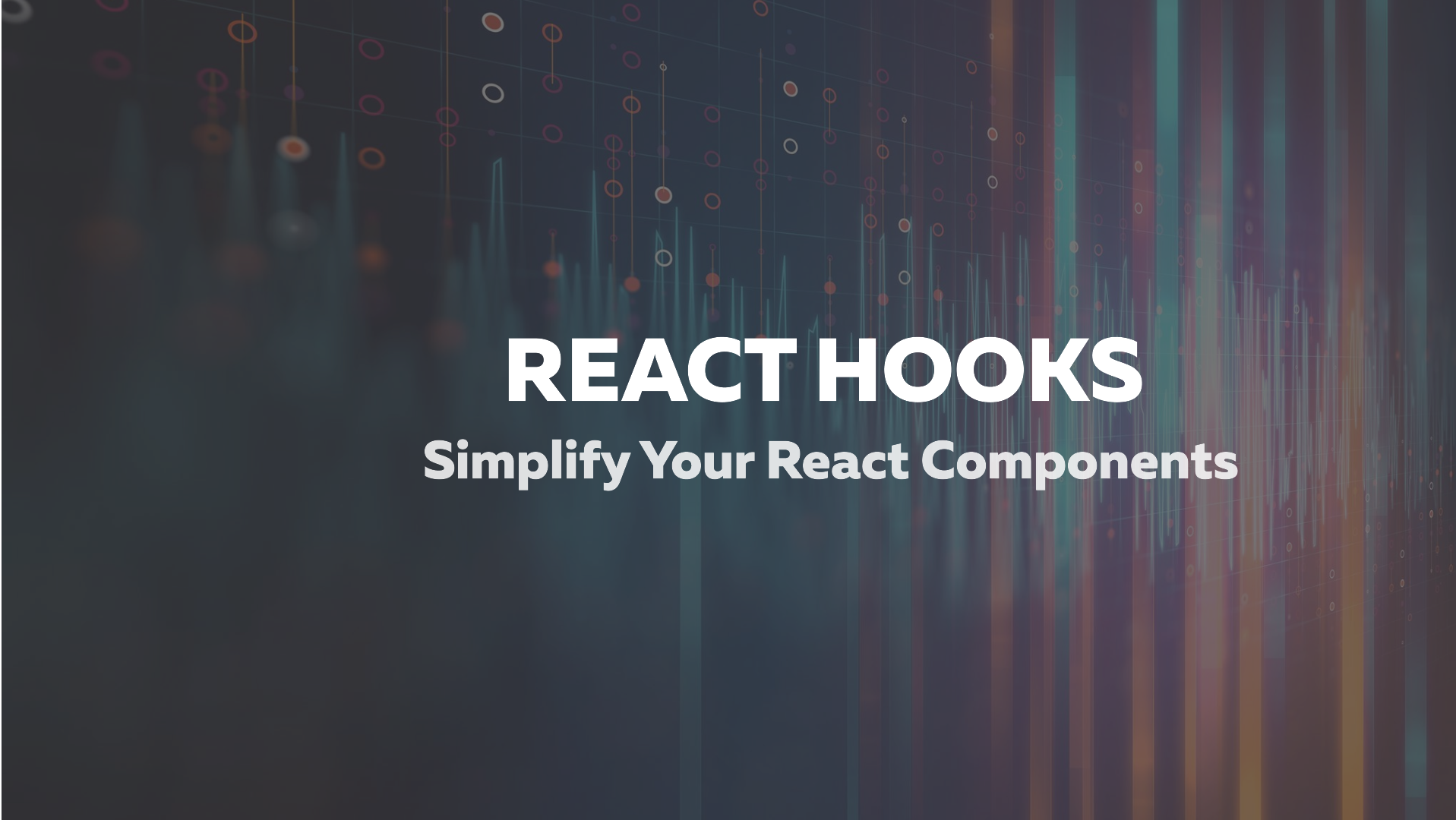
Hooks: Simplifying React Components with Function Power
For years, React developers relied on class components to manage state and side effects. While this approach worked, it often led to complex code, especially in components with intricate logic. React Hooks, introduced in React 16.8, offer a powerful alternative, allowing you to write cleaner and more maintainable code using functional components.
Why Hooks?
Traditionally, using state and side effects in React required class components. This meant managing lifecycle methods like componentDidMount
and componentDidUpdate
to handle data fetching and subscriptions. Hooks streamline this process by providing functions you can call directly within your functional components.
Here are some key benefits of using Hooks:
Improved Readability: Functional components with Hooks are generally easier to understand due to their concise syntax.
Better Reusability: Hooks promote code reusability, as logic can be extracted into custom Hooks usable across different components.
Reduced Boilerplate: Hooks eliminate the need for lifecycle methods and complex class structures, leading to cleaner code.
Common Hooks for Simplified React
Let's delve into some of the most commonly used Hooks and explore how they simplify React development:
- useState: This Hook is the foundation for managing state within functional components. It returns an array containing the current state value and a function to update it.
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
- useEffect: This Hook handles side effects in functional components. It takes a callback function and an optional dependency array. The callback runs after the component renders and whenever a dependency in the array changes.
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('https://api.example.com/users')
.then(response => response.json())
.then(data => setUsers(data));
}, []); // Empty dependency array ensures data is fetched only once
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
- useContext: This Hook provides a way to access React Context from functional components. It's useful for sharing data across deeply nested components without prop drilling.
const ThemeContext = React.createContext('light');
function ThemeButton() {
const theme = useContext(ThemeContext);
return (
<button style={{ backgroundColor: theme === 'light' ? 'white' : 'black' }}>
Click Me
</button>
);
}
These are just a few examples of how Hooks can simplify your React code. With more Hooks available (useReducer, useCallback, etc.), you can tackle complex functionalities while keeping your components clean and maintainable.
Beyond the Basics
Hooks open doors for creating custom Hooks that encapsulate reusable logic. This promotes better code organization and makes your components more focused on UI and state management.
By embracing Hooks, you can streamline your React development process, write cleaner code, and build more maintainable user interfaces. Happy Hooking!
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
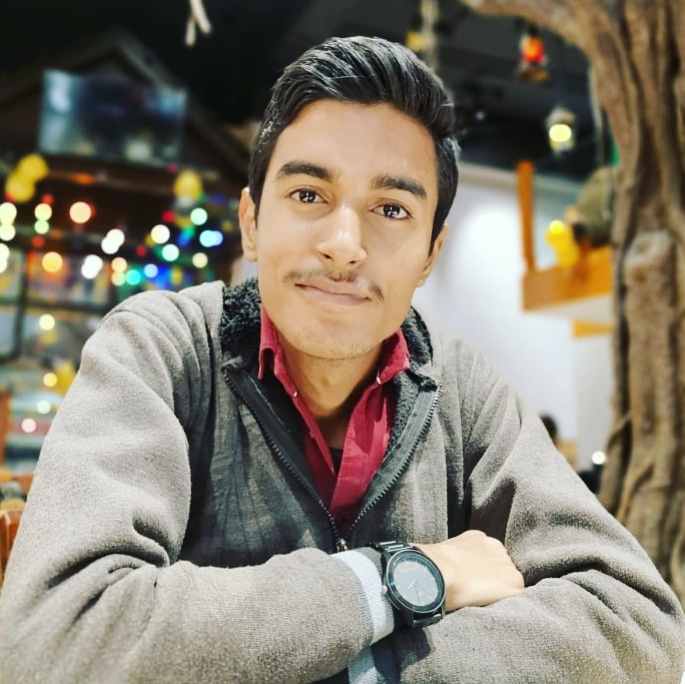
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.