Understanding the Cyclic Sort algorithm
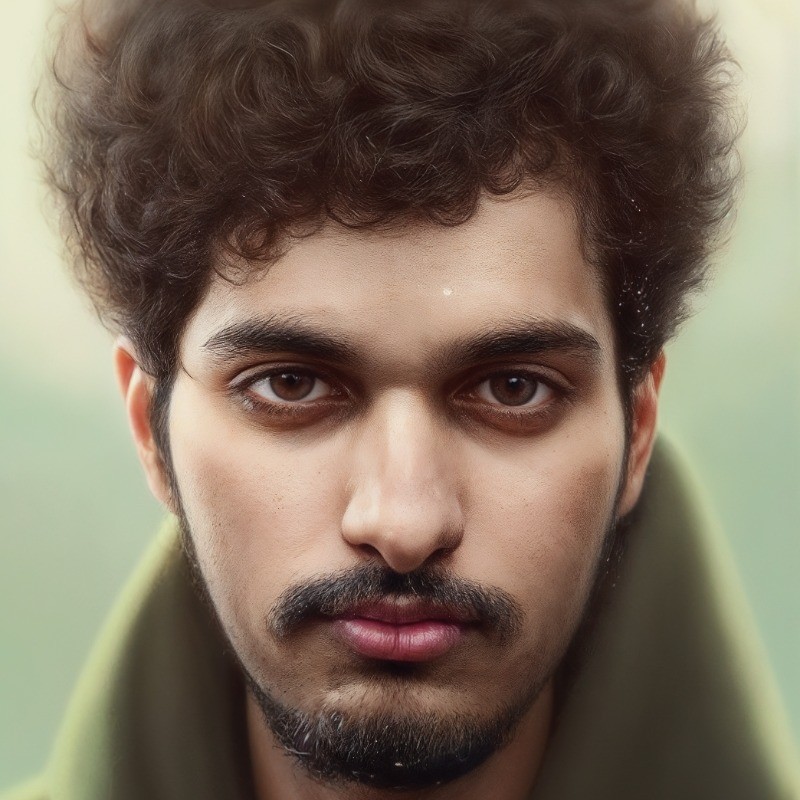
Cyclic Sort is a specialized sorting algorithm that is particularly useful for sorting arrays where each element is in the range from 1 to n (or 0 to n-1), and each element appears exactly once or is missing at most one element. The algorithm works by placing each element in its correct position in the array.
Process
Initialization: Start by iterating through the array.
Placement: For each element, check if it is already in its correct position (i.e., the element at index
i
should bei+1
for 1-based indexing ori
for 0-based indexing). Swap it with the element at its correct index if incorrectly positioned.Cyclic Swap: Continue swapping the element with the element at its correct index until the current index is in its correct position.
Iteration: Move to the next element in the array and repeat the process until all elements are in their correct positions.
Termination: The process terminates when all elements have been processed and are in their correct positions.
When to Use
Finding Missing Numbers: To find missing numbers in an array where each number from 1 to n should appear exactly once.
Finding Duplicates: To identify duplicate numbers in an array where each number from 1 to n should appear exactly once.
Sorting Permutations: To sort arrays that are permutations of the numbers from 1 to n.
How Does It Reduce Time Complexity?
Linear Time Complexity: The cyclic sort algorithm has a time complexity of O(n), where n is the number of elements in the array. This is because each element is constantly moved into its correct position and is processed at most once.
In-Place Sorting: The algorithm sorts the array in place without requiring additional space, making it space-efficient.
Example problem for better understanding
Thank you for reading!
You can support me by buying me a book.
Subscribe to my newsletter
Read articles from Vineeth Chivukula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
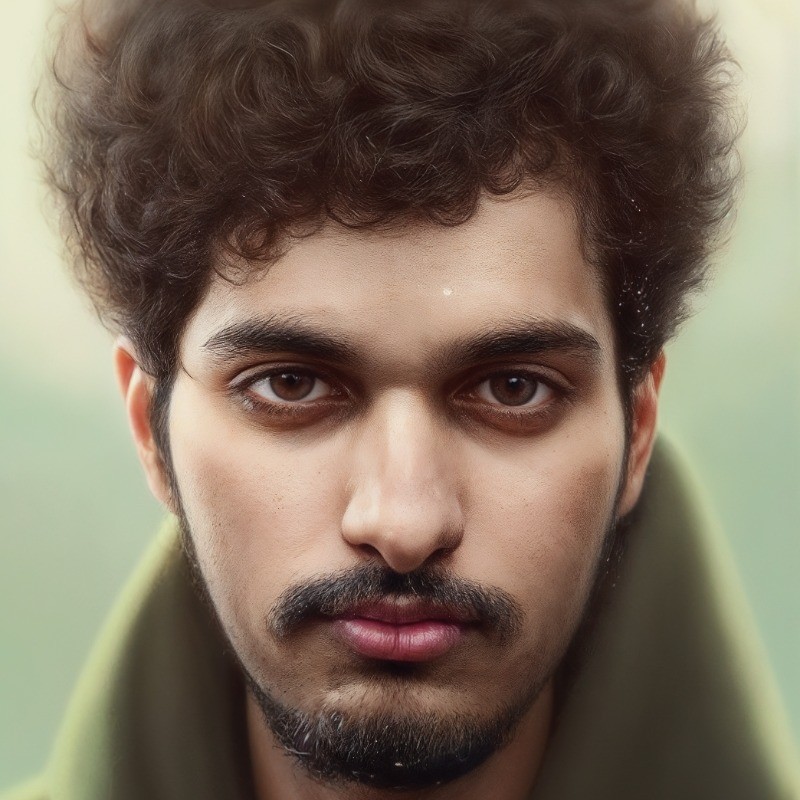
Vineeth Chivukula
Vineeth Chivukula
There's this guy who's mad about editing and programming. It's his jam, you know?