Unlocking the Secrets of Access Tokens and Refresh Tokens: A Beginner's Guide
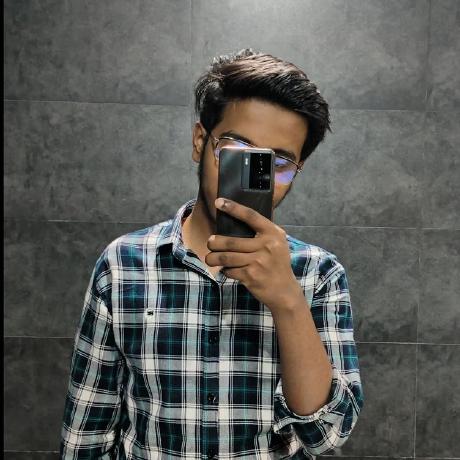
As beginner Backend or Full Stack Developers, we all encounter authentication during our learning phase, where we come across two crucial terms: 'Access Token' and 'Refresh Token'.
What exactly are they? ๐ค
What's the difference between the two? ๐ง
How are they useful? ๐คทโโ๏ธ
Let's break it down in a simple and engaging way! ๐
Why do we need these tokens anyway?
We need these tokens for authorization purposes. They make it easy for developers to implement security checks and authenticate users in our applications.
These tokens provide a seamless user experience by allowing users to register or log in once and continue using the application without repeated logins.
How does this work? Let's find out! ๐ช
Access Token - Lives fast and dies young
An access token is used for features where authentication and authorization are needed for data access.
For example:
You can see your posts directly on your social media after logging in once. You don't need to log in every time you open the app.
This is where the access token shines. It allows users to access their data without having to log in with their email or password repeatedly.
Where does this access token exist?
It exists with the user in the form of cookies in their browser and is very short-lived (dying young).
How does it authenticate the user?
Usually, this token is generated in the form of JWT (JsonWebTokens). We define a secret for the JWT signature, making each token unique. This generated JWT is then given to the user for authorization.
Here's the code in javascript which uses MongoDB for database and nodejs and expressjs for the backend:
/*suppose your user database is called userSchema
To generate the access token for each user we add the
logic as a method to the schema itself */
userSchema.methods.generateAccessToken = function(){
return jwt.sign(
{
_id:this._id,
email:this.email,
username: this.username,
fullName : this.fullName
},
process.env.ACCESS_TOKEN_SECRET,
{
expiresIn: process.env.ACCESS_TOKEN_EXPIRY
}
)
}
Why short-lived?
Access Tokens are short-lived (usually in terms of hours or a day at most), meaning they expire much sooner than refresh tokens for security reasons. This way, if an attacker tries to steal resources through the user/client side, the token will expire quickly, and access will be denied automatically. This enhances the security of our application and protects user data.
But if it expires so soon, how does the user experience seamless access to their data without re-logging in? ๐ฑ
_This is where the Refresh Token comes in_๐
Refresh Token - The Overseer
Refresh Token is the token continuosly taking care of our access token upon expiry.
Every time the access token's validity is expired, the refresh token is used to hit an endpoint which generates a new access token in place of the old one.
Where does this refresh token exist?
Refresh tokens are stored in our application's database and with the user in the form of cookies. We use these tokens to validate the user and create a new access token for them upon validation.
Because of this, the lifespan of a refresh token is much longer (usually in terms of days) compared to an access token. We need to have a refresh token every time the access token expires.
Here's the code for generating refresh token in javascript which uses MongoDB for database and nodejs and expressjs for the backend, the process is pretty much same to as what we saw above in access token:
userSchema.methods.generateRefreshToken = function(){
return jwt.sign(
{
_id:this._id,
},
process.env.REFRESH_TOKEN,
{
expiresIn: process.env.REFRESH_TOKEN_EXPIRY
}
)
}
How is a new access token generated using a refresh token?
To understand this, let's consider a scenario where a client's access token has expired, and the server responds with a 401 status code, indicating they are unauthorized.
In this situation, instead of asking the client/user to log in again, we can write some code that makes our application hit an endpoint whenever a 401 response is received. This endpoint will provide the client with a new access token.
How does this work? We send the refresh token in the request to the endpoint. The server then compares the incoming refresh token with the one stored in the database. If they match, a new access token is generated using the same method we discussed earlier and sent to the client as a cookie.
In conclusion, the client/user session is restarted upon access token expiry without requiring them to log in again with their email or password. This is because the user already has a refresh token, which we use to generate and send a new access token.
So, that's what I personally understood about access tokens and refresh tokens while learning from a resource.
This is my first blog, so there might be some mistakes or things I missed. All feedback is welcome in the comments.
***Any questions about the content are also welcome!***๐
Subscribe to my newsletter
Read articles from AYUSH KUMAR GUPTA directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
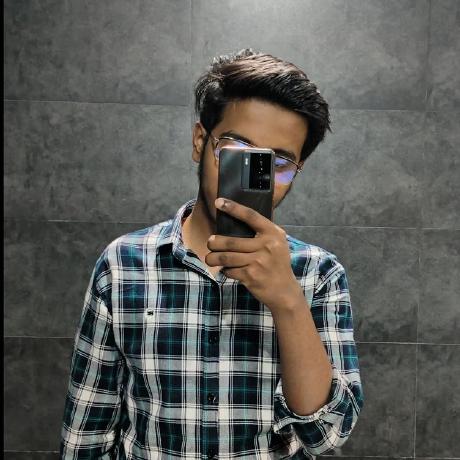
AYUSH KUMAR GUPTA
AYUSH KUMAR GUPTA
I'm a college student specializing in Full Stack Web Development, with a solid grasp of programming fundamentals and expertise in C,C++, Java, JavaScript and Python. Passionate about crafting seamless digital experiences, I'm on a quest to understand the intricacies of web development from backend to frontend. Eager to connect with individuals and organizations that share a zeal for innovation and learning in tech