Understanding Currying in JavaScript: A Friendly Introduction

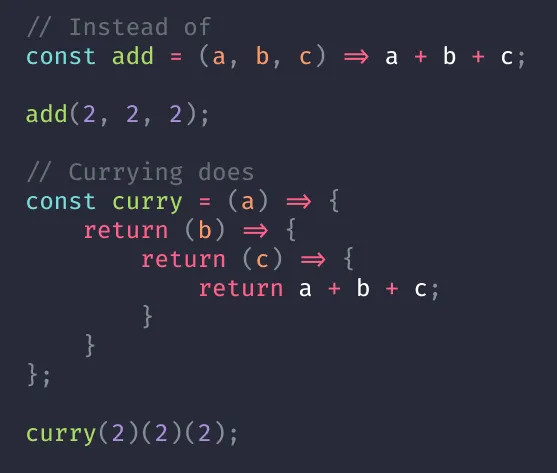
Hey there, fellow JavaScript enthusiasts! Today, let's dive into a fascinating and super useful concept in functional programming called currying. Whether you're a seasoned developer or just starting out, currying can add a new level of elegance and reusability to your code.
What Exactly is Currying?
Currying transforms a function that takes multiple arguments into a series of functions, each taking a single argument. Imagine you have a function f(a, b, c)
. Currying transforms it into f(a)(b)(c)
. Each function takes one argument and returns another function that takes the next argument, and so on.
Why Should You Care About Currying?
Reusability: Smaller functions are easier to reuse in different parts of your application.
Partial Application: Currying allows you to fix a few arguments of a function and generate a new function. Super handy!
Function Composition: It makes it easier to build complex functions by combining simpler ones.
Let's See Currying in Action
Here's a straightforward example to illustrate currying in JavaScript:
// A simple add function
function add(a, b) {
return a + b;
}
// Curried version of the add function
function curriedAdd(a) {
return function(b) {
return a + b;
};
}
// Usage
const addFive = curriedAdd(5); // Returns a function that adds 5 to its argument
console.log(addFive(10)); // Outputs 15
In this example, curriedAdd
takes an argument a
and returns a new function that takes another argument b
. When the inner function is called, it has access to both a
and b
, allowing it to return their sum.
A Simpler Way to Curry
We can make currying even easier with a simple utility function:
function curry(fn) {
return function(a) {
return function(b) {
return fn(a, b);
};
};
}
// Example usage
const add = (a, b) => a + b;
const curriedAdd = curry(add);
console.log(curriedAdd(2)(3)); // Outputs 5
In this simplified version, our curry
function transforms a function that takes two arguments into a curried version that takes one argument at a time.
A More Complex Example
Let's look at a function with three arguments and see how we can transform it into a curried version:
function sum(a, b, c) {
return a + b + c;
}
// Curried version
function curriedSum(a) {
return function(b) {
return function(c) {
return a + b + c;
};
};
}
// Usage
console.log(curriedSum(1)(2)(3)); // Outputs 6
Here, curriedSum
breaks down the sum
function into a series of nested functions, each taking one argument. You can call these functions step-by-step or store the intermediate results, like so:
const sum1 = curriedSum(1);
const sum2 = sum1(2);
const result = sum2(3);
console.log(result); // Outputs 6
Currying vs. Partial Application
Currying and partial application are related but distinct concepts. Currying transforms a function so that it takes one argument at a time, while partial application fixes a few arguments of a function and generates a new function.
For example, using partial application:
function partialSum(a) {
return function(b, c) {
return a + b + c;
};
}
const addTen = partialSum(10);
console.log(addTen(5, 3)); // Outputs 18
Advanced Currying
For more advanced currying, you can use libraries like Lodash, which provide a _.curry
function. This allows a function to be called both normally and partially:
const _ = require('lodash');
function sum(a, b) {
return a + b;
}
const curriedSum = _.curry(sum);
console.log(curriedSum(1, 2)); // Outputs 3
console.log(curriedSum(1)(2)); // Outputs 3
Wrapping Up
Currying might sound a bit academic, but it's a game-changer in writing clean, modular, and reusable JavaScript code. By breaking down functions into a series of unary (single-argument) functions, currying opens up new possibilities for partial application and function composition. Give it a try in your next project—you might just find it makes your code more elegant and fun to write.
Happy coding!
Subscribe to my newsletter
Read articles from Forhad Hossain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Forhad Hossain
Forhad Hossain
Hi, I'm Farhad Hossain, a passionate web developer on a journey to build impactful software and solve real-world problems. I share coding tips, tutorials, and my growth experiences to inspire others.