Implementing Microfrontends with React and Vue Using Single-SPA
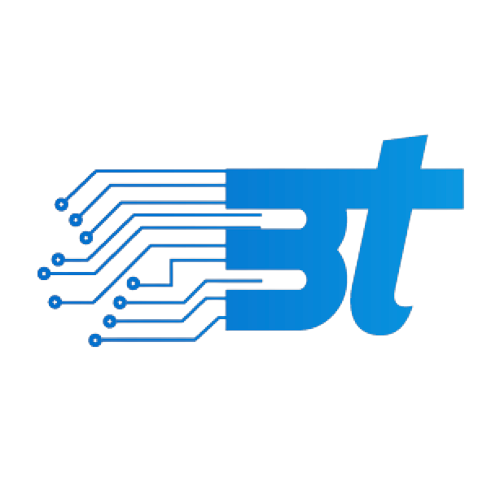
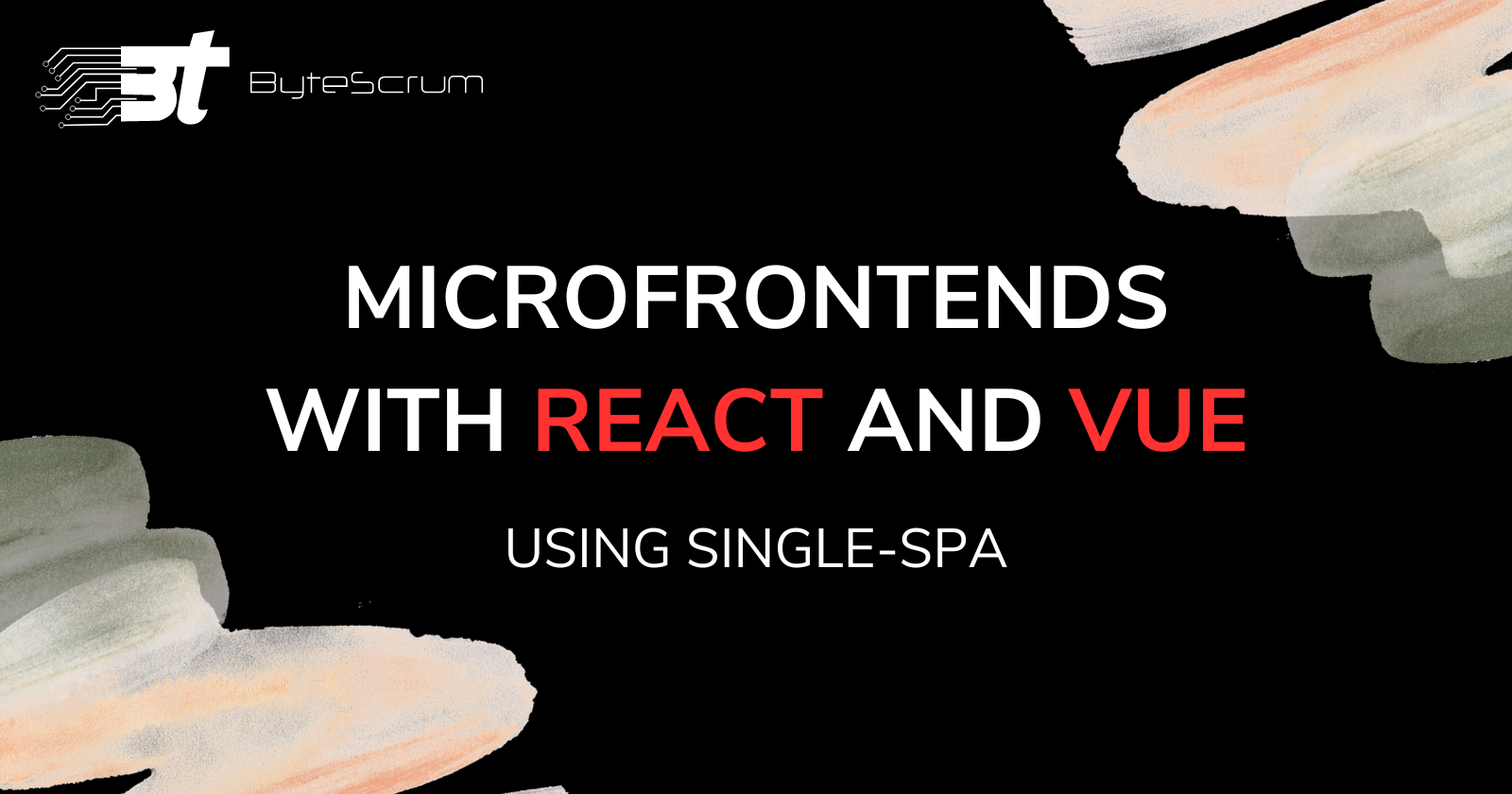
Microfrontends have revolutionized the way we build web applications by enabling multiple teams to develop, deploy, and maintain frontend components independently. Single-SPA is a framework that helps you orchestrate multiple microfrontends, even if they are built with different frameworks such as React and Vue.
In this blog, we'll walk through setting up a microfrontend architecture using Single-SPA with examples in React and Vue.
What is Single-SPA?
Single-SPA (Single Single Page Application) is a JavaScript framework for front-end microservices. It allows you to use multiple frameworks in a single-page application, which means you can have different parts of your application written in different libraries like React, Vue, Angular, etc.
Setting Up the Environment
To get started, we'll set up a basic Single-SPA environment that includes a root application and two microfrontends built with React and Vue.
Step 1: Create the Root Single-SPA Application
First, you need to set up a root configuration for Single-SPA. This root configuration will orchestrate the loading of your microfrontends.
1.1 Initialize the Project
mkdir microfrontend-root
cd microfrontend-root
npx create-single-spa
Choose
single-spa root config
when prompted.Follow the prompts to complete the setup.
1.2 Install Required Dependencies
npm install single-spa-react single-spa-vue
Step 2: Set Up the React Microfrontend
Create a React application that will serve as one of your microfrontends.
2.1 Initialize the React Microfrontend
npx create-single-spa
Choose
single-spa application / parcel
when prompted.Choose
react
as the framework.Follow the prompts to complete the setup.
2.2 Configure the React Microfrontend
In the React application's src
directory, you should see a file named root.component.js
. This file exports the root component for the microfrontend.
// src/root.component.js
import React from "react";
export default function Root(props) {
return <section>{props.name} is mounted!</section>;
}
Step 3: Set Up the Vue Microfrontend
Similarly, create a Vue application for the other microfrontend.
3.1 Initialize the Vue Microfrontend
npx create-single-spa
Choose
single-spa application / parcel
when prompted.Choose
vue
as the framework.Follow the prompts to complete the setup.
3.2 Configure the Vue Microfrontend
In the Vue application's src
directory, you should see a file named App.vue
. This file exports the root component for the microfrontend.
<!-- src/App.vue -->
<template>
<section>{{ name }} is mounted!</section>
</template>
<script>
export default {
name: "App",
props: {
name: String,
},
};
</script>
Step 4: Integrate Microfrontends with the Root Application
In your root Single-SPA application, you need to register the React and Vue microfrontends.
4.1 Register Microfrontends
Edit the src/index.js
file in your root configuration to include the microfrontends.
// src/index.js
import { registerApplication, start } from "single-spa";
import {
constructApplications,
constructRoutes,
constructLayoutEngine,
} from "single-spa-layout";
const routes = constructRoutes(document.querySelector("#single-spa-layout"));
const applications = constructApplications({
routes,
loadApp({ name }) {
return System.import(name);
},
});
const layoutEngine = constructLayoutEngine({ routes, applications });
applications.forEach(registerApplication);
layoutEngine.activate();
start();
Create an HTML layout file to define where each microfrontend will be rendered.
<!-- public/index.html -->
<!DOCTYPE html>
<html>
<head>
<title>Microfrontend Example</title>
<script type="systemjs-importmap">
{
"imports": {
"react-app": "//localhost:8500/react-app.js",
"vue-app": "//localhost:8501/vue-app.js"
}
}
</script>
</head>
<body>
<single-spa-router>
<main>
<route path="react">
<application name="react-app"></application>
</route>
<route path="vue">
<application name="vue-app"></application>
</route>
</main>
</single-spa-router>
<script src="https://cdn.jsdelivr.net/npm/systemjs/dist/system.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/single-spa-layout"></script>
<script src="/src/index.js"></script>
</body>
</html>
Step 5: Run the Microfrontends
Run each microfrontend on a different port to simulate a real-world scenario where microfrontends are hosted independently.
5.1 Run the React Microfrontend
Navigate to the React microfrontend directory and start the development server.
cd react-microfrontend
npm start
Ensure it runs on port 8500
as specified in the import map.
5.2 Run the Vue Microfrontend
Navigate to the Vue microfrontend directory and start the development server.
cd vue-microfrontend
npm start
Ensure it runs on port 8501
as specified in the import map.
Benefits of Microfrontends
1. Independent Development and Deployment
Each microfrontend can be developed, tested, and deployed independently, allowing teams to work on different parts of the application simultaneously without interfering with each other.
2. Technology Agnostic
Different microfrontends can use different technologies, frameworks, or libraries. This flexibility allows teams to choose the best tools for their specific needs and can help in gradually adopting new technologies.
3. Scalability
Microfrontends make it easier to scale both development and deployment processes. Teams can scale independently, and individual components can be optimized without affecting the entire application.
4. Improved Maintainability
By breaking down a large application into smaller, more manageable pieces, microfrontends can reduce the complexity of the codebase, making it easier to maintain and update.
5. Enhanced Collaboration
Microfrontends enable different teams to work on separate parts of the application without stepping on each other's toes. This autonomy promotes better collaboration and productivity, as teams can focus on their specific areas of expertise.
Disadvantages of Microfrontends
1. Increased Complexity
While microfrontends can reduce the complexity within each component, they introduce a new layer of complexity in managing multiple applications, ensuring they work seamlessly together. Coordinating deployments, handling version mismatches, and managing shared state across microfrontends can be challenging.
2. Performance Overhead
Integrating multiple microfrontends can introduce performance overhead due to increased network requests, heavier page loads, and potential duplication of resources. Optimizing performance and ensuring a smooth user experience requires careful planning and implementation.
3. Consistency and Coordination
Maintaining a consistent look and feel across different microfrontends can be difficult, especially when different teams use different technologies. Establishing shared design systems and guidelines is essential to ensure a unified user experience.
4. Shared State Management
Managing shared state and communication between microfrontends can be complex. Solutions like custom events, shared services, or global state management libraries need to be implemented to handle cross-microfrontend interactions effectively.
5. Operational Overhead
With multiple microfrontends, there is an increased operational overhead in terms of infrastructure, monitoring, logging, and deployment pipelines. Ensuring seamless integration and efficient operations across microfrontends requires additional tools and processes.
"Modularization is not just a coding principle; it's a pathway to innovation and agility." - Anonymous
Conclusion
Embrace the power of microfrontends and Single-SPA to build robust and scalable web applications!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
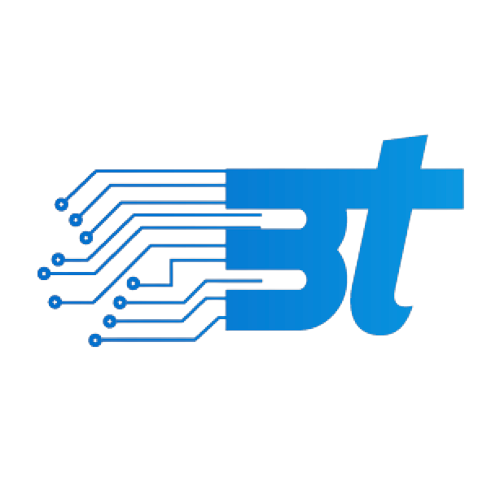
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.