Data Type in C#
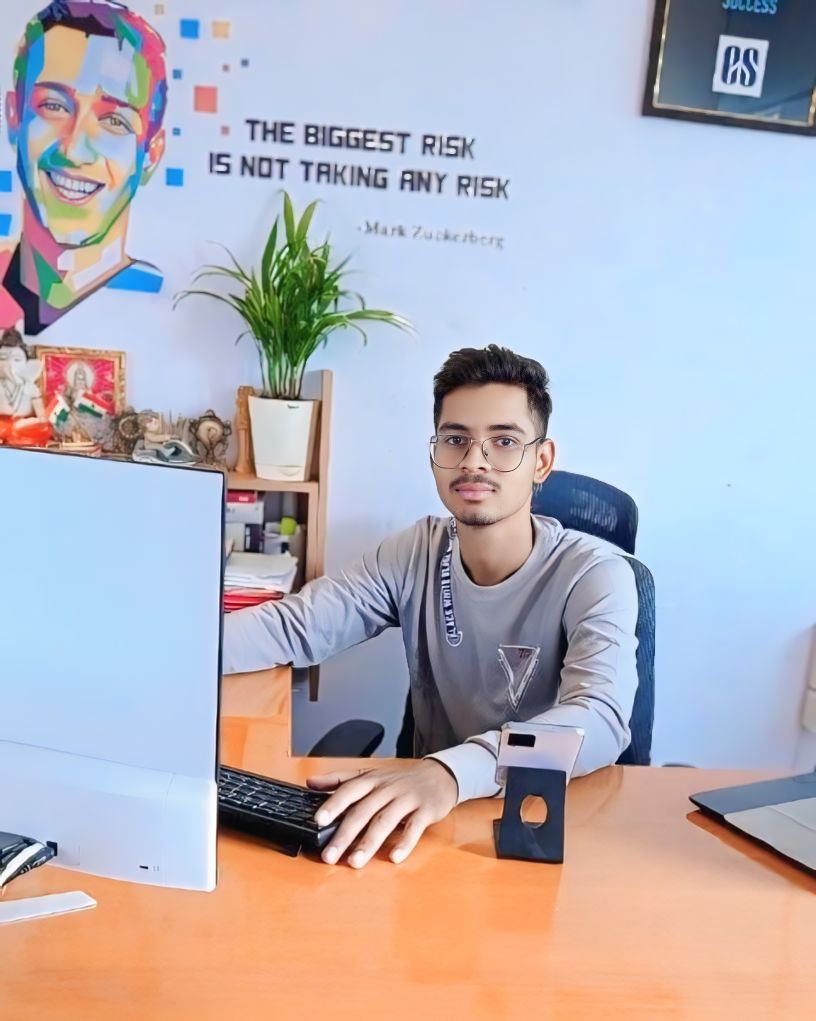
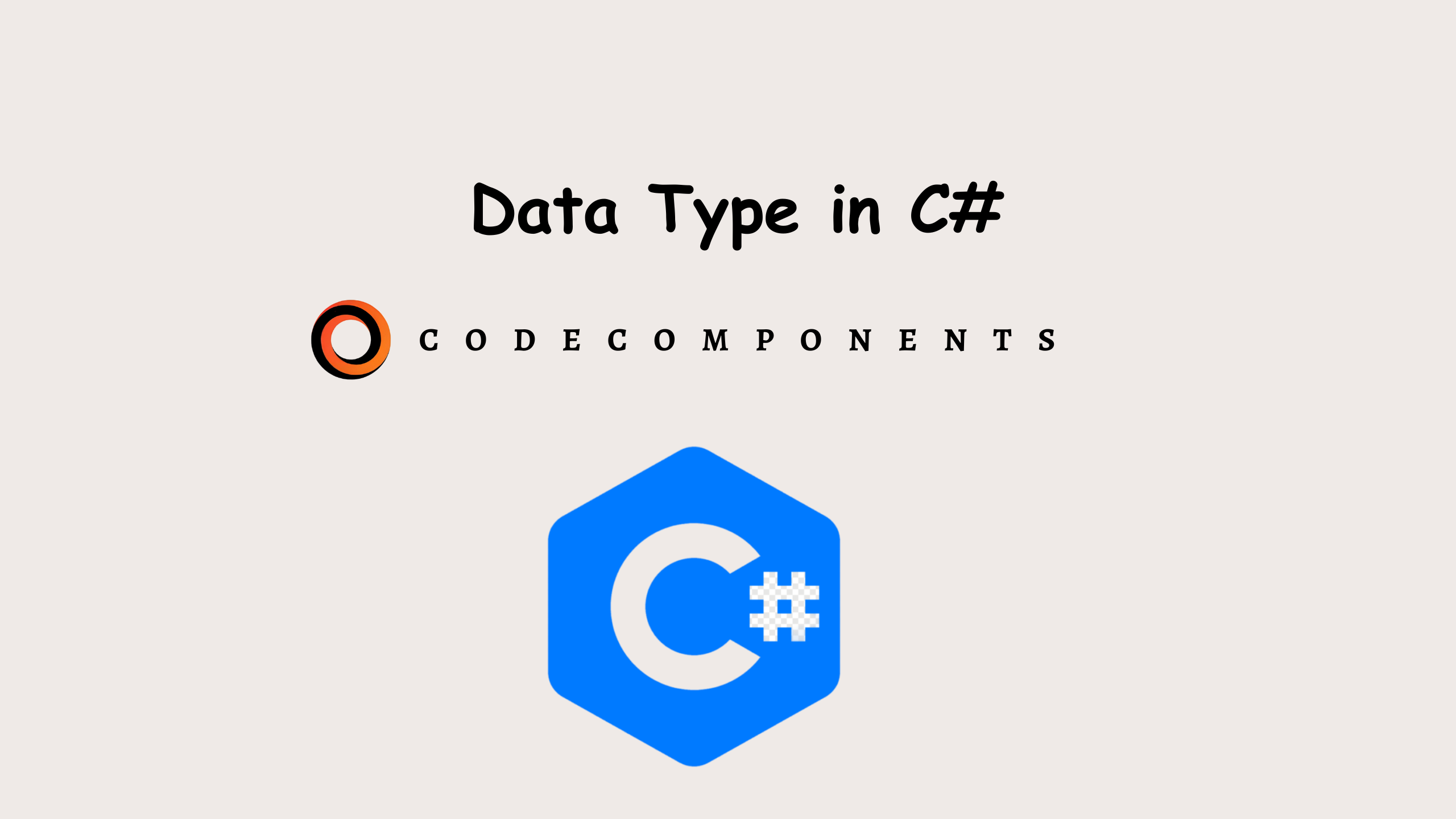
In C#, a data type defines what kind of value a variable can hold. Data types are essential in any programming language because they decide what operations can be done on the data and how the data is stored in memory.
In c# there are 3 type's of data types:
Value types
Refrence type
Pointer type
C# Data Types
|
|-- Value Types
| |-- Primitive Data Types
| | |-- Integral Types (byte, sbyte, short, ushort, int, uint, long, ulong, char)
| | |-- Floating-Point Types (float, double)
| | |-- Decimal Type (decimal)
| | |-- Boolean Type (bool)
| |
| |-- Non-Primitive Value Types
| | |-- Structs (custom structs, DateTime, TimeSpan)
| | |-- Enums (custom enums)
|
|-- Reference Types (Non-Primitive Types)
| |-- Classes (custom classes, String)
| |-- Interfaces (custom interfaces)
| |-- Arrays (int[], int[,], int[][])
| |-- Delegates (custom delegates)
| |-- Strings (string)
|
|-- Pointer Types (int*, char*)
Value Type:
**Value types are typically allocated on the stack memory .**Each variable holds its own copy of the data.
Value Types (e.g., int
, float
, struct
): The actual value is stored directly on the stack when they are local variables. Each variable of a value type has its own copy of the data.
Local variables:
Local variables are variables that are declared within a method, constructor, or block of code (such as within a loop or conditional statement). They are temporary and exist only during the execution of the method or block of code in which they are declared. Once the method or block completes execution, the local variables are destroyed and their memory is released.
Characteristics of Local Variables:
Scope: Local variables are accessible only within the method, constructor, or block of code where they are declared. They cannot be accessed outside of this scope.
Lifetime: The lifetime of a local variable begins when the method or block of code in which it is declared is entered and ends when the method or block of code is exited.
Storage: Local variables are typically stored on the stack. When the method or block of code completes execution, the stack memory used by the local variables is reclaimed.
Initialization: Local variables must be initialized before they are used. Using an uninitialized local variable will result in a compile-time error.
Range & bytes of value data type :
int
(4 bytes) and (-231 to 231 -1 Range).long
(8 bytes) and (-263 to 263 -1 Range).float
(4 bytes) and (-3.4 x 1038 to 3.4 x 1038 -1 Range).double
(8 bytes) and (-1.7 x 10308 to 1.7 x 10308 -1 Range).char
(2 bytes).string
(2 bytes per character).bool
(1 bytes).
Integer classified in 3 way's:
Int 16 ( 2 bytes or 16 bits)int , it's short int.
Int 32 ( 32-bit integer).
Int 64 (8 bytes or 64 bits).
Characteristics of Value Types
Direct Storage: The data for a value type is stored directly in the memory allocated for the variable, not in a separate heap-allocated object.
Stack Allocation: Value types are usually stored on the stack, a region of memory that provides fast allocation and deallocation.
Immutability in Structs: While not a strict rule, it is common practice to design structs (which are value types) to be immutable to avoid unintended side effects.
No Null Assignment: Value types cannot be assigned
null
unless they are defined as nullable types (e.g.,int?
,float?
).
In value type contain Primitive data type and none primitive data type.
Primitive data:
Primitive data types are the most basic types in C#. They represent single values and are usually built into the language.
Characteristics of Primitive Data Types
Built-in: Provided by the language itself.
Simple: Represent a single value.
Direct Storage: Store data directly in memory.
Non-Primitive Data Types:
Non-primitive data types are more complex and are not built into the language in the same way. They are usually user-defined or provided by the .NET framework and can represent a collection of values or a complex object.
Characteristics of Non-Primitive Data Types
Complex: Can represent multiple values or objects with various attributes.
Reference Types: Most non-primitive types are reference types and are stored on the heap.
User-Defined: Often defined by the programmer or provided by the .NET framework.
Reference Types:
Reference Types (e.g., class
,objects
, array
, string
,delegate
): The reference (pointer) to the data is stored on the stack if it's a local variable, but the actual data is stored on the heap. Variables of reference types share the same data by holding references to the same memory location.
References (Pointers to Objects): In C#, when you create an object of a class or an array, the reference (or pointer) to that object is typically stored in the stack if it is a local variable. However, the actual data (the object's fields or the array elements) is stored in the heap.
You already know about value types and reference types. Here is an explanation with an example:
public class Person
{
public string Name { get; set; }
}
public void ExampleMethod()
{
int age = 30; // Value type stored in stack
Person person = new Person(); // Reference to Person stored in stack, Person object stored in heap
person.Name = "Alice"; // Name is a string, so the reference to the string is stored in the heap
}
age
is a value type and is stored on the stack.person
is a reference type. The reference (pointer) to thePerson
object is stored on the stack, while the actualPerson
object is allocated on the heap.The
Name
property of thePerson
object is also a reference type (string
). The reference to the string "Alice" is stored in the heap, and the actual string data is also stored in the heap.
Stack and Heap Memory:
Value Types: Stored directly on the stack. Each variable holds its own copy of the data.
Reference Types: References stored on the stack, actual data stored on the heap. Multiple references can point to the same data on the heap.
Stack Memory: Fast access, automatically managed, stores value types and references to reference types.
Heap Memory: Flexible size, managed by garbage collector, stores actual data for reference types.
Stack Memory:
The stack is used for static memory allocation. This includes:
Local variables and parameters of methods.
Primitive data types (e.g.,
int
,float
,char
) and value types (e.g.,structs
).The stack operates in a last-in, first-out (LIFO) manner, which means that variables are added and removed in a specific order.
Characteristics:
Used for static memory allocation.
Operates in a last-in, first-out (LIFO) manner.
Fast access due to its LIFO nature.
Usage:
Stores value types and references to reference types (local variables).
Automatically managed: Variables are allocated and deallocated as methods are called and return.
Heap Memory:
The heap is used for dynamic memory allocation. This includes:
Objects and instances of classes.
Reference types (e.g., arrays, strings, custom class objects).
The heap does not have the same order constraints as the stack, which allows for more flexible memory allocation but also requires garbage collection to manage memory usage.
Characteristics:
Used for dynamic memory allocation.
Objects are accessed via references.
Managed by the garbage collector in C#, which deallocates memory that is no longer in use.
Usage:
- Stores the actual data for reference types.
Detailed Examples and Diagrams:
Example 1: Value Type
Consider the following code:
public void ExampleMethod() { int x = 10; // Value type int y = x; // Copy of value }
Memory Allocation:
x
is anint
stored directly on the stack.
y
is a copy ofx
and also stored on the stack.Diagram:
Stack: +-------+ | x = 10| +-------+ | y = 10| +-------+
Example 2: Reference Type
Consider the following code:
public class Person { public string Name { get; set; } } public void ExampleMethod() { Person person1 = new Person(); person1.Name = "Alice"; Person person2 = person1; person2.Name = "Bob"; }
Memory Allocation:
person1
is a reference stored on the stack.A
Person
object is created on the heap.
person2
is a reference to the samePerson
object.The
Name
property points to a string on the heap.Diagram:
Stack: +------------------+ | person1 (ref)----|----+ | person2 (ref)----|----| +------------------+ | | Heap: | +-------------------+ | | Person Object |<---+ | Name = "Bob" | +-------------------+
Example 3: Array (Reference Type)
Consider the following code:
public void ExampleMethod() { int[] numbers = new int[3]; numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; }
Memory Allocation:
numbers
is a reference stored on the stack.The actual array is allocated on the heap.
The array elements are stored within this allocated heap memory.
Diagram:
Stack: +--------------------+ | numbers (ref)------|----+ +--------------------+ | | Heap: | +--------------------+ | | Array Object |<--+ | [0] = 1 | | [1] = 2 | | [2] = 3 | +--------------------+
Pointer type:
Another category of data types.
In C#, the use of pointers is primarily intended for interoperability and performance-critical scenarios, and it is generally not recommended for regular application development due to the potential for unsafe operations. However, C# does support pointer types within an unsafe
context, which is a special mode that allows for operations not verified by the CLR (Common Language Runtime) for type safety.
Pointer Types in C#
Pointers in C# are similar to pointers in languages like C and C++. They hold the memory address of another type, rather than a direct value.
Syntax
To use pointers in C#, you need to:
Declare a method, class, or block of code as
unsafe
.Use the
*
symbol to denote pointer types.
Example of Pointer Declaration and Usage
Here is an example that demonstrates the basic usage of pointers in C#:
using System;
class Program
{
static unsafe void Main()
{
int number = 42;
int* p = &number; // Pointer to the variable 'number'
Console.WriteLine("Value of number: " + number); // Output: 42
Console.WriteLine("Address of number: " + (long)p); // Output: Address of 'number' in memory
Console.WriteLine("Value at address: " + *p); // Output: 42
*p = 100; // Change the value at the address pointed to by 'p'
Console.WriteLine("Value of number after change: " + number); // Output: 100
}
}
Key Points:
Unsafe Context: The
unsafe
keyword is required to use pointers in C#. Code that uses pointers must be compiled with the/unsafe
compiler option.Pointer Operators:
*
: Used to declare a pointer type and to dereference a pointer to access the value at the address.&
: Used to obtain the address of a variable.->
: Used to access members of a struct through a pointer.[]
: Used to index arrays when using pointers.
Fixed Statement: When working with managed objects (like arrays or strings), the
fixed
statement is used to pin the object in memory to prevent the garbage collector from moving it.csharpCopy codeunsafe { fixed (int* p = &number) { // Use pointer 'p' } }
Example of Using fixed
with Arrays
using System;
class Program
{
static unsafe void Main()
{
int[] array = { 1, 2, 3, 4, 5 };
fixed (int* p = array)
{
for (int i = 0; i < array.Length; i++)
{
Console.WriteLine("Element {0}: {1}", i, *(p + i));
}
}
}
}
In C#, the ->
operator is used to access members of a struct through a pointer, and the []
operator is used to index arrays when using pointers. These operators are typically used in an unsafe
context. Let's look at examples to illustrate how these operators are used.
Example of Using->
to Access Members of a Struct Through a Pointer
First, let's define a simple struct:
struct Point
{
public int X;
public int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
}
Now, let's use a pointer to access the members of this struct:
using System;
class Program
{
static unsafe void Main()
{
Point point = new Point(10, 20);
Point* p = &point;
// Access members using the -> operator
Console.WriteLine("X: " + p->X); // Output: X: 10
Console.WriteLine("Y: " + p->Y); // Output: Y: 20
// Modify members using the -> operator
p->X = 30;
p->Y = 40;
Console.WriteLine("Modified X: " + p->X); // Output: Modified X: 30
Console.WriteLine("Modified Y: " + p->Y); // Output: Modified Y: 40
}
}
Example of Using[]
to Index Arrays When Using Pointers
When working with arrays in an unsafe
context, you can use pointers to directly manipulate array elements. Here is an example:
using System;
class Program
{
static unsafe void Main()
{
int[] array = { 1, 2, 3, 4, 5 };
// Pin the array in memory using fixed
fixed (int* p = array)
{
// Access array elements using the [] operator
for (int i = 0; i < array.Length; i++)
{
Console.WriteLine("Element {0}: {1}", i, p[i]);
}
// Modify array elements using the [] operator
p[0] = 10;
p[1] = 20;
p[2] = 30;
// Verify the changes
for (int i = 0; i < array.Length; i++)
{
Console.WriteLine("Modified Element {0}: {1}", i, p[i]);
}
}
}
}
Considerations:
Security and Stability: Using pointers can lead to potential security vulnerabilities and stability issues because it bypasses the type-safety and memory management features of C#.
Performance: Pointers can offer performance benefits in certain scenarios, particularly in high-performance computing and systems programming.
Interoperability: Pointers are often used for interoperation with unmanaged code, such as calling functions from C/C++ libraries.
Summary
->
Operator: Used to access members of a struct through a pointer.- Example:
p->X
accesses theX
member of the struct pointed to byp
.
- Example:
[]
Operator: Used to index arrays when using pointers.- Example:
p[i]
accesses thei
-th element of the array pointed to byp
.
- Example:
Important Notes
Unsafe Code: Both examples require an
unsafe
context because pointer operations bypass the type-safety features of C#.Fixed Statement: The
fixed
statement is used to pin the array in memory to ensure that the garbage collector does not move it while it is being accessed through a pointer.Usage Considerations: While pointers can be powerful, they should be used with caution due to potential security and stability risks. They are generally used in performance-critical applications, low-level system programming, or for interoperability with unmanaged code.
While pointers can be powerful, their use should be limited to scenarios where their benefits clearly outweigh the risks and complexities they introduce. For most application development tasks, the managed features of C# provide a safer and more robust alternative.
Subscribe to my newsletter
Read articles from Mritunjay Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
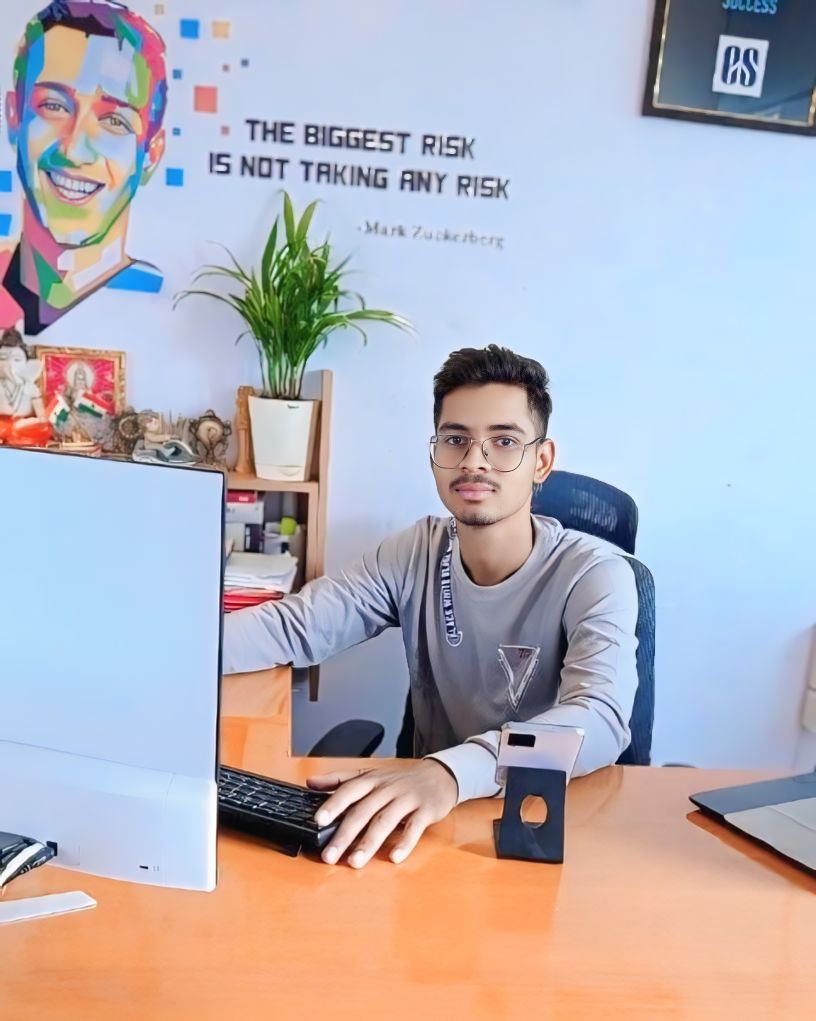