Bootstrap Customization: A Guide for Beginners
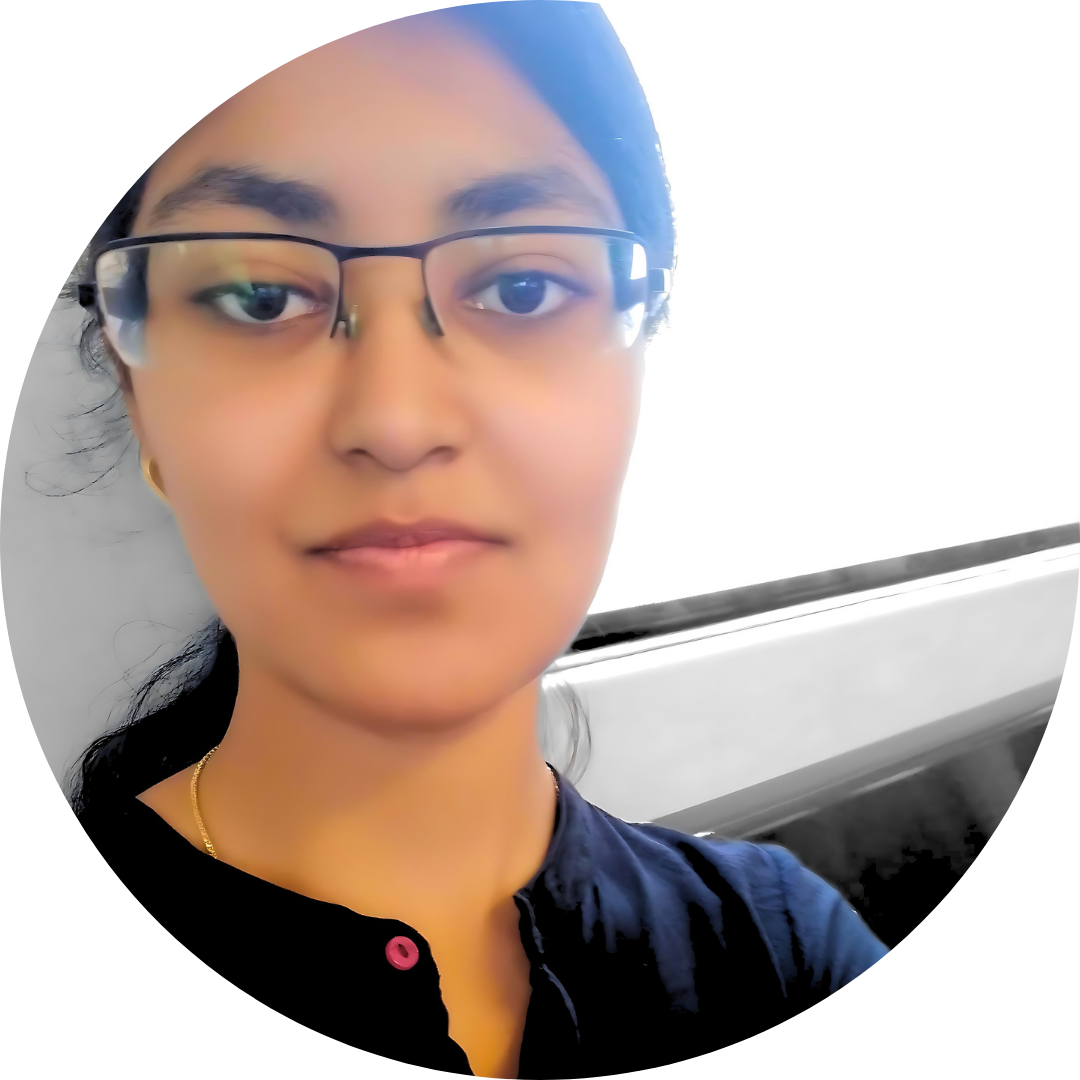
Table of contents
- what is bootstrap and why should we use it ?
- how to use Bootstrap in my projects?
- customising Bootstrap
- mini-project setup
- main.scss example file
- bootstrap customisation via scss map amends
- bootstrap components customisation example -- accordion customisation
- creating 3 different custom accordions and use them globally
- adding a new utility using bootstrap utility API
- final note
- deploying project to github pages
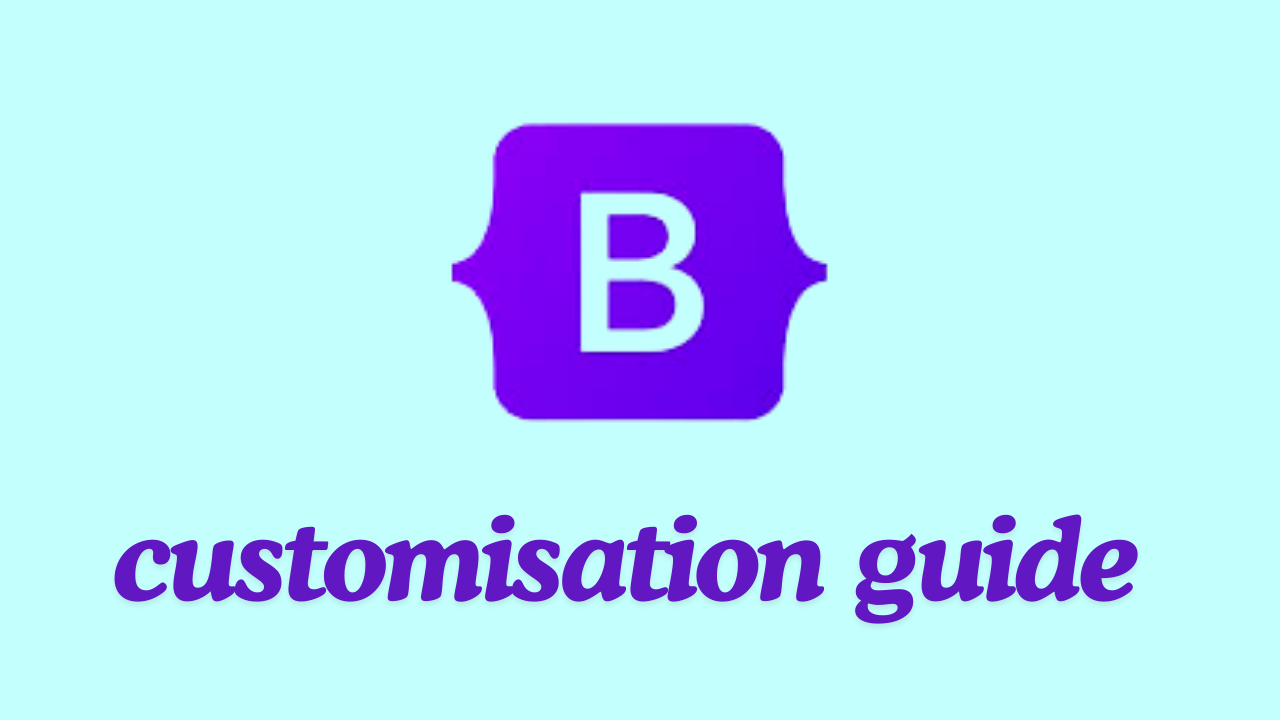
what is bootstrap and why should we use it ?
Bootstrap is a popular front-end framework that includes a collection of CSS and JS files, it is used to develop responsive and mobile-first web pages.
Bootstrap achieves responsiveness by using
Responsive Grid System
Flexible Images and Media [.img-fluid, .embed-responsive ....]
Media Queries [col-12, col-sm-6, col-md-4 ....]
Pre-designed responsive Components
Think of Bootstrap as a box of Lego blocks for websites, with ready-made pieces like buttons, menus, and forms etc ..., Just pick and assemble the pieces to quickly create a website that looks great on all devices.
how to use Bootstrap in my projects?
Bootstrap can be incorporated into your projects in two main ways:
compiled version
source code version
compiled version
it includes precompiled CSS and JavaScript files. These files are ready to be included directly in your project without any additional processing.
link to get precompiled bootstrap into your project from CDN
pros
Quick and Easy Setup , No build tools or preprocessing needed
better performance, CDNs can improve load times and cache the files for repeated use
cons
Includes all of Bootstrap files, even if you only need a subset [ however, you can use CSS purging in this case to decrease file size by removing unused styles]
Customizing the default styles is not possible without modifying the source files.
source code version
it includes Bootstrap’s source files, including SASS, JavaScript. This allows for extensive customization and optimization.
checkout this link to install bootstrap via package manager
Pros
Full Customization: Easily modify Bootstrap’s default styles to fit your project’s needs.
Optimized Builds: Include only the parts of Bootstrap you need, reducing file sizes.
Cons
Complex Setup: Requires a development environment and familiarity with build tools.
More Time-Consuming: Customizing and building Bootstrap takes more time than using the precompiled version.
customising Bootstrap
mini-project setup
- Ensure you have Node.js and npm installed.
npm init -y //creates default package.json file
npm install --save-dev parcel //Installs Parcel as a dev dependency
npm i bootstrap@latest //Installs bootstrap as a project dependency
// parcel is a build tool like webpack
create a src folder and put project's source code[ HTML, CSS, JavaScript, images, and other assets] into this folder. it's where you write and edit code during development.
//package.json
{
"scripts": {
"start": "parcel src/index.html", // Development server
}
}
//make this change in package.json to run your project
run -- npm run start to start a local server
/project-root
├── /node_modules # Managed by npm, contains dependencies
│ ├── /parcel-bundler # Parcel build tool
│ └── /bootstrap # Bootstrap framework files
│
├── /src
│ ├── /scss
│ │ ├── /abstracts
│ │ │ ├── _variables.scss # Global variables (overrides for Bootstrap variables)
│ │ ├── /base
│ │ │ ├── _reset.scss
│ │ │ └── _typography.scss
│ │ ├── /components
│ │ │ ├── _buttons.scss
│ │ │ └── _forms.scss
│ │ ├── /layout # Styles for the overall layout (grid, header, footer)
│ │ │ ├── _grid.scss
│ │ │ ├── _header.scss
│ │ │ └── _footer.scss
│ │ ├── /pages # Page-specific styles (home page, about page, etc.)
│ │ │ ├── _home.scss
│ │ │ └── _about.scss
│ │ ├── /themes # Styles for different themes (dark theme, light theme)
│ │ │ ├── _theme-dark.scss
│ │ │ └── _theme-light.scss
│ │ ├── /vendors # Styles from third-party libraries or frameworks
│ │ └── main.scss # Main SCSS file importing all partials
│ ├── /js
│ │ ├── _bootstrap_custom.js # Custom Bootstrap JavaScript
│ │ ├── _modal.js # Custom modal functionality
│ │ └── main.js # Main JavaScript file importing custom scripts
│ ├── index.html # Main HTML file
│ └── ... # Other project assets
└── package.json
finally our project structure must look like above diagram
checkout this link to learn more about sass
main.scss
- we donot write any scss in main.scss, we just import all scss partials here and link this file to html, same happens with main.js
//index.html
<link rel="stylesheet" href="./scss/main.scss" />
<script src="./js/main.js" type="module"></script>
/* dont forget to use type='module'*/
main.scss example file
//main.scss
/*Functions are imported first because they might be used
in variable declarations and mixins that follow*/
@import "../../node_modules/bootstrap/scss/functions";
// Import Bootstrap's variables and later mixins
@import "../../node_modules/bootstrap/scss/variables";
/* Mixins are imported after variables because mixins
often utilize variables*/
@import "../../node_modules/bootstrap/scss/mixins";
@import './abstracts/variables';
@import './base/typography';
@import './components/buttons';
/*always bootstrap scss variable overrides must be imported before main
bootstrap scss import else compiler thinks there is no overrided
styles, it should apply the default styles*/
@import '../../node_modules/bootstrap/scss/bootstrap.scss';
/*always bootstrap css-variables/css must be overrided
after main bootstrap imports */
@import './abstracts/css-variables';
@import './components/css-dropdown';
@import './components/css-card';
bootstrap customisation via scss map amends
//_variables.scss
// Amend the bootstrap $theme-colors map with custom colors
$theme-colors: map-merge(
$theme-colors,
(
"primary": #00baf2,
"secondary":hsl(218, 100%, 22%),
"light": #e0f5fd,
"dull-white":#F5F7FA,
"white":#FEFEFE
)
)
/*using this way of amending you can change bootstrap default colors
and also add new colors to the themecolors map so that these
customisations apply globally*/
/*in same way you can amend any maps in bootstrap and customise them*/
in the above way you can amend any sass map of bootstrap [$spacers map, $grid-breakpoints map, $font-sizes map, $btn-sizes map etc.. ]
// style.css
:root{
--primary:#{map-get($theme-colors,'primary')};
}
to use amended colors in your stylesheets use interpolation , map-get in your css like above
bootstrap components customisation example -- accordion customisation
to change the bootstrap accordion appearance and look globally in your project, like changing text-colors, background-color, border etc... you can change sass variables, css variables of accordion
//_accordion.scss
$accordion-border-width: 0;
$accordion-button-icon: '';
$accordion-button-active-icon: '';
$accordion-button-focus-border-color: none;
$accordion-button-focus-box-shadow: none;
$accordion-button-active-bg: none;
$accordion-color: map-get($theme-colors,'accordion-grey');
$accordion-button-color: map-get($theme-colors,'accordion-grey');
$accordion-button-active-color: map-get($theme-colors,'accordion-grey');
//_css-accordion.scss
.accordion-button {
font-size: 0.85rem;
font-weight: 700;
span {
margin-right: 5px;
}
}
above changes are example scss and css variable changes i made to customise bootstrap accordion
so after that wherever in the project, you try to use bootstrap accordion, your customised bootstrap accordion appears
checkout more bootstrap accordion sass,css variables in this link
you can also capture collapse events of accordion and make some javascript customisations also for accordion
//accordion.js
let accordion = document.querySelector('.accordion');
accordion.addEventListener('show.bs.collapse', function(event) {
const button = event.target.closest('.accordion-item').querySelector('.accordion-button');
const icon = button.querySelector('.fa-plus');
if (icon) {
icon.classList.remove('fa-plus');
icon.classList.add('fa-circle-xmark');
}
});
above is an example where i changed accordion's button icon on open of accordion collapse [show.bs.collapse event]
checkout this link to checkout more bootstrap toggle events
with above example you can directly customise bootstrap accordion globally, but by doing this you will not be able to use bootstrap default accordion anywhere in your project, so if your project needs same type of custom accordion in all pages then only use this method
creating 3 different custom accordions and use them globally
to do so you should not touch default bootstrap sass, css variables . create you own css classes globally and do required changes to those css classes, like new bgcolors,fonts,colors,borders ,paddings,margins etc...
apply those css classes along with bootstrap accordion default classes
- as in main.scss you have imported custom css file after bootstrap imports , your custom class styles will have more specificity and they will be applied to the accordion
in this way you can create number of accordion styles globally based on your requirement
adding a new utility using bootstrap utility API
for example i dont have any bootstrap utility classes to specify z-index, so i can create this new custom utility in my project and use it all over project by following process
// Define custom utilities in _utilities.scss
$utilities: map-merge(
$utilities,
(
"zindex": (
property: z-index,
class: z,
values: (
"0": 0,
"10": 10,
"20": 20,
"30": 30,
"40": 40,
"50": 50,
"auto": auto
)), ));
//index.html usage
<div class="position-relative z-10">Element with z-index 10</div>
"zindex"
: This is the key for the custom utility. It will be used as the prefix for the generated CSS classes.
class: z
: Defines a class prefix to use instead of the key. This means that instead of .zindex-10
, the class will be .z-10
.
final note
by using above way you can make a fully customised bootstrap for your projects by doing
color customisations
typography customisations
spacing customisation
component customisation
utility customisation etc ...
deploying project to github pages
npm install --save-dev gh-pages // install ghpages package
//package.json
"build": "parcel build src/index.html --public-url ./",
"predeploy": "npm run build",
"deploy": "gh-pages -d dist"
//add above 3 scripts in package.json
npm run build
npm run deploy
after deploying go to github > project-repositary > settings > pages > select gh-pages branch > click on save [thats it project will be deployed]
Subscribe to my newsletter
Read articles from prasanna pinnam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
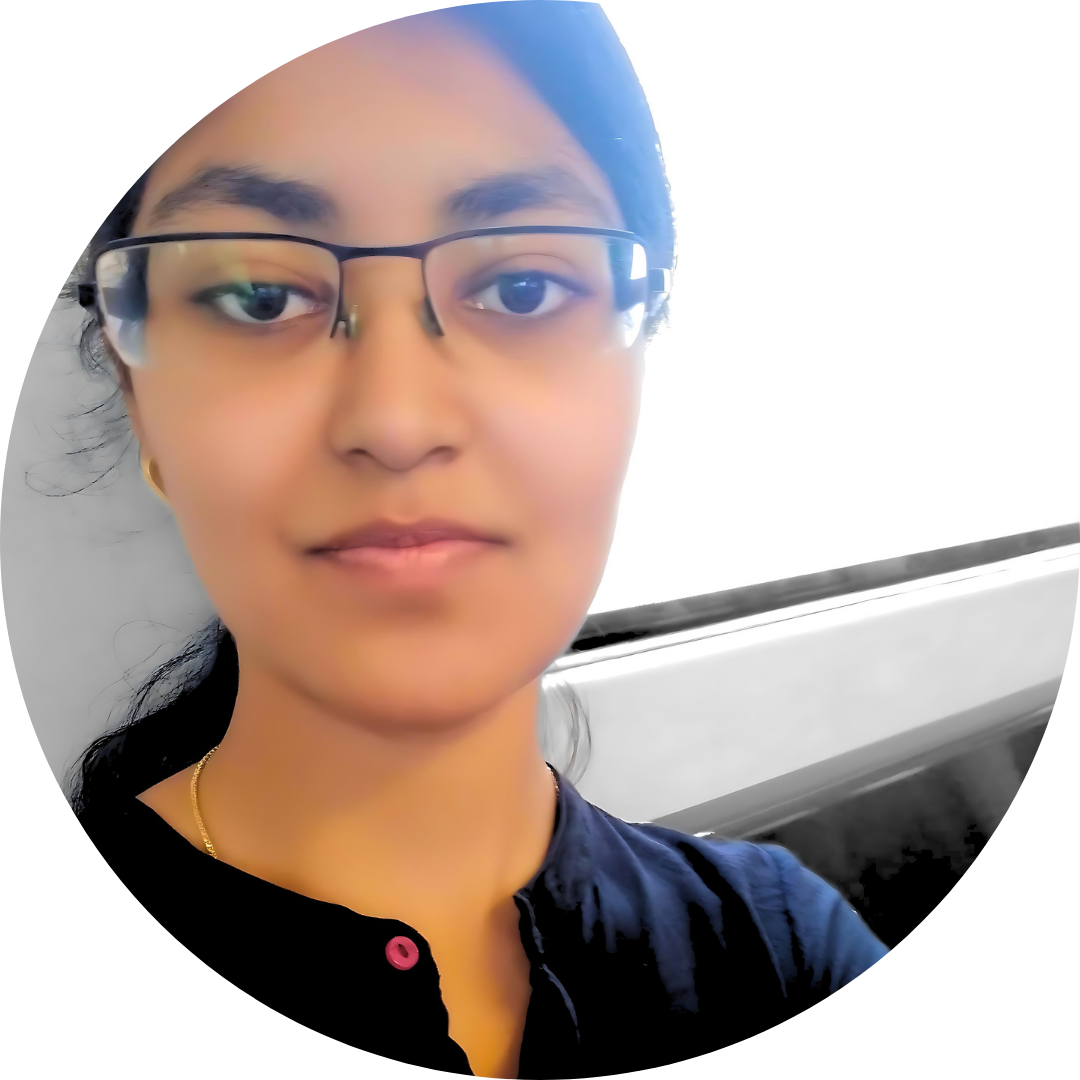