How to implement drag-and-drop functionality for rows in Material-UI DataGrid using React Beautiful DnD?
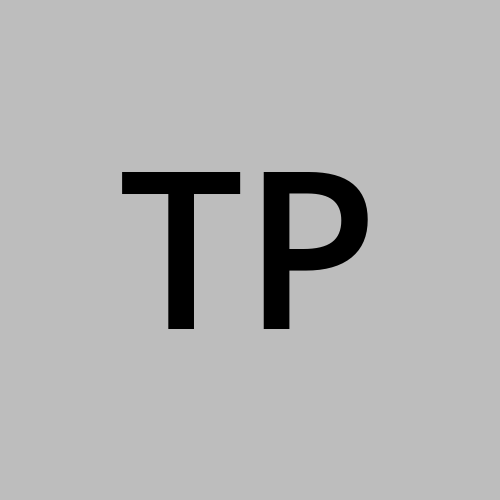
Drag and drop functionality is a powerful feature in web applications that enhances user interaction and usability. In this blog, we'll explore how to integrate drag and drop capabilities into a Material-UI (MUI) Data Grid using react-beautiful-dnd
. By the end, you'll be able to reorder rows within the Data Grid seamlessly.
Prerequisites
Basic knowledge of React and JavaScript.
Node.js and npm (or yarn) installed on your machine.
An existing React project set up.
Step 1: Setting Up Your React Project
If you haven't already set up a React project, you can create a new one using Create React App:
npx create-react-app drag-and-drop-mui-data-grid cd drag-and-drop-mui-data-grid
Next, install the required dependencies:
bashCopy codenpm install @mui/material @emotion/react @emotion/styled react-beautiful-dnd
Step 2: Integrating MUI Data Grid
Let's start by setting up a basic MUI Data Grid component with mock data. Open
App.js
(or your desired component file) and import the necessary components and styles:import React, { useState } from 'react'; import { DataGrid, GridRow, GridCell } from '@mui/x-data-grid'; import { DragDropContext, Droppable, Draggable } from 'react-beautiful-dnd'; const columns = [ { field: 'id', headerName: 'ID', width: 90 }, { field: 'name', headerName: 'Name', width: 150 }, { field: 'age', headerName: 'Age', width: 90 }, ]; const rows = [ { id: '1', name: 'John Doe', age: 30 }, { id: '2', name: 'Jane Smith', age: 25 }, { id: '3', name: 'User 3', age: 23 }, { id: '4', name: 'User 4', age: 24 }, { id: '5', name: 'User 5', age: 25 }, ];
Step 3: Creating Custom Row Component
Next, let's create a custom row component (
CustomRowWrapper
) usingDraggable
fromreact-beautiful-dnd
. This component will wrap each row in the Data Grid to make them draggable:const CustomRowWrapper = ({ row, index, ...rest }) => { const rowStyle = { padding: '8px', margin: '4px 0', backgroundColor: 'red', border: '5px solid black', }; return ( <Draggable key={`row-${row.id}`} draggableId={`row-${row.id}`} index={index} > {(provided, snapshot) => ( <GridRow index={index} row={row} {...rest} ref={provided.innerRef} {...provided.draggableProps} {...provided.dragHandleProps} sx={{ ...provided.draggableProps.style, ...rowStyle, backgroundColor: snapshot.isDragging ? '#f0f0f0' : 'inherit', }} > <GridCell>{row.id}</GridCell> <GridCell>{row.name}</GridCell> <GridCell>{row.age}</GridCell> </GridRow> )} </Draggable> ); };
Step 4: Implementing Drag and Drop Functionality
Now, let's integrate
DragDropContext
andDroppable
fromreact-beautiful-dnd
around the MUI Data Grid. We'll handle drag events (onDragEnd
) to update the order of rows (reorderedRows
state):const CustomDataGrid = () => { const [reorderedRows, setReorderedRows] = useState(rows); const onDragEnd = (result) => { if (!result.destination) { return; } const newReorderedItems = Array.from(reorderedRows); const [removed] = newReorderedItems.splice(result.source.index, 1); newReorderedItems.splice(result.destination.index, 0, removed); setReorderedRows(newReorderedItems); }; return ( <DragDropContext onDragEnd={onDragEnd}> <Droppable droppableId="data-grid"> {(provided) => ( <div ref={provided.innerRef} {...provided.droppableProps}> <DataGrid rows={reorderedRows} columns={columns} slots={{ row: CustomRowWrapper, }} /> {provided.placeholder} </div> )} </Droppable> </DragDropContext> ); };
Conclusion
In this tutorial, we've explored how to integrate drag and drop functionality into a MUI Data Grid using react-beautiful-dnd
. By following these steps, you can create a more interactive and user-friendly data management experience in your React applications.
Experiment with different configurations and explore more features offered by react-beautiful-dnd
to further enhance your application's drag and drop capabilities.
Subscribe to my newsletter
Read articles from Tarun Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
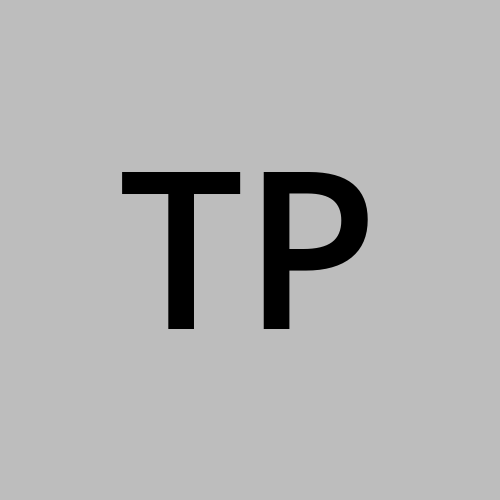