Understanding Decision Trees: Features, Benefits, and Implementation
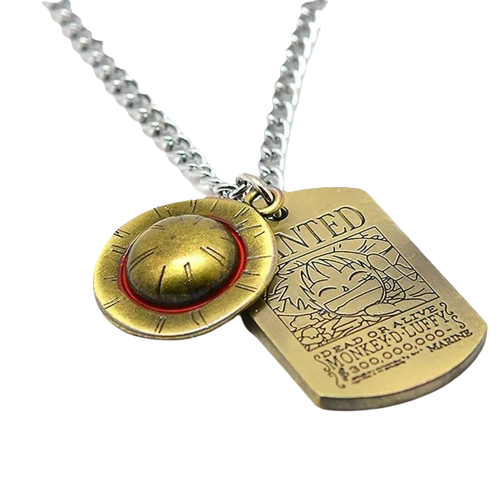
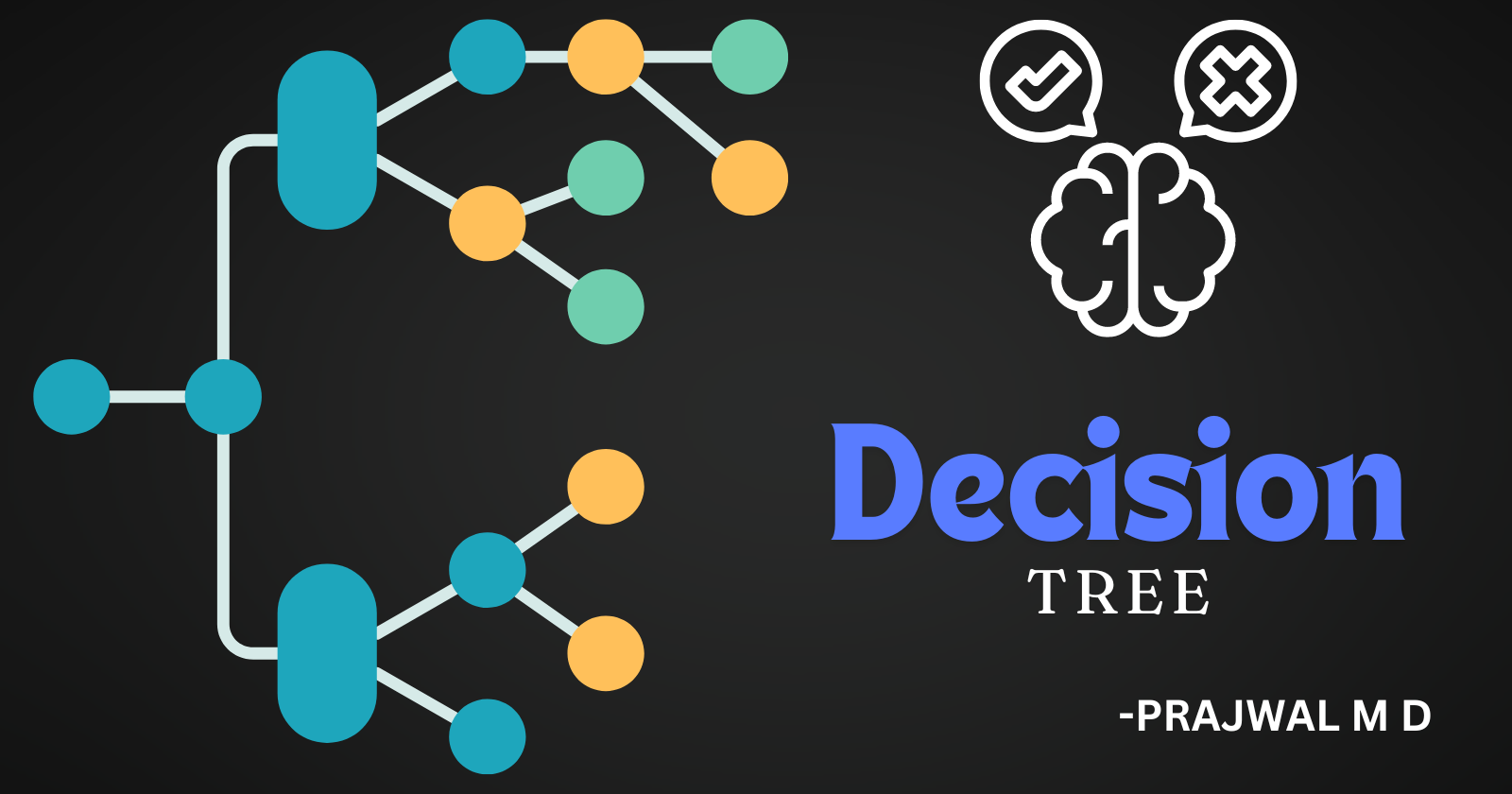
Introduction to Decision Tree Algorithm
If you’ve ever had to make a complex decision and found yourself sketching out a list of pros and cons, you’ve already engaged in a form of decision tree analysis. Decision trees are a powerful yet intuitive tool for solving classification and regression problems in data science. They are used to make predictions by learning simple decision rules inferred from the data.
Overview of Decision Trees
A decision tree is a flowchart-like structure in which each internal node represents a decision based on the value of a feature, each branch represents the outcome of that decision, and each leaf node represents a final outcome or prediction. They are called "trees" because they start from a single root and grow into a structure that resembles a tree, though they are typically represented upside down, with the root at the top.
Why Decision Trees are Important
Decision trees are popular in machine learning because they are easy to understand and interpret. They require little data preparation, can handle both numerical and categorical data, and are capable of performing well with even relatively small datasets. Their simplicity and transparency make them an excellent starting point for many data science projects.
How Decision Trees Work
Decision trees split data into branches based on feature values, creating a path from the root of the tree to a leaf node that predicts the target variable. Let's break down the process with an example.
Explanation of the Algorithm
Choose the Best Feature to Split On: The algorithm starts at the root node and evaluates all features to find the one that best splits the data into distinct groups. The "best" feature is typically the one that provides the most information gain, which is a measure of how well the feature separates the data into different classes.
Split the Data: The data is then split into subsets based on the chosen feature. Each subset forms a branch of the tree.
Repeat the Process: The splitting process is repeated recursively on each branch, using the remaining features, until one of the stopping criteria is met. Common stopping criteria include reaching a maximum tree depth, having a minimum number of samples per leaf node, or having all the samples in a node belonging to the same class.
Make Predictions: Once the tree is built, it can be used to make predictions. New data points are passed through the tree, following the decision rules at each node, until they reach a leaf node. The prediction is the value at the leaf node.
Example with a Step-by-Step Process
Imagine you are a bank trying to decide whether to approve loan applications. You have data on past applicants, including their income, credit score, and loan status (approved or not approved). You want to build a decision tree to help with future decisions.
Choosing the First Feature:
- You find that splitting on "Credit Score" provides the highest information gain.
Splitting the Data:
- Applicants with a credit score above 700 go down one branch, while those below 700 go down another.
Repeating the Process:
- For applicants with a credit score above 700, you might next split based on income level. Those with an income above $50,000 might have a high likelihood of loan approval, while those below $50,000 might require further assessment.
Making Predictions:
- For a new applicant with a credit score of 750 and an income of $60,000, the tree predicts loan approval.
Features of Decision Tree Algorithm
Decision trees come with several features that make them unique and versatile for various data science tasks.
Key Features and Their Significance
Simplicity and Interpretability:
- Decision trees are easy to understand and interpret. The visual representation of decision-making paths helps in explaining the model to stakeholders.
Handling Different Data Types:
- Decision trees can handle both numerical and categorical data, making them versatile for different types of datasets.
No Need for Data Normalisation:
- Unlike some other algorithms, decision trees do not require data normalisation, simplifying the preprocessing steps.
Ability to Handle Non-linear Relationships:
- They can capture non-linear relationships between features and the target variable, which can be beneficial for complex datasets.
Robustness to Outliers:
- Decision trees are relatively robust to outliers since splits are based on feature thresholds that are not influenced by extreme values.
Advantages and Disadvantages
Advantages:
Ease of Understanding: They are intuitive and easy to explain to non-experts.
No Data Preparation Needed: They require minimal data preprocessing.
Versatile: Can handle both regression and classification tasks.
Non-parametric: They make no assumptions about the data distribution.
Disadvantages:
Overfitting: They can easily overfit the training data, capturing noise rather than the underlying pattern.
Instability: Small changes in the data can lead to completely different trees.
Bias Towards Dominant Features: Decision trees can be biased towards features with more levels.
Practical Example and Implementation
Let’s look at a practical example and see how to implement a decision tree in Python.
Example: Classifying Customer Feedback
Imagine you are working for a company that receives customer feedback and you want to classify the feedback into positive or negative categories. You have a dataset with feedback texts and corresponding labels.
Steps to Implement a Decision Tree in Python:
Load the Data:
import pandas as pd data = pd.read_csv('customer_feedback.csv')
Preprocess the Data:
from sklearn.feature_extraction.text import TfidfVectorizer vectorizer = TfidfVectorizer() X = vectorizer.fit_transform(data['feedback_text']) y = data['label']
Split the Data:
from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
Train the Model:
from sklearn.tree import DecisionTreeClassifier clf = DecisionTreeClassifier() clf.fit(X_train, y_train)
Evaluate the Model:
from sklearn.metrics import accuracy_score y_pred = clf.predict(X_test) print('Accuracy:', accuracy_score(y_test, y_pred))
This code demonstrates a basic workflow for implementing a decision tree in Python to classify customer feedback.
Handling Overfitting in Decision Trees
Overfitting is a common problem with decision trees, where the model learns the training data too well, capturing noise along with the underlying pattern. Here are some techniques to prevent overfitting.
Techniques to Prevent Overfitting
Pruning:
- Pruning involves removing parts of the tree that do not provide additional power to classify instances. This helps simplify the model and reduce overfitting.
Setting Maximum Depth:
- Limiting the depth of the tree prevents it from becoming too complex and capturing noise from the training data.
Minimum Samples per Leaf Node:
- Setting a minimum number of samples required to be in a leaf node ensures that the model does not create branches for very few samples.
Using Ensemble Methods:
- Techniques like Random Forest and Gradient Boosting combine multiple decision trees to create a more robust model that generalises better to unseen data.
Best Practices
Cross-validation: Use cross-validation to evaluate the performance of your decision tree and ensure it generalises well to new data.
Feature Importance: Analyse feature importance to understand which features contribute the most to the model and remove irrelevant ones.
Conclusion
Decision trees are a versatile and intuitive tool for tackling both classification and regression problems. Their ease of use and interpretability make them an excellent choice for initial data analysis and modelling. While they can suffer from overfitting and instability, proper techniques like pruning and setting appropriate parameters can help mitigate these issues.
By understanding how decision trees work and implementing them effectively, you can harness their power to make informed decisions and gain valuable insights from your data. Whether you are a beginner in data science or a seasoned professional, decision trees offer a solid foundation for building more complex models and exploring the vast landscape of machine learning.
Subscribe to my newsletter
Read articles from Prajwal M D directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
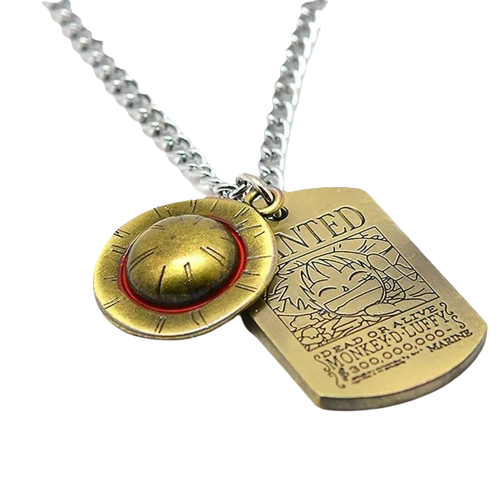
Prajwal M D
Prajwal M D
I'm a innovative individual, fervently converting complex challenges into elegantly simple and intuitively seamless designs and developments, skillfully amalgamating Data Analytics and Machine Learning Algorithms".