Let's Get into DOM Manipulation
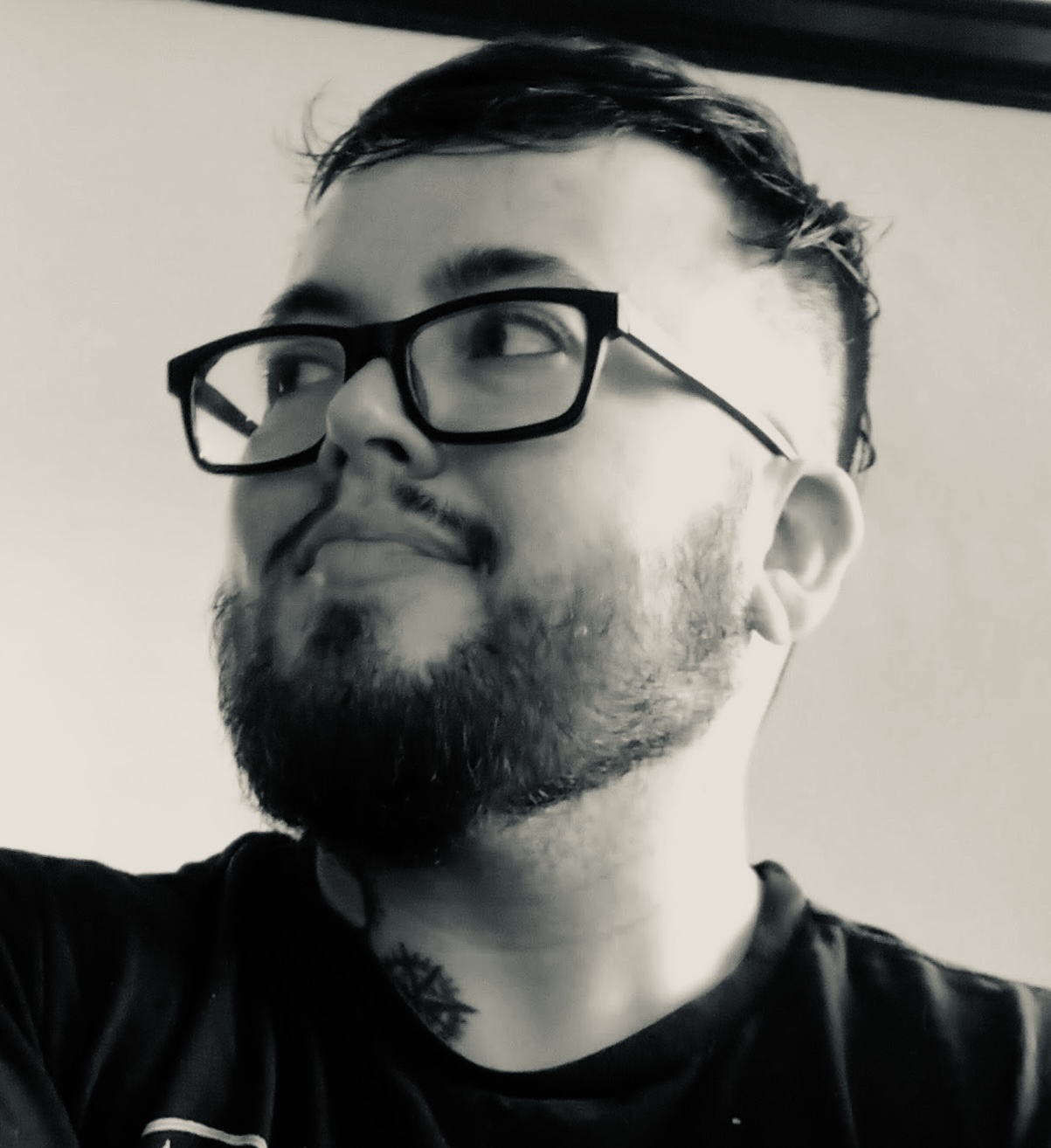
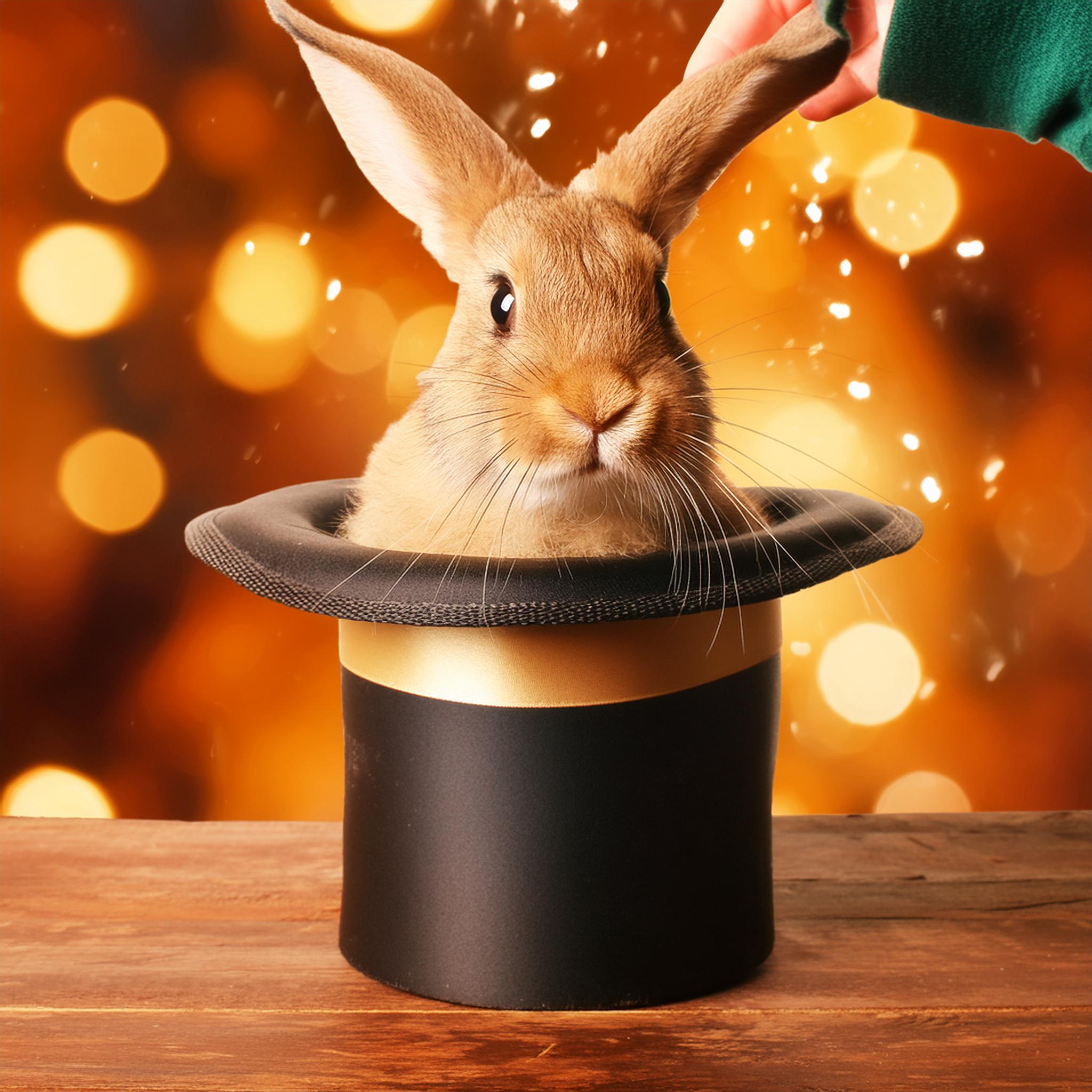
DOM (Document Object Model) manipulation is a cornerstone of modern web development. It allows developers to create dynamic, interactive websites by programmatically changing the content and structure of web pages. In this post, we'll delve into the essentials of DOM manipulation, its importance, and some practical examples using JavaScript.
What is the DOM?
The DOM is a programming interface for web documents. It represents the structure of a document as a tree of objects, where each node is an object representing a part of the document. For example, elements, attributes, and text within an HTML document are all part of the DOM. This tree structure allows developers to access and manipulate the content, structure, and style of web pages dynamically.
Why is DOM Manipulation Important?
DOM manipulation is crucial because it enables dynamic updates to the web page without requiring a full reload. This is essential for creating modern, responsive web applications where user interactions can lead to immediate visual feedback. Some common use cases include:
Form Validation: Displaying error messages dynamically as users fill out forms.
Content Updates: Loading new content based on user actions, like clicking a button or scrolling.
Animations and Effects: Creating interactive experiences with animations and effects that respond to user inputs.
Single Page Applications (SPAs): Changing views and content dynamically without reloading the entire page.
Basic DOM Manipulation with JavaScript
To manipulate the DOM, you need to use JavaScript. Here are some basic techniques for selecting and modifying elements:
Selecting Elements
You can select DOM elements using various methods provided by the document
object. Some commonly used methods include:
Consider this html element:
<div id="main-section" class="main-section"><h1>Gimme the bits!</h1></div>
Lets create a new variable and use different methods to select it, assigning it to the variable for manipulation.
getElementById
: Selects a single element by the ID you give it in your html,let myDopeElement = document.getElementById('main-section');
getElementsByClassName
: Selects all elements with a given class name.let myDopeElement = document.getElementsByClassName('main-section');
getElementsByTagName
: Selects all elements with a given tag name.let myDopeElement = document.getElementsByTagName('h1');
querySelector
: Selects the first element that matches a CSS selector. In the html snippet above, the class is 'main-section
', hence to select it in our CSS we'd use a class selector (.main-section
). ThequerySelector
method uses the CSS selector rather than html type/ class/ or ID.let myDopeElement = document.querySelector('.myClass');
querySelectorAll
: Selects all elements that match a CSS selector.let myDopeElement = document.querySelectorAll('.myClass');
In programming there's always many different approaches to achieving the same result, and this is a perfect example. If you're like me, you're always wondering "which one should I use?" A quick note about selectElementByX
and querySelector
methods and when to use which:
Use querySelector
when:
You need to select the first matching element.
You are using complex CSS selectors, including IDs, attributes, and nested selectors.
You prefer a concise and versatile method that aligns with CSS selector syntax.
Use selectElementByX
when:
You need to select multiple elements with a specific class name.
You want better performance for class name selections in large documents.
You are working with older codebases where this method is commonly used.
Modifying Elements
Now that you know different ways to select an element, the fun really begins- you can modify it using various properties and methods:
Changing Content: Use
innerHTML
ortextContent
to change the content of an element.myDopeElement.innerHTML = 'New content'; myDopeElement.textContent = 'New text content';
Changing Attributes: Use
setAttribute
to change an attribute of an element.myDopeElement.setAttribute('src', 'newImage.png');
Changing Styles: Modify the
style
property to change the CSS styles of an element.myDopeElement.style.color = 'blue'; myDopeElement.style.fontSize = '20px';
Adding/Removing Classes: Use
classList
to add or remove classes.myDopeElement.classList.add('newClass'); myDopeElement.classList.remove('oldClass');
Using Event Listeners to Make the Page Interactive
From this point we can do even more. Event listeners are a crucial part of making web pages interactive. They allow you to execute JavaScript code in response to various user interactions, such as clicks, mouse movements, keyboard events, and more. This is where we can really use JS to make our page interactive!
Adding Event Listeners
To add an event listener to an element, you use the addEventListener
method. This method takes two main arguments: the type of event to listen for and the function to execute when the event occurs.
Syntax:
myDopeElement.addEventListener('event', function);
Common Event Types
Here are some common event types you might use to 'fire' a corresponding behavior. Below that are examples of some ways we could use them:
click
: Fires when an element is clicked.mouseover
: Fires when the mouse pointer is over an element.mouseout
: Fires when the mouse pointer leaves an element.keydown
: Fires when a key is pressed down.keyup
: Fires when a key is released.
Example: Click Event Listener
Let's add a click event listener to a button that changes its text content when clicked:
<!DOCTYPE html>
<html lang="en">
<body>
<button id="myButton">Click Me!</button>
<!-- Here we will use an event listener to change the button's text from 'Click Me!' to 'I was Clicked!' -->
<script>
let button = document.getElementById('myButton');
button.addEventListener('click', function() {
button.textContent = 'I was clicked!';
});
</script>
</body>
</html>
Example: Mouseover Event Listener
Here's how you can change the background color of a button when the pointer hovers over it:
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Below are the styles currently applied to our html -->
<style>
#myButton {
width: 100px;
height: 100px;
background-color: lightblue;
}
</style>
</head>
<body>
<!-- Let's give a button the id that we will the select for manipulation -->
<button id="myButton"></button>
<!-- Use a function and an event listener to turn the button's color to yellow -->
<!-- Removing the mouse will turn it back to light blue -->
<script>
let button = document.getElementById('myButton');
button.addEventListener('mouseover', function() {
div.style.backgroundColor = 'yellow';
});
button.addEventListener('mouseout', function() {
div.style.backgroundColor = 'lightblue';
});
</script>
</body>
</html>
In this example, the button's background color changes to yellow when the pointer is over it and reverts to light blue when the pointer is removed.
Conclusion
DOM manipulation is a fundamental skill for web developers, enabling the creation of interactive and dynamic web applications. By understanding how to select and modify elements in the DOM, you can enhance the user experience and build more engaging websites. By adding event listeners to respond to user interactions like clicks, mouse movements, and keyboard events, you can make your web applications interactive. This is where JavaScript meets html/css to make magic!
Combine these techniques and explore different options- hop on Codepen or Replit and try creating a button that changes text when you click it, or maybe it can change the background color of a div and header. The possibilities are endless.
Feliz coding fam!
Subscribe to my newsletter
Read articles from Millan Figueroa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
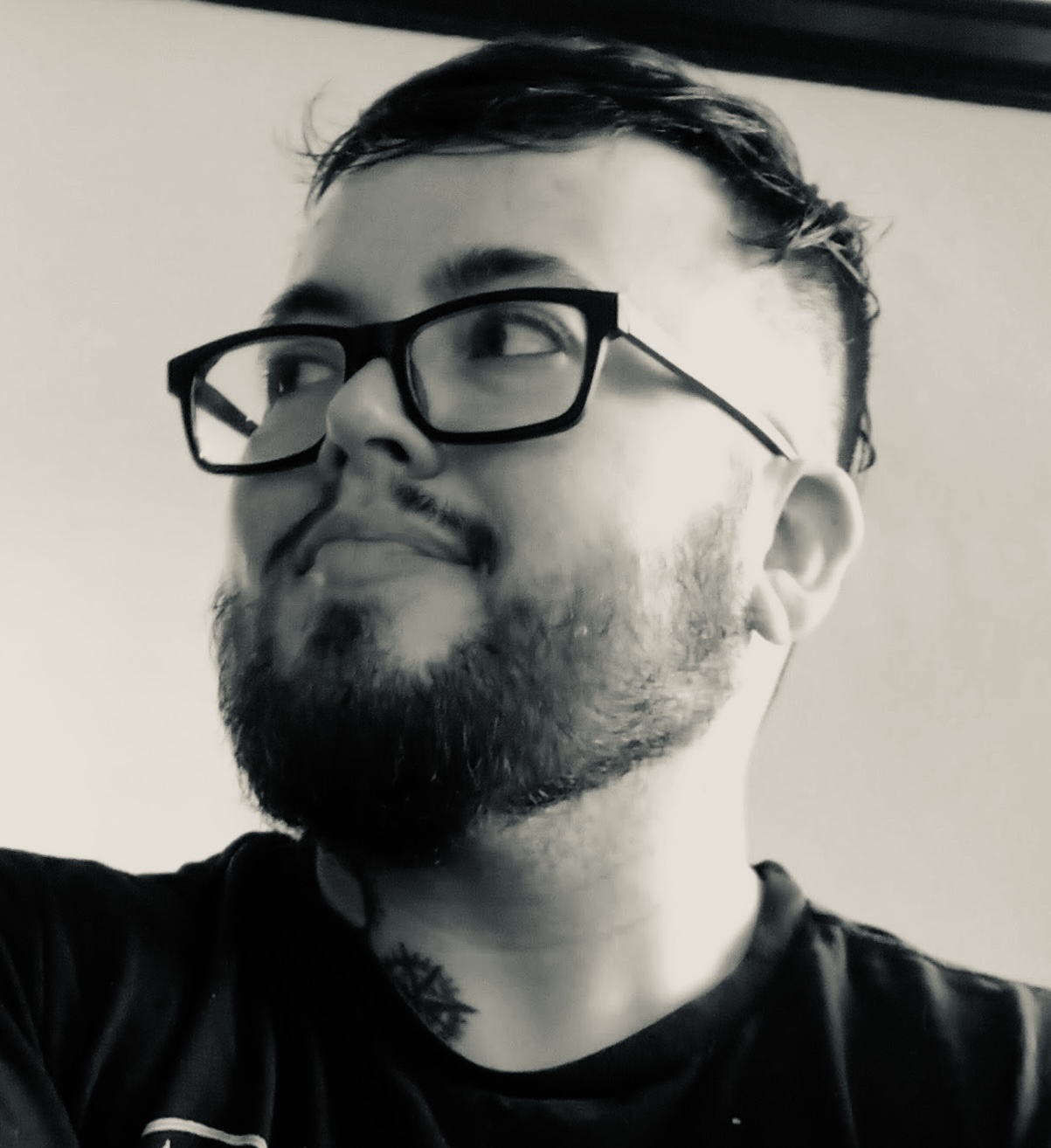
Millan Figueroa
Millan Figueroa
๐ Hi, Iโm @millan-figueroa ๐ Queer Chileno / Visionary entrepreneur / Designer / Hacktivist / Aspiring Developer / Founder of R A D I C A L B L O C K S / Tech Equity Advocate ๐ฑ Iโm currently learning full stack Javascript development, applying to Codesmith's Remote Immersive program. ๐๏ธ Iโm looking for other disruptors. ๐ซ How to reach me: millan.fig@gmail.com. Gracias :) ๐ F R E E P A L E S T I N E ! ๐