Understanding the Flow of Data in React Components

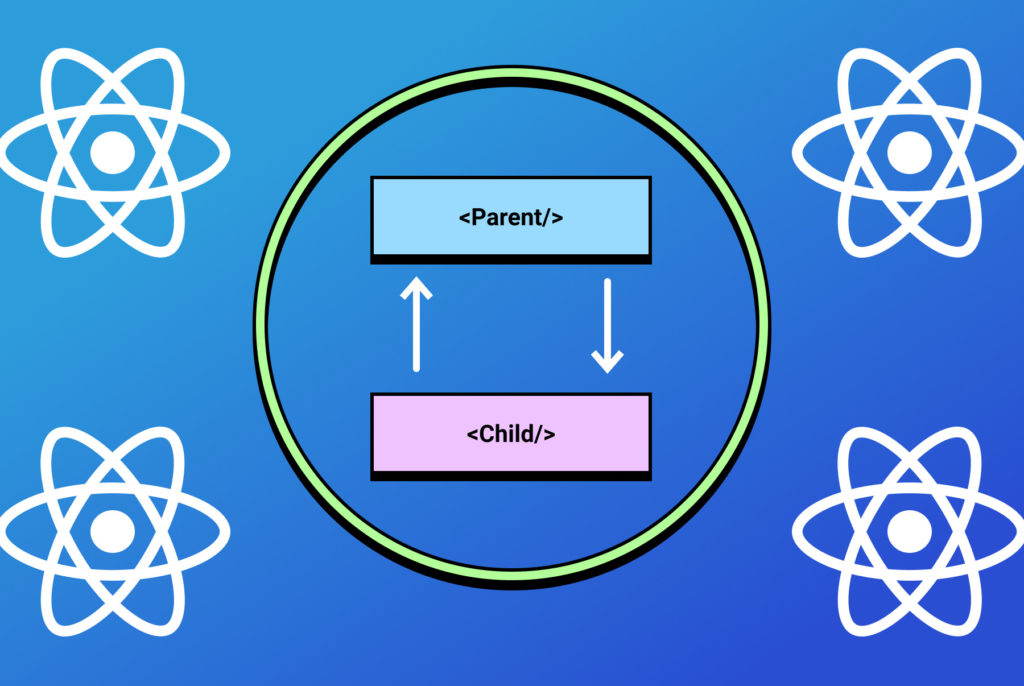
Summary: This article explores how data flows within React App components, covering the composition of components, passing data from parent to child components through props, and passing data from child to parent components via functions. Understanding these concepts is essential for building efficient and scalable React applications.
1. Composition of a React App
A React App is composed of components that build up the user Interface.
Components let you split the UI into independent, reusable pieces, and think about each piece in isolation.
Conceptually, components are like JavaScript functions. They accept arbitrary inputs (called “props”) and return React elements describing what should appear on the screen.
Simple React component example
// From React docs, click on link above.
// We discuss Props below
// The components below can be full pages or sections of the layout.
// functional component with props
// pass props to the component is not must, pass if used.
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
// class component accessing props
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
A single React section or page, let's say the Login Page, can comprise several components, each having a single responsibility as shown in the code example below.
NB: We shall be using Functional components
// Login Page Components
export defualt function Login() {
return (
<>
<NavBar /> // Navbar component
<MiniHero /> // Mini Hero component
<Map /> // Map component
<ContactForm /> // Contact Form Component
<Footer /> // Footer Component
</>
)
}
Components can be divided into UI components and Business Logic components.
UI components to handle the UI code (JSX), what the user sees. The login page would be the input fields and other elements without the logic of submitting the form.
Business logic components handle the business logic side of the UI components, adding functionality to what the user sees like the logic of submitting the login form data to the backend.
The two kinds of components above can be combined to form a whole component or page within your React App with all the design and the proposed functionality.
Each of these component divisions i.e. UI components or Business Logic components can be broken down into small sub-components with a specific responsibility or functionality, to build up a single component.
2. Composition of components in Reactjs
React components are composed of :
Parent components
Child / Children components
Parent Component: This component holds the child components and acts as an entry point for the child components it returns.
It is safe to say, by default components are parent components, and when used or returned in other functions / Classes or components, they become child components.
A parent component can influence the child component's reactions with props.
A component can be a parent and a child and vice versa at the same time.Below is an example of a Parent component with no child or children components.
function ParentComponent(){ return ( <> <h1>I am a parent Component </h1> </> ) }
Child / Children component: This is a React Component that is nested within the parent component and the parent component can influence its behaviors through props although it is an independent component with a certain role. Child components, on the one hand, can also be parent components i.e. a child component can have child components within it too.
Below is an example of a child component and it is returned in a parent component.
// Child Component
function ChildComponent(){
return (
<>
<h1>I am a child Component </h1>
</>
)
}
// Parent component
function ParentComponent(){
return (
<>
<h1>I am a parent Component </h1>
<childComponent /> // Rendering Child component
<childComponent /> // Rendering Child component again
</>
)
}
3. Data Flow within React components.
When dealing with data flow in components, it is worth noting that React components interact through Props. Data flows down to child components through props and data moves up to parent components through props as functions.
From Parent To Child
let's pass data from parent to child
// Parent Component Passing Props
// importing child component, assuming it's in same folder
import ChildComponent from "./ChildComponent"
export defualt function Parent() {
//declaring local variable called "name"
//and assigning "Geofrey" string to it.
let name = "Geofrey"
return (
// Rendering out the child component.
// Passing "Username" prop key and "name" variable from
// above to the child component as a value.
// Prop keys can be any name, for this case Username
<ChildComponent Username={name} />
)
}
From the snippet above, we have a Parent which is importing a ChildComponent and then rendering out the child component while passing props to it.
<ChildComponent Username={name} />
// Username = prop key
// name = prop value
Prop key can be called anything, for this case it is used Username
In the child component, we can access the props value through the prop key from the parent, for this case "Username".
Example of using props in the child component below
// Method One of accessing props in the child component.
export defualt ChildComponent(props) {
return (
<h1>Hello {props.Username}</h1>
)
}
// Method Two of accessing props, destructuring props
export defualt ChildComponent({ Username }) {
return (
<h1>Hello {Username}</h1>
)
}
From child to Parent.
Data moves from child to parent by props functions. A function is passed from the parent to the child and the child can pass the value to that function, and then the value is later passed back to the parent component.
function Parent() {
// Function that logs the message from the child component
const childsMessage = (message) => {
console.log(message)
}
// toParent = prop key
// childsName = prop value which is a function above.
return (
<ChildComponent toParent={ childsMessage } />
)
}
In Child Component
function ChildComponent({ toParent }) {
let message = "I am from a child component"
return (
// onClick has a function because we are passing "childsName" to
// "toParent" prop function.
<button onClick={ () => toParent(message) } >
Send Message To Parent
</button>
)
}
// if passing data to a prop function e.g toParent getting childsName
// then we need function in the onClick
onClick={ () => toParent(message) }
// if there is no pass data to the prop function, then we do not need
// the callback function in the onClick as below.
// We just call the prop function in the onClick.
onClick={ toParent }
4. Conclusion
Understanding the flow of data in React components is crucial for building robust and maintainable applications. As we've explored, data in React is primarily moved through props, with props allowing for data to be passed down from parent to child components and child-to-parent.
As you continue to develop your skills in React, remember that a clear understanding of data flow will not only make your code more predictable and easier to debug but also improve the overall performance and user experience of your applications. Keep experimenting, stay curious, and embrace the React philosophy of declarative programming to build efficient and effective web applications.
Thanks & Happy Coding
Subscribe to my newsletter
Read articles from isiagi geofrey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
